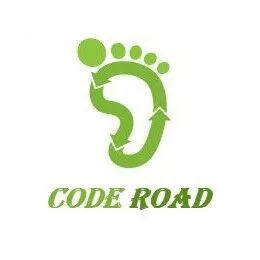
It takes about 6 minutes to read this article.
From: Selected Java Interview Questions
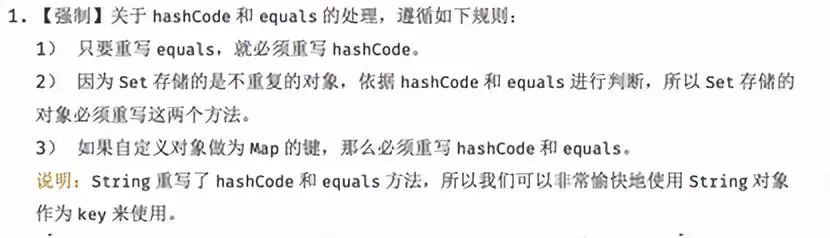
Introduction
equals()
method is used to determine whether two objects are equal.hashCode()
method is used to obtain the hash code, also known as the hash value; it actually returns an int integer. The purpose of this hash code is to determine the index position of the object in the hash table.Relationship
hashCode()
and equals()
in two scenarios based on the “purpose of the class”.1. No “hash table for the class” is created
equals()
method is used to compare whether two objects of this class are equal. The hashCode()
method is completely irrelevant.import java.util.*;
import java.lang.Comparable;
/**
* @desc Compare the values of hashCode() when equals() returns true and false.
*
*/
public class NormalHashCodeTest{
public static void main(String[] args) {
// Create 2 Person objects with the same content,
// and compare them using equals to see if they are equal
Person p1 = new Person("eee", 100);
Person p2 = new Person("eee", 100);
Person p3 = new Person("aaa", 200);
System.out.printf("p1.equals(p2) : %s; p1(%d) p2(%d)\n", p1.equals(p2), p1.hashCode(), p2.hashCode());
System.out.printf("p1.equals(p3) : %s; p1(%d) p3(%d)\n", p1.equals(p3), p1.hashCode(), p3.hashCode());
}
/**
* @desc Person class.
*/
private static class Person {
int age;
String name;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name + " - " +age;
}
/**
* @desc Override equals method
*/
public boolean equals(Object obj){
if(obj == null){
return false;
}
// If it is the same object, return true, otherwise return false
if(this == obj){
return true;
}
// Check if the types are the same
if(this.getClass() != obj.getClass()){
return false;
}
Person person = (Person)obj;
return name.equals(person.name) && age==person.age;
}
}
}
p1.equals(p2) : true; p1(1169863946) p2(1901116749)
p1.equals(p3) : false; p1(1169863946) p3(2131949076)
2. A “hash table for the class” is created
-
If two objects are equal, then their hashCode() values must be the same. Here, equality means that when comparing two objects using equals(), it returns true.
-
If the hashCode() values of two objects are equal, they are not necessarily equal. Because in a hash table, equal hashCode() values mean that the hash values of two key-value pairs are equal. However, equal hash values do not necessarily imply equal key-value pairs. To add,: “Two different key-value pairs can have the same hash value,” which is known as a hash collision.
import java.util.*;
import java.lang.Comparable;
/**
* @desc Compare the values of hashCode() when equals() returns true and false.
*
*/
public class ConflictHashCodeTest1{
public static void main(String[] args) {
// Create a Person object,
Person p1 = new Person("eee", 100);
Person p2 = new Person("eee", 100);
Person p3 = new Person("aaa", 200);
// Create a HashSet object
HashSet set = new HashSet();
set.add(p1);
set.add(p2);
set.add(p3);
// Compare p1 and p2, and print their hashCode()
System.out.printf("p1.equals(p2) : %s; p1(%d) p2(%d)\n", p1.equals(p2), p1.hashCode(), p2.hashCode());
// Print set
System.out.printf("set:%s\n", set);
}
/**
* @desc Person class.
*/
private static class Person {
int age;
String name;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return "("+name + ", " +age+")";
}
/**
* @desc Override equals method
*/
@Override
public boolean equals(Object obj){
if(obj == null){
return false;
}
// If it is the same object, return true, otherwise return false
if(this == obj){
return true;
}
// Check if the types are the same
if(this.getClass() != obj.getClass()){
return false;
}
Person person = (Person)obj;
return name.equals(person.name) && age==person.age;
}
}
}
p1.equals(p2) : true; p1(1169863946) p2(1690552137)
set:[(eee, 100), (eee, 100), (aaa, 200)]
import java.util.*;
import java.lang.Comparable;
/**
* @desc Compare the values of hashCode() when equals() returns true and false.
*
*/
public class ConflictHashCodeTest2{
public static void main(String[] args) {
// Create Person objects,
Person p1 = new Person("eee", 100);
Person p2 = new Person("eee", 100);
Person p3 = new Person("aaa", 200);
Person p4 = new Person("EEE", 100);
// Create a HashSet object
HashSet set = new HashSet();
set.add(p1);
set.add(p2);
set.add(p3);
// Compare p1 and p2, and print their hashCode()
System.out.printf("p1.equals(p2) : %s; p1(%d) p2(%d)\n", p1.equals(p2), p1.hashCode(), p2.hashCode());
// Compare p1 and p4, and print their hashCode()
System.out.printf("p1.equals(p4) : %s; p1(%d) p4(%d)\n", p1.equals(p4), p1.hashCode(), p4.hashCode());
// Print set
System.out.printf("set:%s\n", set);
}
/**
* @desc Person class.
*/
private static class Person {
int age;
String name;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name + " - " +age;
}
/**
* @desc Override hashCode
*/
@Override
public int hashCode(){
int nameHash = name.toUpperCase().hashCode();
return nameHash ^ age;
}
/**
* @desc Override equals method
*/
@Override
public boolean equals(Object obj){
if(obj == null){
return false;
}
// If it is the same object, return true, otherwise return false
if(this == obj){
return true;
}
// Check if the types are the same
if(this.getClass() != obj.getClass()){
return false;
}
Person person = (Person)obj;
return name.equals(person.name) && age==person.age;
}
}
}
p1.equals(p2) : true; p1(68545) p2(68545)
p1.equals(p4) : false; p1(68545) p4(68545)
set:[aaa - 200, eee - 100]
Principles
Recommended Reading:
The graduation project has a landing! An open-source license plate recognition system based on SpringBoot
The incident of the overselling of Feitian Maotai: Please use Redis distributed locks with caution!
Scan the QR code to follow my public account
I have read