Using PyInstaller to Package Python Programs!
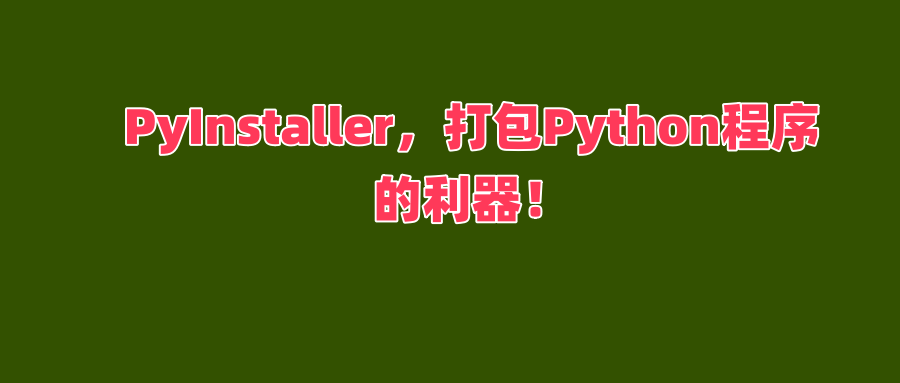
Hello everyone, it’s Guoguo again!
Today, I want to introduce you to a particularly useful tool – PyInstaller.
Have you ever thought about packaging your Python program into an executable file (.exe) so that friends who don’t have Python installed can run it?
PyInstaller is here to help you achieve that wish!
What is PyInstaller?
PyInstaller is a super handy tool for packaging Python programs.
It can package your Python scripts, along with all the dependent library files, into a standalone executable file.
This way, other users do not need to install the Python environment; they can just double-click to run your program!
Installing PyInstaller
We need to install PyInstaller.
Open the command line and enter the following command:
pip install pyinstaller
Once installed, we can start our packaging journey!
Basic Packaging Operations
Let’s start with a simple example.
Suppose we have a program called hello.py:
import time
def main():
print("Welcome to my first packaged program!")
time.sleep(1)
name = input("Please enter your name:")
print(f"Hello, {name}! Nice to meet you!")
if __name__ == '__main__':
main()
To package this program, simply navigate to the directory where hello.py is located in the command line and enter:
pyinstaller -F hello.py
Tip: The -F parameter indicates that a single executable file will be generated. After packaging, the executable file will be located in the dist directory.
Common Packaging Parameters
Let me introduce a few commonly used packaging parameters:
- -w: For windowed programs, does not display the console window
pyinstaller -F -w myapp.py
- -i icon.ico: Set the program icon
pyinstaller -F -i myicon.ico hello.py
- –name: Specify the name of the generated executable file
pyinstaller -F --name MyProgram hello.py
Note: Make sure all referenced modules are correctly installed when packaging, otherwise it may fail to package!
Packaging Considerations
- Path Issues: When the packaged program accesses resource files, be careful to use relative or absolute paths.
- Hidden Imports: Some libraries may need to be manually specified for import. For example:
- File Size: The packaged file may be relatively large because it includes all necessary dependencies.
pyinstaller -F --hidden-import pandas hello.py
Practical Exercise
Try packaging a simple calculator program:
import tkinter as tk
from tkinter import messagebox
class Calculator:
def __init__(self):
self.window = tk.Tk()
self.window.title("Guoguo Calculator")
self.window.geometry("200x150")
self.num1 = tk.Entry(self.window)
self.num1.pack()
self.num2 = tk.Entry(self.window)
self.num2.pack()
self.btn = tk.Button(self.window, text="Calculate", command=self.calculate)
self.btn.pack()
self.window.mainloop()
def calculate(self):
try:
result = float(self.num1.get()) + float(self.num2.get())
messagebox.showinfo("Result", f"The result is: {result}")
except:
messagebox.showerror("Error", "Please enter valid numbers!")
if __name__ == '__main__':
Calculator()
Save the above code as calculator.py, then use the following command to package it:
pyinstaller -F -w calculator.py
Summary
Today we learned:
- Basic usage of PyInstaller
- Common packaging parameters
- Considerations when packaging
- Example of packaging in a real project
Friends, that’s all for today’s Python learning journey! Remember to code actively, and feel free to ask Guoguo any questions in the comments section. Wish everyone happy learning and continuous improvement in Python!