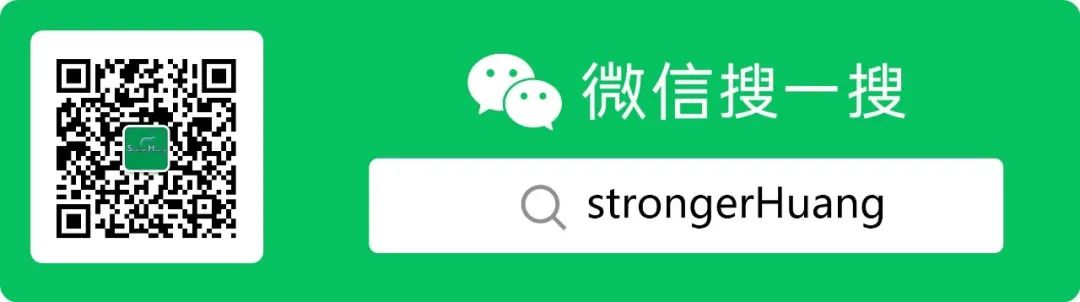
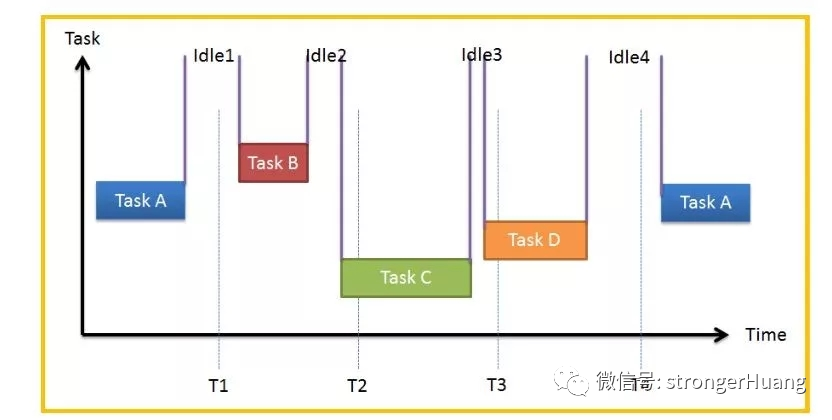
Logical Systems
1. Polling System
A polling system initializes the relevant hardware first and then lets the main program run in an infinite loop, sequentially doing various tasks. The pseudo code is illustrated in the code listing:
int main(void)
{
/* Hardware related initialization */
HardWareInit();
/* Infinite loop */
for (;;) {
/* Handle task 1 */
DoSomething1();
/* Handle task 2 */
DoSomethingg2();
/* Handle task 3 */
DoSomethingg3();
}
}
A polling system is a very simple software structure, usually only suitable for tasks that can be completed by sequential code execution without needing external events to drive them. In code listing 1-1, if only LED toggling, serial output, or LCD display operations are needed, then using a polling system would be perfect. However, if external signal events such as button operations are added to simulate emergency alarms, the real-time response capability of the entire system will be compromised.
Assuming DoSomethingg3 is button scanning, when an external button is pressed, it acts like an alarm, requiring an immediate response and urgent handling. However, at that moment, the program is executing DoSomethingg1, which takes a long time to complete. If it takes so long that the button is released before DoSomethingg1 finishes executing, the event will be missed when it finally executes DoSomethingg3. Therefore, polling systems are only suitable for sequentially executed functional code; when external events drive the system, real-time performance decreases.
2. Foreground-Background System
Compared to the polling system, the foreground-background system adds interrupts. The response to external events is completed in the interrupt, and the event handling is still completed in the polling system. Here, we refer to the interrupt as the foreground, and the infinite loop in the main function as the background. The pseudo code is illustrated in the code listing:
int flag1 = 0;
int flag2 = 0;
int flag3 = 0;
int main(void)
{
/* Hardware related initialization */
HardWareInit();
/* Infinite loop */
for (;;) {
if (flag1) {
/* Handle task 1 */
DoSomething1();
}
if (flag2) {
/* Handle task 2 */
DoSomethingg2();
}
if (flag3) {
/* Handle task 3 */
DoSomethingg3();
}
}
}
void ISR1(void)
{
/* Set flag */
flag1 = 1;
/* If the event handling time is short, handle it in the interrupt
If the event handling time is long, handle it in the background */
DoSomething1();
}
void ISR2(void)
{
/* Set flag */
flag2 = 2;
/* If the event handling time is short, handle it in the interrupt
If the event handling time is long, handle it in the background */
DoSomething2();
}
void ISR3(void)
{
/* Set flag */
flag3 = 1;
/* If the event handling time is short, handle it in the interrupt
If the event handling time is long, handle it in the background */
DoSomething3();
}
While executing the background program sequentially, if an interrupt occurs, the interrupt will interrupt the normal execution flow of the background program and execute the interrupt service routine. In the interrupt service routine, events are flagged, and if the event handling is short, it can be handled in the interrupt service routine; if it is longer, it returns to the background program for handling.
Although the response and handling of events are separated, event handling still executes sequentially in the background. However, compared to the polling system, the foreground-background system ensures that events are not lost. Additionally, with the ability to nest interrupts, this greatly improves the program’s real-time response capability. In most small to medium-sized projects, a well-implemented foreground-background system can achieve an effect similar to that of an operating system.
Multi-Threaded Systems
When an urgent event is flagged in the interrupt, if the corresponding thread’s priority is high enough, it will respond immediately. Compared to the foreground-background system, the real-time performance of multi-threaded systems is further improved.
The pseudo code for a multi-threaded system is illustrated in the code listing:
int flag1 = 0;
int flag2 = 0;
int flag3 = 0;
int main(void)
{
/* Hardware related initialization */
HardWareInit();
/* OS Initialization */
RTOSInit();
/* OS Start, begin multi-thread scheduling, do not return */
RTOSStart();
}
void ISR1(void)
{
/* Set flag */
flag1 = 1;
}
void ISR2(void)
{
/* Set flag */
flag2 = 2;
}
void ISR3(void)
{
/* Set flag */
flag3 = 1;
}
void DoSomething1(void)
{
/* Infinite loop, cannot return */
for (;;) {
/* Thread entity */
if (flag1) {
}
}
}
void DoSomething2(void)
{
/* Infinite loop, cannot return */
for (;;) {
/* Thread entity */
if (flag2) {
}
}
}
void DoSomething3(void)
{
/* Infinite loop, cannot return */
for (;;) {
/* Thread entity */
if (flag3) {
}
}
}
Compared to the sequentially executing program body in the background of the foreground-background system, in the multi-threaded system, the program body is divided into independent, infinite loops that cannot return, which we call threads.
Each thread is independent, does not interfere with others, and has its own priority, managed by the operating system. With the addition of an operating system, programming becomes simpler as there is no need to carefully design the execution flow of the program and no concern about interference between functional modules.
With the addition of the operating system, our programming actually becomes simpler. The only additional overhead is the small amount of FLASH and RAM occupied by the operating system. Nowadays, the FLASH and RAM of microcontrollers are increasing, more than enough to cover the overhead of RTOS.
Differences between polling, foreground-background, and multi-threaded system software models:

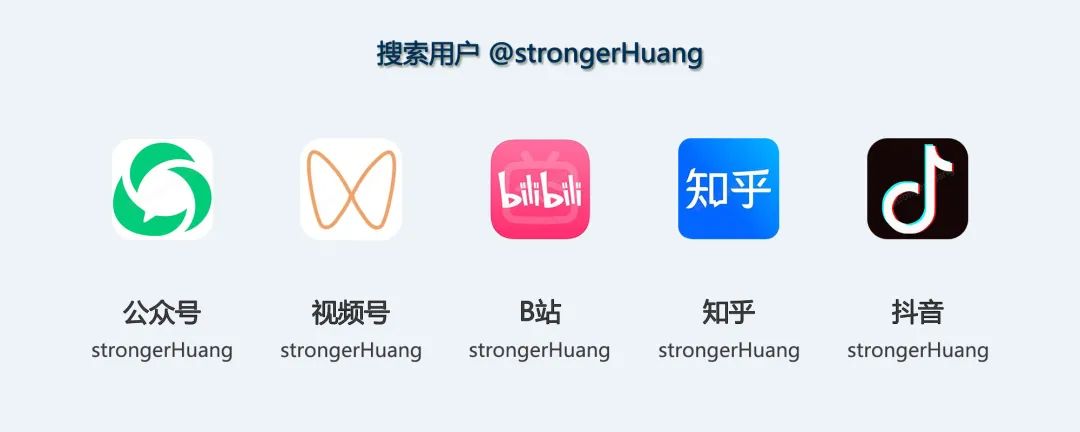
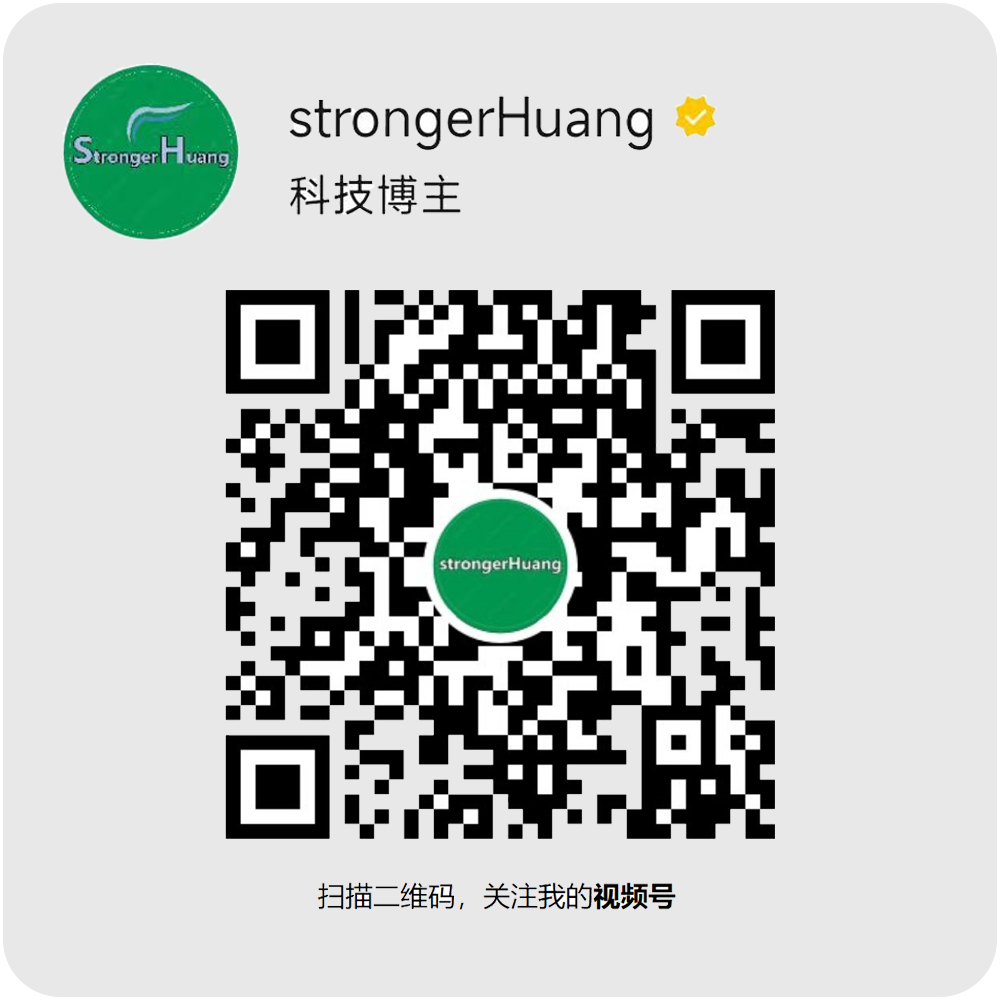