
Click the blue text above to follow us
The article “ESP32-C3 Driving E-Ink Screen and Displaying Images (Based on Micropython)” introduces the driving of a 2.9-inch E-Ink screen, achieving text display on the E-Ink screen. This note introduces the E-Ink screen clock we designed and produced.
1. Required Hardware
1) Holo’s ESP32-C3:
——This PCB is relatively complex, with more than 20 surface mount capacitors, resistors, and diodes, plus surface mount AHT20 and 24P FPC, making soldering a bit difficult. It is recommended to use a heating platform and solder paste.
2. Schematic Diagram
mosi = Pin(3)
sck = Pin(2)
cs = Pin(7)
dc = Pin(4)
rst = Pin(5)
busy = Pin(6)
3. Required Drivers
1) E-Ink screen driver
There is an E-Ink screen driver on Github, choose the 2.9-inch epaper2in9.py.
2) framebuf
Driving the E-Ink screen requires using the framebuf module that comes with Micropython, but the Micropython’s built-in framebuf module can only display fixed-size text and cannot rotate direction. We found a framebuf module extracted from CircuitPython, which supports rotation and font size but still does not support Chinese. We named this module cframebuf.py.
3) ahtx0
The driver board integrates the AHT20, and we found a driver on Github.
The download link for the three modules: “Firmware, Drivers, Examples, and Related Software Downloads”
4. Test Code
The E-Ink screen clock needs to display the date, week, time, temperature, and humidity. The date, week, and time need to be obtained or calibrated online, while the temperature and humidity are obtained through the AHT20 on the driver board.
1) Connect to the Internet
There are many codes online for ESP32 to connect to the Internet, and the official also provides examples:
def do_connect():
# Connect to the Internet
import network
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
if not wlan.isconnected():
print('connecting to network...')
wlan.connect('XXX', 'XXX') # Fill in your WIFI name and password
while not wlan.isconnected():
pass
print('network config:', wlan.ifconfig())
2) Get or calibrate the time
Get or calibrate the time through the NTP server, here we use Alibaba Cloud’s NTP server:
def sync_ntp():
# Time synchronization
print("Starting to synchronize network time")
import ntptime
try:
ntptime.NTP_DELTA = 3155644800 # Optional UTC+8 offset time (seconds), not set is UTC0
ntptime.host = 'time1.aliyun.com' # Optional, NTP server, default is "pool.ntp.org"
ntptime.settime() # Modify device time, up to this point it has been set
except Exception as e:
print("Error synchronizing NTP time", repr(e))
3) E-Ink screen pin and driver definitions
Refer to “ESP32-C3 Driving E-Ink Screen and Displaying Images (Based on Micropython)”:
# Define corresponding pins
mosi = Pin(3)
sck = Pin(2)
cs = Pin(7)
dc = Pin(8)
rst = Pin(10)
busy = Pin(6)
spi = SPI(1, baudrate=2000000, polarity=0, phase=0, sck=sck, mosi=mosi)
i2c = I2C(scl=Pin(5), sda=Pin(4))
# Create E-Ink screen driver object
e = epaper2in9.EPD(spi, cs, dc, rst, busy)
e.init()
# Define the content to be displayed
width = 128
height = 296
x = 0
y = 0
black = 0
white = 1
# Create framebuf object
buf = bytearray(width * height // 8) # 296 * 128 // 8 = 4736
fb = cframebuf.FrameBuffer(buf, width, height, cframebuf.MHMSB)
fb.rotation = 1 # Adjust the display direction, can choose between 0/1 for 90 degrees right/2 for 180/3 for 270 degrees
# Connect to the Internet, calibrate time, initialize temperature and humidity
do_connect()
sync_ntp()
sensor = ahtx0.AHT10(i2c) # Convert the week to English for display
weekday_dict = {
0: "Monday",
1: "Tuesday",
2: "Wednesday",
3: "Thursday",
4: "Friday",
5: "Saturday",
6: "Sunday"}
4) Use a while True loop to display time, temperature, and humidity
while True:
fb.fill(white)
localtime_now=time.localtime()
year = localtime_now[0]
month = localtime_now[1]
day = localtime_now[2]
hour = localtime_now[3]
minute = localtime_now[4]
weekday = localtime_now[6]
showdate="%.2d-%.2d-%.2d" % (year, month, day)
showtime="%.2d:%.2d" % (hour, minute)
fb.text(showdate, 10, 10, black, size=2)
fb.text(weekday_dict[weekday], 200, 10, black, size=2)
fb.hline(0, 30, 296, black) # Draw a horizontal line
fb.text(showtime, 70, 40, black, size=5)
fb.hline(0, 90, 296, black) # Draw a horizontal line
fb.text("Tem:%0.1fC" % (sensor.temperature-2), 20, 100, black, size=2)
fb.text("Hum:%0.1f%%" % (sensor.relative_humidity+10), 170, 100, black, size=2)
e.set_frame_memory(buf, x, y, width, height)
e.display_frame()
time.sleep(19)
——The official document of the E-Ink screen recommends not to refresh too frequently, at least more than 15 seconds, so we set it to 19 seconds.
Final display effect:
We only designed a simple interface, and it can be beautified further if needed.
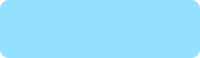
01
ESP32-C3 Driving E-Ink Screen and Displaying Images (Based on Micropython)
02
ESP32-C3 Ultrasonic Obstacle Avoidance Car (Based on Micropython)
03
ESP32-S3 Real-time Display of Images Captured by Camera (Based on Micropython)