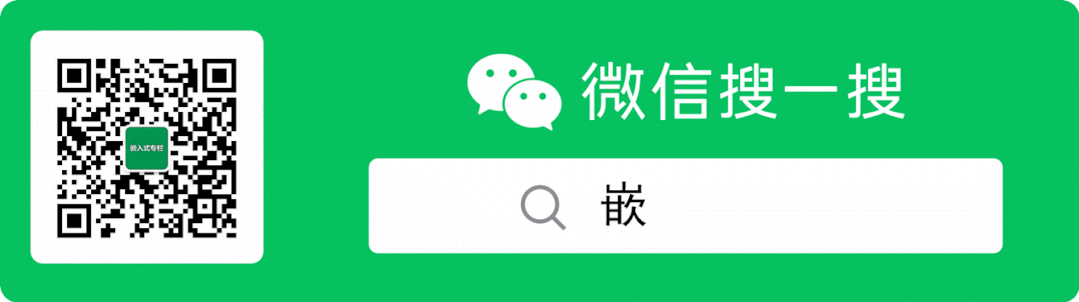
Nowadays, the types and quantities of sensors are increasing, and many of these sensors use analog signals, which rely on ADC.
However, the analog signals collected by our microcontroller’s ADC generally need to go through “filtering” processing before they can be used. Below are some common ADC filtering algorithms.
1. Clipping Filter
1. Method
-
Based on experience, determine the maximum allowable deviation A between two samples
-
When judging each new value: if the difference between the current value and the last value is <= A, then the current value is valid; if the difference is > A, the current value is invalid, and the last value is used instead.
2. Advantages and Disadvantages
-
Overcomes pulse interference but cannot suppress periodic interference; poor smoothness.
3. Code
/* A value is adjusted based on actual conditions, Value is the valid value, new_Value is the current sample value, the program returns the valid actual value */
#define A 10
char Value;
char filter()
{
char new_Value;
new_Value = get_ad(); // Get sample value
if( abs(new_Value - Value) > A) return Value; // abs() function for absolute value
return new_Value;
}
2. Median Filter
1. Method
-
Continuously sample N times, arrange in size order
-
Take the middle value as the valid value
2. Advantages and Disadvantages
-
Overcomes fluctuation interference; has a good filtering effect on slowly changing measured parameters such as temperature; not suitable for rapidly changing parameters.
3. Code
#define N 11
char filter()
{
char value_buf[N];
char count,i,j,temp;
for(count = 0;count < N;count++) // Get sample values
{
value_buf[count] = get_ad();
delay();
}
for(j = 0;j<(N-1);j++)
for(i = 0;i<(N-j);i++)
if(value_buf[i]>value_buf[i+1])
{
temp = value_buf[i];
value_buf[i] = value_buf[i+1];
value_buf[i+1] = temp;
}
return value_buf[(N-1)/2];
}
3. Arithmetic Average Filter
1. Method
-
Continuously sample N times and take the average
-
With a larger N, smoothness is high but sensitivity is low
-
With a smaller N, smoothness is low but sensitivity is high
-
Generally, N=12
2. Advantages and Disadvantages
-
Suitable for systems with random interference; occupies a lot of RAM and is slow.
3. Code
#define N 12
char filter()
{
int sum = 0;
for(count = 0;count<n;count++) (char)(sum="" +="get_ad();" <="" code="" n);="" return="" sum="" }=""></n;count++)>
4. Recursive Average Filter
1. Method
-
Take N sample values to form a queue, first in first out
-
Take the average
-
Generally, N=4~12
2. Advantages and Disadvantages
-
Good suppression of periodic interference, high smoothness
-
Suitable for high-frequency vibration systems
-
Low sensitivity, large RAM usage, serious pulse interference
3. Code
/* A value is adjusted based on actual conditions, Value is the valid value, new_Value is the current sample value, the program returns the valid actual value */
#define A 10
char Value;
char filter()
{
char new_Value;
new_Value = get_ad(); // Get sample value
if( abs(new_Value - Value) > A) return Value; // abs() function for absolute value
return new_Value;
}
5. Median Average Filter
1. Method
-
Sample N values, remove the maximum and minimum
-
Calculate the average of N-2
-
N= 3~14
2. Advantages and Disadvantages
-
Combines the advantages of median and average values
-
Eliminates pulse interference
-
Slow computation speed, high RAM usage
3. Code
char filter()
{
char count,i,j;
char Value_buf[N];
int sum=0;
for(count=0;count<n;count++) for(i="0;i<(N-j);i++)" for(j="0;j<(N-1);j++)" if(value_buf[i]="" value_buf[count]="get_ad();">Value_buf[i+1])
{
temp = Value_buf[i];
Value_buf[i]= Value_buf[i+1];
Value_buf[i+1]=temp;
}
for(count =1;count<n-1;count++) (char)(sum="" (n-2));="" +="Value_buf[count];" <="" code="" return="" sum="" }=""></n-1;count++)></n;count++)>
6. Clipping Average Filter
1. Method
-
Each time the sample data is clipped before being sent to the queue
-
Take the average
2. Advantages and Disadvantages
-
Combines the advantages of clipping, averaging, and queues
-
Eliminates pulse interference; uses more RAM
3. Code
#define A 10
#define N 12
char value,i=0;
char value_buf[N];
char filter()
{
char new_value,sum=0;
new_value=get_ad();
if(Abs(new_value-value)<a) (char)(sum="" ;count<n;count++)="" <="" code="" for(count="0" if(i="N)i=0;" n);="" return="" sum+="value_buf[count];" value_buf[i++]="new_value;" }=""></a)>
7. First Order Lag Filter
1. Method
-
Take a=0~1
-
This filtering result = (1-a) * current sample + a * last result
2. Advantages and Disadvantages
-
Good for periodic interference; suitable for high fluctuation frequency scenarios
-
Low sensitivity, phase lag
3. Code
/* To speed up program processing, take a=0~100 */
#define a 30
char value;
char filter()
{
char new_value;
new_value=get_ad();
return ((100-a)*value + a*new_value);
}
8. Weighted Recursive Average Filter
1. Method
-
Improvement on the recursive average filter, assigning different weights to data at different times, usually with newer data having greater weight, resulting in high sensitivity but low smoothness.
2. Advantages and Disadvantages
-
Suitable for systems with a large lag time constant and short sampling periods; cannot quickly respond to interference for signals with small lag time constants and long sampling periods.
3. Code
/* coe array is the weighting coefficient table */
#define N 12
char code coe[N]={1,2,3,4,5,6,7,8,9,10,11,12};
char code sum_coe={1+2+3+4+5+6+7+8+9+10+11+12};
char filter()
{
char count;
char value_buf[N];
int sum=0;
for(count=0;count<n;count++) (char)(sum="" <="" code="" for(count="0;count<N;count++)" return="" sum+="value_buf[count]*coe[count];" sum_coe);="" value_buf[count]="get_ad();" {="" }=""></n;count++)>
9. Debouncing Filter
1. Method
-
Set a filtering counter
-
Compare the sampled value with the current valid value
-
If the sampled value equals the current valid value, reset the counter
-
If the sampled value does not equal the current valid value, increment the counter
-
If the counter overflows, replace the current valid value with the sampled value, and reset the counter
2. Advantages and Disadvantages
-
Good filtering effect on slowly changing signals; poor effect on rapidly changing signals
-
Avoids jitter near critical values; if the counter overflows and an interference value is sampled, filtering cannot be performed
3. Code
#define N 12
char filter()
{
char count=0,new_value;
new_value=get_ad();
while(value!=new_value)
{
count++;
if(count>=N) return new_value;
new_value=get_ad();
}
return value;
}
10. Clipping Debouncing Filter
1. Method
-
Clip first, then debounce
2. Advantages and Disadvantages
-
Combines the advantages of clipping and debouncing
-
Avoids introducing interference values; not suitable for rapidly changing signals
3. Code
#define A 10
#define N 12
char value;
char filter()
{
char new_value,count=0;
new_value=get_ad();
while(value!=new_value)
{
if(Abs(value-new_value)<a) count++;="" if(count="" {="">=N) return new_value;
new_value=get_ad();
}
return value;
}
}
</a)>
———— END ————
● Column “Embedded Tools”
● Column “Embedded Development”
● Column “Keil Tutorial”
● Selected Tutorials from the Embedded Column
Follow the public account reply “Join Group” to join the technical exchange group according to the rules, reply “1024” to see more content.
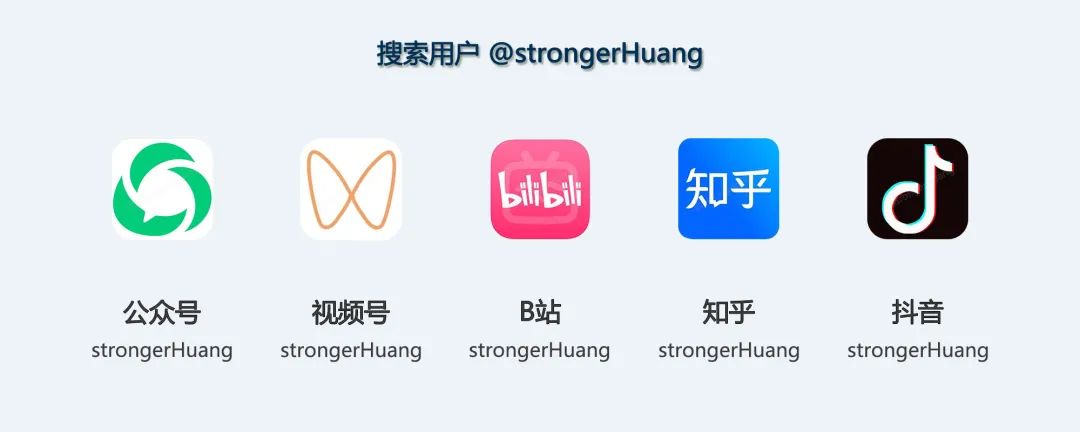
Click “Read the Original” to see more shares.