Basic Principles of Arduino Serial Communication
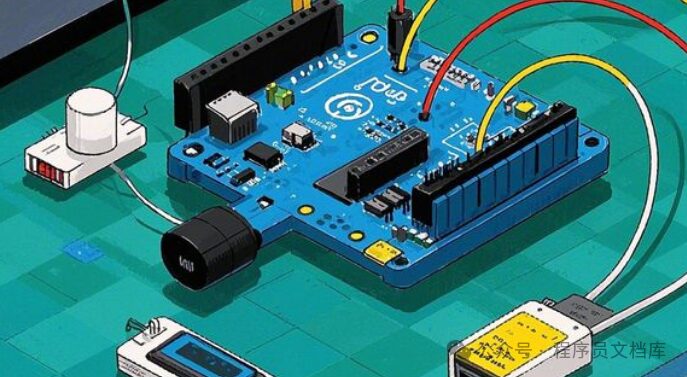
-
Communication Methods and Implementation
Serial communication is a method of transmitting data in a bit-wise order. In Arduino, serial communication is mainly achieved through UART (Universal Asynchronous Receiver-Transmitter). UART utilizes two pins, TX (transmit) and RX (receive), to complete the data sending and receiving tasks.
Data is transmitted in frames, with each frame containing components such as start bits, data bits, parity bits, and stop bits. The start bit is usually a low level, marking the beginning of a frame of data transmission; the data bits carry the actual data to be sent, for example, Arduino defaults to using 8 data bits, meaning 1 byte of data can be transmitted at a time; the parity bit is used for error checking and can be set to odd, even, etc., but Arduino defaults to no parity bit; the stop bit is generally a high level, indicating the end of data transmission for that frame.
Development boards like Arduino Uno connect to the computer’s USB port via RX (0) and TX (1) digital pins through a serial conversion chip, enabling communication with the computer. Arduino Mega is relatively more complex, as it has three additional serial ports: Serial 1 uses RX (19) and TX (18), Serial 2 uses RX (17) and TX (16), and Serial 3 uses RX (15) and TX (14). To communicate with a personal computer using these three serial ports, an additional USB-to-serial adapter is required. When communicating with external TTL serial devices, the TX pin should be connected to the corresponding device’s RX pin, and the RX pin should be connected to the device’s TX pin, while the GND should be connected to the device’s GND (be careful not to connect these pins directly to RS232 ports, as their operating voltage is +/- 12V, which may damage the Arduino control board).
-
Key Settings for Baud Rate
The baud rate is a physical quantity that measures the speed of data transmission over a serial line, indicating the number of bits (or bytes, depending on the specific definition and context) transmitted per second. The Arduino Serial library provides functions to set the baud rate, commonly using Serial.begin(9600), where 9600 is the specified baud rate value.
When performing serial communication, the baud rates of the sending and receiving devices must match; otherwise, communication will fail. For example, if one device is set to a baud rate of 9600 and another device communicating with it is set to 115200, then neither will correctly recognize the data sent by the other.
Additionally, choosing the appropriate baud rate is critical. A baud rate that is too high may lead to data loss because the data transmission speed is too fast for the receiving end to process, or the serial buffer may not be able to store incoming data in time; conversely, a baud rate that is too low could affect communication efficiency, causing data transmission to be too slow, which may not meet the requirements in some real-time scenarios. For simple debugging scenarios, a relatively low baud rate like 9600 is commonly used for easy data observation; while for projects requiring higher transmission speeds, a higher baud rate like 115200 may be selected. Furthermore, parameters such as data bits, parity bits, and stop bits can also be configured via Serial.begin(speed, config); for example, Serial.begin(9600, SERIAL_8E2) sets the serial baud rate to 9600, data bits to 8 bits, uses even parity, and stop bits to 2.
Analysis of Common Arduino Serial Communication Functions
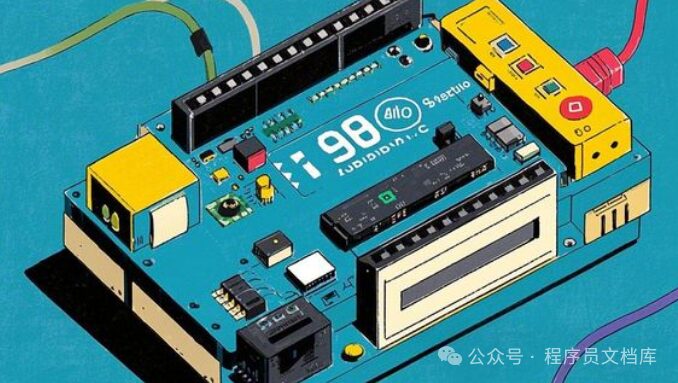
-
Data Sending Functions
In Arduino, common data sending functions include Serial.print() and Serial.println(), which help us send various types of data from Arduino.
The Serial.print() function can send multiple data types, such as strings and numbers. For example, Serial.print(“Hello, world!”) will send the string “Hello, world!”, while Serial.print(123) can send the number 123. It also supports formatted output, such as Serial.print(78, BIN) will output “1001110” (binary form of 78), Serial.print(78, OCT) will output “116” (octal form of 78), Serial.print(78, DEC) will output “78” (decimal form of 78), and Serial.print(78, HEX) will output “4E” (hexadecimal form of 78), etc.
The Serial.println() function is similar to Serial.print(), with the difference that Serial.println() adds a carriage return and newline. For instance, Serial.println(b) outputs the ASCII value of b in decimal form, followed by a carriage return and newline; Serial.println(b, DEC) also outputs the ASCII value of b in decimal form with a carriage return and newline; Serial.println(b, HEX) outputs the ASCII value of b in hexadecimal form, accompanied by a carriage return and newline; Serial.println(b, OCT) outputs the ASCII value of b in octal form, also with a carriage return and newline; Serial.println(b, BIN) outputs the ASCII value of b in binary form, similarly with a carriage return and newline; Serial.print(b, BYTE) outputs b as a single byte and follows with a carriage return and newline; if the parameter is a string or array, such as Serial.println(str), it will output the entire ASCII encoded string of str; while using Serial.println() alone will only output a carriage return and newline.
In practical use, it is essential to ensure that the encoding format of the Arduino board and the device it communicates with (e.g., the serial monitor on the computer side) are consistent, especially when sending Chinese characters from Arduino, as the encoding format is based on the code file’s encoding format; thus, the serial monitor’s encoding format should be set to match that of the code file to ensure correct data transmission and display.
-
Data Receiving Functions
The main functions for receiving serial data in Arduino are Serial.read() and Serial.available(), mastering their usage is crucial for correctly obtaining data sent from external sources.
The Serial.available() function returns the number of characters currently remaining in the serial buffer. We generally use this function to determine whether there is data in the serial buffer. When Serial.available() > 0, it indicates that data has been received, and reading operations can be performed; conversely, if Serial.available() equals 0, it indicates that there is currently no data to read in the buffer.
The Serial.read() function is used to read and retrieve one byte of data from the serial buffer. For example, when a device sends data to Arduino via the serial port, we can use Serial.read() to read the sent data. A typical usage is to read data through a code logic similar to the following:
while (Serial.available() > 0) {
int data = Serial.read();
// Further processing of the read data can be done here, such as judging, converting, etc.
}
The above code means that when data is received via the serial port (determined by Serial.available() > 0), it repeatedly reads the data from the serial port and assigns it to a variable (like the data here, through Serial.read()), until all data is read (i.e., when Serial.available() = 0).
Additionally, when reading specific line data from serial communication, the following steps can be implemented: first, use the Serial.begin() function to initialize serial communication and set the baud rate; then use the Serial.available() function to check if there is available data to read; next, use the Serial.read() function to read characters one by one, storing them in a buffer, and when a newline character (‘\n’) is read, it indicates that a line of data has been completely read, at which point the read line data can be processed, such as outputting to the serial monitor or performing further business logic operations, etc., and after processing that line of data, the line buffer can be cleared in preparation for reading the next line of data.
Common Issues and Solutions in Arduino Serial Communication
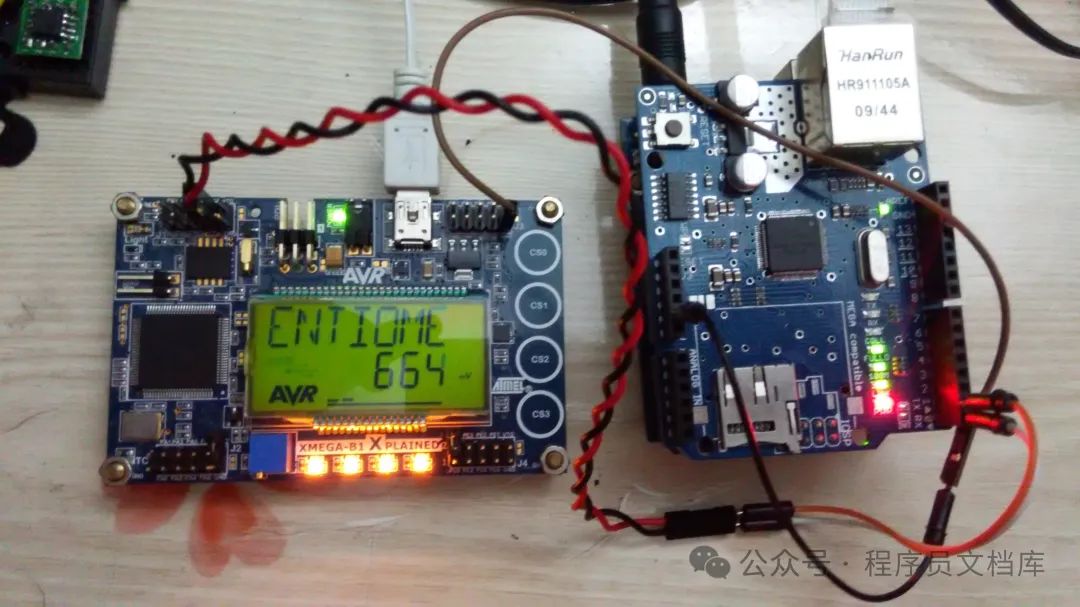
-
Hardware Connection Issues
In Arduino serial communication, various hardware connection issues may affect normal communication.
First, the serial cable is a part that is prone to issues; check whether the serial cable is properly connected, and the tightness of the cable should be moderate to avoid looseness or damage. Sometimes, if the serial cable itself is of poor quality or has malfunctioned after long-term use, it may lead to communication interruptions; in this case, try replacing it with a new serial cable to see if that resolves the issue.
Secondly, incorrect serial port selection is also a common situation. For example, in the Arduino IDE, it is necessary to accurately select the serial port number corresponding to the actual connection; if selected incorrectly, the computer will be unable to communicate with the Arduino board through the correct serial channel. You can view and select available serial ports under the “Tools” menu in the Arduino IDE to ensure the selection is correct.
Moreover, for development boards like Arduino Uno, which communicate with the computer’s USB port through digital pins 0 (RX) and 1 (TX), while Arduino Mega has multiple additional serial ports (Serial 1 uses 19 (RX) and 18 (TX), Serial 2 uses 17 (RX) and 16 (TX), Serial 3 uses 15 (RX) and 14 (TX)), if using these additional serial ports to communicate with a computer, an additional USB-to-serial adapter must be equipped, and when connecting, ensure that the pins correspond correctly, connecting the TX pin to the corresponding device’s RX pin, the RX pin to the device’s TX pin, and the GND to the device’s GND, while also being particularly careful not to connect these pins directly to RS232 ports (as their operating voltage is +/- 12V, which may damage the Arduino control board). If communication is abnormal after hardware connections, you can use a multimeter to test the continuity of the cable and check the continuity between each pin to ensure correct connections.
-
Serial Port Setting Issues
Setting serial port parameters is crucial in Arduino serial communication; once parameter settings are inconsistent, communication failures can easily occur.
The baud rate setting must be consistent between the sending and receiving devices. For instance, if the baud rate set on the Arduino side is 9600, while the baud rate of the computer’s serial monitor or other receiving device is set to 115200, then neither party will be able to correctly recognize the data sent by the other, and communication cannot proceed normally. Common baud rates include 9600, 115200, etc.; it is essential to choose the appropriate baud rate based on specific communication requirements and device support.
In addition to baud rate, parameters such as data bits, stop bits, and parity bits are also critical. Arduino defaults to using 8 data bits, no parity bits, and 1 stop bit, but in some special communication scenarios or when communicating with specific devices, these parameters may need to be adjusted. For example, if a device requires even parity, then in the Arduino code, the serial baud rate should be set to 9600, with data bits set to 8 bits, even parity, and 2 stop bits, by using a format like Serial.begin(9600, SERIAL_8E2) to ensure compatibility with the device’s requirements. When encountering communication issues, carefully verify the serial port parameters of both devices; if inconsistent, promptly adjust them in the code or the receiving device’s configuration to restore normal communication.
-
Code Issues
There are often errors in the Arduino code related to serial communication that affect the correct sending and receiving of data.
On one hand, improper function usage is quite common. For example, when using the Serial.read() function, if the Serial.available() function is not first used to check whether there is data available in the serial buffer, it may lead to reading empty data or errors in the program. The correct approach is to follow a code logic like this:
while (Serial.available() > 0) {
int data = Serial.read();
// Further processing of the read data can be done here, such as judging, converting, etc.
}
That is, first check that there is data in the buffer before reading. Additionally, it is important to understand the difference between Serial.print() and Serial.println(); Serial.println() includes a carriage return and newline, so if you expect the output to display data after a newline, you should use the correct function; otherwise, the display effect may not meet expectations.
On the other hand, syntax errors should not be overlooked. Syntax issues such as unmatched parentheses or undefined variables can lead to compilation failures or runtime exceptions, affecting the implementation of serial communication functionality. Moreover, when sending and receiving data, appropriate delays may sometimes need to be added; for instance, when continuously sending multiple data rapidly, if there are no delays, the receiving end may not process the incoming data in time, leading to data loss or communication errors. Therefore, when checking the code, pay attention to these aspects, verify that functions are used correctly, syntax is correct, and whether necessary delays are missing, and promptly correct any issues in the code to ensure that the serial communication code can correctly implement data sending and receiving.
Arduino Serial Communication Project Examples and Practice
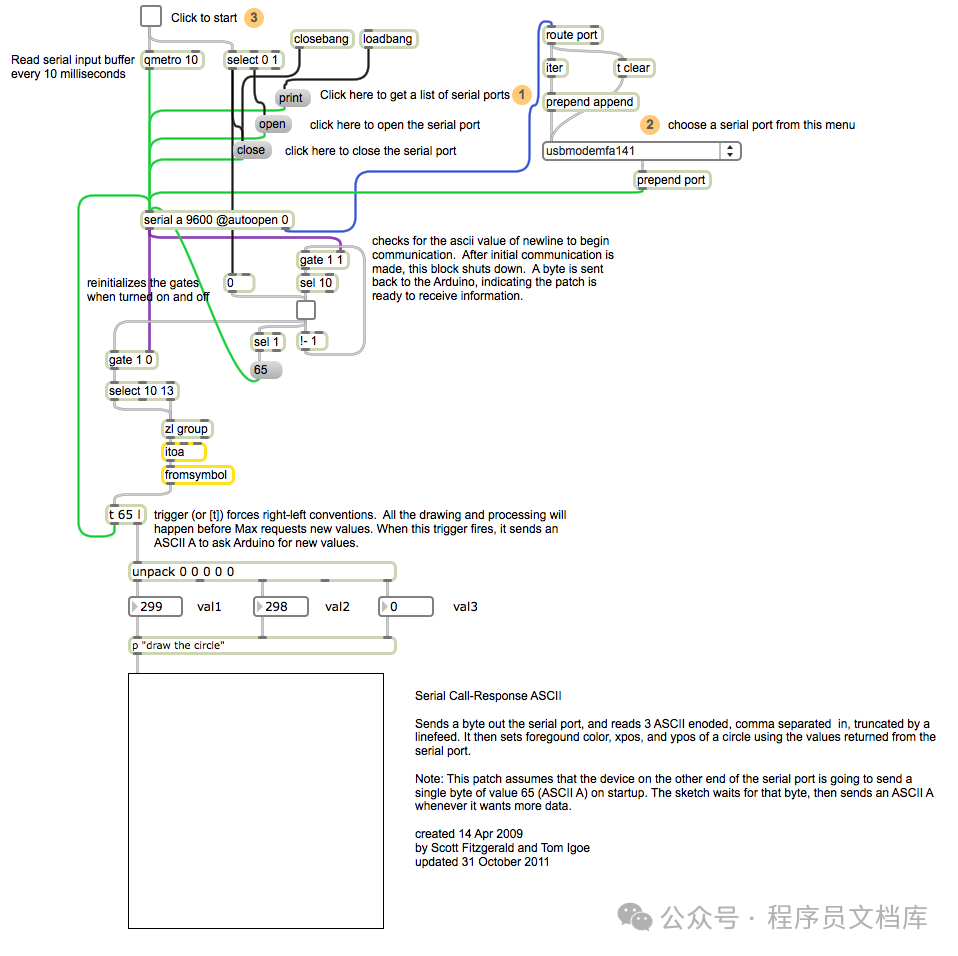
-
Simple Project Example
The following is a basic project example of Arduino communicating with a computer via serial, demonstrating the complete process from hardware connection to software programming for bidirectional communication, making it convenient for you to practice.
-
Hardware Connection:
Prepare an Arduino Uno development board, connecting it to the computer via a USB data cable. The 0 pin (RX) and 1 pin (TX) of the Arduino Uno are used for serial communication, connecting to the computer’s USB port through the serial conversion chip on the development board for data exchange with the computer.
-
Software Programming:
Open the Arduino IDE, create a new blank project file, and enter the following code:
void setup() {
Serial.begin(9600); // Initialize serial communication, set baud rate to 9600; this baud rate can be adjusted according to actual needs, but must match the receiving end
}
void loop() {
// Send data to the computer
Serial.print("Hello from Arduino!"); // Send string
Serial.println(); // Send a newline character, equivalent to Serial.println("");
delay(1000); // Delay 1 second to avoid sending data too frequently
// Check if there is data received from the computer
if (Serial.available() > 0) {
// Read the received data
String data = Serial.readStringUntil('\n'); // Read until newline character
// Print the received data
Serial.print("Received: ");
Serial.println(data);
}
}
In the above code, in the setup function, Serial.begin(9600) initializes the serial port, setting the baud rate to 9600. In the loop function, it first uses Serial.print and Serial.println functions to send data to the computer, then uses Serial.available function to check if there is data coming from the computer; if there is, it reads data until it encounters a newline character using Serial.readStringUntil, and prints the received data, thus achieving simple bidirectional serial communication between Arduino and the computer.
-
Debugging and Testing Methods
Debugging is an important part of Arduino serial communication; below are several common debugging methods.
Using the Serial Monitor in Arduino IDE:
Arduino IDE comes with a useful tool called the Serial Monitor. The steps are as follows:
First, ensure your Arduino development board is correctly connected to the computer via USB and that the corresponding serial communication program code has been uploaded to the board. Then, in the Arduino IDE, click on the “Tools” menu and select the “Serial Monitor” option (you can also use the shortcut Ctrl + Shift + M) to open the Serial Monitor window. In the window, you need to select the correct baud rate, which should match the baud rate set in your code (for example, 9600 in the previous example).
Once opened, you will see the data sent from Arduino in this window. For example, when Arduino executes the Serial.print(“Hello from Arduino!”); statement, the corresponding string will display in the Serial Monitor. You can also input data in the Serial Monitor’s input box and send it (press the enter key to send); if the Arduino side has data receiving logic (such as Serial.available and Serial.read related code), it will receive the data you send and process and display it accordingly. By observing whether the sent and received data meets expectations, you can determine whether the communication is normal.
Using Other Serial Debugging Tools:
There are many useful serial debugging tools available on the market, such as:
- XCOM Serial Debugging Assistant
: After installing the software, the system will automatically recognize available serial ports upon startup; you can also manually input relevant messages for debugging. It supports single and multiple sending operations, multi-port operations, and includes detailed help to guide users. For example, when debugging serial communication between multiple Arduino boards or between Arduino and multiple different serial devices, the multi-port operation feature allows you to conveniently view the data situation for each communication link.
- Awen Serial Debugging Assistant
: This software is compact and easy to use, able to adjust, verify, and debug baud rates ranging from 300 to 115200. If you need to try different baud rates in a project to find the most suitable communication settings, it can be very helpful, meeting diverse usage needs, whether for learning or debugging serial communication in work scenarios.
- Deep Blue Serial Debugging Tool
: Utilizing powerful multithreading technology, it supports modifying serial baud rates, parity bits, data bits, and serial stop bits, and also supports manual or automatic data saving, effectively preventing data loss. When it is necessary to finely adjust various serial parameters or when there are concerns about data loss affecting debugging results, it is an excellent choice.
When using these serial debugging tools, it is also important to first connect and configure them with the corresponding serial port of the Arduino development board (generally selecting the correct serial port number and setting the baud rate, etc.), and then you can send data to Arduino and view the data returned by Arduino, analyzing the situation of sent and received data.