1. Image Overlay
The original purpose of the program was to process blurred images, meaning that there are two images with different degrees of blur, which can be synthesized into a clearer image through this program. However, after improvements, this program can achieve the fusion of any two images.
2. Design Idea:
First, unify the size of the two images and determine the number of layers in the Gaussian pyramid based on the image size; then determine the Gaussian kernel, use the Gaussian pyramid to compress the images and calculate the residuals using the Laplacian pyramid; compare the data points of the two images and select the clearer data points; finally, overlay them to obtain the combined image.
3. Functionality:
comsize: Unifies the size of the two images, taking the larger number of rows and columns, because subsequent processing using the pyramid will scale the image by a factor of 2, so the number of pyramid layers is determined based on the image size; myconv2: Performs convolution, a self-written program to implement the function of the library function conv2. When using conv2, the array size was not quite right, so I wrote myconv2; get_lap: Gaussian pyramid and Laplacian pyramid; fuzzy_image_process: Main program for processing blurred images.
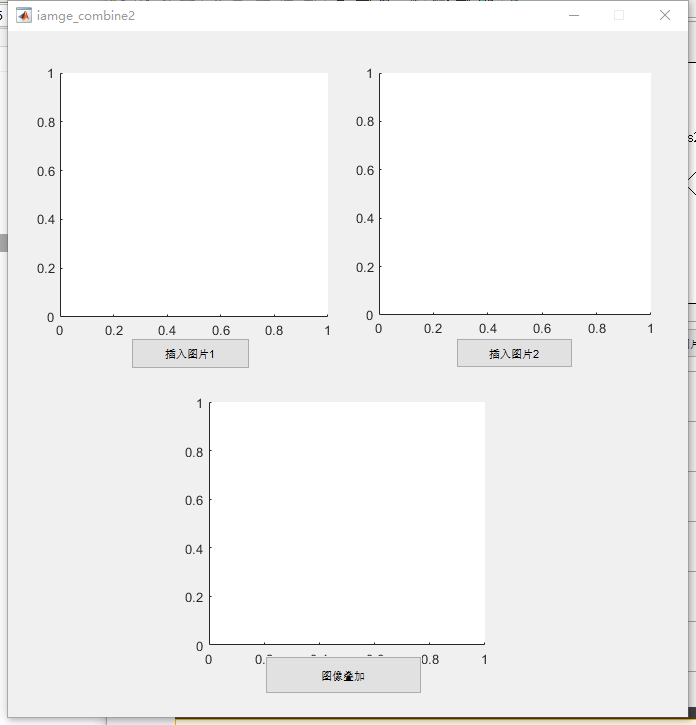
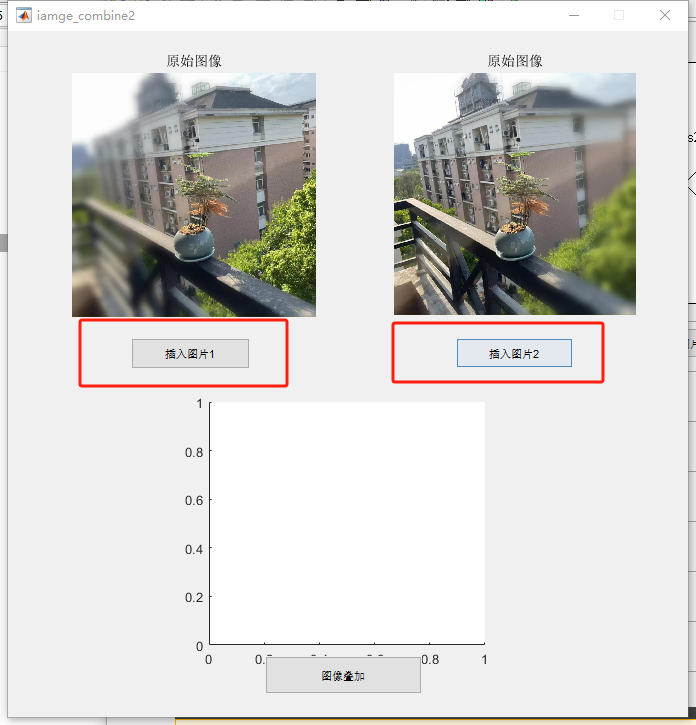
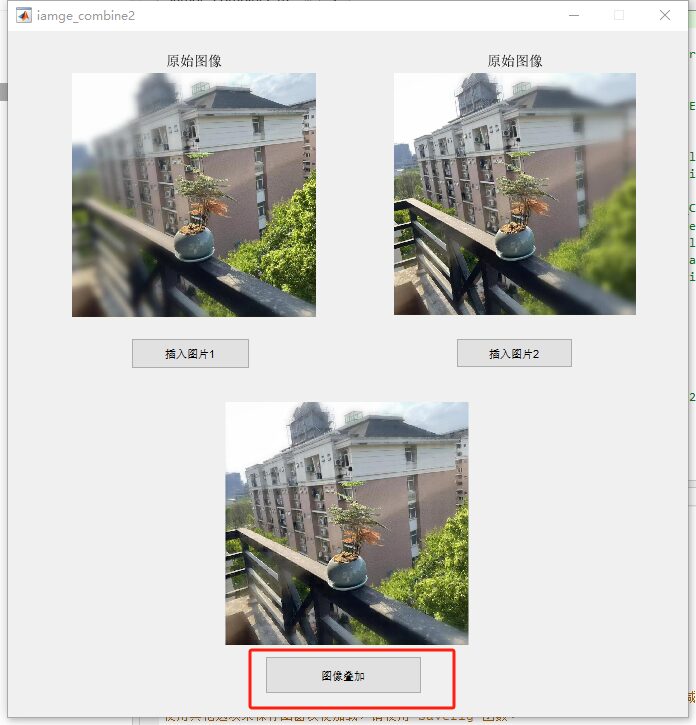
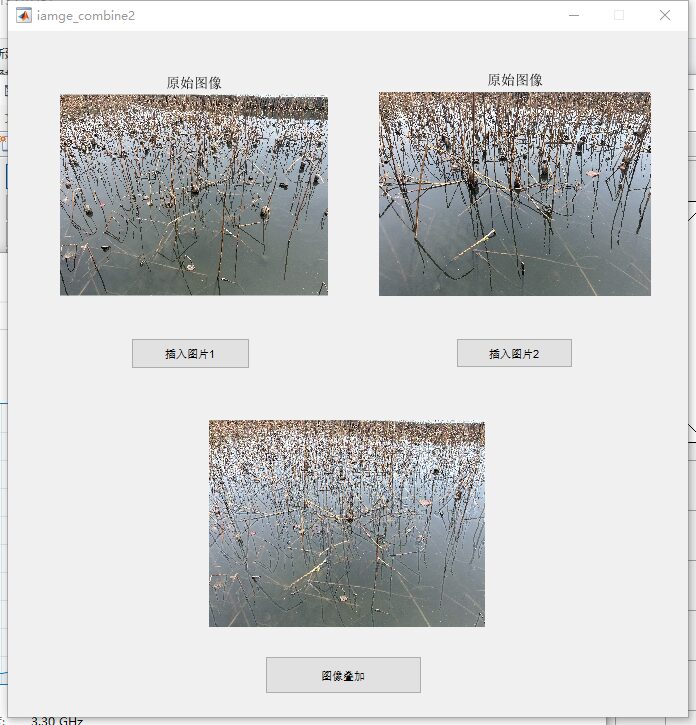
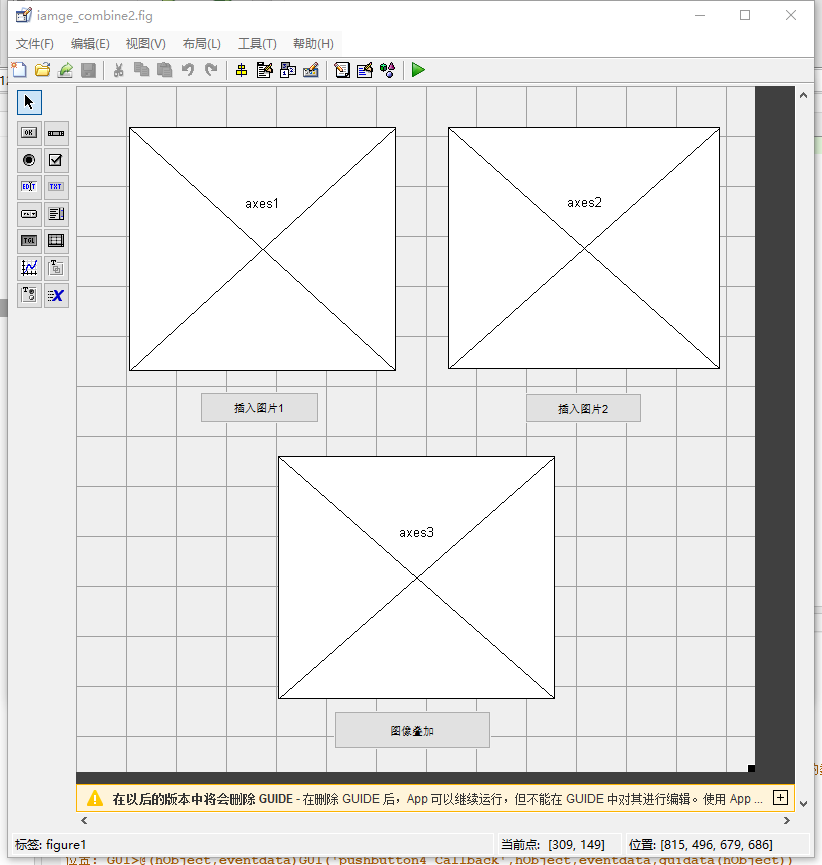
function varargout = iamge_combine2(varargin)% IAMGE_COMBINE2 MATLAB code for iamge_combine2.fig% Last Modified by GUIDE v2.5 10-May-2023 23:09:07% Begin initialization code - DO NOT EDITgui_Singleton = 1;gui_State = struct('gui_Name', mfilename, ... 'gui_Singleton', gui_Singleton, ... 'gui_OpeningFcn', @iamge_combine2_OpeningFcn, ... 'gui_OutputFcn', @iamge_combine2_OutputFcn, ... 'gui_LayoutFcn', [] , ... 'gui_Callback', []);if nargin && ischar(varargin{1}) gui_State.gui_Callback = str2func(varargin{1});endif nargout [varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});else gui_mainfcn(gui_State, varargin{:});end% End initialization code - DO NOT EDIT
function combine_image=fuzzy_image_process% Image Readingglobal image1;global image2;img1 = imread(image1);img2 = imread(image2);[cimg1,cimg2,level]=comsize(img1,img2);im1 = im2double(cimg1);im2 = im2double(cimg2);% Get Laplacian PyramidL1 = get_lap(im1,level);L2 = get_lap(im2,level);ksize = 5;sigma = 3;Image = cell(1,level);for k=1:level % Store the points with larger absolute values in Image, i.e., the clearer points a = abs(L1{k}) > abs(L2{k}); Image{k} = a.*L1{k} + ~a.*L2{k};endgauss = zeros(ksize, ksize);for i = 1 : ksize for j = 1 : ksize gauss(i, j) = exp(-((i - ceil(ksize/2))^2 + (j - ceil(ksize/2))^2)/(2 * sigma^2)); endendgauss = gauss / sum(gauss(:));%% Image integration, overlaying Image and Laplacian, i.e., overlaying clearer points and actual pointsfor i = level-1: -1 : 1 [rows, cols, ~] = size(Image{i+1}); upsampled = zeros(2*rows,2*cols,3); upsampled(2:2:end,1:2:end,1) = Image{i+1}(:,:,1); upsampled(2:2:end,1:2:end,2) = Image{i+1}(:,:,2); upsampled(2:2:end,1:2:end,3) = Image{i+1}(:,:,3); upsampled(:,:,1) = 4*myconv2(upsampled(:,:,1), gauss); upsampled(:,:,2) = 4*myconv2(upsampled(:,:,2), gauss); upsampled(:,:,3) = 4*myconv2(upsampled(:,:,3), gauss); Image{i} = Image{i} + upsampled; end combine_image=Image{1};
function [sz1,sz2,level]=comsize(origin1,origin2)[row1,colon1,layer1] = size (origin1);[row2,colon2,layer2] = size (origin2);if row1>row2 crow=row1;else crow=row2;endif colon1>colon2 ccolon=colon1;else ccolon=colon2;endsz1=imresize(origin1,[crow,ccolon]);sz2=imresize(origin2,[crow,ccolon]);n=1;while rem(crow,2^n)==0&&rem(ccolon,2^n)==0 n=n+1;endlevel=n-1;
function laplacian = get_lap(img,level)%% Gaussian Kernel Generationsigma = 3; % Standard deviation of Gaussian kernelksize = 5; % Kernel sizeGauss = zeros(ksize, ksize);for i = 1 : ksize for j = 1 : ksize Gauss(i, j) = exp(-((i - ceil(ksize/2))^2 + (j - ceil(ksize/2))^2)/(2 * sigma^2)); endendGauss = Gauss / sum(Gauss(:));%% Gaussian Pyramidpyramid = cell(1, level);pyramid{1} = img;for i = 2 : level % Reduce image [rows,cols,~] = size(pyramid{i-1}); pyramid{i} = pyramid{i-1}(1:2:rows,1:2:cols,:); % Smooth the image using Gaussian filtering pyramid{i}(:,:,1) = myconv2(pyramid{i}(:,:,1), Gauss); pyramid{i}(:,:,2) = myconv2(pyramid{i}(:,:,2), Gauss); pyramid{i}(:,:,3) = myconv2(pyramid{i}(:,:,3), Gauss);end%% laplacian stores the residuals, which are the missing data pointslaplacian = cell(1, level); laplacian{level} = pyramid{level}; % First upsample, then expand to suitable sizefor i = level-1: -1 : 1 [rows, cols, ~] = size(pyramid{i+1}); upsampled = zeros(2*rows,2*cols,3); upsampled(2:2:end,1:2:end,1) = pyramid{i+1}(:,:,1); upsampled(2:2:end,1:2:end,2) = pyramid{i+1}(:,:,2); upsampled(2:2:end,1:2:end,3) = pyramid{i+1}(:,:,3); upsampled(:,:,1) = 4*myconv2(upsampled(:,:,1), Gauss); upsampled(:,:,2) = 4*myconv2(upsampled(:,:,2), Gauss); upsampled(:,:,3) = 4*myconv2(upsampled(:,:,3), Gauss); laplacian{i} = pyramid{i} - upsampled; end
function output = myconv2(input, kernel) [m, n] = size(input); [k, l] = size(kernel); pad_input = padarray(input, [floor(k/2), floor(l/2)], 'replicate', 'both'); output = zeros(m, n); for i = 1 : m for j = 1 : n output(i, j) = sum(sum(pad_input(i:i+k-1, j:j+l-1) .* kernel)); end endend
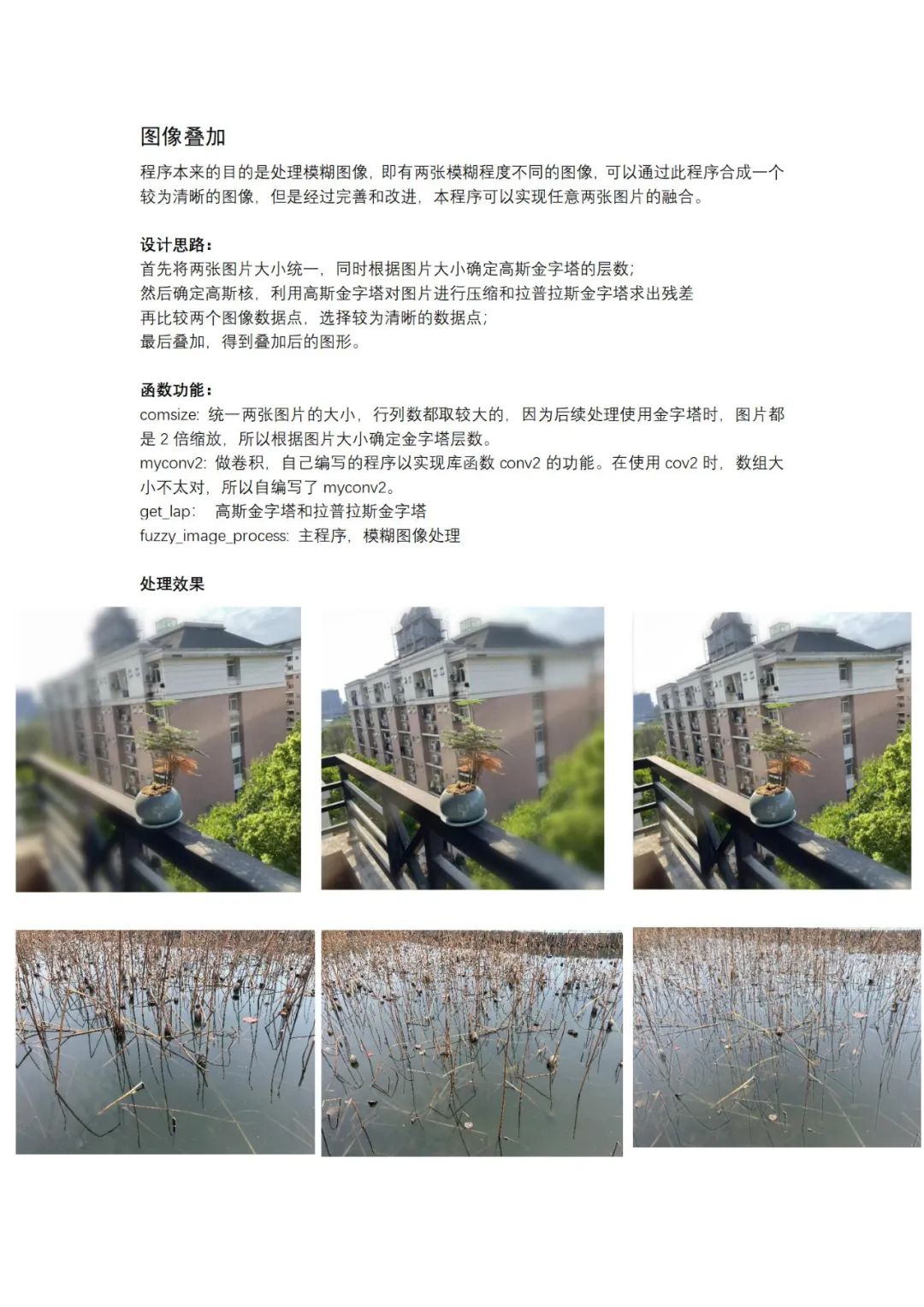
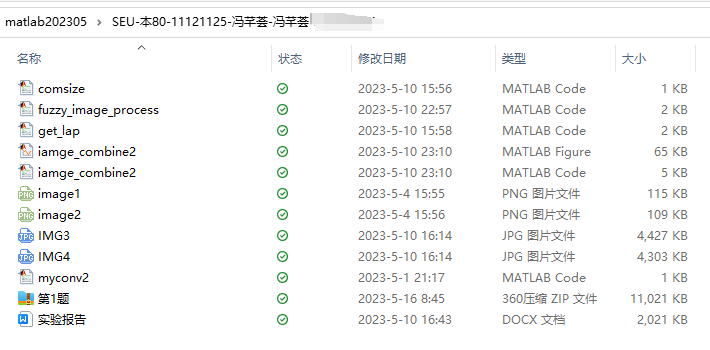
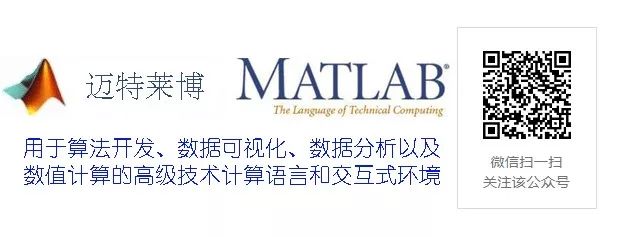