Hello everyone, I am Alisa.
The topic we are going to discuss is very interesting, and programmers who deal with hardware usually feel very excited—how to use Python to control hardware and embedded systems programming.
You heard it right, our goal today is to understand how to use Python to control hardware devices and even enter the world of embedded systems programming.
Some of you may be thinking, “Python is so advanced, how can it directly interact with hardware?”
Don’t worry, today I will take you to explore how to use Python to interact with hardware, communicate with embedded systems, and demonstrate a series of practical tips and steps.
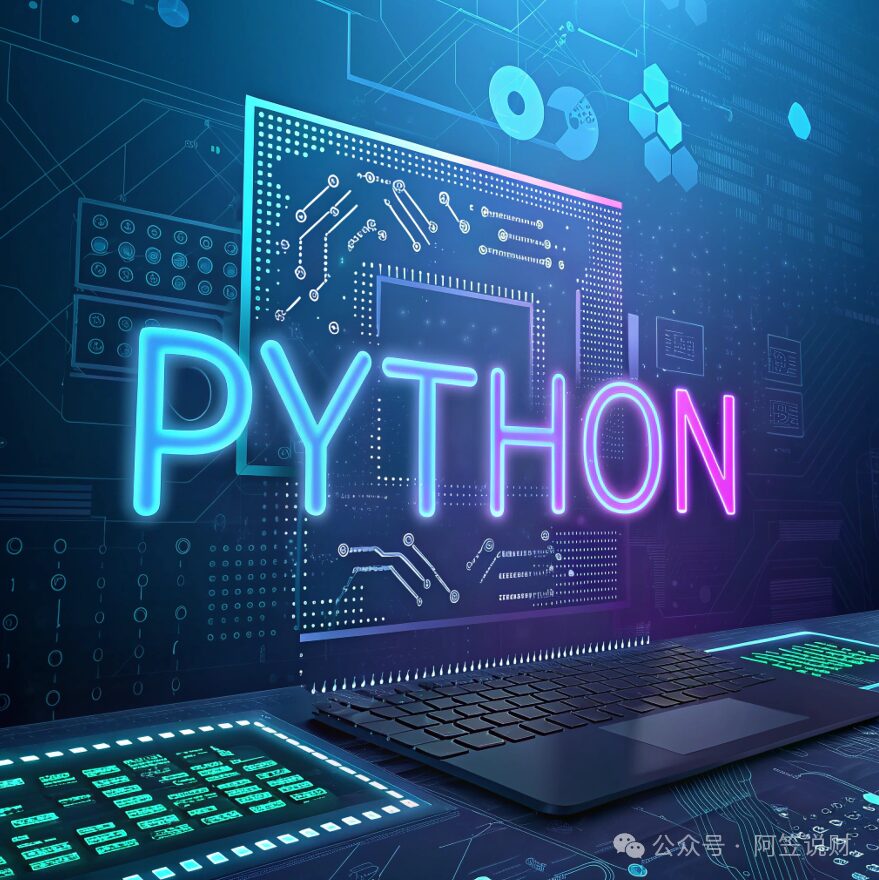
The World of Hardware Programming: You Are No Longer an Outsider
Hardware programming generally refers to controlling the behavior of hardware devices through software. In the past, hardware programming often involved low-level languages such as C or assembly language. However, with the rise of open-source hardware platforms and the popularity of Python, Python’s role in hardware development has gradually increased, especially in the fields of embedded systems and the Internet of Things (IoT).
How to Control Hardware with Python
Python does not directly interact with hardware, but with the help of some external libraries, we can easily interact with hardware. We can achieve interaction with hardware in several ways:
-
GPIO (General Purpose Input Output) Control: Control the input and output pins on Raspberry Pi or similar single-board computers. -
Serial Communication: Exchange data with hardware through serial ports (like USB). -
I2C and SPI Buses: Control devices such as sensors and displays. -
USB Devices and HID (Human Interface Devices): Control USB devices like keyboards, mice, or other peripherals through Python.
Among these, we will focus on how to control hardware on Raspberry Pi using Python and how to perform serial communication. Additionally, we will cover the basic concepts of embedded systems programming.
Controlling GPIO Pins on Raspberry Pi
Raspberry Pi is one of the most popular development boards, featuring a rich set of hardware interfaces and supporting multiple communication protocols. With Raspberry Pi, you can control various hardware devices using Python, including sensors, lights, motors, etc. One of the most commonly used libraries is <span>RPi.GPIO</span>
, which allows you to control the GPIO pins on Raspberry Pi.
3.1 Installing RPi.GPIO Library
First, we need to install the <span>RPi.GPIO</span>
library on Raspberry Pi. For most Raspberry Pi operating systems, <span>RPi.GPIO</span>
is already pre-installed. If not, you can install it with the following command:
sudo apt-get update
sudo apt-get install python3-rpi.gpio
3.2 Controlling LED Blinking
Next, we will understand how to use Python to control the blinking of an LED through a classic example.
First, prepare the hardware:
-
An LED -
A 330Ω resistor -
A jumper wire (connected to the GPIO pin of Raspberry Pi)
Connection method:
-
The long leg (positive) of the LED is connected to GPIO pin 17 of Raspberry Pi. -
The short leg (negative) of the LED is connected to a 330Ω resistor, then connected to the GND pin of Raspberry Pi via a jumper wire.
3.3 Python Code to Control LED
import RPi.GPIO as GPIO
import time
# Set GPIO mode
GPIO.setmode(GPIO.BCM)
# Set GPIO 17 as output mode
GPIO.setup(17, GPIO.OUT)
# Control LED blinking
try:
while True:
GPIO.output(17, GPIO.HIGH) # Turn on LED
time.sleep(1) # Wait 1 second
GPIO.output(17, GPIO.LOW) # Turn off LED
time.sleep(1) # Wait 1 second
except KeyboardInterrupt:
pass
finally:
GPIO.cleanup() # Clean up all GPIO settings
Explanation:
-
We set the GPIO pin numbering method (BCM numbering) using <span>GPIO.setmode(GPIO.BCM)</span>
. -
Then we set GPIO pin 17 to output mode using <span>GPIO.setup(17, GPIO.OUT)</span>
. -
Using <span>GPIO.output(17, GPIO.HIGH)</span>
to turn on the LED, and<span>GPIO.LOW</span>
to turn it off. -
Using <span>time.sleep(1)</span>
to make the LED blink once per second.
This example is very simple, but it demonstrates how to control hardware devices using Python.
3.4 Controlling a Button
In addition to controlling the LED, we can also read the state of a button using Python. We can use <span>GPIO.input()</span>
to read the pin level state.
import RPi.GPIO as GPIO
# Set GPIO mode
GPIO.setmode(GPIO.BCM)
# Set GPIO 17 as input mode (connected to button)
GPIO.setup(17, GPIO.IN, pull_up_down=GPIO.PUD_UP)
try:
while True:
input_state = GPIO.input(17)
if input_state == GPIO.LOW:
print("Button pressed!")
else:
print("Button not pressed!")
except KeyboardInterrupt:
pass
finally:
GPIO.cleanup() # Clean up all GPIO settings
Explanation:
-
The button is connected to GPIO pin 17, set to input mode with a pull-up resistor enabled ( <span>GPIO.PUD_UP</span>
). -
<span>GPIO.input(17)</span>
checks if the button is pressed. When the button is pressed, the pin level is LOW.
Serial Communication: Another Way for Python to Interact with Hardware
Serial communication is a common way to communicate with external hardware devices, especially in embedded systems, where many sensors and devices exchange data with the host via serial ports. In Python, we can use the <span>pyserial</span>
library for serial communication.
4.1 Installing pyserial
pip install pyserial
4.2 Serial Communication Example: Communicating with Arduino
Assuming you have an Arduino that sends data to Raspberry Pi via serial, we can use Python to read this data.
import serial
# Set up serial port (assuming Arduino is connected to /dev/ttyUSB0)
ser = serial.Serial('/dev/ttyUSB0', 9600)
# Loop to read serial data
try:
while True:
if ser.in_waiting > 0:
data = ser.readline().decode('utf-8').strip()
print(f"Received data: {data}")
except KeyboardInterrupt:
pass
finally:
ser.close() # Close serial connection
Explanation:
-
We open the serial port using <span>serial.Serial('/dev/ttyUSB0', 9600)</span>
, specifying the baud rate as 9600. -
<span>ser.readline()</span>
reads a line of data, and<span>decode('utf-8')</span>
converts the byte data to a string. -
<span>ser.in_waiting</span>
is used to check if there is data available to read.
Basic Concepts of Embedded Systems Programming
In embedded systems, we usually need to write low-level code to interact directly with hardware. Although Python is not suitable for efficient embedded programming, it is very suitable for some basic control and automation tasks.
5.1 Embedded Linux Environment
In embedded development, many devices run Linux systems, especially ARM-based devices like Raspberry Pi and BeagleBone. Python is one of the commonly used scripting languages in these embedded Linux environments, particularly suitable for writing control scripts, data collection, sensor reading, and other tasks.
We can communicate with hardware through interfaces such as serial, I2C, and SPI, and we can also use Python to write device drivers or control hardware features like GPIO, PWM, and ADC.
5.2 Driver Development for Embedded Devices
For lower-level operations, such as writing device drivers or manipulating hardware control registers, C language is usually needed. However, if only simple interactions with hardware devices (like reading sensor data) are required, Python is very efficient.
For example, we can communicate with sensors (like temperature and humidity sensors) via the I2C bus:
import smbus
# Initialize I2C bus
bus = smbus.SMBus(1)
# I2C device address (for example, DHT22 temperature and humidity sensor)
address = 0x40
# Read data
data = bus.read_i2c_block_data(address, 0x00, 4)
print(data)
In this example, we use the <span>smbus</span>
library to communicate with an I2C device (like a temperature and humidity sensor) to read data and print the output.
Conclusion
Through today’s learning, we understood how to use Python to control hardware, especially GPIO control, serial communication, and I2C bus operations on Raspberry Pi. Additionally, we delved into the basic concepts of embedded systems programming and how to use Python for simple hardware control and device interaction.
If you are interested in hardware programming, Python is a great starting point; it is concise, easy to use, and powerful.
You can apply Python in the fields of IoT devices, embedded systems, and automation control to develop various interesting projects.
I hope that through today’s content, you can inspire your interest in hardware programming and start implementing your own hardware control projects!