1. What are the differences between STM32F1 and F4?
2. Describe the STM32 startup process
3. Describe GPIO
APB1 is responsible for DA, USB, SPI, I2C, CAN, Serial 2345, ordinary TIM, PWR
4. UART
-
Question 1: Introduction to Serial Communication
Synchronous communication: I2C half-duplex, SPI full-duplex
Asynchronous communication: RS485 half-duplex, RS232 full-duplex
-
Question 2: Serial Port Configuration
The general steps for serial port settings can be summarized as follows:
(6) Enable serial port
(7) Write interrupt handler function
-
Question 3: Main Features of USART
(1) Full-duplex operation (independent receiving and sending data);
(2) In synchronous operation, can synchronize with master clock or slave clock;
(3) Independent high-precision baud rate generator, does not occupy timer/counter;
(4) Supports serial data frame structure with 5, 6, 7, 8, and 9 data bits, 1 or 2 stop bits;
(5) Hardware-supported parity bit generation and checking;
(6) Data overflow detection;
(7) Frame error detection;
(8) Includes noise filter and digital low-pass filter for detecting error start bits;
(9) Three completely independent interrupts, TX transmission complete, TX data register empty, RX reception complete;
(10) Supports multi-machine communication mode;
(11) Supports double-speed asynchronous communication mode.
Application scenarios: GPS, Bluetooth, 4G modules
5. I2C
/* STM32 I2C Fast Mode */#define I2C_Speed 400000/* Communication speed */I2C_InitStructure.I2C_ClockSpeed = I2C_Speed;
Software simulation: If no communication speed is set, how to calculate it?
Through the I2C bus bit delay function i2c_Delay:
static void i2c_Delay(void){ uint8_t i; /* The following time is obtained through the Anfu Lai AX-Pro logic analyzer test. When the CPU frequency is 72MHz, running in internal Flash, MDK project not optimized, 10 loop counts yield SCL frequency = 205KHz, 7 loop counts yield SCL frequency = 347KHz, SCL high level time 1.5us, SCL low level time 2.87us, 5 loop counts yield SCL frequency = 421KHz, SCL high level time 1.25us, SCL low level time 2.375us. IAR project compilation efficiency is high, cannot be set to 7 */ for (i = 0; i < 10; i++);}
6. SPI
-
Question 1: How many lines does SPI need?
-
Question 2: What are the four modes of SPI communication?
SPI has four working modes, differing in SCLK, specifically determined by CPOL and CPHA.
CPOL=0 means the clock level is low when idle, so when SCLK is active, it is high, referred to as active-high;
CPOL=1 means the clock level is high when idle, so when SCLK is active, it is low, referred to as active-low;
(2) CPHA: (Clock Phase), clock phase.
Phase corresponds to the data sampling at which edge (first edge or second edge), 0 corresponds to the first edge, and 1 corresponds to the second edge.
CPHA=0 indicates the first edge:
For CPOL=0, idle is low, the first edge transitions from low to high, so it is the rising edge;
For CPOL=1, idle is high, the first edge transitions from high to low, so it is the falling edge;
-
Question 3: How to determine which mode to use?
(1) First confirm the SCLK polarity required by the slave, whether it is low or high when idle, thus confirming CPOL as 0 or 1. From the schematic, we set the idle state of the serial synchronous clock to high, so we choose SPI_CPOL_High, which means CPOL is 1.
(2) Then confirm from the slave chip datasheet’s timing diagram whether the slave chip samples data on the falling edge or the rising edge of SCLK.
Translate: W25Q32JV is accessed via SPI-compatible bus, including four signals: serial clock (CLK), chip select (/CS), serial data input (DI), and serial data output (DO).
Standard SPI commands use the DI input pin to serially write commands, addresses, or data to the device on the rising edge of CLK. The DO output pin is used to read data or status from the device on the falling edge of CLK. Supports mode 0 (0,0) and 3 (1,1) for SPI bus operations.
Modes 0 and 3 focus on the normal state of the CLK signal when the SPI bus master is in standby mode and data is not being transmitted to the serial Flash. For mode 0, the CLK signal is usually low on the falling and rising edges/ CS. For mode 3, the CLK signal is usually high on the falling and rising edges of /CS.
Since the idle state of the serial synchronous clock is high, we choose the second edge transition, so we choose SPI_CPHA_2Edge, which means CPHA is 1.
That is, we choose mode 3 (1,1).
7. CAN
-
Question 1: Summary introduction of CAN
The CAN controller determines the bus level based on the potential difference between CAN_L and CAN_H. The bus level is divided into dominant level and recessive level, one of which is sent by changing the bus level to send a message to the receiver. Question 2: What are the steps for CAN initialization configuration?
(1) Configure the multiplexing function of related pins, enable CAN clock
(2) Set CAN working mode and baud rate (CAN initialization loopback mode, baud rate 500Kbps)
(3) Set filters Question 3: CAN data sending format CanTxMsg TxMessage;TxMessage.StdId=0x12; // Standard IdentifierTxMessage.ExtId=0x12; // Set Extended IdentifierTxMessage.IDE=CAN_Id_Standard; // Standard FrameTxMessage.RTR=CAN_RTR_Data; // Data FrameTxMessage.DLC=len; // Length of data to be sent 8 bytesfor(i=0;i < len;i++) TxMessage.Data[i]=msg[i]; // Data
8. DMA
-
Question 1: What is DMA?
Direct Memory Access (DMA) is used to provide high-speed data transfer between peripherals and memory, or between memory and memory. Data can be moved quickly through DMA without CPU intervention, saving CPU resources for other operations.
-
Question 2: How many modes of DMA transfer are there?
Application scenarios: GPS, Bluetooth, both use circular sampling, DMA_Mode_Circular mode.
A relatively important function, to get the current remaining data size, by subtracting the current remaining data size from the set receive buffer size, to get the current received data size.
9. Interrupts
-
Question 1: Describe the interrupt handling process?
(1) Initialize the interrupt, set the trigger mode to rising edge/falling edge/both edges. (2) Trigger the interrupt, enter the interrupt service function -
Question 2: How many external interrupts does the STM32 interrupt controller support?
The STM32 interrupt controller supports 19 external interrupts/event requests:
From the diagram, GPIO pins GPIOx.0~GPIOx.15 (x=A, B, C, D, E, F, G) correspond to interrupt lines 0 ~ 15.
EXTI15_10_IRQHandler
10. How many clock sources does STM32 have?
STM32 has 5 clock sources: HSI, HSE, LSI, LSE, PLL.
① HSI is a high-speed internal clock, RC oscillator, frequency is 8MHz, accuracy is not high.
② HSE is a high-speed external clock, can connect quartz/ceramic resonators, or connect external clock sources, frequency range is 4MHz~16MHz.
③ LSI is a low-speed internal clock, RC oscillator, frequency is 40kHz, providing low-power clock.
④ LSE is a low-speed external clock, connecting a quartz crystal with a frequency of 32.768kHz.
⑤ PLL is a phase-locked loop frequency multiplier output, its clock input source can be selected as HSI/2, HSE or HSE/2. The multiplication factor can be selected from 2 to 16, but its output frequency must not exceed 72MHz.
11. How to write tasks in RTOS? How to switch out this task?
Answer:
Not every task is executed in order of priority, but high-priority tasks monopolize execution; unless they voluntarily give up execution, low-priority tasks cannot preempt them. At the same time, high-priority tasks can reclaim CPU occupancy rights given to low-priority tasks. Therefore, it is important to insert wait delays between tasks in UCOS to allow low-priority tasks to execute.
12. What are the communication methods between tasks in UCOSII?
Answer:
Application example: Mutual exclusion semaphore
As a mutual exclusion condition, the semaphore is initialized to 1.
Goal: To send commands via the serial port, one must wait for the return of the “OK” character before sending the next command. Each task may use this sending function, and conflicts must be avoided!
Concept:
(1) The message queue is essentially an array of mailboxes
(2) Both tasks and interrupts can place a message in the queue, and tasks can retrieve messages from the message queue.
(3) Messages that enter the queue first are sent to tasks first (FIFO).
(4) Each message queue has a waiting list of tasks waiting for messages; if there are no messages in the queue, the waiting tasks are suspended until a message arrives.
Storing external events.
13. The project uses a custom protocol, what is the structure?
Answer:
Structure: Frame header (SDTC) + Frame length + Command + Serial number + Data + CRC check.
14. Differences between uCOSII and Linux?
Answer:
μC/OS-II is specifically designed for embedded applications in computers, featuring high execution efficiency, small space occupancy, excellent real-time performance, and strong scalability; the minimum kernel can be compiled to 2KB. μC/OS-II has been ported to almost all well-known CPUs.
Linux is free, safe, stable, and widely applied, with extensive applications in embedded systems, servers, and personal computers.
Both μC/OS-II and Linux are suitable for embedded use. However, μC/OS-II is specifically designed for embedded systems, resulting in higher running efficiency and less resource consumption.
Linux can be used on servers and has a high usage rate. Although Linux was not specifically developed for servers, its open-source nature allows for modifications, making the differences between the two minimal; the main distribution, Red Hat Linux, is widely used on servers.
15. Git Code Submission
Question: What is the process for Git code submission?
Answer:
1. Display modified files in the working path:
$ git status
$ cd -
$ git diff
$ git add .
$ git commit -s
In addition, it should be noted:
Note: Each folder must be submitted separately.
6. View submitted code
$ tig .
7. Do not modify published submission records! (Use this for future submissions)
$ git commit --amend
8. Push to the server
$ git push origin HEAD:refs/for/master
16. Comparison of uCOSII, uCOSIII, and FreeRTOS
-
Question 1: Comparison of the three?
Answer:
Comparison of uCOSII and FreeRTOS:
(4) Theoretically, FreeRTOS can manage more than 64 tasks, while uCOSII can manage only 64.
Comparison of uCOSII and uCOSIII:
So what are the differences from μC/OS-II to μC/OS-III? There are significant changes.
One is that previously there were only 0-63 priority levels, and priorities could not be duplicated. Now, several tasks can use the same priority, and time-slicing scheduling is supported within the same priority.
The second is that users can dynamically configure real-time operating system kernel resources such as tasks, task stacks, semaphores, event flag groups, message queues, message counts, mutex semaphores, memory block divisions, and timers during program execution, allowing users to avoid resource allocation issues during program compilation.
Improvements have also been made in resource reuse.In μC/OS-II, the maximum number of tasks was 64, but after version 2.82, it increased to 256. In μC/OS-III, users can define any number of tasks, semaphores, mutex semaphores, event flags, message lists, timers, and any allocated memory block capacity, limited only by the amount of RAM available on the user’s CPU.This is a significant expansion.
(Question: Teacher Shao, is this number fixed at startup or can it be defined freely after startup?) It can be freely defined during configuration, as long as your RAM is large enough.
The fourth point is that many new features have been added, which were not present in μC/OS-II.
17. Low Power Modes
-
Question 1: How many types of low-power modes are there? What are the wake-up methods?
Answer:
18. Internet of Things Architecture
-
Question 1: How many layers does the Internet of Things architecture have? What functions does each layer perform?
Answer:
Divided into three layers, the Internet of Things can be divided into perception layer, network layer, and application layer.
(1) Perception Layer: Responsible for information collection and information transmission between objects, including technologies for information collection such as sensors, barcodes and QR codes, RFID technology, audio and video, and other multimedia information.
Information transmission includes short and long-distance data transmission technologies, self-organizing networking technologies, collaborative information processing technologies, and middleware technologies for sensor networks.
The perception layer is the core capability for achieving comprehensive perception in the Internet of Things, and it includes critical technologies, standardization aspects, and industrialization aspects that urgently need breakthroughs, focusing on achieving more accurate and comprehensive perception capabilities while addressing issues of low power consumption, miniaturization, and low cost.
(2) Network Layer: Utilizes wireless and wired networks to encode, authenticate, and transmit collected data. The widespread mobile communication network is the infrastructure for achieving the Internet of Things, and it is the most standardized, industrialized, and mature part of the three layers of the Internet of Things, focusing on optimizing and improving the characteristics of Internet of Things applications to form a collaborative perception network.
(3) Application Layer: Provides rich applications based on the Internet of Things, which is the fundamental goal of the Internet of Things development. It combines Internet of Things technology with industry information needs to realize a wide range of intelligent application solutions, focusing on industry integration, information resource development and utilization, low-cost high-quality solutions, information security guarantees, and the development of effective business models.
19. Memory Management
-
Question 1: What methods are there for memory management in UCOS?
Answer:
The system manages memory partitions through memory control blocks associated with memory partitions.
Dynamic memory management functions include:
Release memory block function OSMemPut();
20. What are the task states in UCOS? What is the relationship diagram between task states?
Answer:
There are 5 states:
Sleep state, Ready state, Running state, Waiting state (waiting for a certain event to occur), and Interrupt service state.
UCOSII task’s 5 state transition relationships:
21. ADC
22. System Clock
-
Briefly describe the basic process for setting the system clock
(4) Enable PLL, switch the system clock source to PLL.
23. HardFault_Handler Processing
-
Question 1: What are the causes?
(1) Array out of bounds operation; (2) Memory overflow, out-of-bounds access; (3) Stack overflow, program runaway; (4) Interrupt handling errors;
-
Question 2: How to handle it?
(1) Find the address remapping of HardFault_Handler in startup_stm32f10x_cl.s, and rewrite it to jump to the HardFaultHandle function.
(2) Print and check the registers R0, R1, R2, R3, R12, LR, PC, PSR.
(3) Check the Fault status register group (SCB->CFSR and SCB->HFSR)
24. TTS Speech Synthesis Method
-
Question 1: What method does sim7600 TTS speech use?
Answer:
(1) Use unicode encoding to synthesize sound
AT+CTTS=1,”6B228FCE4F7F75288BED97F3540862107CFB7EDF”
The content is “Welcome to use the voice synthesis system,” the module sends and receives Chinese messages in unicode encoding, so it is easy to read the messages aloud;
(2) Directly input text, ordinary characters use ASCII code, Chinese characters use GBK encoding.
AT+CTTS=2,”Welcome to use the voice synthesis system”
25. Timer
-
Given that the system clock of STM32 is 72MHz, how to set the relevant registers to achieve a 20ms timer?
Answer:
By using SysTick_Config(SystemCoreClock / OS_TICKS_PER_SEC))//1ms timer
Where:
uint32_t SystemCoreClock = SYSCLK_FREQ_72MHz; /*!< System Clock Frequency (Core Clock) */#define SYSCLK_FREQ_72MHz 72000000#define OS_TICKS_PER_SEC 1000 /* Set the number of ticks in one second
If 20ms is needed, a global variable can be set, initialized to 20, so that every SysTick interrupt reduces this global variable by 1; when it reaches 0, it means the SysTick interrupt has occurred 20 times, and the time is: 1ms * 20 = 20ms, thus achieving a 20ms timer.
26. State Machine
-
Question: What state machine is used?
Answer:
Assume the state transitions of the state machine are shown in the table below:
Implementation: (using switch statement)
// Write horizontallyvoid event0func(void){ switch(cur_state) { case State0: action0; cur_state = State1; break; case State1: action1; cur_state = State2; break; case State2: action1; cur_state = State0; break; default:break; }}void event1func(void){ switch(cur_state) { case State0: action4; cur_state = State1; break; default:break; }}void event2func(void){ switch(cur_state) { case State0: action5; cur_state = State2; break; case State1: action6; cur_state = State0; break; default:break; }}
27. Device Selection
Comparison of STM32F407 VS STM32F103 main functions and resources?
Answer:
Original text: juyou.blog.csdn.net/article/details/116021595
The article is sourced from the internet, and the copyright belongs to the original author. If there is any infringement, please contact for deletion.
END
This issue’s discussion:
1. What level is your salary in your city? Is it enough?
2. Some people feel that working with hardware and embedded systems in first-tier cities at 20K is not as good as 15K in second-tier cities. What do you think?
All participants will receive: 20 E coins
Excellent answers will receive: 300 E coins
Activity time: 2023/07/25 – 2023/08/31
Welcome to join the engineer workplace group
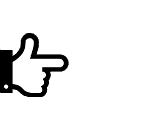