Welcome FPGA engineers to join the official WeChat technical group
Clickthe blue textto follow us at FPGA Home – the best and largest pure FPGA engineer community in China
The serial port is a serial interface, also known as a serial communication interface, which is an extended interface using serial communication. In contrast to the serial port, parallel interfaces such as high-speed AD and DA are used,
these all use parallel interfaces, and programming is also simpler.
The serial port has the following characteristics:
(1) Simple communication lines, only a pair of transmission lines are needed to achieve bidirectional communication.
(2) Simple wiring, low cost.
(3) Long communication distance, capable of achieving communication distances from several meters to thousands of meters.
(4) Slow transmission rate.
Common serial port rates such as 4800, 9600, 115200 bps, represent how many bits of data are sent per second, for example, 9600 bps means sending 9600 bits of data in one second.
Serial port protocol: The protocol is relatively simple, usually consisting of 10 data bits, 1 start bit (low level), followed by eight data bits, with the low bit first, one parity bit, which is generally not used, and finally one stop bit (high level), thus ending a frame of data transmission.
Below is an introduction to my program framework:
The overall framework is divided into two parts: one is the serial port driver part and the other is the serial port data control part. The serial port driver part is responsible for the serial port driving and baud rate selection, while the serial port data control module
is responsible for controlling the data content and the transmission speed.
From the timing diagram above, it can be seen that a frame of data is sent every 10ms, where data_en is responsible for enabling the baud rate driving, and uart_tx_end has two functions: one is to disable data_en, and the other is to reset the 10ms counter.
/*-----------------------------------------------------------------------
Date : 2017-09-03
Description : Design for uart_driver.
-----------------------------------------------------------------------*/
module uart_tx_driver
(
//global clock
input clk , //system clock
input rst_n , //sync reset
//uart interface
output reg uart_tx ,
//user interface
input [1:0] bps_select , //baud rate selection
input [7:0] uart_data ,
input data_en , //data transmission enable
output reg uart_tx_end
);
//--------------------------------
//Funtion : Parameter definition
parameter BPS_4800 = 14'd10417 ,
BPS_9600 = 14'd5208 ,
BPS_115200 = 14'd434 ;
reg [13:0] cnt_bps_clk ;
reg [13:0] bps ;
reg bps_clk_en ; //baud rate enable clock
reg [3:0] bps_cnt ;
wire [13:0] BPS_CLK_V = bps >> 1 ;
//--------------------------------
//Funtion : Baud rate selection
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
bps <= 1'd0;
else if(bps_select == 2'd0)
bps <= BPS_115200;
else if(bps_select == 2'd1)
bps <= BPS_9600;
else
bps <= BPS_4800;
end
//--------------------------------
//Funtion : Baud rate counting
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
cnt_bps_clk <= 1'd0;
else if(cnt_bps_clk >= bps - 1 && data_en == 1'b0)
cnt_bps_clk <= 1'd0;
else
cnt_bps_clk <= cnt_bps_clk + 1'd1;
end
//--------------------------------
//Funtion : Baud rate enable clock
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
bps_clk_en <= 1'd0;
else if(cnt_bps_clk == BPS_CLK_V - 1)
bps_clk_en <= 1'd1;
else
bps_clk_en <= 1'd0;
end
//--------------------------------
//Funtion : Baud rate frame counting
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
bps_cnt <= 1'd0;
else if(bps_cnt == 11)
bps_cnt <= 1'd0;
else if(bps_clk_en)
bps_cnt <= bps_cnt + 1'd1;
end
//--------------------------------
//Funtion : uart_tx_end
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
uart_tx_end <= 1'd0;
else if(bps_cnt == 11)
uart_tx_end <= 1'd1;
else
uart_tx_end <= 1'd0;
end
//--------------------------------
//Funtion : Data transmission
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
uart_tx <= 1'd1;
else case(bps_cnt)
4'd0 : uart_tx <= 1'd1;
4'd1 : uart_tx <= 1'd0; //begin
4'd2 : uart_tx <= uart_data[0];//data
4'd3 : uart_tx <= uart_data[1];
4'd4 : uart_tx <= uart_data[2];
4'd5 : uart_tx <= uart_data[3];
4'd6 : uart_tx <= uart_data[4];
4'd7 : uart_tx <= uart_data[5];
4'd8 : uart_tx <= uart_data[6];
4'd9 : uart_tx <= uart_data[7];
4'd10 : uart_tx <= 1; //stop
default : uart_tx <= 1;
endcase
end
endmodule
/*-----------------------------------------------------------------------
Date : 2017-XX-XX
Description : Design for .
-----------------------------------------------------------------------*/
module uart_tx_control
(
//global clock
input clk , //system clock
input rst_n , //sync reset
//user interface
output reg [7:0] uart_data ,
output reg data_en ,
input uart_tx_end
);
//--------------------------------
//Funtion : Parameter definition
parameter DELAY_10MS = 500_000 ;
reg [31:0] cnt_10ms ;
wire delay_10ms_done ;
//data define
reg [31:0] cnt_1s;
//--------------------------------
//Funtion : cnt_10ms
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
cnt_10ms <= 1'd0;
else if(cnt_10ms == DELAY_10MS - 1 && uart_tx_end == 1'd1)
cnt_10ms <= 1'd0;
else
cnt_10ms <= cnt_10ms + 1'd1;
end
assign delay_10ms_done = (cnt_10ms == DELAY_10MS - 1) ? 1'd1 : 1'd0;
//--------------------------------
//Funtion : data_en
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
data_en <= 1'd0;
else if(delay_10ms_done)
data_en <= 1'd1;
else if(uart_tx_end)
data_en <= 1'd0;
end
///////////////////////Data testing/////////////////////////////
//--------------------------------
//Funtion : cnt_1s
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
cnt_1s <= 1'd0;
else if(cnt_1s == 49_999_999)
cnt_1s <= 1'd0;
else
cnt_1s <= cnt_1s + 1'd1;
end
//--------------------------------
//Funtion : uart_data
always @(posedge clk or negedge rst_n)
begin
if(!rst_n)
uart_data <= 1'd0;
else if(uart_data >= 10)
uart_data <= 1'd0;
else if(cnt_1s == 49_999_999)
uart_data <= uart_data + 1'd1;
end
endmodule
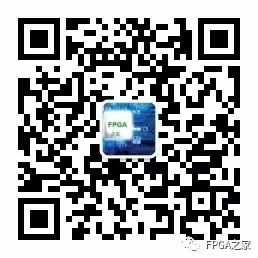
Welcome FPGA engineers and embedded engineers to follow our official account

The largest FPGA WeChat technical group in the country
Welcome everyone to join the national FPGA WeChat technical group, this community has tens of thousands of engineers, a group of engineers who love technology, here FPGA engineers help each other, share, and have a strong technical atmosphere! Hurry up and call your friends to join!!
Press and hold to join the national FPGA technical group
FPGA Home Component City
Advantage component services, if you have needs please scan to contact the group owner: Jin Juan Email: [email protected] Welcome to recommend to procurement
ACTEL, AD part of the advantage ordering (operating the full series):
XILINX, ALTERA advantage stock or ordering (operating the full series):
(The above components are part of the models, for more models please consult the group owner Jin Juan)
Service concept: FPGA Home Component City aims to facilitate engineers to purchase components quickly and conveniently. After years of dedicated service, our customer service is spread across large listed companies, military research units, and small and medium-sized enterprises. Our biggest advantage is emphasizing the service-first concept and achieving fast delivery and favorable prices!
Direct brands: Xilinx ALTERA ADI TI NXP ST E2V, Micron and more than a hundred component brands, especially good at components banned from the US to China,Welcome engineer friends to recommend us to procurement or consult us personally!We will continue to provide the best service in the industry!
FPGA technical group official thanks to the brands: Xilinx, Intel (Altera), Microsemi (Actel), Lattice, Vantis, Quicklogic, Lucent, etc.