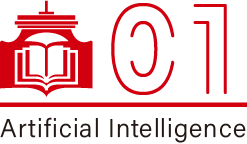
Content Features
The 3rd edition has been completely revised: it includes new information about the ARM Cortex-M4 processor; updates the explanation of the ARM Cortex-M3 processor; and compares the ARM Cortex-M3 and ARM Cortex-M4, facilitating the porting of other processor architectures to ARM Cortex-M3 and ARM Cortex-M4. Other details of this edition’s revisions are as follows:
-
Two new chapters discussing DSP features and the CMSIS-DSP software library have been added, introducing the basics of DSP and how to write DSP software for Cortex-M4, including examples using the CMSIS-DSP library and knowledge about the DSP performance of Cortex-M4.
-
A new chapter introducing the floating-point unit of Cortex-M4 and its use has been added.
-
A new chapter introducing the use of embedded operating systems (based on CMSIS-RTOS) and the processor features that support embedded operating systems has been added.
-
Various debugging techniques and troubleshooting.
-
Content on software porting from other processors.
-
Various easy-to-understand example diagrams and quick reference appendices.
In addition, this book introduces background knowledge of the ARM architecture and instruction set, interrupt handling, and other processor features, and describes how to set up and utilize available advanced features such as the memory protection unit (MPU). The chapters discussing getting started with Keil MDK, IAR EWARM, gcc, and CooCox CoIDE tools can provide beginners with some help in writing program code, including some important software development issues, such as the use of low-power features, handling information input/output, mixed programming in assembly and C, and other advanced technical topics.
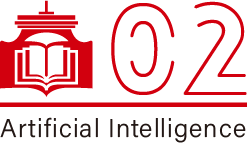
Joseph Yiu is a senior expert at ARM in the UK, with 14 years of experience in the semiconductor industry (over 12 years working at ARM). He has participated in multiple processor design projects, including ARM Cortex-M3/M4 and Cortex-M0, and has been involved in the development of various ARM IP (intellectual property) products. Joseph Yiu is an expert in microcontroller system-level design and has delved into many related fields, including ARM Cortex-M series microcontroller software development, microcontroller market, and system-on-chip design technology. Other representative works include “The Definitive Guide to ARM Cortex-M0” (Simplified Chinese edition published by Tsinghua University Press) and “The Definitive Guide to ARM Cortex-M3 (2nd Edition)” (Simplified Chinese edition published by Tsinghua University Press).
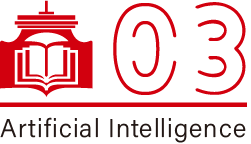
Editor’s Recommendation
-
Joseph Yiu, a microcontroller system-level design expert at ARM, is renowned worldwide for his representative works.
-
Reinhard Keil, director of microcontroller tools at ARM, wrote the preface.
-
A brand new edition, systematically discussing the ARM Cortex-M3 and Cortex-M4 cores, architecture, instruction set, compiler, programming, and software porting, is a classic work.
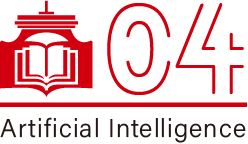
Translator’s Preface 1
Recommended Preface 3
Foreword 5
About This Book 7
Terminology and Abbreviations 9
Conventions of This Book 11
Chapter 1 Introduction to ARM Cortex-M Processors
1.1 What are ARM Cortex-M Processors
1.1.1 Cortex-M3 and Cortex-M4 Processors
1.1.2 Cortex-M Processor Family
1.1.3 Difference Between Processors and Microcontrollers
1.1.4 ARM and Microcontroller Suppliers
1.1.5 Choosing Cortex-M3 and Cortex-M4 Microcontrollers
1.2 Advantages of Cortex-M Processors
1.2.1 Low Power Consumption
1.2.2 Performance
1.2.3 Energy Efficiency
1.2.4 Code Density
1.2.5 Interrupts
1.2.6 Ease of Use
1.2.7 Scalability
1.2.8 Debug Features
1.2.9 OS Support
1.2.10 Various System Features
1.2.11 Software Portability and Reusability
1.2.12 Selection (Devices, Tools, OS, etc.)
1.3 Applications of ARM Cortex-M Processors
1.4 Resources for ARM Processors and ARM Microcontrollers
1.4.1 What is Available on the ARM Website
1.4.2 Documentation Provided by Microcontroller Suppliers
1.4.3 Documentation Provided by Tool Suppliers
1.4.4 Other Resources
1.5 Background and History
1.5.1 Brief History of ARM
1.5.2 Development of ARM Processors
1.5.3 Architecture Versions of Thumb ISA
1.5.4 Processor Naming
1.5.5 About the ARM Ecosystem
Chapter 2 Introduction to Embedded Software Development
2.1 How ARM Microcontrollers are Structured
2.2 What Needs to be Prepared Initially
2.2.1 Development Components
2.2.2 Development Boards
2.2.3 Debug Adapters
2.2.4 Software Device Drivers
2.2.5 Examples
2.2.6 Documentation and Other Resources
2.2.7 Other Devices
2.3 Software Development Process
2.4 Compiling Applications
2.5 Software Flow
2.5.1 Polling
2.5.2 Interrupt-Driven
2.5.3 Multi-Tasking Systems
2.6 Data Types in C Programs
2.7 Input, Output, and Peripheral Access
2.8 Microcontroller Interfaces
2.9 Cortex Microcontroller Software Interface Standard (CMSIS)
2.9.1 Introduction to CMSIS
2.9.2 Standardization Done by CMSIS-Core
2.9.3 Organization of CMSIS-Core
2.9.4 How to Use CMSIS-Core
2.9.5 Advantages of CMSIS
2.9.6 Multiple Versions of CMSIS
Chapter 3 Technical Overview
3.1 General Information about Cortex-M3 and Cortex-M4 Processors
3.1.1 Processor Types
3.1.2 Processor Architecture
3.1.3 Instruction Sets
3.1.4 Module Block Diagrams
3.1.5 Memory Systems
3.1.6 Interrupt and Exception Support
3.2 Characteristics of Cortex-M3 and Cortex-M4 Processors
3.2.1 Performance
3.2.2 Code Density
3.2.3 Low Power Consumption
3.2.4 Memory Systems
3.2.5 Memory Protection Units
3.2.6 Interrupt Handling
3.2.7 OS Support and System-Level Features
3.2.8 Special Features of Cortex-M4
3.2.9 Ease of Use
3.2.10 Debug Support
3.2.11 Scalability
3.2.12 Compatibility
Chapter 4 Architecture
4.1 Introduction to Architecture
4.2 Programming Model
4.2.1 Operating Modes and States
4.2.2 Registers
4.2.3 Special Registers
4.2.4 Floating-Point Registers
4.3 Application State Registers
4.3.1 Integer State Flags
4.3.2 Q State Flags
4.3.3 GE Bits
4.4 Memory Systems
4.4.1 Memory System Characteristics
4.4.2 Memory Mapping
4.4.3 Stack Storage
4.4.4 Memory Protection Units (MPU)
4.5 Exceptions and Interrupts
4.5.1 What are Exceptions
4.5.2 Nested Vector Interrupt Controller (NVIC)
4.5.3 Vector Tables
4.5.4 Error Handling
4.6 System Control Block (SCB)
4.7 Debugging
4.8 Reset and Reset Procedure
Chapter 5 Instruction Set
5.1 Introduction to the Instruction Set of ARM Cortex-M Processors
5.2 Instruction Set Comparison Among ARM Cortex-M Processors
5.3 Understanding Assembly Language Syntax
5.4 Usage of Instruction Suffixes
5.5 Unified Assembly Language (UAL)
5.6 Instruction Set
5.6.1 Data Transfer Within the Processor
5.6.2 Memory Access Instructions
5.6.3 Arithmetic Operations
5.6.4 Logical Operations
5.6.5 Shift and Rotate Instructions
5.6.6 Data Conversion Operations (Unpacking and Reordering)
5.6.7 Bit Field Processing Instructions
5.6.8 Comparison and Testing
5.6.9 Program Flow Control
5.6.10 Saturation Operations
5.6.11 Exception-Related Instructions
5.6.12 Sleep Mode-Related Instructions
5.6.13 Memory Barrier Instructions
5.6.14 Other Instructions
5.6.15 Unsupported Instructions
5.7 Cortex-M4 Specific Instructions
5.7.1 Introduction to Enhanced DSP Extensions of Cortex-M4
5.7.2 SIMD and Saturation Instructions
5.7.3 Multiplication and MAC Instructions
5.7.4 Packing and Unpacking
5.7.5 Floating-Point Instructions
5.8 Barrel Shifter
5.9 Accessing Special Registers and Special Instructions in Programming
5.9.1 Introduction
5.9.2 Intrinsic Functions
5.9.3 Inline Assembly and Embedded Assembly
5.9.4 Using Other Compiler-Related Features
5.9.5 Accessing Special Registers
Chapter 6 Memory Systems
6.1 Introduction to Memory System Characteristics
6.2 Memory Mapping
6.3 Connecting Processors to Memory and Peripherals
6.4 Memory Requirements
6.5 Memory Endianness
6.6 Data Alignment and Unaligned Data Access Support
6.7 Bit Field Operations
6.7.1 Introduction
6.7.2 Advantages of Bit Field Operations
6.7.3 Bit Field Operations of Different Data Sizes
6.7.4 Implementation of Bit Field Operations in C Programs
6.8 Default Memory Access Permissions
6.9 Memory Access Attributes
6.10 Exclusive Access
6.11 Memory Barriers
6.12 Memory Systems in Microcontrollers
Chapter 7 Exceptions and Interrupts
7.1 Introduction to Exceptions and Interrupts
7.2 Types of Exceptions
7.3 Introduction to Interrupt Management
7.4 Priority Definitions
7.5 Vector Tables and Vector Table Relocation
7.6 Interrupt Input and Pending Behavior
7.7 Introduction to Exception Process
7.7.1 Accepting Exception Requests
7.7.2 Exception Entry Process
7.7.3 Executing Exception Handling
7.7.4 Exception Return
7.8 NVIC Register Details for Interrupt Control
7.8.1 Introduction
7.8.2 Interrupt Enable Register
7.8.3 Setting Interrupt Pending and Clearing Interrupt Pending
7.8.4 Active State
7.8.5 Priority
7.8.6 Software Triggered Interrupt Register
7.8.7 Interrupt Controller Type Register
7.9 SCB Register Details for Exception and Interrupt Control
7.9.1 Introduction to SCB Registers
7.9.2 Interrupt Control and State Register (ICSR)
7.9.3 Vector Table Offset Register (VTOR)
7.9.4 Application Interrupt and Reset Control Register (AIRCR)
7.9.5 System Handler Priority Register (SCB->SHP[0~11])
7.9.6 System Handler Control and State Register (SCB->SHCSR)
7.10 Special Register Details for Masking Exceptions or Interrupts
7.10.1 PRIMASK
7.10.2 FAULTMASK
7.10.3 BASEPRI
7.11 Example Steps for Setting Interrupts
7.11.1 Simple Case
7.11.2 Case When Relocating Vector Tables
7.12 Software Interrupts
7.13 Key Points and Tips
Chapter 8 In-Depth Understanding of Exception Handling
8.1 Introduction
8.1.1 About This Chapter
8.1.2 C Implementation of Exception Handling
8.1.3 Stack Frames
8.1.4 EXC_RETURN
8.2 Exception Process
8.2.1 Exception Entry and Stack Push
8.2.2 Exception Return and Stack Pop
8.3 Interrupt Waiting and Exception Handling Optimization
8.3.1 What is Interrupt Waiting
8.3.2 Interrupts During Multi-Cycle Instruction Execution
8.3.3 Tail Chaining
8.3.4 Late Arrival
8.3.5 Stack Pop Preemption
8.3.6 Lazy Stack Push
Chapter 9 Low Power and System Control Features
9.1 Low Power Design
9.1.1 What Low Power Means for Microcontrollers
9.1.2 Low Power System Requirements
9.1.3 Low Power Features of Cortex-M3 and Cortex-M4 Processors
9.2 Low Power Features
9.2.1 Sleep Modes
9.2.2 System Control Register (SCR)
9.2.3 Entering Sleep Mode
9.2.4 Wake-Up Conditions
9.2.5 Sleep Characteristics Upon Exit
9.2.6 Send Event Pending (SEVONPEND)
9.2.7 Sleep Extension/Wake-Up Delay
9.2.8 Wake-Up Interrupt Controller (WIC)
9.2.9 Event Communication Interface
9.3 Using WFI and WFE in Programming
9.3.1 When to Use WFI
9.3.2 Using WFE
9.4 Developing Low Power Applications
9.4.1 Reducing Dynamic Power Consumption
9.4.2 Reducing Active Cycle
9.4.3 Reducing Sleep Mode Current
9.5 SysTick Timer
9.5.1 Why Have a SysTick Timer
9.5.2 SysTick Timer Operation
9.5.3 Using SysTick Timer
9.5.4 Other Considerations
9.6 Self-Reset
9.7 CPU ID Basic Register
9.8 Configuration Control Register
9.8.1 Introduction to CCR
9.8.2 STKALIGN Bit
9.8.3 BFHFNMIGN Bit
9.8.4 DIV_O_TRP Bit
9.8.5 UNALIGN_TRP Bit
9.8.6 USERSETMPEND Bit
9.8.7 NONBASETHRDENA Bit
9.9 Auxiliary Control Register
9.10 Coprocessor Access Control Register
Chapter 10 OS Support Features
10.1 Introduction to OS Support Features
10.2 Shadow Stack Pointer
10.3 SVC Exception
10.4 PendSV Exception
10.5 Actual Context Switching
10.6 Exclusive Access and Embedded OS
Chapter 11 Memory Protection Units
11.1 Introduction to MPU
11.1.1 About MPU
11.1.2 Using MPU
11.2 MPU Registers
11.2.1 MPU Type Register
11.2.2 MPU Control Register
11.2.3 MPU Region Number Register
11.2.4 MPU Base Address Register
11.2.5 MPU Region Base Attributes and Size Register
11.2.6 MPU Alias Register
11.3 Setting Up MPU
11.4 Memory Barriers and MPU Configuration
11.5 Using Subregion Disable
11.5.1 Allowing Efficient Memory Partitioning
11.5.2 Reducing Total Number of Required Regions
11.6 Considerations When Using MPU
11.6.1 Program Code
11.6.2 Data Storage
11.6.3 Peripherals
11.7 Other Uses of MPU
11.8 Differences Between MPU in Cortex-M0+ Processors
Chapter 12 Error Exceptions and Error Handling
12.1 Introduction to Error Exceptions
12.2 Causes of Errors
12.2.1 Memory Management (MemManage) Errors
12.2.2 Bus Errors
12.2.3 Usage Errors
12.2.4 HardFault
12.3 Enabling Error Handling
12.3.1 MemManage Errors
12.3.2 Bus Errors
12.3.3 Usage Errors
12.3.4 HardFault
12.4 Error Status Register and Error Address Register
12.4.1 Introduction
12.4.2 MemManage Error Information
12.4.3 Bus Error Information
12.4.4 Usage Error Information
12.4.5 HardFault Status Register
12.4.6 Debug Error Status Register (DFSR)
12.4.7 Error Address Registers MMFAR and BFAR
12.4.8 Auxiliary Error Status Register
12.5 Analyzing Errors
12.6 Errors Related to Exception Handling
12.6.1 Stack Push
12.6.2 Stack Pop
12.6.3 Lazy Stack Push
12.6.4 Fetching Vector
12.6.5 Illegal Return
12.6.6 Priority and Stack Push or Pop Errors
12.7 Locking
12.7.1 What is Locking
12.7.2 Avoiding Locking
12.8 Error Handling
12.8.1 HardFault for Debugging
12.8.2 Error Masking
12.9 Other Information
12.9.1 Running a System with Two Stacks
12.9.2 Detecting Stack Overflow
Chapter 13 Floating-Point Operations
13.1 About Floating-Point Numbers
13.1.1 Introduction
13.1.2 Single-Precision Floating-Point Numbers
13.1.3 Half-Precision Floating-Point Numbers
13.1.4 Double-Precision Floating-Point Numbers
13.1.5 Floating-Point Support in Cortex-M Processors
13.2 Cortex-M4 Floating-Point Unit (FPU)
13.2.1 Introduction to Floating-Point Unit
13.2.2 Introduction to Floating-Point Registers
13.2.3 CPACR Register
13.2.4 Floating-Point Register Group
13.2.5 Floating-Point Status and Control Register (FPSCR)
13.2.6 Floating-Point Context Control Register (FPU->FPCCR)
13.2.7 Floating-Point Context Address Register (FPU->FPCAR)
13.2.8 Floating-Point Default Status Control Register (FPU->FPDSCR)
13.2.9 Media and Floating-Point Feature Registers (FPU->MVFR0, FPU->MVFR1)
13.3 In-Depth Lazy Stack Push
13.3.1 Key Points of Lazy Stack Push Feature
13.3.2 Case 1: No Floating-Point Context in Interrupted Task
13.3.3 Case 2: Floating-Point Context in Interrupted Task, None in ISR
13.3.4 Case 3: Both Interrupted Task and ISR Have Floating-Point Context
13.3.5 Case 4: Interrupt Nesting, and Floating-Point Context Exists in Second Interrupt Handling
13.3.6 Case 5: Interrupt Nesting, and Both Interrupt Handlings Have Floating-Point Context
13.3.7 Lazy Stack Push Interrupts
13.3.8 Interrupts of Floating-Point Instructions
13.4 Using the Floating-Point Unit
13.4.1 Floating-Point Support in CMSIS-Core
13.4.2 Implementing Floating-Point Programming in C
13.4.3 Compiler Command Line Options
13.4.4 ABI Options: Hard-vfp and Soft-vfp
13.4.5 Special FPU Modes
13.5 Floating-Point Exceptions
13.6 Key Points and Tips
13.6.1 Runtime Libraries for Microcontrollers
13.6.2 Debugging Operations
Chapter 14 Debugging and Tracing Features
14.1 Introduction to Debugging and Tracing Features
14.1.1 What are Debugging Features
14.1.2 What are Tracing Features
14.1.3 Summary of Debugging and Tracing Features
14.2 Debug Architecture
14.2.1 CoreSight Debug Architecture
14.2.2 Processor Debug Interface
14.2.3 Debug Port (DP), Access Port (AP), and Debug Access Port (DAP)
14.2.4 Trace Interface
14.2.5 CoreSight Features
14.3 Debug Modes
14.4 Debug Events
14.5 Breakpoint Features
14.6 Introduction to Debug Components
14.6.1 Processor Debug Support
14.6.2 Flash Patch and Breakpoint (FPB) Unit
14.6.3 Data Watchpoint and Trace (DWT) Unit
14.6.4 Instruction Trace Macrocell
14.6.5 Embedded Trace Macrocell (ETM)
14.6.6 Trace Port Interface Unit (TPIU)
14.6.7 ROM Table
14.6.8 AHB Access Port (AHB-AP)
14.7 Debug Operations
14.7.1 Debug Connection
14.7.2 Flash Programming
14.7.3 Breakpoints
Chapter 15 Getting Started with Keil ARM Microcontroller Development Kit
15.1 Introduction
15.2 Typical Program Compilation Process
15.3 Getting Started with μVision
15.4 Project Options
15.4.1 Device Options
15.4.2 Target Options
15.4.3 Output Options
15.4.4 Listing Options
15.4.5 User Options
15.4.6 C/C++ Options
15.4.7 Asm Options
15.4.8 Linker Options
15.4.9 Debug Options
15.4.10 Utilities Options
15.5 Using IDE and Debugger
15.6 Using Instruction Set Simulator
15.7 Running Programs in SRAM
15.8 Optimization Options
15.9 Other Information and Key Points
15.9.1 Stack and Heap Storage Size Configuration
15.9.2 Other Information
Chapter 16 Getting Started with IAR Embedded Workbench for ARM
16.1 Introduction to IAR Embedded Workbench for ARM
16.2 Typical Program Compilation Process
16.3 Creating a Simple Blinky Project
16.4 Project Options
16.5 Tips and Key Points
Chapter 17 Getting Started with GCC
17.1 GCC Toolchain
17.2 Typical Development Process
17.3 Creating a Simple Blinky Project
17.4 Introduction to Command Line Options
17.5 Flash Programming
17.5.1 Using Keil MDK-ARM
17.5.2 Using Third-Party Flash Programming Tools
17.6 Using Keil MDK-ARM and ARM Embedded Processor GNU Tools Together
17.7 Using CoIDE and ARM Embedded Processor GNU Tools Together
17.8 Commercial Development Components Based on gcc
17.8.1 Atollic TrueSTUDIO for ARM
17.8.2 Red Suite
17.8.3 CrossWorks for ARM
Chapter 18 Input and Output Software Examples
18.1 Generating Output
18.2 Redirecting to Instruction Trace Macrocell (ITM)
18.2.1 Introduction
18.2.2 Keil MDK-ARM
18.2.3 IAR Embedded Workbench
18.2.4 GCC
18.3 Half-Host
18.4 Redirecting to Peripherals
Chapter 19 Using Embedded Operating Systems
19.1 Introduction to Embedded OS
19.1.1 What is Embedded OS
19.1.2 When to Use Embedded OS
19.1.3 Role of CMSIS-RTOS
19.2 Keil RTX Real-Time Kernel
19.2.1 About RTX
19.2.2 Feature Overview
19.2.3 RTX and CMSIS-RTOS
19.2.4 Threads
19.3 CMSIS-RTOS Examples
19.3.1 Simple CMSIS-RTOS with Two Threads
19.3.2 Introduction to Inter-Thread Communication
19.3.3 Signaled Event Communication
19.3.4 Mutex
19.3.5 Semaphores
19.3.6 Message Queues
19.3.7 Mail Queues
19.3.8 Memory Pool Management Features
19.3.9 General Wait Functions and Timeout Values
19.3.10 Timer Features
19.3.11 Accessing Non-Privileged Devices
19.4 OS-Aware Debugging
19.5 Troubleshooting
19.5.1 Stack Size and Stack Alignment
19.5.2 Privilege Levels
19.5.3 Other Issues
Chapter 20 Assembly and Mixed Language Projects
20.1 Using Assembly Code in Projects
20.2 Interaction Between C and Assembly
20.3 Structure of Assembly Functions
20.4 Examples
20.4.1 Simple Example of ARM Toolchain (Keil MDK-ARM, DS-5)
20.4.2 Simple Example of ARM Embedded Processor GNU Tools
20.4.3 Accessing Special Registers
20.4.4 Data Storage
20.4.5 Hello World
20.4.6 Displaying Hexadecimal and Decimal Data
20.4.7 NVIC Interrupt Control
20.4.8 Unsigned Integer Square Root
20.5 Mixed Language Projects
20.5.1 Calling C Functions from Assembly
20.5.2 Calling Assembly Functions from C
20.5.3 Embedded Assembly (Keil MDK-ARM / ARM DS-5 Professional)
20.5.4 Inline Assembly
20.6 Intrinsic Functions
20.7 Idiom Recognition
Chapter 21 ARM Cortex-M4 and DSP Applications
21.1 DSP on Microcontrollers
21.2 Dot Product Examples
21.3 Architecture of Traditional DSP Processors
21.4 DSP Instructions of Cortex-M4
21.4.1 Registers and Data Types
21.4.2 Decimal Operations
21.4.3 SIMD Data
21.4.4 Load and Store Instructions
21.4.5 Arithmetic Instructions
21.4.6 General Optimization Strategies for Cortex-M4
21.4.7 Instruction Limitations
21.5 Writing Optimized DSP Code for Cortex-M4
21.5.1 Biquad Filter
21.5.2 Fast Fourier Transform
21.5.3 FIR Filter
Chapter 22 Using ARM CMSIS-DSP Library
22.1 Introduction to DSP Library
22.2 Pre-Built Binary Code
22.3 Function Command Rules
22.4 Getting Help
22.5 Example 1: DTMF Demodulation
22.5.1 Generating Sine Waves
22.5.2 Using FIR Filters for Analysis
22.5.3 Using FFT for Analysis
22.5.4 Using Biquad Filters for Analysis
22.5.5 DTMF Example Code
22.6 Example 2: Least Squares Motion Tracking
Chapter 23 Advanced Topics
23.1 Decisions and Jumps
23.1.1 Conditional Jumps
23.1.2 Complex Decision Trees
23.2 Performance Considerations
23.3 Double Word Stack Alignment
23.4 Various Methods of Semaphore Design
23.4.1 Implementing Semaphores with SVC Services
23.4.2 Using Bit Fields to Implement Semaphores
23.5 Non-Basic Thread Enablement
23.6 Reentrancy of Interrupt Services
23.7 Bit Data Processing Implemented in C
23.8 Startup Code
23.9 Stack Overflow Detection
23.9.1 Stack Analysis of Toolchain
23.9.2 Testing Analysis of Stack
23.9.3 Detecting Stack Overflow Based on Stack Layout
23.9.4 Using MPU
23.9.5 Using DWT and Debug Monitoring Exceptions
23.9.6 Stack Checks in OS Context Switching
23.10 Flash Patch Features
23.11 Versions of Cortex-M3 and Cortex-M4 Processors
23.11.1 Introduction
23.11.2 Changes from Cortex-M3 r0p0 to r1p0/r1p1
23.11.3 Changes from Cortex-M3 r1p1 to r2p0
23.11.4 Changes from Cortex-M3 r2p0 to r2p1
23.11.5 Changes from Cortex-M4 r0p0 to r0p1
Chapter 24 Software Porting
24.1 Introduction
24.2 Porting from 8-bit/16-bit MCUs to Cortex-M MCUs
24.2.1 Architectural Differences
24.2.2 General Adjustments
24.2.3 Memory Size Requirements
24.2.4 Optimizations No Longer Applicable for 8-bit or 16-bit Microcontrollers
24.2.5 Example: Porting from 8051 to ARM Cortex-M
24.3 Software Porting from ARM7TDMI to Cortex-M3/M4
24.3.1 Introduction to Hardware Differences
24.3.2 Assembly Language Files
24.3.3 C Language Files
24.3.4 Precompiled Object Files and Libraries
24.3.5 Optimizations
24.4 Software Porting Between Different Cortex-M Processors
24.4.1 Differences Between Different Cortex-M Processors
24.4.2 Required Software Changes
24.4.3 Embedded OS
24.4.4 Creating Portable Program Code for Cortex-M Processors
References
Appendices A~I (See the book’s accompanying website)
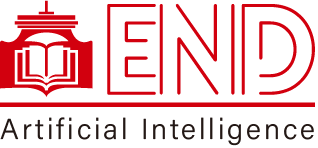
Leave a Comment
Your email address will not be published. Required fields are marked *