Today, Pi Zi Heng will share with you the three implementations of critical section protection in Cortex-M bare metal.
Those who have worked with embedded systems and RTOS are likely familiar with the function codes OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL(). In RTOS, multi-tasking (process) handling often occurs, and in some situations, certain special operations (such as Flash erasing under XIP or switching to low-power mode) cannot be interrupted at will, or some shared data areas cannot be accessed in an unordered manner (Task A is reading while Task B is writing). This is where critical section protection strategies come into play.
The so-called critical section protection strategy means that multiple tasks must mutually exclude access to critical hardware or software resources in the system. In an RTOS environment, there are ready-made critical section protection interface functions, but there is also a need for such protection in bare metal systems. In bare metal systems, critical section protection is mainly related to global interrupt control. Pi Zi Heng previously wrote an article on the general triple interrupt control design in embedded MCUs, where the third and highest level of interrupt control is the system global interrupt control. Today, I will introduce three methods of critical section protection starting from this system global interrupt control:
1. Critical Section Protection Test Scenarios
There are basically two scenarios for critical section protection testing. The first scenario is that multiple protected tasks are unrelated and do not nest. As shown in the code below, task1 and task2 are protected in sequence, so the enter_critical() and exit_critical() critical section protection functions are always executed in strict pairs:
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected task 1
do_task1();
// Exit critical section
exit_critical();
// Enter critical section
enter_critical();
// Perform protected task 2, unrelated to task 1
do_task2();
// Exit critical section
exit_critical();
}
The second scenario is that multiple tasks may be related and there could be nesting situations. As shown in the code below, task2 is a sub-task of task1. In this case, you will find that enter_critical() is executed twice before exit_critical() is executed twice. It is important to note that even though sub-task task3 inside task1 is not actively protected like sub-task task2, it should still be protected because the main task task1 is protected as a whole.
void do_task1(void)
{
// Enter critical section
enter_critical();
// Perform protected task 2, a sub-task of task 1
do_task2();
// Exit critical section
exit_critical();
// Perform task 3
do_task3();
}
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected task 1
do_task1();
// Exit critical section
exit_critical();
}
2. Three Implementations of Critical Section Protection
The above critical section protection test scenarios are quite clear. Now let’s move on to the implementation of the enter_critical() and exit_critical() critical section protection functions:
2.1 Basic Approach
First is a very basic approach, which is simply a wrapper around the system global interrupt control functions __disable_irq() and __enable_irq(). Returning to the previous test scenario, this implementation can handle the protection of non-nested tasks well, but it fails for nested task protection. In the previous test code, task3 should also be protected, but it is not because the exit_critical() directly enables global interrupts right after task2.
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
}
void exit_critical(void)
{
// Enable global interrupts
__enable_irq();
}
2.2 Improved Approach
Can the basic approach be improved? Of course! We just need to add a global variable s_lockObject to keep track of the current count of how many times the critical section has been entered, as shown in the code below. Each time enter_critical() is called, it will disable global interrupts (ensuring that the critical section is protected) and increment the count. When exit_critical() is called, it will only enable global interrupts if the current count is 1 (i.e., not in a nested critical section). Otherwise, it simply decrements the count. The improved implementation can obviously protect task3 in the previous test code.
static uint32_t s_lockObject;
void init_critical(void)
{
__disable_irq();
// Reset counter
s_lockObject = 0;
__enable_irq();
}
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
// Increment counter
++s_lockObject;
}
void exit_critical(void)
{
if (s_lockObject <= 1)
{
// Only enable global interrupts if counter is not greater than 1, and reset counter
s_lockObject = 0;
__enable_irq();
}
else
{
// If counter is greater than 1, just decrement the counter
--s_lockObject;
}
}
2.3 Ultimate Approach
While the improved approach solves the problem of nested critical section task protection, it adds a global variable and an initialization function, making the implementation less elegant. Moreover, global variables in embedded systems can easily be tampered with, posing certain risks. Is there a better implementation? Certainly! This can be achieved by leveraging the special mask register PRIMASK of the Cortex-M processor core. Below is the PRIMASK register bit definition (taken from the ARMv7-M manual), where only the lowest bit PM is valid. When PRIMASK[PM] is 1, global interrupts are disabled (raising execution priority to 0x0/0x80); when PRIMASK[PM] is 0, global interrupts are enabled (no effect on execution priority).
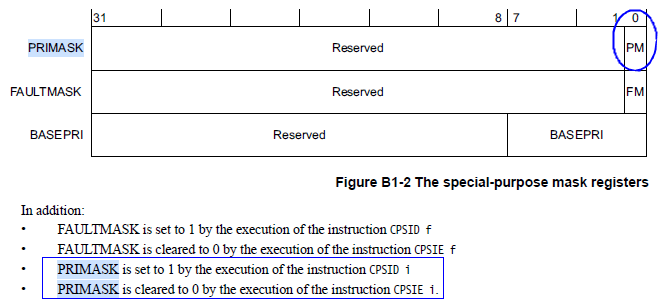
At this point, you should understand that the functions __disable_irq() and __enable_irq() actually operate on the PRIMASK register. Since the PRIMASK register also retains the state of the global interrupt switch, we can use the value of PRIMASK to replace the global variable s_lockObject in the improved approach. The code implementation is as follows:
uint32_t enter_critical(void)
{
// Save current PRIMASK value
uint32_t regPrimask = __get_PRIMASK();
// Disable global interrupts (actually set PRIMASK to 1)
__disable_irq();
return regPrimask;
}
void exit_critical(uint32_t primask)
{
// Restore PRIMASK
__set_PRIMASK(primask);
}
Since the prototypes of enter_critical() and exit_critical() have changed, their usage also needs to be modified accordingly:
void critical_section_test(void)
{
// Enter critical section
uint32_t primask = enter_critical();
// Perform protected task
do_task();
// Exit critical section
exit_critical(primask);
// ...
}
Appendix: PRIMASK Register Setting Functions Implementation in Various IDEs
//////////////////////////////////////////////////////
// Implementation in IAR environment (see cmsis_iccarm.h)
#define __set_PRIMASK(VALUE) (__arm_wsr("PRIMASK", (VALUE)))
#define __get_PRIMASK() (__arm_rsr("PRIMASK"))
//////////////////////////////////////////////////////
// Implementation in Keil environment (see cmsis_armclang.h)
__STATIC_FORCEINLINE void __set_PRIMASK(uint32_t priMask)
{
__ASM volatile ("MSR primask, %0" : : "r" (priMask) : "memory");
}
__STATIC_FORCEINLINE uint32_t __get_PRIMASK(void)
{
uint32_t result;
__ASM volatile ("MRS %0, primask" : "=r" (result) );
return(result);
}
Thus, Pi Zi Heng has completed the introduction of the three implementations of critical section protection in Cortex-M bare metal. Where’s the applause~~~

1. Huawei HarmonyOS Certification Announced: Three Levels
2. Summary of C Language Programming Points in Embedded Development!
3. After the heated discussion, let’s analyze the future of Harmony objectively!
4. The Love-Hate Relationship Between ARM and RISC-V~
5. How Does Code Control Hardware?
6. A Gentle Breeze – Introduction to the New IoT Operating System Zephyr
Disclaimer: This article is a network repost, and the copyright belongs to the original author. If there are any copyright issues, please contact us, and we will confirm the copyright based on the materials you provide and pay remuneration or delete the content.