Hello everyone, I am Pi Zi Heng, a serious technical enthusiast. Today, I will share with you three implementations of critical section protection in Cortex-M bare metal environment.
Friends who have worked with embedded systems and RTOS must be familiar with the function codes OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL(). In RTOS, there is often multi-tasking (process) handling, and in some cases, certain special operations (such as Flash erasure in XIP mode, switching to low-power mode) cannot be interrupted arbitrarily, or some shared data areas cannot be accessed in an unordered manner (Task A is reading while Task B is writing). This is where critical section protection strategies come into play.
Simply put, a critical section protection strategy means that multiple tasks must access hardware or software critical resources mutually exclusively. In an RTOS environment, there are ready-made critical section protection interface functions, but there is also a need for such protection in bare metal systems. In bare metal systems, critical section protection is mainly related to global interrupt control. I previously wrote an article titled “General Triple Interrupt Control Design in Embedded MCU,” where the third and highest level of interrupt control is global interrupt control. Today, I will introduce three methods of critical section protection starting from the use of global interrupt control:
1. Critical Section Protection Test Scenarios
There are essentially two scenarios for critical section protection testing. The first scenario involves multiple tasks that are unrelated and do not nest, as shown in the code below. Task1 and Task2 are protected in sequence, so the enter_critical() and exit_critical() functions are always executed in strict pairs:
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected Task 1
do_task1();
// Exit critical section
exit_critical();
// Enter critical section
enter_critical();
// Perform protected Task 2, unrelated to Task 1
do_task2();
// Exit critical section
exit_critical();
}
The second scenario involves multiple tasks that may be related and can nest, as shown in the code below. Task2 is a sub-task of Task1. In this case, you will find that enter_critical() is called twice before exit_critical() is called twice. It is important to note that although the sub-task Task3 inside Task1 is not actively protected like sub-task Task2, since the main task Task1 is protected as a whole, sub-task Task3 should also be protected.
void do_task1(void)
{
// Enter critical section
enter_critical();
// Perform protected Task 2, which is a sub-task of Task 1
do_task2();
// Exit critical section
exit_critical();
// Perform Task 3
do_task3();
}
void critical_section_test(void)
{
// Enter critical section
enter_critical();
// Perform protected Task 1
do_task1();
// Exit critical section
exit_critical();
}
2. Three Implementations of Critical Section Protection
Now that we have clarified the critical section protection test scenarios, let’s move on to the implementation of the enter_critical() and exit_critical() functions:
2.1 Basic Implementation
The first implementation is a very basic approach, which directly wraps the system global interrupt control functions __disable_irq() and __enable_irq(). In the previous test scenario, this implementation can handle non-nested task protection well, but it fails for nested task protection. In the previous test code, Task3 should also be protected, but it is not protected because the exit_critical() immediately opens the global interrupt right after Task2.
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
}
void exit_critical(void)
{
// Enable global interrupts
__enable_irq();
}
2.2 Improved Implementation
Can the basic implementation be improved? Of course! We just need to add a global variable s_lockObject to keep track of the current number of times the critical section has been entered, as shown in the code below. Each time enter_critical() is called, it will disable global interrupts (ensuring the critical section is protected) and increment the count. When exit_critical() is called, it will only enable global interrupts if the current count is 1 (meaning it is not a nested critical section), otherwise, it will simply decrement the count. The improved implementation can clearly protect Task3 in the previous test code.
static uint32_t s_lockObject;
void init_critical(void)
{
__disable_irq();
// Reset counter
s_lockObject = 0;
__enable_irq();
}
void enter_critical(void)
{
// Disable global interrupts
__disable_irq();
// Increment counter
++s_lockObject;
}
void exit_critical(void)
{
if (s_lockObject <= 1)
{
// Only enable global interrupts when counter is not greater than 1, and reset counter
s_lockObject = 0;
__enable_irq();
}
else
{
// When the counter is greater than 1, simply decrement the counter
--s_lockObject;
}
}
2.3 Ultimate Implementation
Although the improved implementation solves the problem of nested task protection, it introduces a global variable and an initialization function, making the implementation less elegant. Moreover, global variables in embedded systems can be easily tampered with, posing certain risks. Is there a better implementation? Yes, this can be achieved by leveraging the special mask register PRIMASK of the Cortex-M processor core. Below is the definition of the PRIMASK register bits (taken from the ARMv7-M manual), where only the lowest bit PM is valid. When PRIMASK[PM] is 1, global interrupts are disabled (raising the execution priority to 0x0/0x80); when PRIMASK[PM] is 0, global interrupts are enabled (no effect on execution priority).
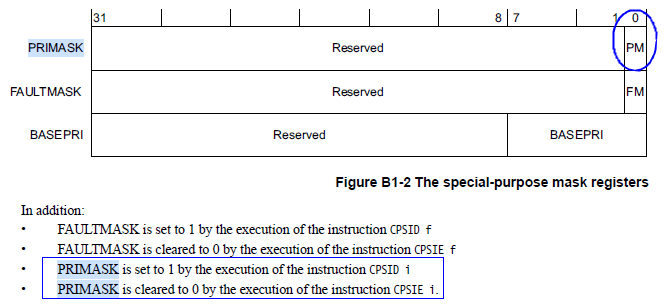
At this point, you should understand that the functions __disable_irq() and __enable_irq() actually operate on the PRIMASK register. Since the PRIMASK register also saves the state of the global interrupt switch, we can obtain the PRIMASK value to replace the global variable s_lockObject in the improved implementation. The code implementation is as follows:
uint32_t enter_critical(void)
{
// Save current PRIMASK value
uint32_t regPrimask = __get_PRIMASK();
// Disable global interrupts (actually set PRIMASK to 1)
__disable_irq();
return regPrimask;
}
void exit_critical(uint32_t primask)
{
// Restore PRIMASK
__set_PRIMASK(primask);
}
Since the prototypes of the enter_critical() and exit_critical() functions have changed, the usage also needs to be adjusted:
void critical_section_test(void)
{
// Enter critical section
uint32_t primask = enter_critical();
// Perform protected task
do_task();
// Exit critical section
exit_critical(primask);
// ...
}
Appendix: PRIMASK Register Setting Functions Implementation in Various IDEs
//////////////////////////////////////////////////////
// Implementation in IAR environment (see cmsis_iccarm.h file)
#define __set_PRIMASK(VALUE) (__arm_wsr("PRIMASK", (VALUE)))
#define __get_PRIMASK() (__arm_rsr("PRIMASK"))
//////////////////////////////////////////////////////
// Implementation in Keil environment (see cmsis_armclang.h file)
__STATIC_FORCEINLINE void __set_PRIMASK(uint32_t priMask)
{
__ASM volatile ("MSR primask, %0" : : "r" (priMask) : "memory");
}
__STATIC_FORCEINLINE uint32_t __get_PRIMASK(void)
{
uint32_t result;
__ASM volatile ("MRS %0, primask" : "=r" (result) );
return(result);
}
Thus, I have introduced the three implementations of critical section protection in the Cortex-M bare metal environment. Where’s the applause~~~