What is IoT (Internet of Things)?
Imagine your appliances thinking for themselves: the refrigerator knows when the milk is running low and orders a delivery; the soil in the flowerpot feels dry and automatically notifies the water pump to water it.
IoT (Internet of Things) is a technology that connects various devices through the Internet, enabling automation and intelligence.
Today, we will build a simple IoT application using Python – a temperature monitoring system.
This system will collect temperature data and send it to the cloud, allowing you to view it on any device.
To build an application that can read temperature data and upload it to the cloud for display.
We will use the following tools and libraries:
-
DHT11 Temperature and Humidity Sensor: to collect temperature data.
-
Raspberry Pi: as our hardware platform (you can use other compatible hardware).
-
Python: to write the program.
-
Flask and HTML: to display the temperature data.
-
Requests library: to send data to the cloud.
Step 1: Prepare Hardware and Install Libraries
DHT11 is an inexpensive temperature and humidity sensor connected to the GPIO pins of the Raspberry Pi. Assume the connections are as follows:
Install Necessary Python Libraries
We need to install two libraries:
-
Adafruit_DHT (to read sensor data)
-
Flask (to build the web application)
Run the following command to install:
pip install Adafruit_DHT flask
Step 2: Collect Temperature Data
Write a Python script to get temperature data from DHT11.
import Adafruit_DHT
# Define sensor type and GPIO pin
SENSOR = Adafruit_DHT.DHT11
PIN = 4
def get_temperature():
# Read data from the sensor
humidity, temperature = Adafruit_DHT.read_retry(SENSOR, PIN)
if humidity is not None and temperature is not None:
return temperature
else:
return "Error: Read failed"
When you run this code, if the DHT11 is connected properly, it will return the current temperature.
Step 3: Upload Data to the Cloud
We will use a hypothetical API to upload temperature data. Assume the API address of the cloud service is http://example.com/api/temperature.
import requests
def upload_temperature(temperature):
url = "http://example.com/api/temperature"
data = {"temperature": temperature}
try:
response = requests.post(url, json=data)
if response.status_code == 200:
print("Data uploaded successfully!")
else:
print("Upload failed:", response.status_code)
except Exception as e:
print("Request failed:", e)
Tip: You can use free IoT platforms (like Thingspeak or MQTT services) to test the upload functionality.
We will use Flask to create a simple web interface to display the current temperature in real-time.
from flask import Flask, jsonify
import threading
import time
app = Flask(__name__)
temperature_data = {"temperature": None}
@app.route('/')
def index():
return f"Current Temperature: {temperature_data['temperature']}°C"
@app.route('/temperature', methods=['GET'])
def get_temperature_api():
return jsonify(temperature_data)
# Background thread: collect data every 5 seconds
def update_temperature():
global temperature_data
while True:
temperature = get_temperature()
temperature_data["temperature"] = temperature
upload_temperature(temperature)
time.sleep(5)
if __name__ == '__main__':
threading.Thread(target=update_temperature, daemon=True).start()
app.run(host='0.0.0.0', port=5000)
Run the Flask application:
Open your browser and visit http://:5000 to see the current temperature.
Congratulations, you have completed your first IoT application! Through this example, we learned:
-
How to collect data from a sensor.
-
How to use Python to upload data to the cloud.
-
How to build a web interface using Flask.
Of course, this is just the beginning of IoT. In the future, you can add more sensors and even implement complex automation processes.
The world of IoT has opened its doors for you; welcome to explore!
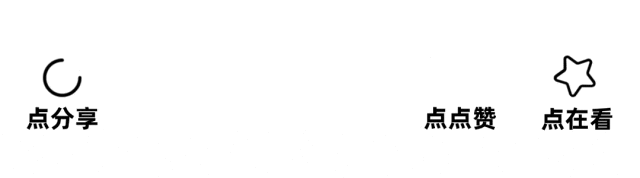