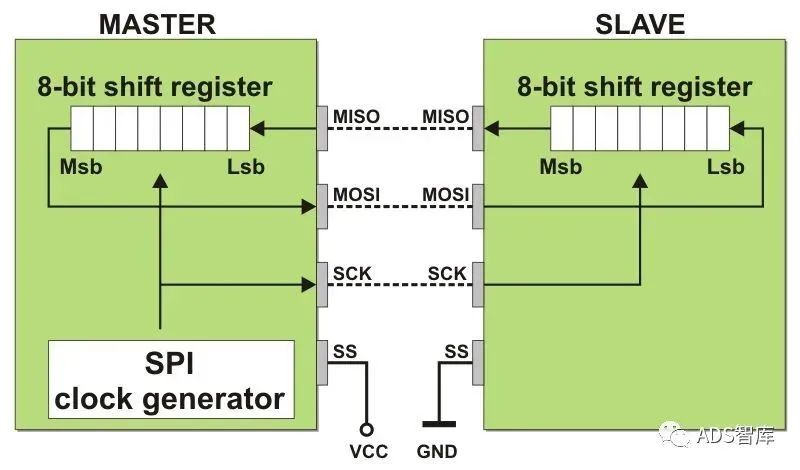
1 Introduction to SPI
SPI, short for Serial Peripheral Interface, is a synchronous serial communication protocol. It was first defined by Motorola for its MC68HCXX series processors. The SPI interface is primarily used in EEPROM, FLASH, real-time clocks, ADCs, as well as between digital signal processors and digital signal decoders. SPI is a high-speed, full-duplex, synchronous communication bus that only occupies four pins on the chip, saving pin space and providing convenience for PCB layout. Due to its ease of use, an increasing number of chips are integrating this communication protocol.
2 Features of SPI
2.1 Master-Slave Control Mode
SPI specifies that communication between two SPI devices must be controlled by the master device. A master device can control multiple slave devices by providing a clock and selecting the slave device through Slave Select. The SPI protocol also stipulates that the clock for the slave device is provided by the master device through the SCK pin; the slave device cannot generate or control the clock on its own. Without a clock, the slave device cannot function properly.
2.2 Synchronous Data Transmission
The master device generates corresponding clock pulses based on the data to be exchanged. These clock pulses form the clock signal, which controls when data is exchanged and when the received data is sampled between the two SPI devices to ensure synchronous data transmission.
2.3 Data Exchanges
The data transfer between SPI devices is also referred to as data exchange because the SPI protocol stipulates that an SPI device cannot act solely as a “transmitter” or “receiver” during data communication. In each clock cycle, the SPI device sends and receives one bit of data, essentially exchanging one bit of data. A slave device must be accessed by the master device before it can receive control signals sent from the master. Therefore, the master must first select the slave device through the SS/CS pin. During data transmission, each piece of received data must be sampled before the next data transmission. If the previously received data is not read, it may be discarded, causing the SPI physical module to fail. Therefore, it is common practice to read the data from the SPI device after the SPI transmission is complete, even if this data (dummy data) is not useful in our program.
2.4 Four Transmission Modes of SPI
There are rising edge, falling edge, leading edge, and trailing edge triggers. There are also MSB and LSB transmission methods.
2.5 Only Master and Slave Modes Exist
There is no distinction between reading and writing, as each SPI transaction involves the master and slave devices exchanging data. In other words, sending data will always result in receiving data; to receive data, data must also be sent first.
3 Operating Mechanism
3.1 Overview
The above image provides a simple description of communication between SPI devices. Below, we will explain the components shown in the image:
SSPBUF, Synchronous Serial Port Buffer, generally refers to the internal buffer of the SPI device, typically in FIFO form, storing temporary data during transmission;
SSPSR, Synchronous Serial Port Register, refers to the shift register inside the SPI device, which shifts data in or out of SSPBUF based on the configured data bit width;
Controller refers to the control register inside the SPI device, which can be configured to set the transmission mode of the SPI bus.Typically, we only need to program the four pins described in the image to control data communication between SPI devices:
SCK, Serial Clock, primarily transmits clock signals from the master device to the slave device, controlling the timing and rate of data exchange;
SS/CS, Slave Select/Chip Select, is used by the master device to select the slave device, allowing the selected slave device to be accessed by the master;
SDO/MOSI, Serial Data Output/Master Out Slave In, also known as the Tx-Channel on the master, serves as the data output, primarily for sending data from the SPI device;
SDI/MISO, Serial Data Input/Master In Slave Out, also known as the Rx-Channel on the master, serves as the data input, primarily for receiving data from the SPI device;
During communication, a data loop is formed between the master and slave devices, as illustrated in the image, with data being shifted in and out of SSPBUF under the control of SSPSR, and the controller determining the communication mode of the SPI bus, while SCK transmits the clock signal.
3.2 Timing
The above image illustrates the working mechanism of the SPI protocol by exchanging 1 Byte of data between the master and slave devices.
First, let’s explain the concepts of phase and polarity.
3.2.1 SPI Related Abbreviations
The polarity and phase of SPI, commonly written as CPOL and CPHA, can also be expressed in other ways. Here’s a brief summary:
(1) CKPOL (Clock Polarity) = CPOL = POL = Polarity = Clock Polarity
(2) CKPHA (Clock Phase) = CPHA = PHA = Phase = Clock Phase
(4) Edge = the moment of clock level change, either rising edge or falling edge
Within a clock cycle, there are two edges: Leading edge = the first edge, which corresponds to the transition from 1 to 0, or from 0 to 1; Trailing edge = the second edge, which occurs after the first edge transition.
3.2.2 Phase and Polarity of SPI
CPOL and CPHA can each be 0 or 1, resulting in four combinations:
3.2.3 CPOL Polarity
First, let’s discuss the idle state of the SCLK clock, which is the state before and after sending 8 bits of data. The corresponding state when SCLK is active is when it is in its normal working state.
In English, this is succinctly explained as: Clock Polarity = IDLE state of SCK. In more detail:
CPOL indicates whether the idle level of SCLK is low (0) or high (1):
If CPOL=0, the idle level is low, thus when SCLK is active, it is high, referred to as active-high;
If CPOL=1, the idle level is high, thus when SCLK is active, it is low, referred to as active-low;
3.2.4 CPHA Phase
To clarify, capture strobe = latch = read = sample, all refer to data sampling, the moment when data is valid. The phase corresponds to which edge (the first or second edge) the data is sampled. 0 corresponds to the first edge, and 1 corresponds to the second edge.
If CPHA=0, it indicates the first edge:
For CPOL=0, the idle state is low, so the first edge is the transition from low to high, which is the rising edge;
For CPOL=1, the idle state is high, so the first edge is the transition from high to low, which is the falling edge;
If CPHA=1, it indicates the second edge:
For CPOL=0, the idle state is low, so the second edge is the transition from high to low, which is the falling edge;
For CPOL=1, the idle state is high, so the second edge is the transition from low to high, which is the rising edge; the above image should make this clearer.
3.2.5 How to Set SPI Polarity and Phase in Software
SPI consists of master and slave devices that communicate via the SPI protocol.
Setting the SPI mode of the slave device determines the mode of the master device.
Therefore, it is necessary to understand the SPI mode of the slave device before configuring the master device’s SPI mode to match the slave device for proper communication.
For the slave device’s SPI mode, there are two cases:
3.2.5.1 Fixed Mode, Determined by SPI Slave Hardware
For the SPI slave device, the specific mode is described in the relevant datasheet, which must be consulted to find the details, such as:
Regarding the SPI slave device, whether it is high or low when idle determines whether CPOL is 0 or 1;
Then find out whether the device samples data on the rising or falling edge, thus determining CPHA based on the established value of CPOL.
3.2.5.2 Configurable, Set by Software
The slave device also acts as an SPI controller, supporting all four modes, allowing for configuration to any desired mode.
Once the mode of the slave device is known, the SPI master device’s mode should be set to match that of the slave device for proper communication.
Configuring CPOL and CPHA is straightforward, typically involving writing to the corresponding bits of the SPI controller’s registers.
SSPSR is the shift register inside the SPI device. Its main function is to move data in or out of SSPBUF based on the state of the SPI clock signal, with the amount of data moved determined by Bus-Width and Channel-Width.
Bus-Width specifies the unit of data transfer from the address bus to the master device.
For example, if we want to write 16 Bytes of data to the SSPBUF of the master device: First, set the Bus-Width of the master’s configuration register to Byte; then write 1 Byte of data to the Tx-Data shift register at the address bus; after writing 1 Byte, the Tx-Data shift register of the master will automatically move the 1 Byte of data into SSPBUF. This action needs to be repeated 16 times.
Channel-Width specifies the unit of data transfer between the master and slave devices. Similar to Bus-Width, the master’s internal shift register will automatically shift data from Master-SSPBUF to Slave-SDI based on Channel-Width, while Slave-SSPSR moves the received data into Slave-SSPBUF. Typically, Bus-Width will always be greater than or equal to Channel-Width to ensure that the data exchange frequency between master and slave does not exceed that between the address bus and master, preventing invalid data from being stored in SSPBUF.
3.4 SSPBUF
It is known that the data exchanged between the master and slave during each clock cycle is actually copied from the internal shift register of the SPI from SSPBUF. We can indirectly manipulate the internal SSPBUF of the SPI by reading and writing data to the Tx-Data / Rx-Data registers corresponding to SSPBUF.
For instance, before sending data, we should first write the data to be sent into the Tx-Data register of the master; this data will be automatically moved into Master-SSPBUF by the Master-SSPSR based on Bus-Width, and then this data will be shifted out from Master-SSPBUF according to Channel-Width through the Master-SDO pin to the Slave-SDI pin, while Slave-SSPSR moves the received data into Slave-SSPBUF. Meanwhile, the data in Slave-SSPBUF is sent to Master-SDI via Slave-SDO, and Master-SSPSR moves the received data into Master-SSPBUF. After a single data transfer is complete, the user program can read the exchanged data from the Rx-Data register of the master device.
3.5 Controller
The controller inside the master device primarily controls the slave device through clock signals and slave select signals. The slave device will continuously wait until it receives the slave select signal from the master, after which it will operate based on the clock signal.
The slave select operation of the master device must be implemented by the program. For example: the program pulls the clock signal low on the SS/CS pin to complete the preliminary work for SPI data communication; when the program wants to end data communication, it pulls the clock signal high on the SS/CS pin.
4 Example of SPI
After discussing so much, let me provide an example to help everyone understand.
SPI is a ring bus structure consisting of SS (CS), SCK, SDI, and SDO, with timing being quite simple, primarily controlled by SCK, allowing two bidirectional shift registers to exchange data.
Assuming the following 8-bit register contains the data to be sent 10101010, sending occurs on the rising edge, receiving on the falling edge, and high bits are sent first.
When the first rising edge occurs, the data will be SDO=1; the register will be 0101010x. When the falling edge arrives, the level on SDI will be stored in the register, making it register=0101010sdi. After 8 clock pulses, the contents of the two registers will have exchanged once. This completes one SPI timing cycle.
For example: assuming the master and slave are initialized and ready, with the master’s sbuff=0xaa and the slave’s sbuff=0x55, below will demonstrate the data situation over the 8 clock cycles of SPI step by step: assuming data is sent on the rising edge.
This completes the 8-bit exchange of the two registers, where the upper indicates the rising edge and the lower indicates the falling edge, with SDI and SDO relative to the master. This is very close to understanding; the next step is to animate the above process.
Source: CSDN, Hardware Questions | Cover Image Source: Internet
-end-
Sharing is not easy; please give a thumbs up and a look!