Background
Recently, some security requirements were rectified in the project. Encryption is one of the commonly used means during the process. Here is a brief summary, hoping to help everyone.
Usage Scenarios
Encryption is a process that transforms raw information (plaintext)
into a form (ciphertext)
that is difficult to understand directly.
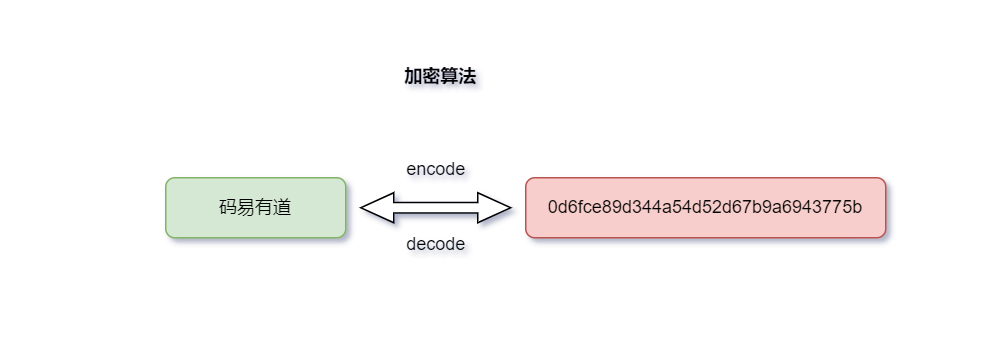
The main purpose is to protect the security and privacy of information. It is mainly applied in the following scenarios:
-
Data Confidentiality: Prevent unauthorized access to personal identity information and sensitive data. Protect personal privacy, business secrets, and national secrets. For example: internal company documents, cloud storage information from interception.
-
Data Integrity: Ensure that data has not been tampered with during transmission or storage. For example: software downloads, bank transfers, etc.
-
Authentication: Verify the identity of both parties in communication, ensuring that data is sent from a trusted source and has not been intercepted or tampered with during the entire transmission process. For example: user login, email authentication, etc.
-
Data Access Control: Encryption can restrict access to data, allowing only users with the correct key to decrypt and access the data. For example: corporate VPNs, etc.
-
Network Security: When transmitting data over the Internet, encryption can prevent man-in-the-middle attacks and other network attacks, ensuring the security of data transmission over the network. For example: SSL/TLS protocols, etc.
-
Financial Transaction Security: In e-commerce and online banking, encryption is used to protect transaction data and prevent credit card fraud and other financial crimes. For example: online payment (RSA signatures and certificates provided by financial institutions), etc.
Commonly Used Encryption Algorithms
1. MD5 Encryption
Applicable Scenarios
MD5, as a widely used hash function, is mainly used in the following scenarios:
-
Data Integrity Check: Verify whether the file has been tampered with during transmission. -
Digital Signature: Ensure the integrity of information transmission and the authenticity of the source. -
Password Storage: Store the hashed value of user passwords in the database instead of plaintext.
Features
-
Fixed Length: Regardless of input length, the output is a 128-bit (16-byte) hash value. -
Fast Calculation: The MD5 algorithm computes quickly, suitable for hashing large amounts of data. -
Irreversibility: MD5 encryption is irreversible, so there is usually only an encryption process, without a decryption process
.
Principle and Flow Chart
The MD5 algorithm processes the input message through padding, segmentation, and cyclic processing steps, ultimately generating a 128-bit hash value.
Using the string “Hello” to illustrate the basic processing flow of the MD5 algorithm:
1. Original input (plaintext)
|
| (converted to binary)
V
2. Binary data "Hello"
|
| (padded to a multiple of 512 bits)
V
3. Padded binary data
|
| (adding a 64-bit representation of the original length)
V
4. Length-augmented binary data
|
| (split into 512-bit message blocks)
V
5. Message block 1 ... Message block n
|
| (each block 512 bits, total 16 32-bit words)
V
6. Initial MD5 value (ABCD)
|
| (process each block through the main loop)
V
7. Main loop (16 steps per block)
|_________|_________|
| | |
V V V
8. 32-bit words processed by functions F, G, H, I
|_________|_________|
| | |
V V V
9. Updated MD5 value
|
V
10. Final MD5 hash value (128 bits)
Code Implementation
Example code for implementing MD5 encryption in Java:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class MD5Example {
// Method to convert byte array to hexadecimal string
public static String toHexString(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
public static String getMD5(String input) {
try {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] messageDigest = md.digest(input.getBytes());
return toHexString(messageDigest);
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
public static void main(String[] args) {
String password = "Hello, World!";
// Output result: 65a8e27d8879283831b664bd8b7f0ad4
System.out.println("MD5 Hash: " + getMD5(password));
}
}
Notes
-
The MD5 algorithm is no longer considered secure and is not recommended for high-security encryption scenarios. -
The output of the MD5 algorithm can be used for data integrity checks, but should not be used for encryption and decryption operations.
2. AES Encryption
Applicable Scenarios
AES (Advanced Encryption Standard) is a widely used symmetric encryption
algorithm, suitable for the following scenarios:
-
Data Transmission Security: Encrypting data in network communication to protect privacy and integrity during data transmission. -
File and Data Storage: Encrypting sensitive files stored on servers or in the cloud. -
VPN Connection: Ensuring the security of remote connections.
Features
-
Security: AES provides strong security and is currently one of the most recommended symmetric encryption algorithms. -
Flexibility: Supports key lengths of 128, 192, and 256 bits, accommodating different security level requirements. -
High Efficiency: The AES algorithm is highly efficient
, suitable for fast encryption of large amounts of data.
Principle and Flow Chart
For example, using the AES encryption algorithm to encrypt the string “hello”:
1. Plaintext "hello"
|
| (converted to binary format)
V
2. 128-bit binary plaintext
|
| (may need padding)
V
3. Padded 128-bit binary data
|
| (key expansion)
V
4. Generate round keys
|
| (add initial round key)
V
5. Data block XORed with round key
|
| (main round encryption processing)
V
|_________________________|
| |
| SubBytes (byte substitution) |
|_________________________|
| |
| ShiftRows (row shifting) |
|_________________________|
| |
| MixColumns (column mixing) |
|_________________________|
| |
| AddRoundKey (round key addition) |
|_________________________|
V V
6. Ciphertext data block
|
V
7. Ciphertext (binary format)
Example Code
Example of AES encryption for the string “hello”:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.NoSuchAlgorithmException;
public class AESExample {
public static void main(String[] args) {
try {
// Instantiate key generator and initialize for AES (128 bits)
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
// Initialize 128 bits, can be modified
keyGenerator.init(128);
SecretKey secretKey = keyGenerator.generateKey();
// Instantiate Cipher object for AES encryption
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Convert plaintext string "hello" to binary data
String plainText = "hello";
byte[] plainTextBytes = plainText.getBytes();
// Encrypt to get ciphertext
byte[] encryptedBytes = cipher.doFinal(plainTextBytes);
// Print encrypted ciphertext (usually represented in hexadecimal)
System.out.println("Encrypted bytes: " + bytesToHex(encryptedBytes));
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
}
// Convert byte array to hexadecimal string
private static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
}
Notes
-
AES encryption is symmetric encryption
, using the samekey
for both encryption and decryption. -
The choice of key length needs to be determined based on specific application scenarios and security requirements.
3. DES Encryption
Applicable Scenarios
DES (Data Encryption Standard) is an older symmetric encryption
algorithm. Due to its key length (56 bits effective key, relatively short), its security is relatively low, and it is still used in some older systems.
Main applicable scenarios include:
-
Data Transmission: In situations where security requirements are not particularly high, DES can still be used for data transmission encryption.
Features
-
Symmetric Encryption: Uses the same key for both encryption and decryption. -
Key Length: The actual effective key length is 56 bits, with an additional 8 bits used for parity check. -
Efficiency: Due to the older algorithm, its relative speed on modern hardware is slower. -
Security: Due to the short key length, DES is susceptible to brute-force attacks, thus considered insecure.
Principle and Flow Chart
We use the following steps to encrypt the string “hello” with DES:
1. Plaintext "hello"
|
| (convert to binary and pad)
V
2. 64-bit binary plaintext
|
| (initial permutation IP)
V
3. Data block after initial permutation
|
| (add key)
V
4. Data block XORed with key
|
| (Feistel network processing)
V
|_________________________|
| |
| Key addition |
|_________________________|
| |
| Permutation |
|_________________________|
| |
| Substitution |
|_________________________|
| |
| Left shift |
|_________________________|
V V
5. Feistel network output
|
| (inverse permutation IP^-1)
V
6. Ciphertext block after inverse permutation
|
V
7. Ciphertext
Example Code (Java)
Here is an example using Java code to encrypt the string “hello” with DES:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
public class DESExample {
public static void main(String[] args) {
try {
// Instantiate key generator and initialize for DES
KeyGenerator keyGenerator = KeyGenerator.getInstance("DES");
keyGenerator.init(56); // DES key length is 56 bits
SecretKey secretKey = keyGenerator.generateKey();
// Instantiate Cipher object for DES encryption
Cipher cipher = Cipher.getInstance("DES/CBC/PKCS5Padding");
IvParameterSpec iv = new IvParameterSpec(new byte[8]); // Use 8-byte IV
cipher.init(Cipher.ENCRYPT_MODE, secretKey, iv);
// Convert plaintext string "hello" to binary data
String plainText = "hello";
byte[] plainTextBytes = plainText.getBytes("UTF-8");
// Encrypt to get ciphertext
byte[] encryptedBytes = cipher.doFinal(plainTextBytes);
// Print encrypted ciphertext (usually represented in Base64)
System.out.println("Encrypted text: " + Base64.getEncoder().encodeToString(encryptedBytes));
} catch (Exception e) {
e.printStackTrace();
}
}
}
Notes
-
Due to its short key length, DES is no longer suitable for scenarios requiring high security. -
In practical use, it is recommended to use AES or other more secure encryption algorithms.
4. National Secret Algorithm Encryption
Applicable Scenarios
The national secret algorithm, i.e., the Chinese national commercial password algorithm standard, includes but is not limited to SM1, SM2, SM3, SM4, etc. It is mainly used for:
-
Financial Services: Used to ensure transaction security in the financial services industry. -
Government Communication: Ensures the security of government data transmission. -
Enterprise Data Protection: Used to protect business secrets and customer data. -
Secure Tokens: Used for the encryption of secure tokens.
Features
-
Standardization: The national secret algorithm complies with China’s commercial password standards. -
Security: Resistant to various known attack methods. -
Diversity: Includes various algorithms, such as SM2 for digital signatures and SM4 for data encryption.
Principle and Flow Chart
Using the string “hello” for illustration:
1. Plaintext "hello"
|
| (converted to binary format)
V
2. Binary plaintext data
|
| (configure keys and parameters)
V
3. Initialize encryption module
|
| (set SM4 key and IV)
V
4. Use SM4 to encrypt plaintext
|
| (multi-round iterative encryption processing)
V
5. Output SM4 ciphertext
Example Code (Java)
Example of using Java pseudocode to encrypt the string “hello” with SM4:
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import java.security.NoSuchAlgorithmException;
import java.security.Security;
public class SM4Example {
public static void main(String[] args) {
try {
// Add Bouncy Castle security provider
Security.addProvider(new BouncyCastleProvider());
// Instantiate key generator and initialize for SM4
KeyGenerator keyGenerator = KeyGenerator.getInstance("SM4");
keyGenerator.init(128); // SM4 key length is 128 bits
SecretKey secretKey = keyGenerator.generateKey();
// Instantiate Cipher object for SM4 encryption
Cipher cipher = Cipher.getInstance("SM4/CBC/PKCS7Padding");
IvParameterSpec iv = new IvParameterSpec(new byte[16]); // 16-byte IV
cipher.init(Cipher.ENCRYPT_MODE, secretKey, iv);
// Convert plaintext string "hello" to binary data
String plainText = "hello";
byte[] plainTextBytes = plainText.getBytes("UTF-8");
// Encrypt to get ciphertext
byte[] encryptedBytes = cipher.doFinal(plainTextBytes);
// Print encrypted ciphertext (usually represented in hexadecimal or Base64)
System.out.println("Encrypted text: " + bytesToHex(encryptedBytes));
} catch (Exception e) {
e.printStackTrace();
}
}
// Convert byte array to hexadecimal string
private static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
}
Notes
-
Key management is an important aspect of the encryption process; keys should be securely generated, stored, and distributed. -
Using national secret algorithms requires compliance with relevant laws, regulations, and standards.
5. RSA Encryption
Applicable Scenarios
Usage scenarios:
-
Secure Email: Use RSA to encrypt email content, ensuring security during email transmission. -
SSL/TLS: In the SSL/TLS protocol, RSA is used to encrypt the exchange of symmetric keys to establish secure communication. -
Digital Signature: RSA is used to generate digital signatures, verifying the integrity and source of software or documents. -
Authentication: Used in the authentication process to ensure the identity of both parties in communication.
Features
-
Asymmetric Encryption: Uses a pair of keys, public key for encryption and private key for decryption. -
Security: Difficult to crack, with high security. -
Key Length: Typically uses keys of 1024 bits, 2048 bits, or longer to enhance security.
Principle and Flow Chart
For example, the encryption and decryption process of the plaintext “hello” is as follows:
1. Plaintext "hello"
|
| (converted to numerical m)
V
2. Encrypt using public key (e, n)
|___________|
| |
| m^e mod n|
|___________|
|
V
3. Get ciphertext c
|
| (decrypt using private key (d, n))
V
|___________|
| |
| c^d mod n|
|___________|
|
V
4. Restore plaintext m
|
V
5. Plaintext "hello"
Program Implementation
Simple example of implementing RSA encryption and decryption in Java:
import javax.crypto.Cipher;
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Base64;
public class RSAExample {
public static void main(String[] args) throws Exception {
// Generate key pair
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
// Public key and private key
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// Plaintext
String plainText = "hello";
byte[] data = plainText.getBytes();
// Encrypt
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedData = cipher.doFinal(data);
System.out.println("Encrypted: " + Base64.getEncoder().encodeToString(encryptedData));
// Decrypt
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedData = cipher.doFinal(encryptedData);
String decryptedText = new String(decryptedData);
System.out.println("Decrypted: " + decryptedText);
}
}
Notes
-
Key Length: It is recommended to use keys of 2048 bits or longer to ensure security. -
Key Management: Public keys can be shared, but private keys must be kept secret to prevent leakage. -
Performance: RSA encryption and decryption are relatively slow, not suitable for encrypting large amounts of data.
Conclusion
In summary, in practical use, the choice is often based on system characteristics such as execution efficiency, data volume, and security compliance requirements. Here is a simplified version of the above summary.
Encryption Algorithm | Name | Effective Length | Characteristics | Encrypt/Decrypt | Advantages | Disadvantages |
---|---|---|---|---|---|---|
MD5 | MD5 Hash Algorithm | 128 bits | Hash Function | No | Fast, simple | Low security, susceptible to collision attacks |
AES | AES Encryption Algorithm | 128/192/256 bits | Symmetric Encryption | Yes | High security, high efficiency | No serious weaknesses |
DES | DES Encryption Algorithm | 56 bits | Symmetric Encryption | Yes | Fast speed, widely used | Short key, low security |
National Secret SM4 | SM4 Encryption Algorithm | 128 bits | Symmetric Encryption | Yes | Complies with domestic standards, good security | Less widely used than AES |
RSA | RSA Encryption Algorithm | 1024/2048 bits | Asymmetric Encryption | Yes | Asymmetric encryption, suitable for digital signatures | High computational load, slow speed |
Conclusion
Sharing is winning together. If this helps, feel free to share
, like
, and view
. Follow the official account 【CodeEasy】, and let’s do long-term and correct things together!!!