1. Introduction
The previous article discussed how to check process priority; this one explains how to set process priority.
There are two methods for setting process priority:
-
Command line; -
Source code;
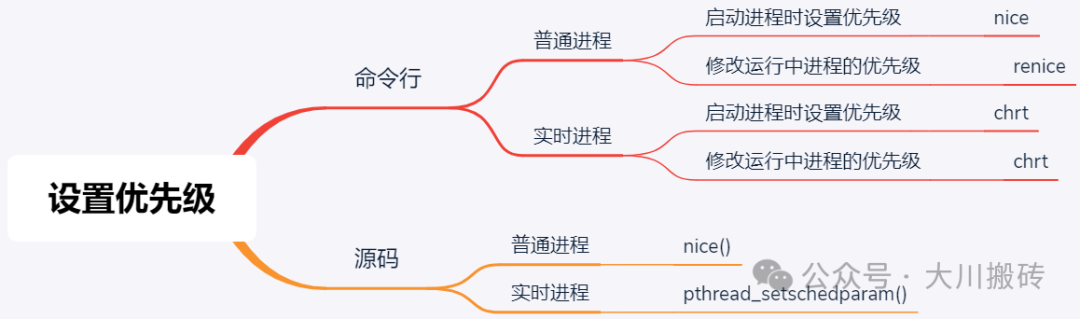
2. Command Line Method
main.c:
#include <stdio.h>
int main(int argc, char *argv[])
{
while(1)
{
;
}
return 0;
}
Compile:
gcc -o proc-pri main.c
To conveniently check the priority, I use the <span><span>top</span></span>
command.
2.1 Normal Processes
2.1.1 Set Priority When Starting a Process
sudo nice -n [nice value] [application]
Test:
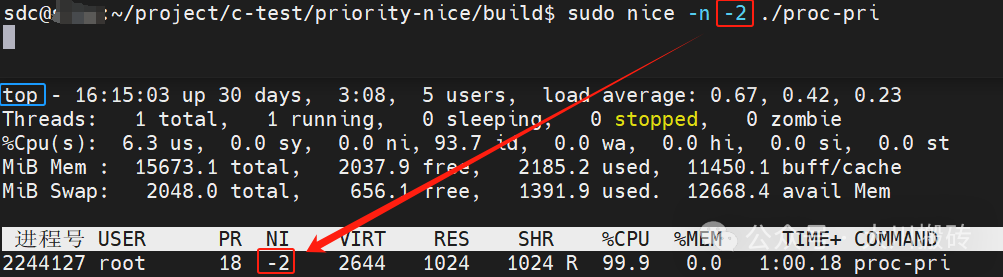
2.1.2 Modify Priority of Running Process
sudo renice -c [nice value] [application pid]
Test:
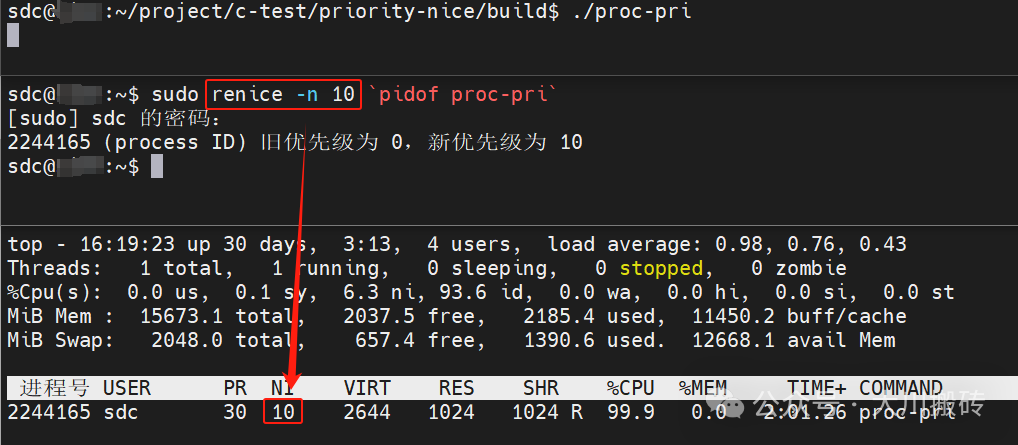
2.2 Real-Time Processes
2.2.1 Set Priority When Starting a Process
sudo chrt -f [priority] [application]
-f: Set the process scheduling policy to SCHED_FIFO;
-r: Set the process scheduling policy to SCHED_RR.
Test:
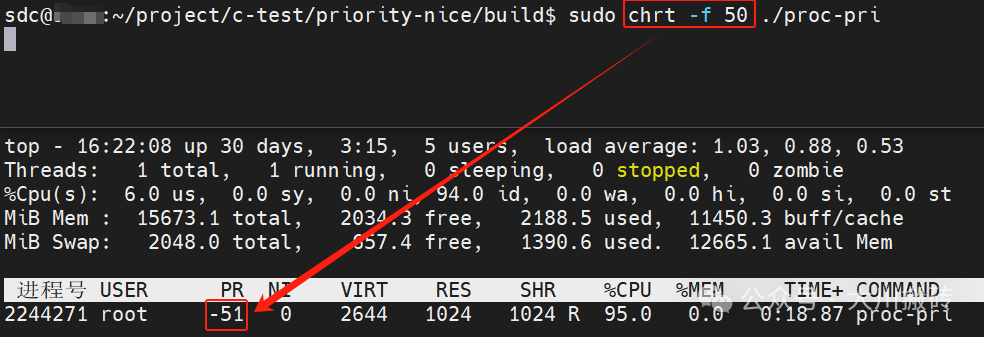
2.2.2 Modify Priority of Running Process
sudo chrt -fp [priority] [application pid]
Test:
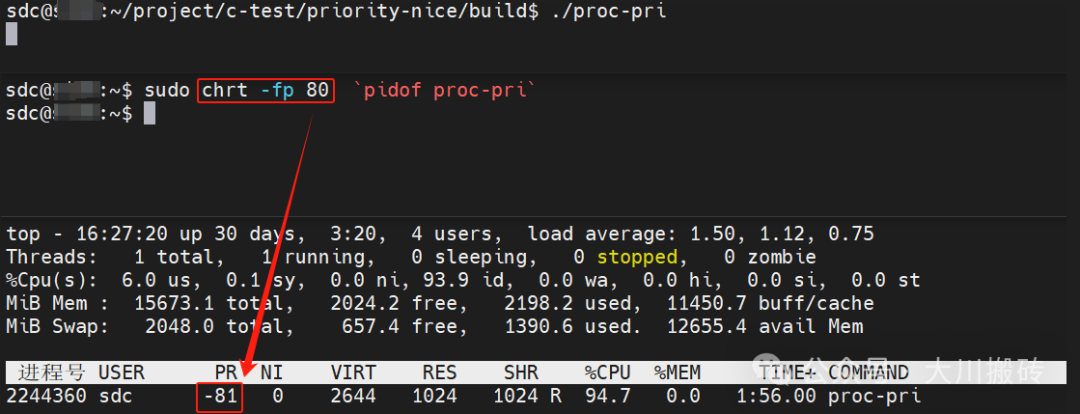
3. Source Code Method
3.1 Normal Processes
int nice(int inc);
main.c:
#include <stdio.h>
#include <unistd.h>
int main(int argc, char *argv[])
{
nice(-15);
while(1)
{
;
}
return 0;
}
Test:
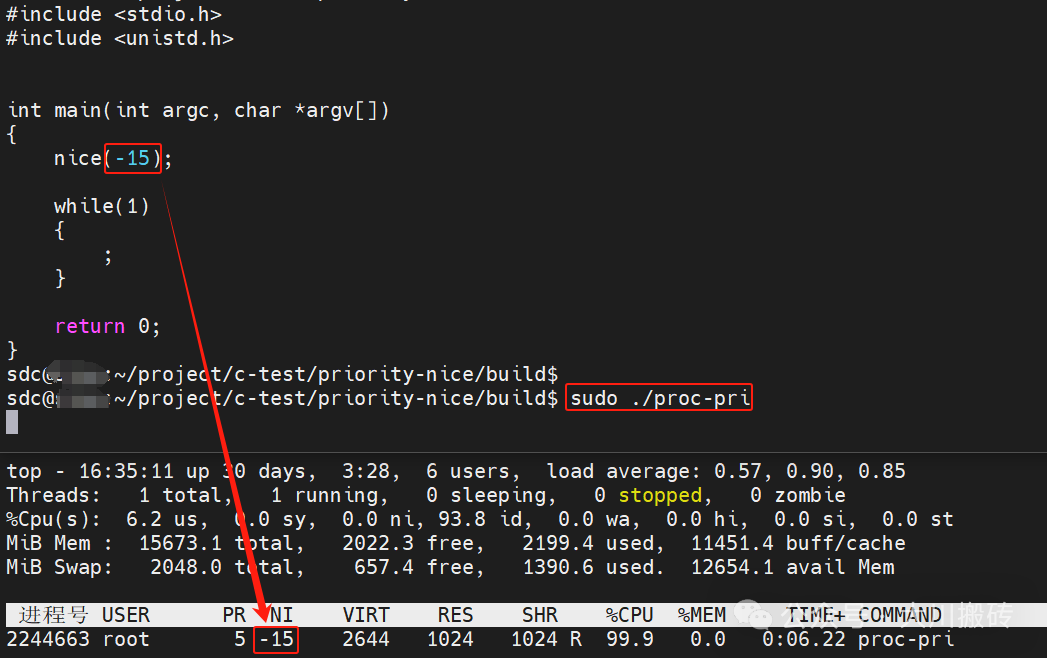
3.2 Real-Time Processes
#include <pthread.h>
int pthread_setschedparam(pthread_t thread, int policy,
const struct sched_param *param);
Test:
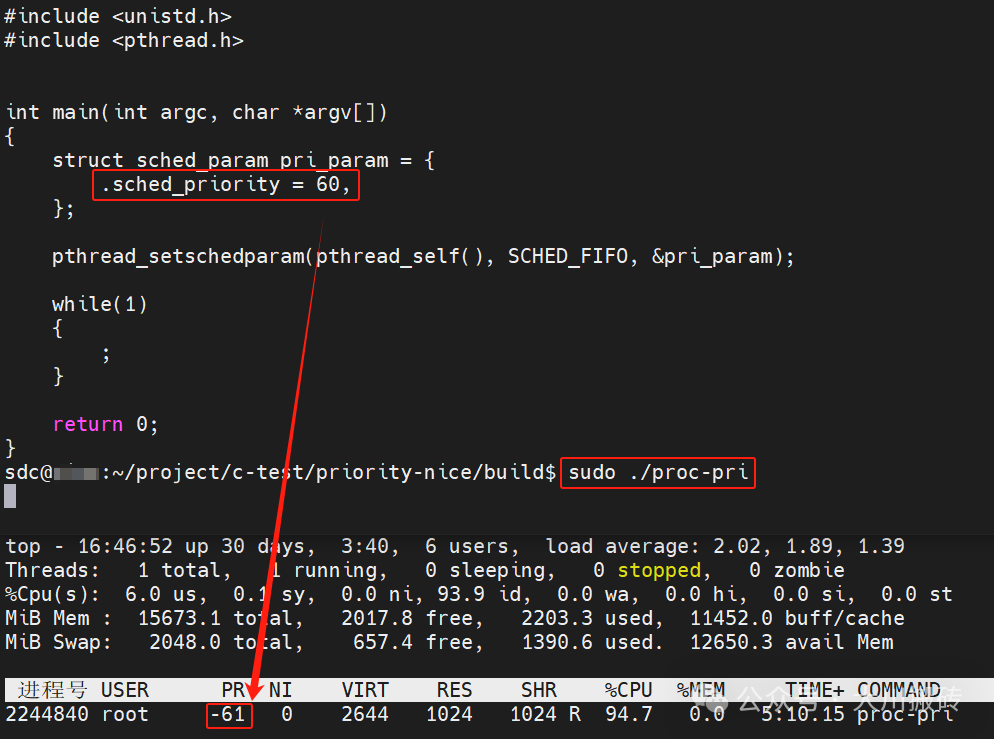
4. Conclusion
If you need to modify the priority of a certain program, you can first use the command line method for testing, and once the test is stable, you can solidify the priority in the code.