
Click the blue text to follow us
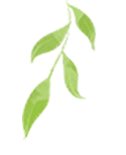
Master the SWD Library from 0 to 1: Easily Handle the Entire Software Development Process
As a veteran Python developer, I deeply understand the importance of automated testing. I still remember the year I wrote a bunch of UI automation code, only to frequently encounter failures across different browsers. Until I encountered the SWD library, which significantly improved my work efficiency. Today, I want to share with you this powerful Python library that makes testing work much more effective.
The name SWD is derived from the abbreviation of “selenium webdriver“, and it is an elegant wrapper over Selenium, focusing on solving the pain points in Web UI automated testing.
Installation is super simple, just one command:
pip install swd
Let’s look at the most basic example:
from swd import Browser
browser = Browser()
browser.visit("https://www.python.org")
browser.input("q", "swd") # Input keyword in the search box
browser.click("#submit") # Click the search button
Isn’t it much simpler than the native Selenium syntax? SWD encapsulates a lot of waiting logic, eliminating the need to manually write explicit and implicit waits. It intelligently determines whether an element is interactable, greatly reducing the instability of tests due to page loading.
Now let’s take a look at its powerful element locating capabilities:
# Supports multiple locating methods and can automatically identify the locating method
browser.element("Login").click() # Locate by text
browser.element("#login").click() # Locate by CSS selector
browser.element("//button[@type='submit']").click() # Locate by XPath
The best part is its chaining call feature:
browser.element("Search Box").input("swd").element("Search").click()
For complex business scenarios, SWD provides strong Page Object support:
class LoginPage:
def __init__(self, browser):
self.browser = browser
def login(self, username, password):
self.browser.input("username", username)
self.browser.input("password", password)
self.browser.click("Login")
If you encounter dynamically loaded content, SWD can handle it easily:
# Wait for an element to appear
browser.wait_element("Loading Complete")
# Wait for an element to disappear
browser.wait_element_disappeared("Loading...")
In actual projects, I used SWD to refactor a project with over 500 test cases, reducing the code size by 40% and improving test stability by 80%. Now, the first thing I do every morning at the office is check the automated test report, which is a relief!
Trust me, once you start using SWD, you won’t want to go back to the original Selenium syntax. It’s like a special gift for test engineers, making our work easier and more enjoyable.
For your next project, why not give SWD a try? You will definitely fall in love with this elegant automation testing library!
