1. What are the differences between STM32F1 and F4?
Answer:
Refer to: STM32 Development – Introduction to STM32Different cores: F1 has a Cortex-M3 core, while F4 has a Cortex-M4 core; different main frequencies: F1 runs at 72MHz, while F4 runs at 168MHz; floating-point operations: F1 lacks a floating-point unit, while F4 has one; functional performance: F4 has more peripherals and stronger capabilities than F1, such as GPIO toggling speed, pull-up/down resistor configuration, ADC accuracy, etc.; memory size: F1 has a maximum of 64K internal SRAM, while F4 has 192K (112+64+16).
2. Describe the STM32 startup process?
Answer:
Refer to: STM32 Development – Startup ProcessThrough the Boot pin setting, find the initial address to initialize the stack pointer __initial_sp pointing to the reset program Reset_Handler, set exception interrupt HardFault_Handler, set system clock SystemInit, call C library function _main
3. Describe GPIO?
Answer:
Refer to: STM32 Development – Detailed GPIOGPIO has 8 working modes (gpio_init.GPIO_Mode):(1) GPIO_Mode_AIN Analog Input (2) GPIO_Mode_IN_FLOATING Floating Input (3) GPIO_Mode_IPD Pull-Down Input (4) GPIO_Mode_IPU Pull-Up Input (5) GPIO_Mode_Out_OD Open-Drain Output (6) GPIO_Mode_Out_PP Push-Pull Output (7) GPIO_Mode_AF_OD Alternate Open-Drain Output (8) GPIO_Mode_AF_PP Alternate Push-Pull Output
APB2 is responsible for AD, I/O, advanced TIM, and UART1.APB1 is responsible for DA, USB, SPI, I2C, CAN, UART2345, general TIM, PWR
GPIO Block Diagram Analysis:Refer to: STM32-GPIO Detailed Explanation
4. UART
-
Question 1: Introduction to serial communication methods? Synchronous communication: I2C half-duplex, SPI full-duplex; asynchronous communication: RS485 half-duplex, RS232 full-duplex
-
Question 2: UART configuration? The general steps for UART setup can be summarized as follows: (1) Enable UART clock, enable GPIO clock (2) Reset UART (3) Set GPIO port mode TX GPIO working mode: GPIO_Mode_AF_PP; // Alternate Push-Pull Output RX GPIO working mode: GPIO_Mode_IN_FLOATING; // Floating Input (4) UART parameter initialization mainly includes: baud rate setting (115200), 8 data bits, 1 stop bit, no parity bit, no hardware flow control, transmit/receive mode. (5) Enable interrupts and initialize NVIC (this step is only needed if interrupts are to be enabled) (6) Enable UART (7) Write interrupt handler function
-
Question 3: Main features of USART? (1) Full-duplex operation (independent reception and transmission of data); (2) In synchronous operation, can be master clock synchronized or slave clock synchronized; (3) Independent high-precision baud rate generator that does not occupy timer/counter; (4) Supports 5, 6, 7, 8, and 9 data bits, with 1 or 2 stop bits for serial data frame structure; (5) Hardware-supported parity bit generation and checking; (6) Data overflow detection; (7) Frame error detection; (8) Includes noise filter and digital low-pass filter for detecting erroneous start bits; (9) Three completely independent interrupts: TX transmission complete, TX data register empty, RX reception complete; (10) Supports multi-master communication mode; (11) Supports double-speed asynchronous communication mode.
Answer:
Refer to: STM32 Development – Detailed UARTApplication scenarios: GPS, Bluetooth, 4G modules
5. I2C
-
Question 1: What are the three types of signals during data transmission on the I2C bus? (1) Start signal: When SCL is high, SDA transitions from high to low to start data transmission. (2) Stop signal: When SCL is high, SDA transitions from low to high to end data transmission. (3) Acknowledge signal: The receiving IC sends a specific low pulse to the sending IC after receiving 8 bits of data, indicating that the data has been received. After the CPU sends a signal to the controlled unit, it waits for the controlled unit to send an acknowledgment signal. After receiving the acknowledgment, the CPU decides whether to continue transmitting based on the actual situation. If no acknowledgment is received, it is judged that the controlled unit has failed.
-
Question 2: How to configure the I2C master mode port? Hardware mode: Alternate Open-Drain Output, neither pull-up nor pull-down. (Fast mode: 400 Kbit/s) Software simulation: Push-Pull Output, configure pull-up resistor.
-
Question 3: I2C arbitration mechanism?Refer to: S5PV210 Development – How Much Do You Know About I2C? (3)The I2C arbitration mechanism is straightforward once you understand the wired-AND concept. In simple terms, it follows the principle of “low level priority,” meaning whoever sends the low level first gains control of the bus.
Answer:
Refer to: STM32 Development – PMIC, I2C Detailed ExplanationHardware mode: Communication speed settings are /* STM32 I2C Fast Mode */#define I2C_Speed 400000
/* Communication speed */I2C_InitStructure.I2C_ClockSpeed = I2C_Speed;
Software simulation: If no communication speed is set, how to calculate it?Through the I2C bus bit delay function i2c_Delay:
static void i2c_Delay(void)
{
uint8_t i;
/*
The following times are obtained through the Anfu Lai AX-Pro logic analyzer.
When the CPU main frequency is 72MHz, running in internal Flash, MDK project not optimized
Loop count of 10 gives SCL frequency = 205KHz
Loop count of 7 gives SCL frequency = 347KHz, SCL high time 1.5us, SCL low time 2.87us
Loop count of 5 gives SCL frequency = 421KHz, SCL high time 1.25us, SCL low time 2.375us
IAR project has high compilation efficiency and cannot be set to 7
*/
for (i = 0; i < 10; i++);
}
Application scenarios: PMIC, accelerometers, gyroscopes
6. SPI
-
Question 1: How many lines does SPI need? The SPI interface generally uses 4 lines for communication: MISO master device data input, slave device data output. MOSI master device data output, slave device data input. SCLK clock signal generated by the master device. CS chip select signal controlled by the master device.
-
Question 2: What are the four modes of SPI communication? SPI has four working modes, differing in SCLK, specifically defined by CPOL and CPHA. (1) CPOL: (Clock Polarity), clock polarity: SPI’s CPOL indicates whether the idle state of SCLK is low (0) or high (1): CPOL=0 means the idle state is low, so when SCLK is active, it is high (active-high); CPOL=1 means the idle state is high, so when SCLK is active, it is low (active-low);
(2) CPHA:(Clock Phase), clock phase: Phase corresponds to the edge at which data is sampled (first edge or second edge), 0 corresponds to the first edge, 1 corresponds to the second edge. For: CPHA=0, the first edge is: for CPOL=0, the idle state is low, the first edge is from low to high, so it is the rising edge; for CPOL=1, the idle state is high, the first edge is from high to low, so it is the falling edge; CPHA=1 indicates the second edge: for CPOL=0, the idle state is low, the second edge is from high to low, so it is the falling edge; for CPOL=1, the idle state is high, the first edge is from low to high, so it is the rising edge;
-
Question 3: How to determine which mode to use? (1) First, confirm the SCLK polarity required by the slave; whether it is low or high when not working, which determines CPOL as 0 or 1. From the schematic, we set the idle state of the serial synchronous clock to high, so we choose SPI_CPOL_High, which means CPOL is 1 (2) Then confirm from the slave chip datasheet’s timing diagram whether the slave chip samples data on the falling edge or the rising edge of SCLK. To translate: W25Q32JV accesses via SPI-compatible bus, including four signals: serial clock (CLK), chip select (/CS), serial data input (DI), and serial data output (DO). Standard SPI commands use the DI input pin to serially send commands, addresses, or data on the rising edge of CLK. The DO output pin is used to read data or status from the device on the falling edge of CLK. Supports SPI bus operations in mode 0 (0,0) and 3 (1,1). Modes 0 and 3 focus on the normal state of the CLK signal when the SPI bus master is idle and no data is being transmitted to the serial Flash. For mode 0, the CLK signal is typically low on the falling and rising edges / CS. For mode 3, the CLK signal is typically high on the falling and rising edges of /CS. Since the idle state of the serial synchronous clock is high, we choose the second edge, so we select SPI_CPHA_2Edge, which means CPHA is 1, thus we choose mode 3 (1,1).
Answer:
Refer to: STM32 Development – W25Q32JV SPI Flash Detailed ExplanationRefer to: Detailed Explanation of CPOL and CPHA in SPIApplication scenarios: SPI Flash, W25Q32 memory capacity 32Mb (4M x 8), which is 4M byte
7. CAN
-
Question 1: Summarize CAN? The CAN controller determines the bus level based on the potential difference between CAN_L and CAN_H. The bus level is divided into dominant level and recessive level, with one being dominant. The sender sends a message to the receiver by changing the bus level.
-
Question 2: Steps for CAN initialization configuration? (1) Configure the multiplexing function of relevant pins, enable the CAN clock (2) Set the CAN working mode and baud rate, etc. (CAN initialization loopback mode, baud rate 500Kbps) (3) Set the filter
-
Question 3: CAN data sending format? CanTxMsg TxMessage; TxMessage.StdId=0x12; // Standard Identifier TxMessage.ExtId=0x12; // Set Extended Identifier TxMessage.IDE=CAN_Id_Standard; // Standard Frame TxMessage.RTR=CAN_RTR_Data; // Data Frame TxMessage.DLC=len; // Length of data to be sent, send 8 bytes for(i=0;i<len;i++) TxMessage.Data[i]=msg[i]; // Data
Answer:
Refer to: STM32 Development – Detailed CAN Bus
8. DMA
-
Question 1: Introduction to DMA? Direct Memory Access (DMA) is used to provide high-speed data transfer between peripherals and memory or between memory and memory. Data can be moved quickly via DMA without CPU intervention, saving CPU resources for other operations.
-
Question 2: How many DMA transfer modes are there? DMA_Mode_Circular Circular Mode DMA_Mode_Normal Normal Buffer Mode Application scenarios: GPS, Bluetooth, both use circular sampling, DMA_Mode_Circular mode.
Answer:
Refer to: STM32 Development – Detailed DMA
A relatively important function, to obtain the current remaining data size, based on the set receive buffer size minus the current remaining data size, to get the current received data size.
9. Interrupts
-
Question 1: Describe the interrupt handling process? (1) Initialize the interrupt, set the trigger method to rising edge/falling edge/both edge triggers. (2) Trigger the interrupt, enter the interrupt service function
-
Question 2: How many external interrupts does the STM32 interrupt controller support?The STM32 interrupt controller supports 19 external interrupts/event requests: From the diagram, GPIO pins GPIOx.0~GPIOx.15 (x=A, B, C, D, E, F, G) correspond to interrupt lines 0 ~ 15. The other four EXTI lines are connected as follows: ● EXTI line 16 connected to PVD output ● EXTI line 17 connected to RTC alarm event ● EXTI line 18 connected to USB wakeup event ● EXTI line 19 connected to Ethernet wakeup event (only applicable to interconnect products) Interrupt service function list: IO external interrupt only allocates 7 interrupt vectors in the interrupt vector table, meaning only 7 interrupt service functions can be used. EXTI0_IRQHandler EXTI1_IRQHandler EXTI2_IRQHandler EXTI3_IRQHandler EXTI4_IRQHandler EXTI9_5_IRQHandler EXTI15_10_IRQHandler
Answer:
Refer to: STM32 Development – Detailed External Interrupt
10. How many clock sources does STM32 have? STM32 has 5 clock sources: HSI, HSE, LSI, LSE, PLL. ① HSI is a high-speed internal clock, RC oscillator, frequency 8MHz, low accuracy. ② HSE is a high-speed external clock, can connect quartz/ceramic resonator, or connect external clock source, frequency range 4MHz~16MHz. ③ LSI is a low-speed internal clock, RC oscillator, frequency 40kHz, provides low-power clock. ④ LSE is a low-speed external clock, connects a quartz crystal with a frequency of 32.768kHz. ⑤ PLL is a phase-locked loop frequency multiplication output, its clock input source can be selected as HSI/2, HSE or HSE/2. The multiplication factor can be selected from 2 to 16, but its output frequency must not exceed 72MHz.
Answer:
Refer to: STM32 Development – Detailed Clock System
11. How to write tasks in RTOS? How to switch out this task?
Answer:
A task, also known as athread. UCOS has a task scheduling mechanism, which schedules based on task priority.One is hardware interrupt, so the system will push the current task-related variables onto the stack, then execute the interrupt service routine, and return after execution. The other is switching between tasks, using task scheduling, each task has its own stack, the same way of pushing onto the stack, then executing another program, and then popping out to return.
Not every task executes in turn by priority order, but high-priority tasks monopolize execution; unless they actively give up execution, low-priority tasks cannot preempt them. At the same time, high-priority tasks can reclaim CPU occupancy rights given to low-priority tasks. Therefore,UCOS tasks need to be careful to insert wait delays to allow UCOS to switch out to let low-priority tasks execute.
12. What are the communication methods between tasks in UCOSII?
Answer:
In UCOSII, signals, mailboxes (message mailboxes), and message queues are used as intermediate links called events to achieve communication between tasks, as well as global variables.Semaphore:Refer to: Summary of UCOSII Semaphore Usage (Example Explanation) Semaphores are used for: 1. Controlling access to shared resources (satisfying mutual exclusion conditions) 2. Indicating the occurrence of a certain time 3. Synchronizing the behavior of two tasks
Application Example: The mutex semaphore serves as a mutual exclusion condition, initialized to 1. The goal is to call the serial port to send commands and must wait for the return of the “OK” character before sending the next command. Each task may use this sending function, and conflicts must not occur!
Mailbox (message mailbox):
Message Queue: Concept: (1) The message queue is essentially an array of mailboxes. (2) Both tasks and interrupts can place a message into the queue, and tasks can retrieve messages from the message queue. (3) The first message to enter the queue is the first to be sent to the task (FIFO). (4) Each message queue has a waiting list of tasks waiting for messages; if there are no messages in the queue, the waiting tasks will be suspended until a message arrives.
Application scenario: Receive buffer in serial port receiving program. Store external events.
13. The project uses a custom protocol, what is the structure?
Answer:
Familiar with the Modbus protocol. The structure is: Frame Header (SDTC) + Frame Length + Command + Serial Number + Data + CRC Check.
14. Differences between uCOSII and Linux?
Answer:
μC/OS-II is specifically designed for embedded applications in computers, μC/OS-II has high execution efficiency, small footprint, excellent real-time performance, and strong scalability, the minimum kernel can be compiled to 2KB. μC/OS-II has been ported to almost all well-known CPUs. Linux is free, safe, stable, and widely used in embedded systems, servers, and home machines. Both μC/OS-II and Linux are suitable for embedded use. However, μC/OS-II is designed specifically for embedded systems, resulting in higher running efficiency and less resource consumption. Linux can be used on servers and has a high usage rate. Although Linux was not specifically developed for servers, its source code is open and can be modified, making the differences between the two not significant. The main distribution, Red Hat Linux, is widely used on servers.
15. Git Commit Process
Question: What is the process for committing code in Git?
Answer:
1. Display modified files in the working path:
$ git status
2. Enter the directory of the modified file:
$ cd -
3. Display differences with the last committed version:
$ git diff
4. Add all current modifications to the next commit:
$ git add .
5. Add relevant function descriptions (use this for the first commit)
$ git commit -s
Also note: Function: Modify code functionality Ticket: Corresponding Bug number Note: Each folder must be submitted again. 6. Check committed code
$ git log
7. Do not modify already published commit records! (Use this for future commits)
$ git commit --amend
In command mode: 😡 (write to file and exit) 8. Push to server
$ git push origin HEAD:refs/for/master
16. Comparison of uCOSII, uCOSIII, and FreeRTOS
-
Question 1: Comparison of the three?
Answer:
Comparison of uCOSII and FreeRTOS: (1) FreeRTOS supports only TCP/IP, while uCOSII has extensive support for FS, USB, GUI, CAN, etc. (We need to use CAN for the tbox, so we choose uCOSII) (2) FreeRTOS is free for commercial use. uCOSII requires payment for commercial use. (3) FreeRTOS supports only queues, semaphores, and mutexes for inter-task communication. In addition to these, uCOSII also supports event flag groups and mailboxes. (4) Theoretically, FreeRTOS can manage more than 64 tasks, while uCOSII can manage only 64.
Comparison of uCOSII and uCOSIII: The changes from μC/OS-II to μC/OS-III are significant. One is that originally there were only 0~63 priority levels, and priority levels could not be repeated. Now it allows several tasks to use the same priority level, and within the same priority, time-slice scheduling is supported; the second is that it allows users to dynamically configure real-time operating system kernel resources during program execution, such as tasks, task stacks, semaphores, event flag groups, message queues, message counts, mutex semaphores, storage block divisions, and timers, which can be changed during program execution. This allows users to avoid resource allocation issues during program compilation. Improvements have also been made in resource reuse. In μC/OS-II, the maximum number of tasks was 64, but after version 2.82, it became 256. In μC/OS-III, users can have any number of tasks, semaphores, mutex semaphores, event flags, message lists, timers, and any allocated storage block capacity, limited only by the amount of RAM the user’s CPU can use. This is also a significant expansion. (Question: Teacher Shao, is this number fixed at startup, or can it be defined freely after startup?) It can be defined freely during configuration, as long as your RAM is large enough. The fourth point is that many functions have been added, and the number of functions is always increasing; everyone can take a look. Originally, these functions were not available in μC/OS-II.
17. Low Power Modes
-
Question 1: How many types of low power modes are there? What are the wake-up methods?
Answer:
18. Architecture of the Internet of Things
-
Question 1: How many layers is the architecture of the Internet of Things divided into? What functions does each layer perform?
Answer:
Divided into three layers, the architecture of the Internet of Things can be divided into the perception layer, network layer, and application layer. (1)Perception Layer: Responsible for information collection and information transmission between things. The technologies for information collection include sensors, barcodes and QR codes, RFID technology, audio and video, and other multimedia information. Information transmission includes near and far data transmission technologies, self-organizing network technologies, collaborative information processing technologies, and middleware technologies for information collection. The perception layer is the core capability for achieving comprehensive perception in the Internet of Things, and it is the part that urgently needs breakthroughs in key technologies, standardization, and industrialization, focusing on achieving more precise and comprehensive perception capabilities while solving low power consumption, miniaturization, and low-cost issues. (2)Network Layer: Utilizes wireless and wired networks to encode, authenticate, and transmit collected data. The widely covered mobile communication network is the infrastructure for realizing the Internet of Things and is the most standardized, industrially capable, and mature part of the three layers of the Internet of Things, focusing on optimizing and improving the characteristics of IoT applications to form a collaborative perception network. (3)Application Layer: Provides rich IoT-based applications, which is the fundamental goal of IoT development, combining IoT technology with industry information needs to implement a wide range of intelligent application solutions, focusing on industry integration, development and utilization of information resources, low-cost high-quality solutions, information security guarantees, and effective business model development.
19. Memory Management
-
Question 1: What methods of memory management are there in UCOS?
Answer:
The system manages memory partitions through memory control blocks associated with the memory partitions.
Dynamic memory management functions include: create dynamic memory partition function OSMemCreate(); request memory block function OSMemGet(); release memory block function OSMemPut();
20. What are the states of tasks in UCOS? Draw a diagram showing the relationship between task states?
Answer:
There are five states: sleep state, ready state, running state, waiting state (waiting for a certain event to occur), and interrupt service state.
The five state transition relationships of UCOSII tasks:
21. ADC
-
Question 1: Briefly describe the functional characteristics of the STM32 ADC system? (1) 12-bit resolution (2) Automatic calibration (3) Programmable data alignment (conversion results support left or right alignment stored in 16-bit data registers) (4) Single and continuous conversion modes.
Answer:
Refer to: STM32 Development – Detailed ADC
22. System Clock
-
Question 1: Briefly describe the basic process of setting the system clock? (1) Turn on HSE, wait for it to be ready, then set Flash wait operation. (2) Set AHB, APB1, APB2 division coefficients to determine their relationship with the system clock. (3) Set the CFGR register to determine the clock source and multiplication factor for PLL (HSE external 8M * 9 times = 72MHz). (4) Enable PLL, switch the system clock source to PLL.
Answer:
23. Handling of HardFault_Handler
-
Question 1: What are the causes? (1) Array out-of-bounds operation; (2) Memory overflow, out-of-bounds access; (3) Stack overflow, program runaway; (4) Interrupt handling errors;
-
Question 2: What are the handling methods? (1) Find the address remapping of HardFault_Handler in startup_stm32f10x_cl.s, and rewrite it to jump to the HardFaultHandle function. (2) Print and check registers R0, R1, R2, R3, R12, LR, PC, PSR. (3) Check the Fault status register group (SCB->CFSR and SCB->HFSR)
Answer:
Refer to: STM32 Development – Handling of HardFault_Handler Refer to: Cortex-M3 and Cortex-M4 Fault Exception Applications – Basic Knowledge
24. TTS Speech Synthesis Method
-
Question 1: What method does sim7600 TTS speech use?
Answer:
(1) Use unicode encoding to synthesize sound AT+CTTS=1,”6B228FCE4F7F75288BED97F3540862107CFB7EDF” The content is “Welcome to the Voice Synthesis System”; the module sends and receives Chinese text messages using unicode encoding, so it is easy to read the messages aloud; (2) Directly input text, ordinary characters use ASCII code, Chinese characters use GBK encoding. AT+CTTS=2,”Welcome to the Voice Synthesis System”
25. Timer
-
Question 1: Given that the STM32 system clock is 72MHz, how to set the relevant registers to achieve a 20ms timer?
Answer:
Refer to: STM32 Development – Systick Timer By using SysTick_Config(SystemCoreClock / OS_TICKS_PER_SEC))//1ms timer
Where:
uint32_t SystemCoreClock = SYSCLK_FREQ_72MHz; /*!< System Clock Frequency (Core Clock) */
#define SYSCLK_FREQ_72MHz 72000000
#define OS_TICKS_PER_SEC 1000 /* Set the number of ticks in one second
If you need 20ms, you can set a global variable and initialize it to 20, so that with each SysTick interrupt, this global variable is decremented by 1, and when it reaches 0, it means the SysTick interrupt has occurred 20 times, resulting in a time of: 1ms * 20 = 20ms, thus achieving a 20ms timer.
26. Priority
-
Question 1: How to run two tasks with the same priority?
Answer:
Temporarily elevate the priority of the task acquiring the semaphore to one level higher than the highest priority of all tasks during the use of shared resources, so that the task is not interrupted by other tasks, allowing it to quickly use and release the shared resource, and then restore its original priority after releasing the semaphore.
27. State Machine
-
Question 1: What state machine is used?
Answer:
Refer to: STM32 Development – State Machine and State Switching Logic
Finite State Machine (FSM) is also known as a finite state automaton, abbreviated as state machine.Refer to: Detailed Explanation and Implementation of Finite State Machine FSM
Assuming the state transitions of the state machine are shown in the table below:
Implementation: (using switch statements)
// Written horizontally
void event0func(void)
{
switch(cur_state)
{
case State0:
action0;
cur_state = State1;
break;
case State1:
action1;
cur_state = State2;
break;
case State2:
action1;
cur_state = State0;
break;
default:break;
}
}
void event1func(void)
{
switch(cur_state)
{
case State0:
action4;
cur_state = State1;
break;
default:break;
}
}
void event2func(void)
{
switch(cur_state)
{
case State0:
action5;
cur_state = State2;
break;
case State1:
action6;
cur_state = State0;
break;
default:break;
}
}
28. Device Selection
-
Question 1: Comparison of STM32F407 VS STM32F103 main functions and resources?
Answer:
Refer to: Comparison of STM32F407 VS STM32F103 main functions and resources
Original text source: https://juyou.blog.csdn.net/article/details/116021595
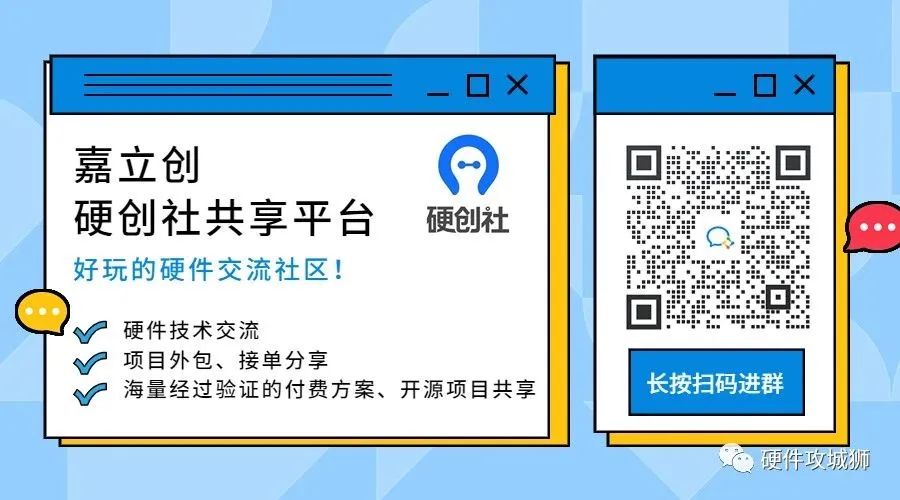