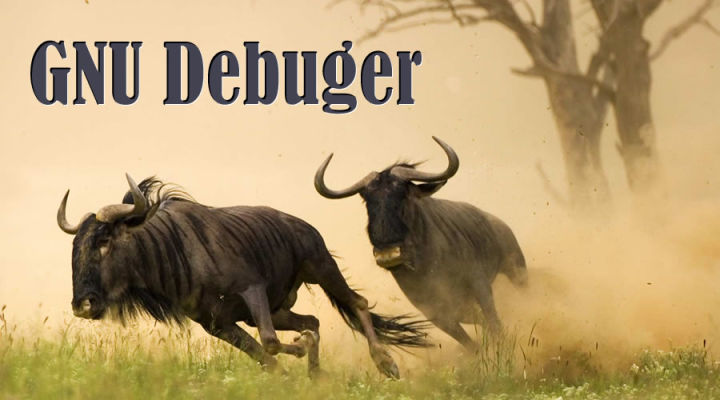
Table of Contents
b
i b
dis
d
break
, but will be automatically deleted after the program stops for the first times
n
fin
u
c
p
h
r
bt
break
Use the break
command (abbreviated b
) to set breakpoints.
Usage:
break
when no parameters are provided, sets a breakpoint at the next instruction executed in the selected stack frame.break <function-name>
sets a breakpoint at the entry of the function body; in C++, you can specify the function name using class::function
or function(type, ...)
format.break <line-number>
sets a breakpoint at the start of the specified line in the current source file.break -N
break +N
sets a breakpoint at the start of the line before or after the current source line, where N
is a positive integer.break <filename:linenum>
sets a breakpoint at line linenum
of the source file filename
.break <filename:function>
sets a breakpoint at the entry of the function function
in the source file filename
.break <address>
sets a breakpoint at the address of the program instruction.break ... if <cond>
sets a conditional breakpoint; ...
represents one of the above parameters (or no parameters), and cond
is the condition expression that pauses program execution only when cond
is non-zero.See official documentation[1] for details.
info breakpoints
View the list of breakpoints, watchpoints, and catchpoints.
Usage:
info breakpoints [list...]
info break [list...]
list...
is used to specify several breakpoint numbers (optional), which can be 2
, 1-3
, 2 5
, etc.disable
Disable some breakpoints. The parameters are space-separated breakpoint numbers. To disable all breakpoints, do not add parameters.
Disabled breakpoints are not forgotten, but will not take effect until re-enabled.
Usage:
disable [breakpoints] [list...]
breakpoints
is a subcommand of disable
(optional), and list...
is described in info breakpoints
.See official documentation[2] for details.
enable
Enable some breakpoints. Provide breakpoint numbers (space-separated) as parameters. Without parameters, all breakpoints are enabled.
Usage:
enable [breakpoints] [list...]
enables the specified breakpoints (or all defined breakpoints).enable [breakpoints] once list...
temporarily enables the specified breakpoints. GDB will disable these breakpoints immediately after stopping your program.enable [breakpoints] delete list...
enables the specified breakpoints once and then deletes them. Once your program stops, GDB will delete these breakpoints. This is equivalent to breakpoints set with tbreak
.breakpoints
is described in disable
.
See official documentation[3] for details.
clear
Clear breakpoints at the specified line or function. The parameters can be a line number, function name, or *
followed by an address.
Usage:
clear
without parameters clears all breakpoints in the source line currently being executed in the selected stack frame.clear <function>
, clear <filename:function>
deletes any breakpoints set at the entry of the named function.clear <linenum>
, clear <filename:linenum>
deletes any breakpoints set in the code at the specified line number in the specified file.clear <address>
clears the breakpoint at the address of the specified program instruction.See official documentation[4] for details.
delete
Delete some breakpoints or automatically displayed expressions. The parameters are space-separated breakpoint numbers. To delete all breakpoints, do not add parameters.
Usage: delete [breakpoints] [list...]
See official documentation[5] for details.
tbreak
Set a temporary breakpoint. The parameters are in the same form as break
.
Except that the breakpoint is temporary, other aspects are the same as break
, so it will be deleted when hit.
See official documentation[6] for details.
watch
Set a watchpoint for an expression.
Usage: watch [-l|-location] <expr>
The watchpoint will pause program execution whenever the value of an expression changes.
If -l
or -location
is provided, it will evaluate expr
and observe the memory it points to. For example, watch *(int *)0x12345678
will observe a 4-byte area at the specified address (assuming int occupies 4 bytes).
See official documentation[7] for details.
step
Step through the program until reaching a different source line.
Usage: step [N]
The parameter N
indicates to execute N
times (or until the program stops for another reason).
Warning: If the step
command is used in a function compiled without debugging information, execution will continue until control reaches a function with debugging information. Similarly, it will not enter functions compiled without debugging information.
To execute a function without debugging information, use the stepi
command, see below.
See official documentation[8] for details.
reverse-step
Step backwards through the program until reaching the beginning of another source line.
Usage: reverse-step [N]
The parameter N
indicates to execute N
times (or until the program stops for another reason).
See official documentation[9] for details.
next
Step through the program, finishing subroutine calls.
Usage: next [N]
Unlike step
, if the current source line calls a subroutine, this command will not enter the subroutine, treating it as a single source line and continuing execution.
See official documentation[10] for details.
reverse-next
Step backwards through the program, finishing subroutine calls.
Usage: reverse-next [N]
If the source line to be executed calls a subroutine, this command will not enter the subroutine, treating the call as a single instruction.
The parameter N
indicates to execute N
times (or until the program stops for another reason).
See official documentation[11] for details.
return
You can use the return
command to cancel the execution of a function call. If you provide an expression parameter, its value is used as the return value of the function.
Usage: return <expression>
will use the value of expression
as the return value of the function and make the function return directly.
See official documentation[12] for details.
finish
Execute until the selected stack frame returns.
Usage: finish
will print the returned value and place it in the value history.
See official documentation[13] for details.
until
Execute until the program reaches the current line after the current line in the current stack frame (with the same parameters as break[14] command). This command is used to bypass a loop by executing multiple times.
Usage: until <location>
or u <location>
continues running the program until reaching the specified location or returning from the current stack frame.
See official documentation[15] for details.
continue
Continue running the program being debugged after a signal or breakpoint.
Usage: continue [N]
If starting from a breakpoint, you can use the number N
as a parameter, which sets the ignore count for that breakpoint to N - 1
(so that the breakpoint will not interrupt before the N
th time it is reached). If non-stop mode is enabled (check with show non-stop
), only the current thread will continue; otherwise, all threads in the program will continue.
See official documentation[16] for details.
Evaluate and print the value of expression EXP. Accessible variables are from the lexical environment of the selected stack frame, as well as all variables in the global scope or throughout the file.
Usage:
print [expr]
or print /f [expr]
expr
is an expression (in the source language).By default, the value of expr
is printed in a format suitable for its data type; you can choose a different format by specifying /f
, where f
is a letter specifying the format; see Output Formats[17] for details.
If expr
is omitted, GDB will display the last value again.
To print a struct variable in a format with one member per line and indentation, use the command set print pretty on
; to cancel this, use set print pretty off
.
You can use the command show print
to see all print settings.
See official documentation[18] for details.
x
Examine memory.
Usage: x/nfu <addr>
or x <addr>
where n
, f
, and u
are optional parameters used to specify how to display memory and how to format it. addr
is the expression for the address to start displaying memory.
n
is the repeat count (default is 1), specifying how many units (as specified by u
) of memory values to display.
f
is the display format (initial default is x
), with display formats being one of print('x','d','u','o','t','a','c','f','s')
formats, plus i
(machine instructions).
u
is the unit size, where b
indicates single byte, h
indicates double byte, w
indicates four bytes, and g
indicates eight bytes.
For example:
x/3uh 0x54320
means to display 3 memory values starting from address 0x54320 in unsigned decimal integer format, with double bytes as the unit.
x/16xb 0x7f95b7d18870
means to display 16 memory values starting from address 0x7f95b7d18870 in hexadecimal integer format, with single bytes as the unit.
See official documentation[19] for details.
display
Print the value of expression EXP each time the program pauses.
Usage: display <expr>
, display/fmt <expr>
or display/fmt <addr>
fmt
is used to specify the display format, similar to print[20] command’s /f
.
For formats i
or s
, or including unit size or unit count, add the expression addr
as the memory address to check each time the program stops.
See official documentation[21] for details.
info display
Print the list of automatically displayed expressions, each with an item number but not showing their values.
Includes disabled expressions and expressions that cannot be displayed immediately (currently unavailable automatic variables).
undisplay
Cancel the automatic display of certain expressions when the program pauses. The parameters are the numbers of the expressions (use info display
to query the numbers). Leaving out parameters means canceling all automatically displayed expressions.
delete display
has the same effect as this command.
disable display
Disable the automatic display of certain expressions when the program pauses. Disabled display items will not be printed automatically but will not be forgotten. They may be enabled again later.
The parameters are the numbers of the expressions (use info display
to query the numbers). Leaving out parameters means disabling all automatically displayed expressions.
enable display
Enable the automatic display of certain expressions when the program pauses.
The parameters are the numbers of the expressions to be re-displayed (use info display
to query the numbers). Leaving out parameters means enabling all automatically displayed expressions.
help
Print the command list.
You can use help
without parameters (abbreviated as h
) to display a brief list of command category names.
Using help <class>
you can get a list of various commands in that class. Use help <command>
to show how to use that command.
See official documentation[22] for details.
attach
Attach to a process or file outside of GDB. This command can take a process ID or device file as parameters.
For process IDs, you must have permission to send signals to the process and must have a valid uid equal to that of the debugger.
Usage: attach <process-id>
GDB will pause the process after scheduling debugging for the specified process.
You can use GDB commands to inspect and modify the attached process, whether attached via the attach
command or started via the run
command.
See official documentation[23] for details.
run
Start the program being debugged.
You can directly specify parameters or set (required) parameters with set args[24].
For example: run arg1 arg2 ...
is equivalent to
set args arg1 arg2 ...
run
Input and output redirection is also allowed using >
, <
, or >>
.
See official documentation[25] for details.
backtrace
Print overall stack frame information.
bt
prints overall stack frame information, one line per stack frame.bt n
is similar to the above but prints only the innermost n stack frames.bt -n
is similar to the above but prints only the outermost n stack frames.bt full n
is similar to bt n
, but also prints the values of local variables.where
and info stack
(abbreviated info s
) are aliases for backtrace
. The call stack information is similar to the following:
(gdb) where
#0 vconn_stream_run (vconn=0x99e5e38) at lib/vconn-stream.c:232
#1 0x080ed68a in vconn_run (vconn=0x99e5e38) at lib/vconn.c:276
#2 0x080dc6c8 in rconn_run (rc=0x99dbbe0) at lib/rconn.c:513
#3 0x08077b83 in ofconn_run (ofconn=0x99e8070, handle_openflow=0x805e274 <handle_openflow>) at ofproto/connmgr.c:1234
#4 0x08075f92 in connmgr_run (mgr=0x99dc878, handle_openflow=0x805e274 <handle_openflow>) at ofproto/connmgr.c:286
#5 0x08057d58 in ofproto_run (p=0x99d9ba0) at ofproto/ofproto.c:1159
#6 0x0804f96b in bridge_run () at vswitchd/bridge.c:2248
#7 0x08054168 in main (argc=4, argv=0xbf8333e4) at vswitchd/ovs-vswitchd.c:125
See official documentation[26] for details.
ptype
Print the definition of type TYPE.
Usage: ptype[/FLAGS] TYPE-NAME | EXPRESSION
The parameters can be type names defined by typedef
, or struct STRUCT-TAG
, or class CLASS-NAME
, or union UNION-TAG
, or enum ENUM-TAG
.
Find the name based on the lexical context of the selected stack frame.
A similar command is whatis
, which differs in that whatis
does not expand data types defined by typedef
, while ptype
does expand them, as illustrated below:
/* Type declaration and variable definition */
typedef double real_t;
struct complex {
real_t real;
double imag;
};
typedef struct complex complex_t;
complex_t var;
real_t *real_pointer_var;
These two commands give the following output:
(gdb) whatis var
type = complex_t
(gdb) ptype var
type = struct complex {
real_t real;
double imag;
}
(gdb) whatis complex_t
type = struct complex
(gdb) whatis struct complex
type = struct complex
(gdb) ptype struct complex
type = struct complex {
real_t real;
double imag;
}
(gdb) whatis real_pointer_var
type = real_t *
(gdb) ptype real_pointer_var
type = double *
See official documentation[27] for details.
References
Translator: robot527 Proofread by: mudongliang, wxy
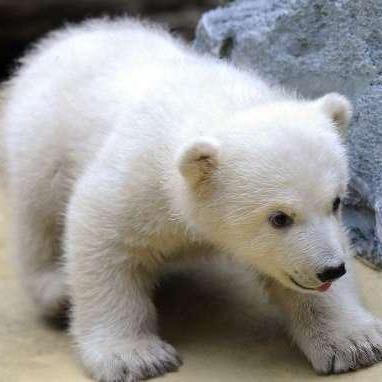
Recommended Articles
< Swipe left and right to view related articles >
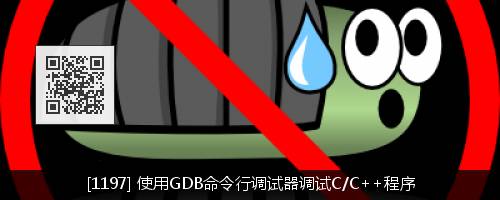
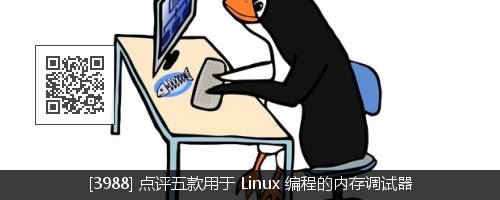
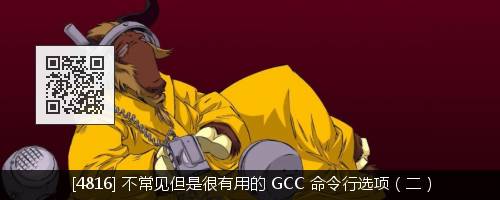
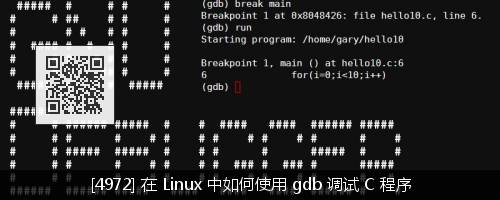
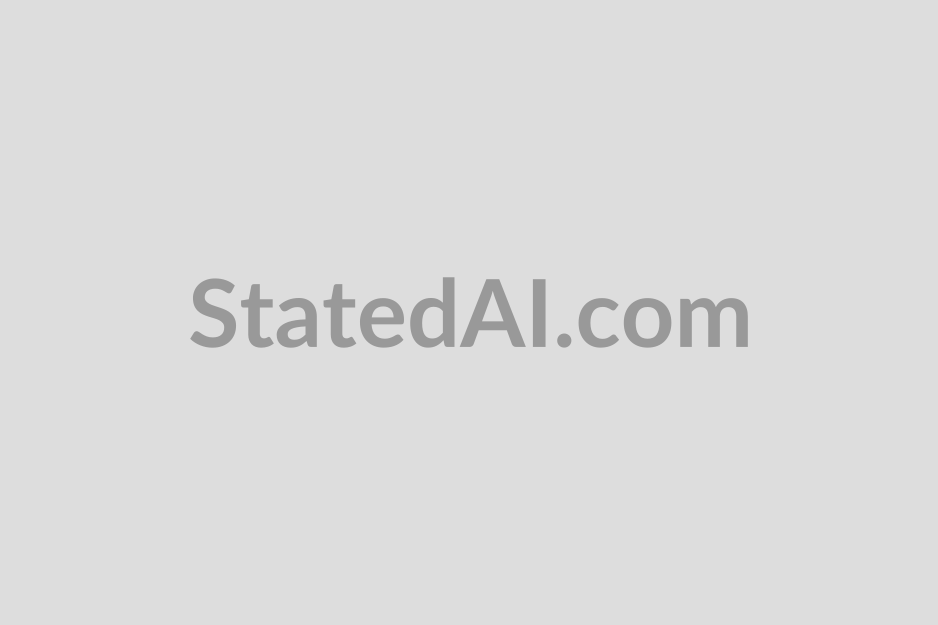
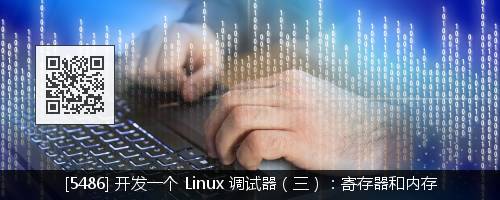
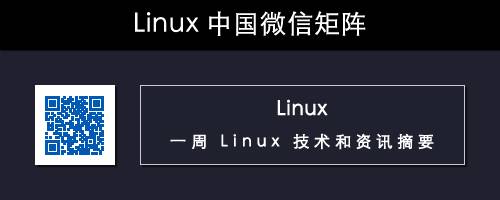
Click the image, enter the article ID, or scan the QR code to go directly