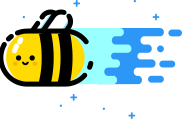
Click the blue text to follow!
The code used in this article can be obtained from https://github.com/FantasyGao/FantasyGao.github.io.1. The Significance of Cryptographic Algorithms
Simply put, the emergence of cryptographic algorithms is to solve the problems of data privacy and security in the Internet of Everything. When we are surfing the web, data is constantly being exchanged and flowing. Without cryptographic algorithms, our various passwords or some private information would be exposed on the network. If an attacker intercepts the requests made when you exchange data, it means you have no privacy.
2. Scenarios for Using Cryptographic Algorithms
From the technology I am currently exposed to, there are not many scenarios where encryption is used. The Internet is fundamentally based on connectivity and information sharing. For many websites, the starting point is to allow people to read and understand them. However, when you want to let others know that this record was made by you, you need to create a website identity, just like in real life. This operation is the process of registering an account, which requires a password to ensure that only you can log in to this account. Therefore, this password must not be known to others. When you fill in the password and submit it, an encrypted string is sent to the website backend for storage. To ensure security and prevent data breaches, the website only stores this string, which is generally unknown to anyone except you, including the website personnel. This is why you can only reset your password instead of retrieving it if you forget it. This encryption method is non-decryptable. In the registration process, we often fill in our phone numbers. However, storing user data in the database is also very insecure. Therefore, the phone number needs to be encrypted before being stored. However, after storing the phone number, the website usually needs to send you a verification code, which cannot be done using the same non-decryptable method as the password. At this point, a decryptable algorithm is required. Generally, in this step, the backend uses symmetric encryption algorithms to encrypt your phone number for storage. This operation requires an encryption key. When sending you marketing information, the key can be used to decrypt it.
There are many types of non-decryptable algorithms, such as MD5, SHA1, SHA0, SHA256, SHA384, etc. Below are a few examples:
// node.js
require('crypto')
.createHash('md5'|'sha'|'sha1'|'sha256')
.update('password', 'utf8')
.digest('hex');
# mysql
select MD5|SHA|SHA1('password');
select SHA2('password', 256| 384 | 512);
As shown above, all can achieve the effect of encryption. As for the differences among various methods, MD5 and SHA, SHA1 have already been used in high-standard encryption scenarios. For example, GitHub used SHA1 for logs in the past but later switched to SHA256 due to its shorter bit length. In an environment with increasingly faster computers, the process of reverse cracking is also accelerated, making it less secure. It should be noted that any encryption algorithm mentioned above can potentially be reverse-cracked; it is merely a matter of computational power and time. The result after encryption is ultimately a finite result set. For example, the SHA2 algorithm with 256 bits has 2 to the power of 256 results. Once you enumerate all results, there may be a result that matches your input password, thereby impersonating you and logging into the website, which is unsafe. However, this is currently very difficult to achieve, so this algorithm is considered secure at present.
Symmetric encryption algorithms include AES, DES, TripleDES, RC2, RC4, RC5, and Blowfish. Those who have used Shadowsocks will definitely know that when selecting a method, you need to choose an algorithm for your data encryption. The algorithms there are all symmetric encryption methods. Below is an example of symmetric encryption:
// node.js
const aes = require('crypto').createCipher('aes192', 'my-key')
const secret = aes.update('phone number','utf8', 'hex') + aes.final('hex')
console.log(secret);
const des = require('crypto').createDecipher('aes192', 'my-key')
des.update(secret, 'hex', 'utf8')
console.log(des.final('utf8'));
//mysql-aes
require('mysql-aes').encrypt('phone number', 'my-key');
require('mysql-aes').decrypt('480AE3E13FA619C5CBF3921E447A6C79', 'my-key');
# mysql
select AES_decrypt(AES_ENCRYPT('phone number', 'my-key'), 'my-key')
select DES_decrypt(DES_ENCRYPT('phone number', 'my-key'), 'my-key')
When we are registering, we may not want to expose our phone number, but we cannot use symmetric encryption because it requires a key for collaboration. At this time, we need a new method. First, we need to encrypt the data transmitted from the front end. Secondly, the registration information must be decipherable in the backend. This requires two keys: one that can be public and one that cannot be public. They must be a one-to-one pair. The client encrypts the data with the public key and returns it to the server, which uses the private key to decrypt the data, completing the process. This method is called asymmetric encryption.
Symmetric encryption is more efficient and faster than asymmetric encryption, while asymmetric encryption is more secure. Therefore, a choice should be made during usage to select the appropriate method.
3.HTTPS and Cryptographic Algorithms
Before HTTPS, we used HTTP, which is essentially “naked”. All data exchanged is transmitted in plaintext, which is less secure. The emergence of HTTPS is to encrypt data transmission, enhancing security and reliability. HTTPS equals HTTP plus SSL/TLS. HTTPS employs both symmetric and asymmetric encryption, fully utilizing the advantages of each algorithm. You can refer to this article for the principles of HTTPS, which I think is very well written.
4. MD5 Collision
Collision means that two different sources yield the same result. This was demonstrated in an example I saw while studying.
const crypto = require('crypto');
const hexStr2hexArr = str => {
let result = []
for(let i = 0; i<str.length (i+1)*2)="" .createhash('md5')="" .digest('hex')="" .update(buffer(bt))="" .update(buffer(bt1))="" 16))="" 2;="" bt="hexStr2hexArr(tst)" bt1="hexStr2hexArr(tst1)" code="" console.log('hash',="" console.log(bt)="" console.log(bt1)="" const="" hash="crypto" hash)="" hash1="crypto" hash1)<="" hexstr="str.slice(i*2," i++)="" result="" result.push(parseint(hexstr,="" return="" tst="0e306561559aa787d00bc6f70bbdfe3404cf03659e704f8534c00ffb659c4c8740cc942feb2da115a3f4155cbb8607497386656d7d1f34a42059d78f5a8dd1ef" tst1="0e306561559aa787d00bc6f70bbdfe3404cf03659e744f8534c00ffb659c4c8740cc942feb2da115a3f415dcbb8607497386656d7d1f34a42059d78f5a8dd1ef" var="" {="" }=""></str.length>
5. SHA1 Collision
Google engineers found that two different PDFs produced the same hash value when using the SHA1 algorithm to obtain the file hash.pdf1pdf2
const stream1 = require('fs').createReadStream('./20181230_2.pdf')
const stream2 = require('fs').createReadStream('./20181230_3.pdf')
const hash1 = require('crypto').createHash('sha1')
const hash2 = require('crypto').createHash('sha1')
stream1.on('data', data => hash1.update(data))
stream2.on('data', data => hash2.update(data))
stream1.on('end', () => console.log('file1 hash', hash1.digest('hex')))
stream2.on('end', () => console.log('file2 hash', hash2.digest('hex')))
$ node sha1.js
file1 hash 38762cf7f55934b34d179ae6a4c80cadccbb7f0a
file2 hash 38762cf7f55934b34d179ae6a4c80cadccbb7f0a
6. Conclusion
Cryptographic algorithms serve as an identity card for the data world. They are finite, but data is infinite. Any hash algorithm can potentially have duplicates, though the possibility is so small that it can be ignored.
References: https://www.jianshu.com/p/bf1d7eee28d0 https://juejin.im/post/5b48b0d7e51d4519962ea383 https://blog.csdn.net/caiqiiqi/article/details/68953730 https://cherryblog.site/HTTPS.html
After reading the article, take two steps:
-
If you think the article is good, you can click “Read” in the bottom right corner or share the article so that more people can see it.
-
Join my “Front-end Advancement Group” to discuss technology with group members and learn about cutting-edge knowledge. You can add my WeChat “yck05016” for more details.