Today, I will share with you three implementations of critical section protection in Cortex-M bare metal environments.
Friends who have worked with embedded systems and RTOS are probably very familiar with the functionality codes OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL(). In an RTOS, there are often multi-task (process) handling situations where certain special operations (such as Flash erase in XIP mode, low-power mode switching) cannot be interrupted arbitrarily, or certain shared data areas cannot be accessed in a disordered manner (Task A is reading while Task B is writing). At this time, a critical section protection strategy is needed.
The so-called critical section protection strategy simply means that multiple tasks must mutually exclude access to critical hardware or software resources in the system. In an RTOS environment, there are ready-made critical section protection interface functions, but there is also a need for this in bare metal systems. In bare metal systems, critical section protection is mainly related to global interrupt control. I previously wrote an article titled “Universal Triple Interrupt Control Design in Embedded MCUs,” which introduced that the third level, which is the highest level of interrupt control, is global interrupt control. Today, I will introduce three methods of critical section protection starting from the use of this global interrupt control:
1. Testing Scenarios for Critical Section Protection
There are mainly two testing scenarios for critical section protection. The first scenario is that multiple tasks under protection are unrelated and will not nest, as shown in the code below. Task1 and Task2 are protected in sequence, so the critical section protection functions enter_critical() and exit_critical() are always executed in strict pairs:
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected task 1
do_task1();
// Exit critical section
exit_critical();
// Enter critical section
enter_critical();
// Perform protected task 2, unrelated to task 1
do_task2();
// Exit critical section
exit_critical();
}
The second scenario is that multiple tasks may be related and there will be nesting situations, as shown in the code below. Task2 is a sub-task of Task1. In this case, you will find that enter_critical() is executed twice first, followed by exit_critical() executed twice. It is important to note that although sub-task Task3 inside Task1 is not actively protected like sub-task Task2, the entire main task Task1 is protected, so sub-task Task3 should also be protected.
void do_task1(void)
{
// Enter critical section
enter_critical();
// Perform protected task 2, which is a sub-task of task 1
do_task2();
// Exit critical section
exit_critical();
// Perform task 3
do_task3();
}
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected task 1
do_task1();
// Exit critical section
exit_critical();
}
2. Three Implementations of Critical Section Protection
Now that the testing scenarios for critical section protection are clear, let’s move on to the implementation of the critical section protection functions enter_critical() and exit_critical():
2.1 Basic Approach
The first approach is very basic, which is to directly wrap the global interrupt control functions __disable_irq() and __enable_irq(). Returning to the previous testing scenario, this implementation can handle non-nested task protection well, but it fails for mutually nested task protection. In the previous testing code, Task3 should also be protected, but it is not actually protected because the exit_critical() immediately opens the global interrupt right after Task2.
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
}
void exit_critical(void)
{
// Enable global interrupts
__enable_irq();
}
2.2 Improved Approach
Can the basic approach be improved? Of course, we just need to add a global variable s_lockObject to keep track of the current number of times the critical section protection has been entered, as shown in the following code. Each time enter_critical() is called, it will directly disable global interrupts (ensuring that the critical section is definitely protected) and record the count, while exit_critical() will only enable global interrupts when the current count is 1 (indicating that the current situation is not a nested critical section protection), otherwise it will just decrement the count. The improved implementation can clearly protect Task3 in the previous testing code.
static uint32_t s_lockObject;
void init_critical(void)
{
__disable_irq();
// Reset counter
s_lockObject = 0;
__enable_irq();
}
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
// Increment counter
++s_lockObject;
}
void exit_critical(void)
{
if (s_lockObject <= 1)
{
// Only enable global interrupts and reset counter when the count is not greater than 1
s_lockObject = 0;
__enable_irq();
}
else
{
// When the count is greater than 1, just decrement the counter
--s_lockObject;
}
}
2.3 Ultimate Approach
Although the improved approach solves the problem of nested task protection in critical sections, it introduces a global variable and an initialization function, making the implementation less elegant. Moreover, global variables in embedded systems can easily be tampered with, posing certain risks. Is there a better implementation? Certainly, this can be done with the help of the special masking register PRIMASK of the Cortex-M processor core. Below is the definition of the PRIMASK register bits (taken from the ARMv7-M manual). Only the lowest bit PM is effective; when PRIMASK[PM] is 1, the global interrupts are disabled (raising the execution priority to 0x0/0x80); when PRIMASK[PM] is 0, the global interrupts are enabled (having no effect on execution priority).
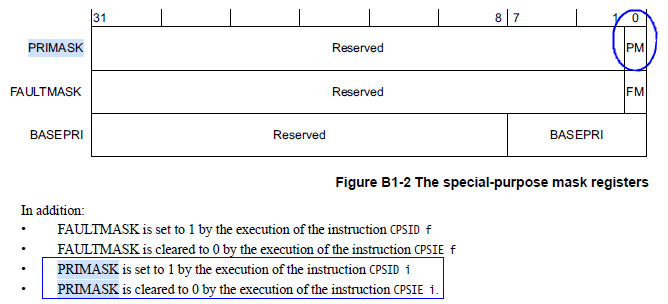
At this point, you should understand that the functions __disable_irq() and __enable_irq() actually operate on the PRIMASK register. Since the PRIMASK register also saves the state of the global interrupt switch, we can replace the function of the global variable s_lockObject in the improved approach by obtaining the PRIMASK value. The code implementation is as follows:
uint32_t enter_critical(void)
{
// Save the current PRIMASK value
uint32_t regPrimask = __get_PRIMASK();
// Disable global interrupts (actually setting PRIMASK to 1)
__disable_irq();
return regPrimask;
}
void exit_critical(uint32_t primask)
{
// Restore PRIMASK
__set_PRIMASK(primask);
}
Since the prototypes of enter_critical() and exit_critical() have changed, their usage must also be modified accordingly:
void critical_section_test(void)
{
// Enter critical section
uint32_t primask = enter_critical();
// Perform protected task
do_task();
// Exit critical section
exit_critical(primask);
// ...
}
Appendix: PRIMASK Register Setting Functions Implemented in Various IDEs
//////////////////////////////////////////////////////
// Implementation in IAR environment (see cmsis_iccarm.h file)
#define __set_PRIMASK(VALUE) (__arm_wsr("PRIMASK", (VALUE)))
#define __get_PRIMASK() (__arm_rsr("PRIMASK"))
//////////////////////////////////////////////////////
// Implementation in Keil environment (see cmsis_armclang.h file)
__STATIC_FORCEINLINE void __set_PRIMASK(uint32_t priMask)
{
__ASM volatile ("MSR primask, %0" : : "r" (priMask) : "memory");
}
__STATIC_FORCEINLINE uint32_t __get_PRIMASK(void)
{
uint32_t result;
__ASM volatile ("MRS %0, primask" : "=r" (result) );
return(result);
}
Thus, the three implementations of critical section protection in the Cortex-M bare metal environment have been explained. Have you learned it?

Click “Read Original” to see more shares. Welcome to share, bookmark, like, and view.