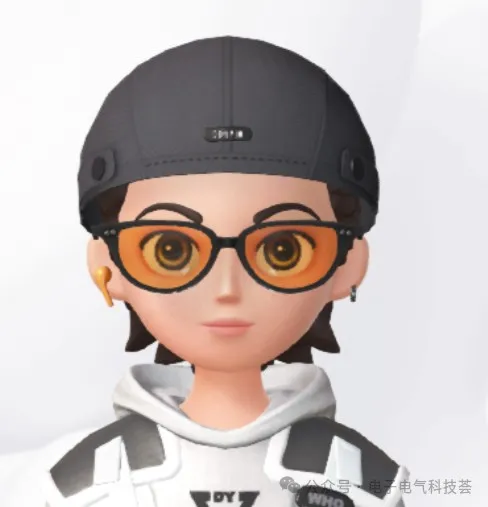
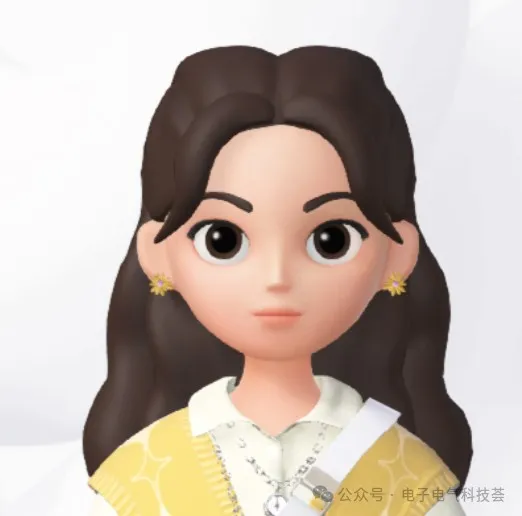
Real-Time Operating Systems (RTOS) are widely used in consumer electronics, entertainment products, household appliances, industrial equipment, medical instruments, military weapons, and scientific research equipment. They play a crucial role in safety-critical areas such as aerospace control systems, automotive industry, banking and finance, robotic systems, security and telecommunications, and traffic control.
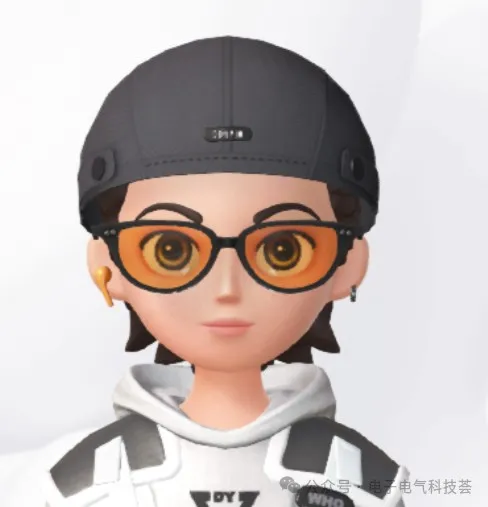
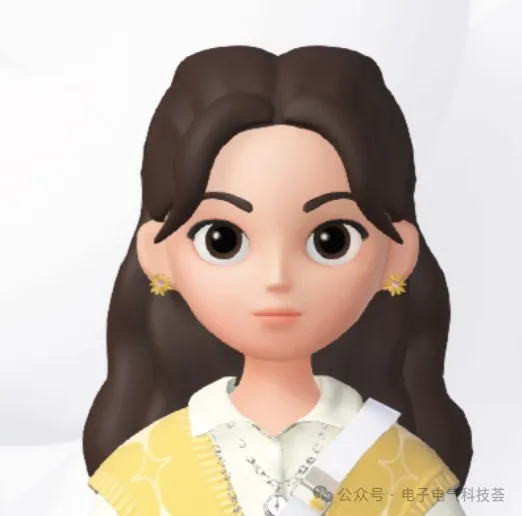
RTOS must support priority-based preemptive scheduling, task synchronization and communication must avoid priority inversion, and provide high-precision timers. RTOS often runs on resource-constrained devices, and the determinism of scheduling resources and scheduling time is an important requirement, which can ensure the use of system resources within a predetermined time.
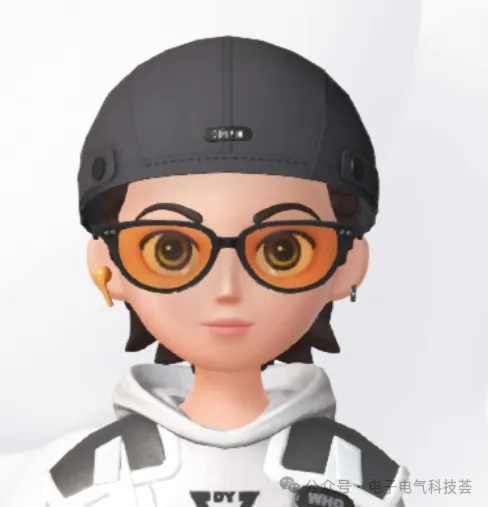
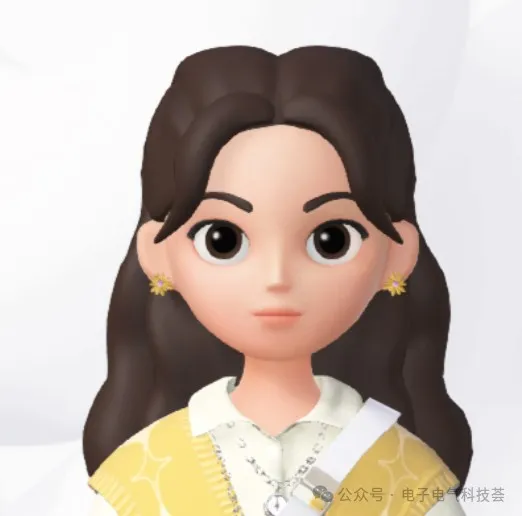
In our example, when a higher-priority task enters the ready state, the current task will stop running. This means a task can be in one of three states: running (executing), ready, and suspended. The behavior of tasks in preemptive scheduling can be described using the following state transition diagram.
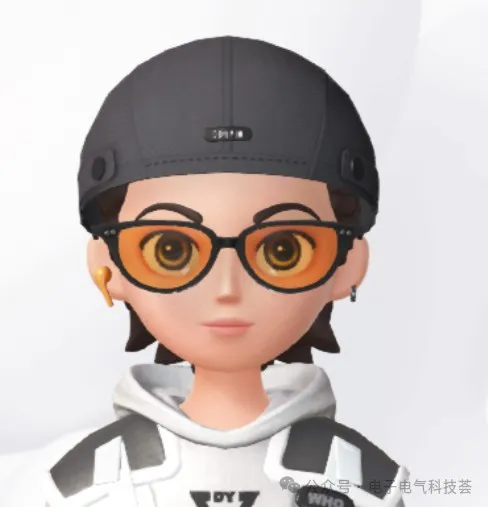
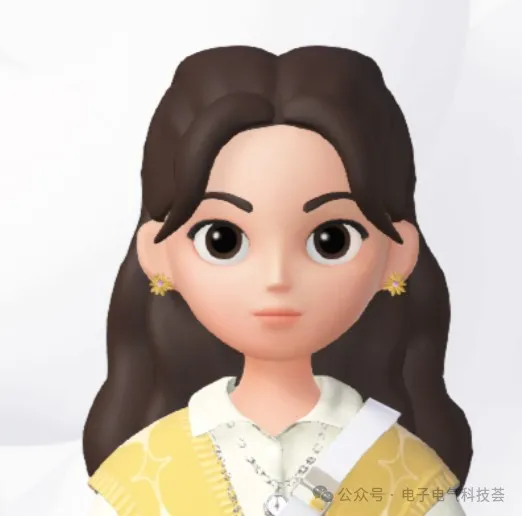
A task leaves the running state when it is suspended or preempted. When a task finishes execution or cannot continue for some reason, it will be suspended, for example, in this case, if the task needs to delay for a specific duration. When a task enters the suspended state, it releases the processor’s usage, allowing other tasks to run (see below). The release can be triggered by the task itself or enforced by the RTOS. A running task can release the processor in two ways: either the task completes the necessary operations and exits; or the task relinquishes control of the processor due to an internally generated signal (internal event).
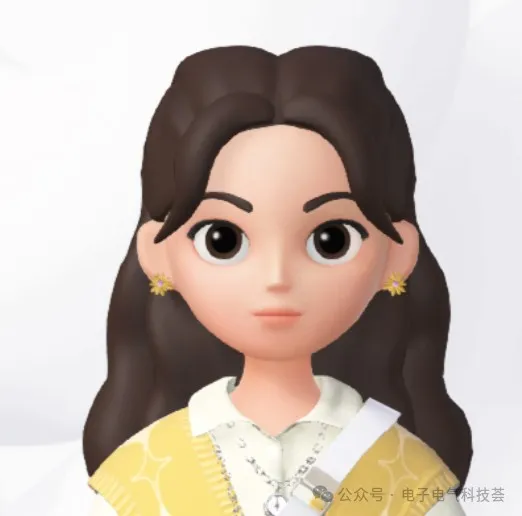
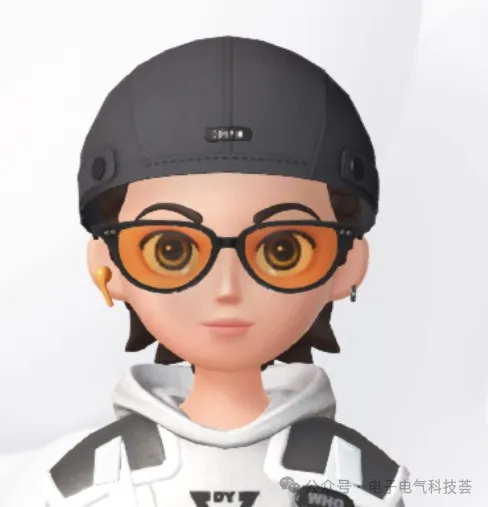
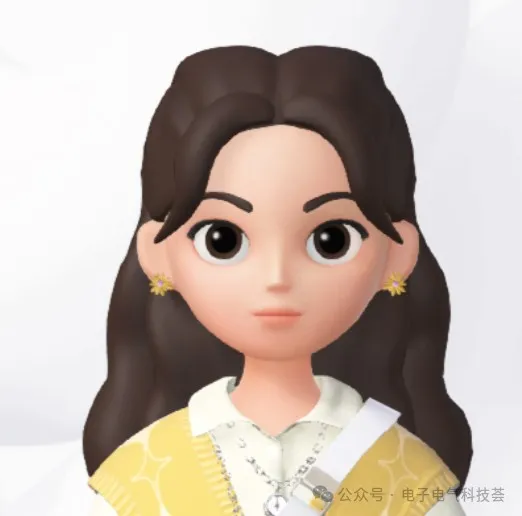
Chapter 1: Basics of Real-Time Operating Systems
1.1 Background
1.2 Developing High-Quality Software
1.3 Software Modeling
1.4 Importance of Time and Timing
1.5 Handling Multiple Tasks
1.6 Complex Situations with Multiple Tasks
1.7 Interrupts as Execution Engines – Simple Pre-Concurrency
1.8 Basic Functions of Real-Time Operating Systems
1.9 Execution Systems, Kernels, and Operating Systems
1.10 Task-Based Software Design – Review
1.11 Review
Chapter 2: Scheduling – Concepts and Implementations
2.1 Introduction
2.2 Simple Looping, Periodic Looping, and Cooperative Scheduling
2.3 Time-Slicing Scheduling
2.4 Task Priorities
2.5 Using Queues
2.6 Priority-Based Preemptive Scheduling
2.7 Implementation of Task Queues – Task Control Blocks
2.8 Process Descriptors
2.9 Ticks
2.10 Priority and System Response Speed
2.11 Bypassing the Scheduler
2.12 Code Sharing and Re-entrancy
2.13 Unpredictability of Runtime Behavior
2.14 More Details About Tasks
2.15 Review
Chapter 3: Controlling Resource Sharing with Mutex Mechanisms
3.1 Problems in Shared Resource Usage
3.2 Implementing Mutual Exclusion with a Single Flag
3.3 Semaphores
3.3.1 Binary Semaphores
3.3.2 General or Counting Semaphores
3.3.3 Limitations and Defects of Semaphores
3.4 Mutexes
3.5 Simple Monitors
3.6 Overview of Mutual Exclusion Mechanisms
3.7 Review
Chapter 4: Resource Sharing and Contention Issues
4.1 Detailed Explanation of Deadlock Issues Arising from Resource Contention
4.2 Designing Deadlock-Free Systems
4.3 Preventing Deadlocks
4.3.1 Allowing Resource Sharing
4.3.2 Allowing Request Preemption
4.3.3 Controlling Resource Allocation
4.4 Priority Inversion and Task Blocking
4.4.1 Priority Inversion Issues
4.4.2 Basic Priority Inheritance Protocol
4.4.3 Immediate Priority Ceiling Protocol
4.5 Deadlock Prevention and Performance Issues
4.6 Review
Chapter 5: Inter-Task Communication
5.1 Introduction
5.1.1 Overview of Inter-Task Communication
5.1.2 Cooperation and Synchronization
5.2 Task Interaction Without Data Transfer
5.2.1 Task Cooperation Mechanisms
5.2.2 Using Event Flags for Unidirectional Task Synchronization
5.2.3 Using Signals for Bidirectional Task Synchronization
5.3 Data Transfer Without Task Synchronization or Cooperation
5.3.1 Overview
5.3.2 Memory Pools
5.3.3 Queues
5.4 Task Synchronization with Data Transfer
5.5 Review
Chapter 6: Usage and Management of Memory
6.1 Storing Digital Information in Embedded Systems
6.1.1 Introduction
6.1.2 Non-Volatile Data Storage
6.1.3 Volatile Data Storage
6.1.4 Memory Devices – A Simple Comparison of Flash and RAM
6.1.5 Memory Devices – A Simple Comparison of SRAM and DRAM
6.1.6 Embedded Systems – Structure of Storage Devices
6.2 Concepts and Implementations of Storage
6.3 Eliminating Inter-Task Interference
6.3.1 A Simple Method to Control Memory Access
6.3.2 Using Memory Protection Units to Control Memory Access
6.3.3 Using Memory Management Units to Control Memory Access
6.4 Dynamic Memory Allocation and Its Issues
6.4.1 Memory Allocation and Fragmentation
6.4.2 Memory Allocation and Leaks
6.4.3 Safe Memory Allocation
6.5 Memory Management and Solid State Drives
6.6 Review
Chapter 7: Multiprocessor Systems
7.1 What is an Embedded Multiprocessor?
7.1.1 Why Use Multiprocessors?
7.1.2 Overview of Processor Architectures
7.1.3 Multicore Processors – Homogeneous and Heterogeneous Types
7.1.4 Multi-Machine System Structures
7.2 Software Issues – Job Partitioning and Allocation
7.2.1 Introduction
7.2.2 Building Software as a Set of Functions
7.2.3 Building Software as a Set of Data Processing Operations
7.3 Issues in Software Control and Execution
7.3.1 Basic Operating System Issues
7.3.2 Scheduling and Execution in AMP Systems
7.3.3 Scheduling and Execution in SMP Systems
7.3.4 Scheduling and Execution in BMP and Hybrid Systems
7.3.5 Comparison Between Multiprocessor Modes
7.4 Review
Chapter 8: Distributed Systems
8.1 Software Structure of Distributed Systems
8.2 Communication and Timing Issues in Distributed Systems
8.3 Mapping Software to the Hardware of Distributed Systems
8.4 Review
Chapter 9: Analysis of Scheduling Strategies
9.1 Overview
9.2 Priority-Based Non-Preemptive Scheduling Strategies
9.3 Priority-Based Static Preemptive Scheduling Strategies – Overview
9.4 Priority-Based Static Preemptive Scheduling Strategies – Monotonic Rate Scheduling
9.5 Priority-Based Static Preemptive Scheduling Strategies – Heuristic Methods Combining Priority and Importance
9.6 Priority-Based Dynamic Preemptive Scheduling Strategies – Overview
9.7 Priority-Based Dynamic Preemptive Scheduling Strategies – Earliest Deadline Scheduling
9.8 Priority-Based Dynamic Preemptive Scheduling Strategies – Computation Time Scheduling
9.9 Priority-Based Dynamic Preemptive Scheduling Strategies – Idle Time/Slack Scheduling
9.10 Improving Processor Utilization – Rate Monotonic
9.11 Scheduling Strategies – Final Explanation
9.12 Scheduling Timing Diagrams – Symbol Overview
9.13 Review
Chapter 10: Operating Systems: Basic Structures and Functions
10.1 Background
10.2 Implementing Simple Multitasking via Interrupts
10.3 Microkernel
10.4 Microkernel
10.5 General Embedded RTOS
10.6 Review
Chapter 11: Performance and Benchmarking of RTOS
11.1 Overview
11.2 Measuring Computer Performance – Benchmarking
11.2.1 Overview
11.2.2 Computational Performance Benchmarking
11.2.3 Operating System Performance
11.3 Time Overheads in Processor Systems
11.4 Operating System Performance and Representative Benchmarking
11.5 Operating System Performance and Comprehensive Benchmarking
11.5.1 Overview
11.5.2 Basic Requirements
11.5.3 Testing Categories
11.5.4 Baseline (Reference) Testing Data
11.5.5 Stress Testing Methods
11.6 Review
Chapter 12: Testing and Debugging of Multitasking Software
12.1 Scenario Introduction
12.2 Testing and Developing Multitasking Software – Professional Methods
12.3 In-Target Testing – Practical Tool Functionality
12.3.1 Overview
12.3.2 Testing RTOS Using Dedicated Control and Data Acquisition Tools
12.3.3 Testing RTOS Using On-Chip Data Storage Methods
12.3.4 Testing RTOS Using Host System Data Storage Facilities
12.4 Target System Testing – Practical Points
12.4.1 Introduction
12.4.2 Testing Concurrency of Individual Tasks
12.4.3 Implementing and Testing Concurrent Operations
12.5 Review
Chapter 13: Using RTOS in Critical Systems
13.1 Introduction to Critical Systems and Safety Integrity Levels
13.2 Operating System Issues
13.3 Issues and Remedies in RAM Usage
13.3.1 Overview
13.3.2 Memory Loss
13.3.3 Memory Exhaustion
13.4 Stack Usage
13.4.1 Stack Using Statically Allocated RAM
13.4.2 Improving Stack Reliability
13.5 Runtime Issues
13.5.1 Overview
13.5.2 Deadline and Response Time Issues
13.5.3 Reducing Interference Between Tasks
13.5.4 Handling Unpredictable Functional Behavior
13.6 Monitoring and Detecting Runtime Failures
13.6.1 Introduction to Watchdog Timers
13.6.2 Using WDT in Single-Task Designs
13.6.3 Windowed Watchdog Timer
13.6.4 Using WDT in Multi-Task Designs
13.7 Operating Systems and Critical Distributed Applications
13.8 Running Multiple Different Applications Through Time Partitioning
13.9 Design Guidelines
13.10 Review
Chapter 14: Conclusion
14.1 Tasks, Threads, and Processes
14.1.1 Overview
14.1.2 Program Execution in Embedded Environments – Introductory Guidance
14.1.3 Activities, Applications, and Tasks of Software
14.1.4 Concurrency Within Single Processor Tasks
14.1.5 Running Multiple Applications
14.1.6 Summary
14.2 Comparison of RTOS and GPOS
Appendix A Important Infrastructure
A.1 Inter-Processor Communication
A.2 Graphical User Interfaces in Embedded Systems
A.3 Review
Appendix B Reference Guide
Appendix C Glossary
Book Purchase Link