WeChat Official Account: Jiu Geek
Welcome to star and follow Jiu Geek, let’s discuss technology and architecture together!
Your likes, favorites, and comments are very important. If this article helps you, please share and support, thank you!
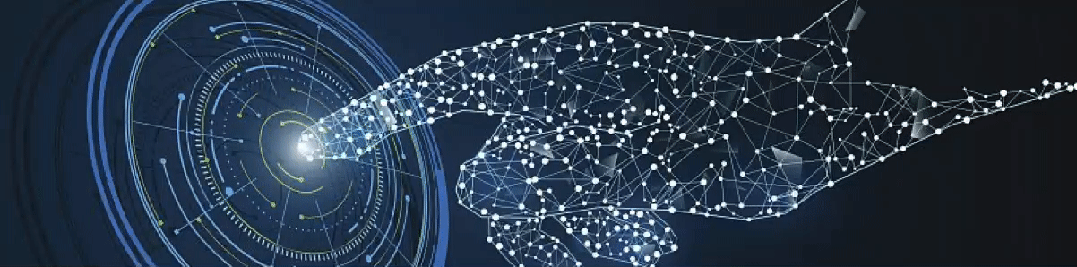
1. Lightweight: CoAP is a lightweight protocol designed to run on resource-constrained devices. Its message format is compact, reducing communication overhead, making it suitable for low-power devices and limited network bandwidth.
2. RESTful: CoAP adopts the design philosophy of REST (Representational State Transfer), making it easier to integrate with existing web architectures. Resources (such as sensors and actuators) are identified by URIs and accessed and manipulated via standard HTTP methods (GET, POST, PUT, DELETE).
3. Supports Multiple Transport Layers: CoAP can run over UDP and DTLS (Datagram Transport Layer Security), making it suitable for different network environments. UDP provides low latency and small communication overhead, while DTLS provides security guarantees for communication.
4. Low Energy Consumption: The CoAP protocol is designed to run on low-power devices, supporting quick sleep and wake-up. Its lightweight features and support for trigger-based communication help reduce device energy consumption.
5. Trigger-Based Communication: CoAP supports trigger-based communication, which allows devices to actively send notifications of trigger events. This method is very useful for real-time data transmission and event-driven application scenarios.
6. Reliable and Unreliable Communication: CoAP provides two message transmission modes: reliable (CON) and unreliable (NON). The reliable mode uses a retransmission mechanism to ensure reliable message delivery, while the unreliable mode is suitable for scenarios where real-time requirements are not high.
7. Simplified Proxies and Caching: CoAP supports proxies and caching, making it easier to deploy and manage in large-scale systems.

Step 1: Add Dependencies
pom.xml
):1<dependency>
2 <groupid>org.eclipse.californium</groupid>
3 <artifactid>californium-core</artifactid>
4 <version>2.6.0</version> <!-- Choose the version suitable for your project -->
5</dependency>

Step 2: Create CoAP Server Bean
1import org.eclipse.californium.core.CoapServer;
2import org.eclipse.californium.core.server.resources.CoapResource;
3import org.springframework.context.annotation.Bean;
4import org.springframework.context.annotation.Configuration;
5
6@Configuration
7public class CoapConfig {
8
9 @Bean
10 public CoapServer coapServer() {
11 CoapServer server = new CoapServer();
12 server.add(new CoapResource("example") {
13 @Override
14 public void handleGET(CoapExchange exchange) {
15 exchange.respond("Hello, CoAP!");
16 }
17 });
18 return server;
19 }
20}

Step 3: Create CoAP Client Bean
1import org.eclipse.californium.core.CoapClient;
2import org.springframework.context.annotation.Bean;
3import org.springframework.context.annotation.Configuration;
4
5@Configuration
6public class CoapClientConfig {
7
8 @Bean
9 public CoapClient coapClient() {
10 return new CoapClient("coap://localhost/example");
11 }
12}

Step 4: Start Spring Boot Application
@SpringBootApplication
), then run the application. The CoAP server will start when the application starts.1@SpringBootApplication
2public class MyCoapApplication {
3
4 public static void main(String[] args) {
5 SpringApplication.run(MyCoapApplication.class, args);
6 }
7}

Step 5: Test CoAP Server
coap://localhost/example
and expect to receive the response “Hello, CoAP!”. 1import org.eclipse.californium.core.CoapClient;
2import org.springframework.web.bind.annotation.GetMapping;
3import org.springframework.web.bind.annotation.RequestMapping;
4import org.springframework.web.bind.annotation.RestController;
5
6@RestController
7@RequestMapping("/smart-home")
8public class SmartHomeController {
9
10 private final CoapClient lightClient = new CoapClient("coap://light-device-uri");
11
12 @GetMapping("/turn-on-light")
13 public String turnOnLight() {
14 // Send CoAP request to turn on light
15 lightClient.post("on", 0).getResponseText();
16 return "Light turned on!";
17 }
18
19 @GetMapping("/turn-off-light")
20 public String turnOffLight() {
21 // Send CoAP request to turn off light
22 lightClient.post("off", 0).getResponseText();
23 return "Light turned off!";
24 }
25}
In this example, SmartHomeController
contains two simple endpoints for controlling the smart light’s on and off state via CoAP requests. This is just a simple example; in real scenarios, you may need more complex logic and device interactions.
WebClient: Unlocking New Skills for Web Interaction
MyBatis Plus Enum Type Handling: Mapping Database and Java Enums
Spring Boot Integration with PLC4X for S7 Communication
Upgrade Journey: Exploring Spring Boot RESTful Services from RestTemplate to WebClient
Integrating PLC4X in Spring Boot for OPC Communication