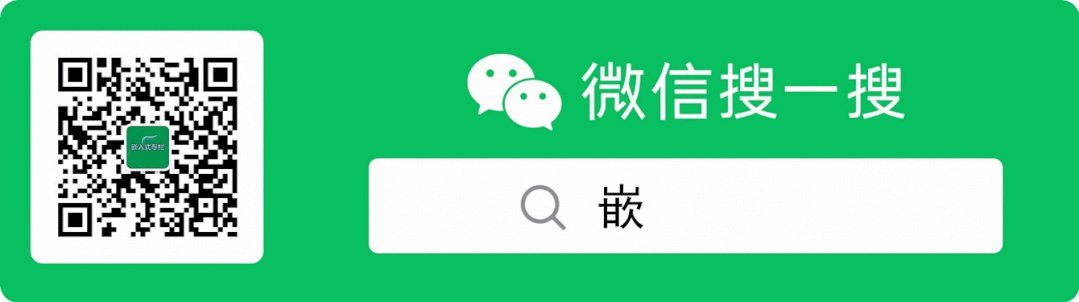
1. Preparation
-
Hardware: STM32 development board, Modbus adapter (such as RS485 converter), Dupont wires, etc.
-
Software: STM32CubeIDE or Keil MDK-ARM, Modbus library (such as libmodbus).
2. Configure STM32
-
Use STM32CubeMX or Keil’s configuration tool to configure the appropriate clock, GPIO, etc. for your STM32.
-
Make sure you have enabled UART communication.
3. Install Modbus Library
-
Download and install the libmodbus library. This is a popular open-source Modbus library that can be used for C and C++.
-
Copy the libmodbus library files into your STM32 project directory.
4. Configure Modbus Library
-
In your code, configure libmodbus to use the correct serial port and baud rate.
-
You may need to modify the source code of libmodbus to fit your hardware and requirements.
5. Implement Modbus Functionality
-
Implement Modbus functionality according to your needs, such as reading and writing coils, holding registers, etc.
-
Use the functions provided by the libmodbus library to communicate with Modbus devices.
6. Testing and Debugging
-
Run your program on the development board and use serial tools (such as PuTTY) or other Modbus tools for testing and debugging.
-
Check if the data is transmitted correctly and handle any communication errors.
7. Optimization and Improvement
-
Optimize the code as needed to improve communication efficiency.
-
Adjust Modbus functionality according to actual application requirements.
Notes
-
Modbus protocol has various variants (such as Modbus TCP, Modbus RTU, Modbus ASCII), and you need to choose the appropriate implementation based on your needs.
-
Ensure that you follow the relevant Modbus specifications and standards.
-
When communicating, pay attention to data verification, error handling, and retry mechanisms.
This tutorial is a high-level overview, and the specific implementation details will vary depending on your hardware and requirements. You may need to refer to more detailed documents and tutorials to gain a deeper understanding of each step.
Source Code Module
From an application perspective, to use Modbus, the interfaces that need to be called are actually just a few. Here, I will share some commonly used source code modules during the porting process.
/* Include necessary header files */ #include <modbus.h> #include "stm32xxxx.h" // Replace according to your STM32 model /* Module 1: Serial Communication Module */ // Used to initialize the serial port and configure serial communication parameters void serial_init(void) { // Use STM32's standard library functions to initialize USART, adjustments are needed based on your specific hardware configuration } /* Module 2: Modbus Configuration Module */ // Used to configure the libmodbus library and set Modbus parameters void modbus_config(void) { modbus_t *ctx; // Context pointer for the libmodbus library uint8_t *tab_slave_ids; // Pointer to the slave ID array int nb_slaves; // Number of available slaves // Create Modbus RTU communication context ctx = modbus_new_rtu("/dev/ttyUSB0", SERIAL_PORT_SPEED, 'N', 8, 1); if (ctx == NULL) { fprintf(stderr, "Unable to create the libmodbus context\n"); return; } // Set slave ID modbus_set_slave(ctx, MODBUS_SLAVE_ID); // Get the number of available slaves nb_slaves = modbus_get_slave_nb(ctx); if (nb_slaves <= 0) { fprintf(stderr, "No slaves available\n"); return; } // Get the slave ID array tab_slave_ids = modbus_get_slave_ids(ctx); if (tab_slave_ids == NULL) { fprintf(stderr, "Unable to get slaves ids\n"); return; } // More configurations can be added here, such as handling read/write requests, error handling, etc. } /* Module 3: Modbus Data Processing Module */ // Core logic for processing Modbus requests and responses, can be further expanded and customized based on needs void modbus_data_handler(modbus_t *ctx) { // Add code logic to handle Modbus requests and responses here } /* Main function */ int main(void) { /* Initialize serial communication */ serial_init(); // Initialize serial communication /* Configure libmodbus library */ modbus_config(); // Configure libmodbus library and set Modbus parameters /* Main loop, process Modbus requests and responses */ while (1) { modbus_data_handler(ctx); // Core logic for handling Modbus data requests and responses // More logic can be added here, such as error handling, sending heartbeat packets, etc. } return 0; }
———— END ————
● Column “Embedded Tools”
● Column “Embedded Development”
● Column “Keil Tutorial”
● Selected Tutorials from Embedded Column
Follow the public account and reply “Add Group” to join the technical exchange group according to the rules, reply “1024” to see more content.
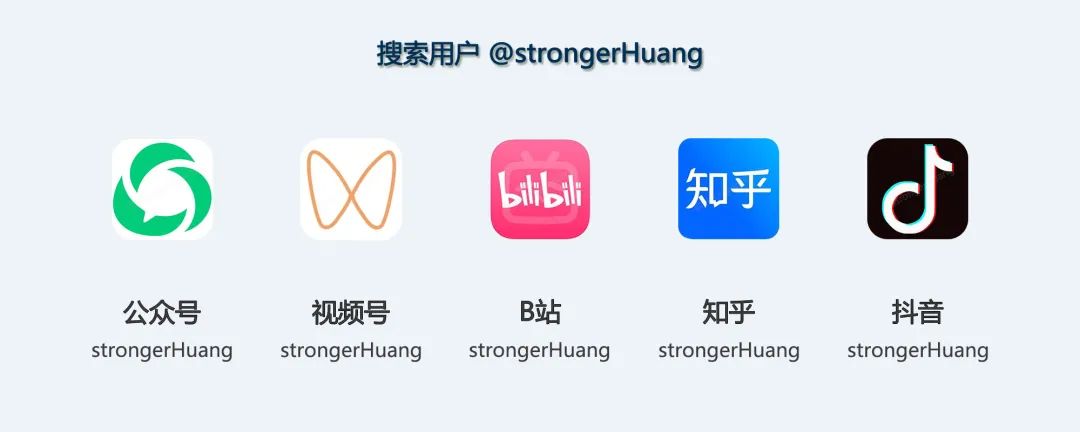
Click “Read the original text” to see more shares.