This article is reprinted from the SegmentFault community
Community Column: Love Frontend
Author: linshuai
In today’s world, where information security is becoming increasingly important, various encryption methods in frontend development have also become more critical. Typically, in interactions with servers, to ensure the security of data transmission and prevent data tampering, in addition to the use of https, it is necessary to perform encryption and decryption on the transmitted data.
The common encryption algorithms can be divided into three categories
-
Symmetric encryption algorithms:AES,… -
Asymmetric encryption algorithms:RSA,… -
Hash algorithms:MD5,…
1
Symmetric Encryption Algorithms
Symmetric encryption (also known as private key encryption) refers to encryption algorithms that use the same key for both encryption and decryption. It requires the sender and receiver to agree on a key before secure communication. The security of symmetric algorithms relies on the key; if the key is leaked, anyone can decrypt the messages they send or receive, making the confidentiality of the key crucial for secure communication.
Characteristics
-
Advantages: algorithms are public, low computational cost, fast encryption speed, high encryption efficiency. -
Disadvantages: before data transmission, the sender and receiver must agree on a key, and both parties must keep the key secure. If one party’s key is leaked, the encrypted information becomes insecure. -
Use cases: local data encryption, https communication, network transmission, etc.
AES
AES: Advanced Encryption Standard is the most common symmetric encryption algorithm (the encryption transmission of WeChat mini-programs uses this encryption algorithm).
The key: a password used to encrypt plaintext. The key is generated through negotiation between the receiver and sender but should not be transmitted directly over the network to avoid leakage. It is usually encrypted using asymmetric encryption algorithms and then transmitted over the network, or discussed face-to-face. The key must never be leaked; otherwise, attackers can restore the ciphertext and steal data.
When you need to use AES encryption in a project, you can use the open-source crypto-js library:
crypto-js:
https://github.com/brix/crypto-js
var CryptoJS = require('crypto-js');
var data = { id: 1, text: 'Hello World' };
// Encrypt to generate ciphertext
var ciphertext = CryptoJS.AES.encrypt(JSON.stringify(data), 'secret_key_123').toString();
// Decrypt to get plaintext
var bytes = CryptoJS.AES.decrypt(ciphertext, 'secret_key_123');
var decryptedData = JSON.parse(bytes.toString(CryptoJS.enc.Utf8));
2
Asymmetric encryption algorithms
Asymmetric encryption algorithms require two keys: a public key (publickey: abbreviated as public key) and a private key (privatekey: abbreviated as private key). The public key and private key are a pair; if data is encrypted with the public key, only the corresponding private key can decrypt it. Because encryption and decryption use two different keys, this algorithm is called an asymmetric encryption algorithm.
Characteristics
-
Advantages: asymmetric encryption is more secure than symmetric encryption. -
Disadvantages: encryption and decryption take longer, and the speed is slow, suitable only for encrypting small amounts of data. -
Use cases: early https sessions, CA digital certificates, information encryption, login authentication, etc.
RSA
RSA encryption algorithm is the most common asymmetric encryption algorithm.RSA was proposed in 1977 by Ron Rivest, Adi Shamir, and Leonard Adleman. The name RSA is formed from the initials of their last names.
When you need to use RSA encryption in a project, you can use the open-source jsencrypt library:
jsencrypt:
https://github.com/travist/jsencrypt
// Encrypt using public key
var publicKey = 'public_key_123';
var encrypt = new JSEncrypt();
encrypt.setPublicKey(publicKey);
var encrypted = encrypt.encrypt('Hello World');
// Decrypt using private key
var privateKey = 'private_key_123';
var decrypt = new JSEncrypt();
decrypt.setPrivateKey(privateKey);
var uncrypted = decrypt.decrypt(encrypted);
3
Hash, generally translated as “hash”, is also directly transliterated as “哈希” (hā xī), which transforms an input of any length (also called pre-image) into a fixed-length output through a hash algorithm. This transformation is a compression mapping, meaning the space of hash values is usually much smaller than the input space, and different inputs may hash to the same output, while it is impossible to uniquely determine the input value from the hash value. In simple terms, it is a function that compresses messages of arbitrary length into a fixed-length message digest.
Characteristics
-
Advantages: irreversible, easy to compute, characterized -
Disadvantages: hash collisions may occur -
Use cases: file or string consistency verification, digital signatures, authentication protocols
MD5
MD5 is a relatively common hash algorithm. For MD5, two characteristics are very important: first, the value after hashing plaintext data is of fixed length; second, for any piece of plaintext data, the result after hashing must always be the same. The former means that it is possible for two pieces of plaintext to hash to the same result, while the latter means that if we hash specific data, the result will definitely be the same.
For example, when logging in, the password is encrypted with md5 and then transmitted to the server, where the password is also stored encrypted with md5, so it is sufficient to verify whether the encrypted ciphertext matches.
When you need to use MD5 encryption in a project, you can use the open-source JavaScript-MD5
JavaScript-MD5:
https://github.com/blueimp/JavaScript-MD5
var hash = md5('Hello World');
// b10a8db164e0754105b7a99be72e3fe5
4
Base64 Encoding
Base64 encoding is merely a coding format and not an encryption algorithm; it can be used to transmit longer identification information in an HTTP environment.
Characteristics
-
Can encode any binary data into Base64 format -
Data volume increases after encryption, approximately by 1/3 -
A notable characteristic after encoding is the trailing = sign -
Can be decoded back -
Base64 encoding has unreadability
Modern browsers provide Base64 encoding and decoding methods, btoa() and atob()
var enc = window.btoa('Hello World');
// SGVsbG8gV29ybGQ=
var str = window.atob(enc);
// Hello World
5
Summary
In business http requests, the AES key is randomly generated on the frontend, and the RSA public key is obtained from the server. The AES key is then asymmetrically encrypted and sent to the server in the request header, while the AES is used to encrypt the body. The server receives the encrypted key in the request header, decrypts it using the RSA key to obtain the plaintext AES key, which can then be used to decrypt the body. The md5 has the characteristic of verifying string consistency, and to prevent the request from being intercepted and tampered with, the body string can be md5 encrypted before transmission in the request header. After the server receives the request, it decrypts the body and then md5 checks it against the request header to verify if the request has been tampered with.
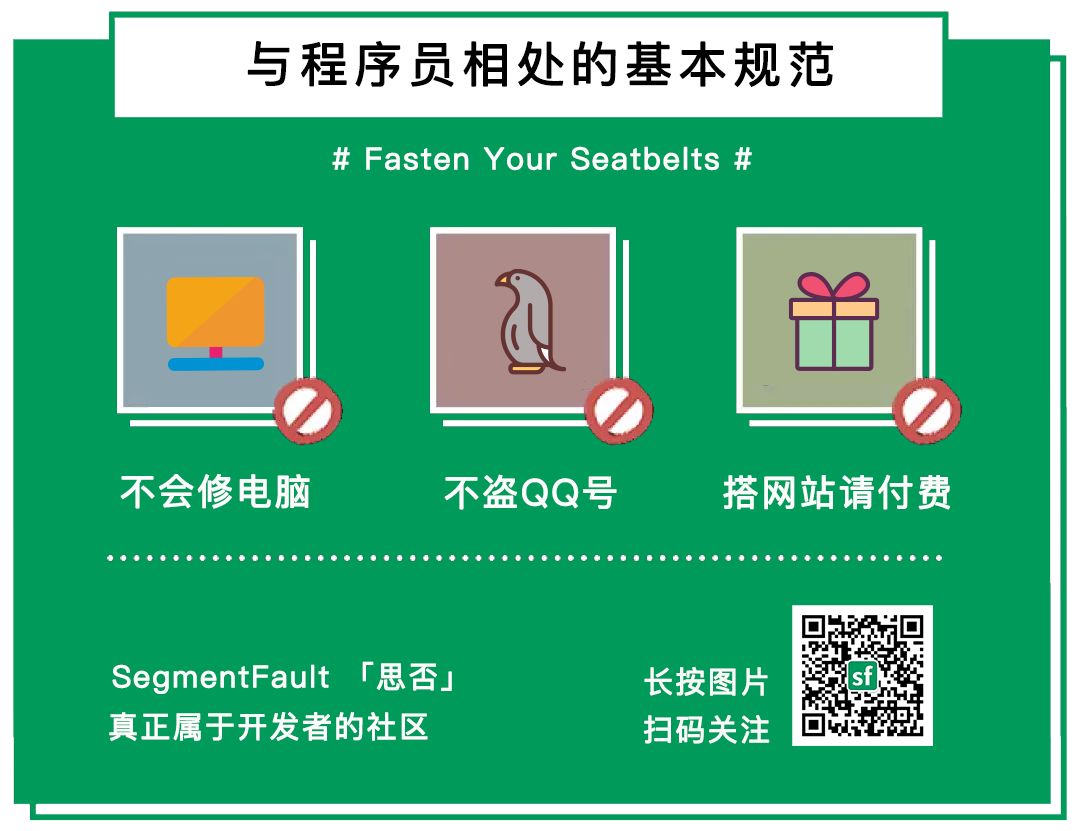