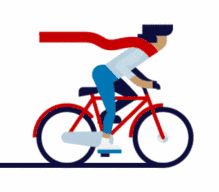
Click the blue text above to follow us
Transitioning embedded SoC development from single-process multi-threaded architecture to multi-process architecture is a task that requires careful planning and implementation, especially in resource-constrained embedded systems.
This architectural change is typically aimed at improving system stability, isolation, security, and concurrent processing capabilities.
In a single-process multi-threaded architecture, multiple threads share the same memory space, file descriptors, and global variables, which is suitable for handling lightweight concurrent tasks.
However, the drawbacks of this architecture include:
-
Complex shared resource management: Threads sharing memory can easily lead to data races and resource conflicts, especially when lock mechanisms are misused.
-
High impact of crashes: If one thread crashes, the entire process is affected, leading to system instability.
-
Difficult debugging: Debugging memory issues and thread synchronization problems is complex due to shared state among threads.
The multi-process architecture divides the application into multiple independent processes, each with its own memory space and resource control.
The benefits of this architecture include:
-
Enhanced stability: A crash in one process does not affect other processes, making the system more resilient.
-
Resource isolation: Different processes are independent of each other, reducing data race issues.
-
Improved security: Different processes can run under different permissions, enhancing system security.
1
Steps for Architecture Migration
1. Analyze system functional modules
First, analyze the functional modules in the existing single-process multi-threaded architecture.
Identify which modules can run independently and determine which modules have tight communication dependencies.
These dependencies will be handled via inter-process communication (IPC) in the multi-process architecture.
2. Determine process division
Design the system as multiple independent processes based on the functional module division.
For example:
-
Data collection process: Specifically handles sensor data collection.
-
Data processing process: Performs data analysis and processing.
-
Communication process: Responsible for communication with external devices or servers.
-
User interface process: Interacts with the user.
3. Implement inter-process communication (IPC)
In a multi-process architecture, the shared memory of threads becomes independent, so information needs to be transmitted through inter-process communication mechanisms.
Common IPC mechanisms include:
-
Message queues: Suitable for passing short messages, with a priority mechanism.
-
Shared memory: Used for efficient transmission of large amounts of data, but requires additional synchronization control.
-
Semaphores: Used to synchronize operations between processes, ensuring orderly execution.
-
Pipes: Unidirectional communication channels suitable for data transmission between parent and child processes.
-
Sockets: Used for communication between different devices or across networks.
4. Process creation and management
In a multi-process architecture, use fork() system call or other multi-process frameworks to create multiple processes.
You can manage the startup, stopping, and monitoring of child processes through daemon processes.
5. Exception handling and monitoring
Use wait() and waitpid() system calls to monitor the status of child processes, ensuring that processes can exit or restart correctly.
You can design a monitoring process to regularly check the health status of each child process.
6. Resource allocation and optimization
In a multi-process architecture, since each process has its own memory space, resource allocation and usage need to be controlled more precisely.
You can optimize memory usage across different processes through a memory sharing pool to ensure the performance of the embedded system.
2
Key Points of Architecture Migration
Performance and resource management
The use of multi-process architecture in embedded systems may increase memory and resource overhead since each process has its own independent address space and resources.
To optimize performance, consider using lightweight IPC mechanisms or adjusting the number and distribution of processes according to needs.
Choice of process communication
Besides shared memory, inter-process communication can also use message queues, pipes, or sockets, choosing the most suitable method based on actual needs.
Although shared memory is efficient for large data transfers, it requires synchronization handling (e.g., using semaphores) to avoid concurrent access conflicts.
Stability and isolation
The multi-process architecture greatly enhances the isolation of the system.
If a process crashes, other processes can continue to work normally, improving system stability.
By monitoring the status of child processes from the parent process, automatic restart functionality can be implemented in case of crashes, further enhancing the system’s fault tolerance.
3
Migration Example
Here, we take a simple embedded system as an example to gradually demonstrate how to transition from a single-process multi-threaded architecture to a multi-process architecture.
In this architecture, the system has three main functional modules:
-
Temperature sensor data collection thread.
-
Data processing thread.
-
Communication module thread, which sends processed data to a remote server.
These threads share the same memory space, exchanging data through shared global variables.
The original multi-threaded code is as follows:
// Global shared variablefloat temperature_data = 0.0; // Simulate reading temperature sensor datafloat read_temperature_sensor() { return 25.0 + (rand() % 10); // Randomly simulate temperature} // Simulate processing datavoid process_temperature_data(float temp) { printf("Processing temperature data: %.2f\n", temp); sleep(1); // Simulate data processing time} // Simulate sending data to servervoid send_data_over_network(float temp) { printf("Sending data over network: %.2f\n", temp); sleep(1); // Simulate network communication time} // Temperature collection threadvoid* temperature_thread(void* arg) { while (1) { temperature_data = read_temperature_sensor(); printf("Temperature read: %.2f\n", temperature_data); sleep(2); // Simulate collection period } return NULL; } // Data processing threadvoid* processing_thread(void* arg) { while (1) { process_temperature_data(temperature_data); } return NULL; } // Communication threadvoid* communication_thread(void* arg) { while (1) { send_data_over_network(temperature_data); } return NULL; } int main() { pthread_t temp_thread, proc_thread, comm_thread; // Create three threads pthread_create(&temp_thread, NULL, temperature_thread, NULL); pthread_create(&proc_thread, NULL, processing_thread, NULL); pthread_create(&comm_thread, NULL, communication_thread, NULL); // Wait for threads to complete pthread_join(temp_thread, NULL); pthread_join(proc_thread, NULL); pthread_join(comm_thread, NULL); return 0;}
Problems exist:
-
Data races: Multiple threads accessing and modifying the same global variable temperature_data can easily lead to data races.
-
Stability: If one thread crashes, the entire process will terminate.
-
Difficult debugging: Debugging memory issues is complex due to shared resources among threads.
Optimizations for the multi-process architecture:
Creating shared memory
Use ftok() to generate a unique key to identify the shared memory.
shmget() creates a shared memory segment, specifying the size of the shared memory as sizeof(float) to store temperature data.
shmat() maps the shared memory to the process’s address space, allowing each child process to access this shared memory.
Creating child processes
Use fork() to create three child processes, each responsible for temperature data collection, data processing, and data sending.
Each child process has its own independent memory space but exchanges data through shared memory.
Data transmission
Temperature collection process: Responsible for reading sensor data and writing data into shared memory’s temperature_data.
Data processing process: Reads temperature data from shared memory and performs corresponding processing operations.
Communication process: Reads processed data from shared memory and sends it over the network to a remote server.
Process management
The parent process uses the wait() function to wait for the execution of child processes to end, ensuring all child processes run correctly.
When the system terminates, the parent process will detach and delete the shared memory to prevent memory leaks.
The optimized multi-process architecture code is as follows:
// Simulate reading temperature sensor datafloat read_temperature_sensor() { return 25.0 + (rand() % 10); // Randomly simulate temperature} // Simulate processing datavoid process_temperature_data(float temp) { printf("Processing temperature data: %.2f\n", temp); sleep(1); // Simulate data processing time} // Simulate sending data to servervoid send_data_over_network(float temp) { printf("Sending data over network: %.2f\n", temp); sleep(1); // Simulate network communication time} int main() { // Create shared memory segment key_t key = ftok("shmfile", 65); // Generate shared memory key int shmid = shmget(key, sizeof(float), 0666 | IPC_CREAT); // Create shared memory float *temperature_data = (float *) shmat(shmid, NULL, 0); // Map shared memory to process address space // Parent process creates child processes pid_t pid = fork(); if (pid == 0) { // Child process 1: responsible for temperature collection while (1) { *temperature_data = read_temperature_sensor(); printf("Temperature read: %.2f\n", *temperature_data); sleep(2); // Simulate collection period } } else { pid_t pid2 = fork(); if (pid2 == 0) { // Child process 2: responsible for data processing while (1) { process_temperature_data(*temperature_data); } } else { pid_t pid3 = fork(); if (pid3 == 0) { // Child process 3: responsible for data sending while (1) { send_data_over_network(*temperature_data); } } else { // Parent process: wait for all child processes wait(NULL); // Wait for the first child process to finish wait(NULL); // Wait for the second child process to finish wait(NULL); // Wait for the third child process to finish // Detach and delete shared memory shmdt(temperature_data); shmctl(shmid, IPC_RMID, NULL); } } } return 0;}
Transitioning from a single-process multi-threaded architecture to a multi-process architecture can significantly improve the stability, isolation, and security of embedded systems.
Through inter-process communication mechanisms such as shared memory and message queues, effective data exchange between processes can be achieved.
Although the multi-process architecture incurs some resource overhead, its advantages are very clear when handling complex applications, especially for systems requiring high concurrency, fault tolerance, and modular design.
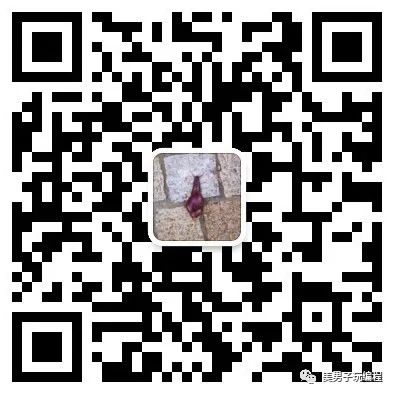