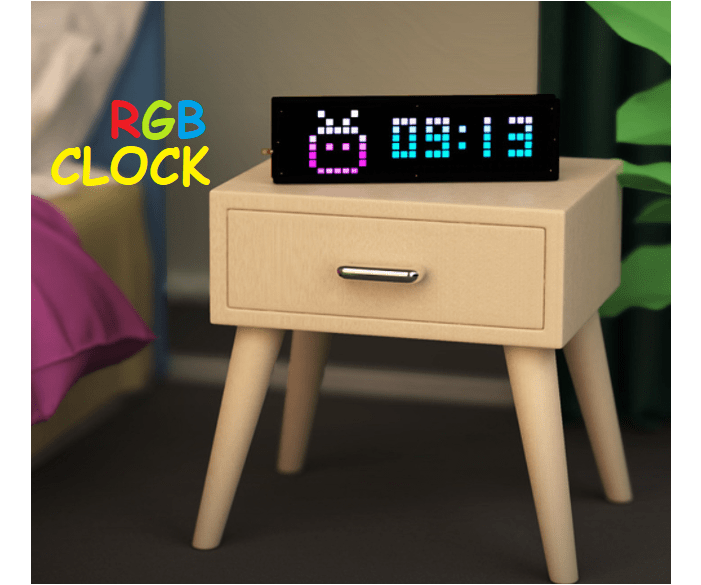
Sharing a project to create a stylish RGB clock using ESP8266!
This clock also features automatic brightness control and is equipped with a temperature sensor!
Materials Preparation
-
PCB -
ESP8266 or NodeMCU -
Jumper wires -
Soldering tools
Step 1: Preparation
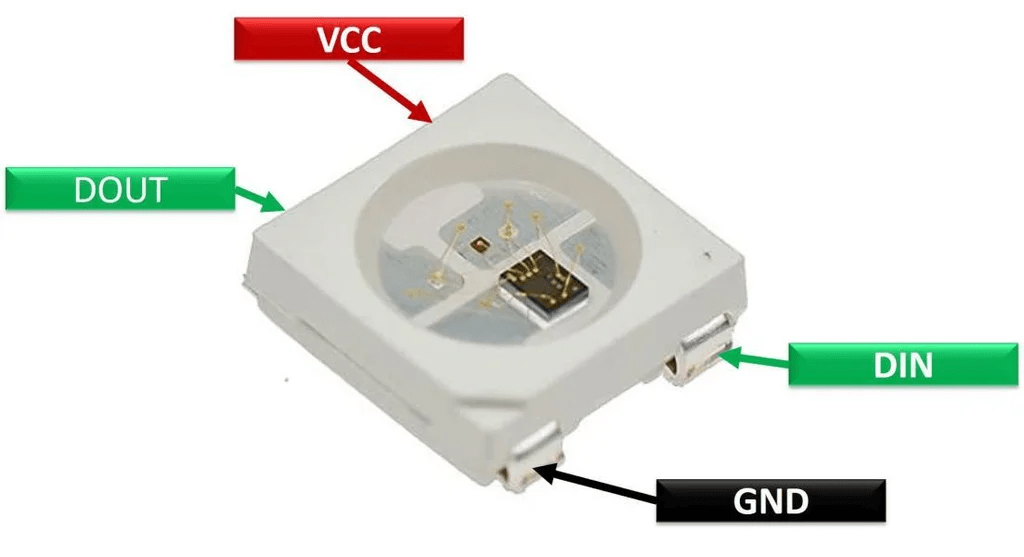
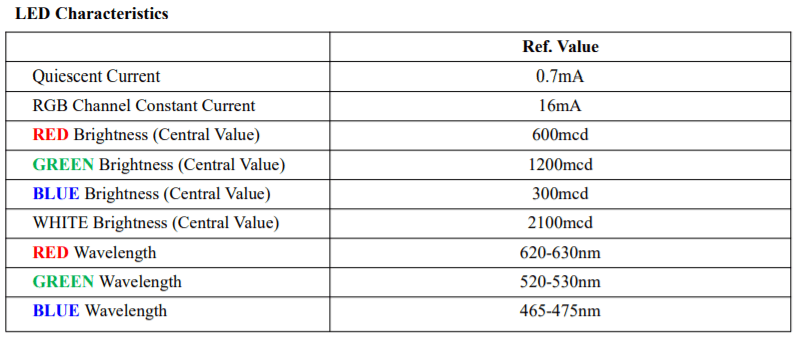
Neo Pixel is an addressable LED, we can program it to display any color or number.
Neo Pixel comes in different SMD packages, this project uses Ws2812b-5050 mini RGB.
This mini LED has a rated voltage of 3.0V to 5.5V and a current of 16mA (per LED).
NodeMCU has a 3.3V regulator that can drive all the LEDs properly.
Step 2: Create a 7-segment display using Neo Pixel LED
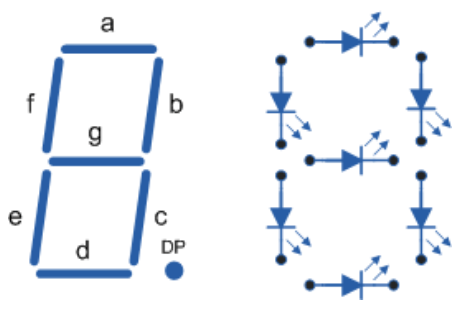
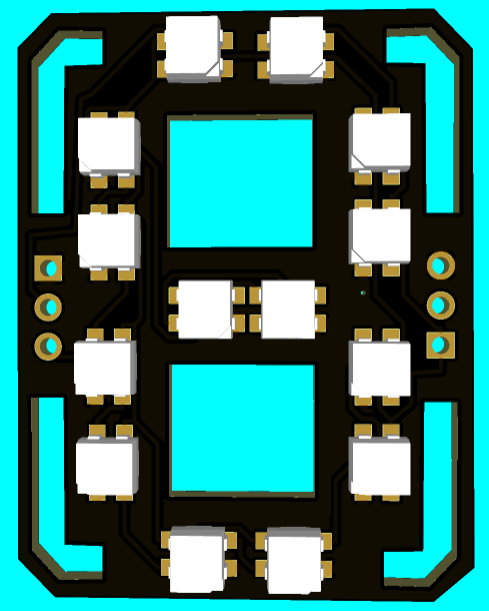
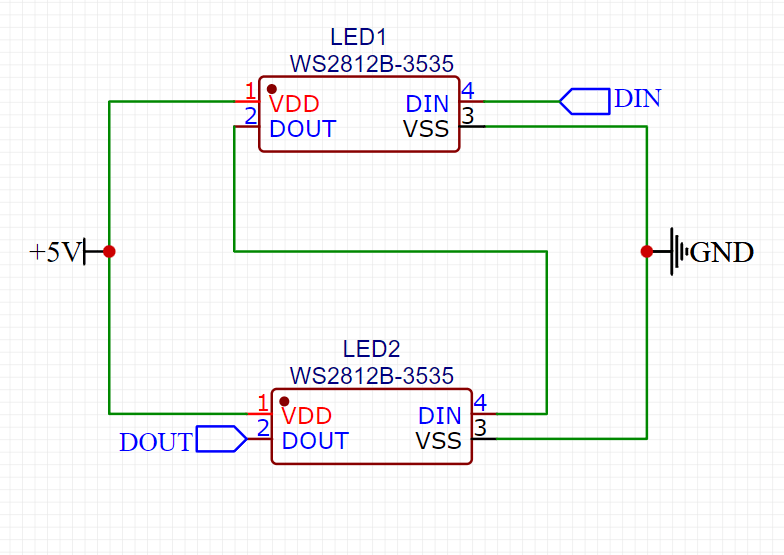
Here, we need to parallel all the power connections and connect all the data in series, using the 7-segment display method, as shown in the image, to connect all the LEDs.
Each segment has 2 LEDs, and the entire panel has a total of 14 LEDs.
We need 4 panels to display time (2 for hours, 2 for minutes).
Of course, we can connect two more panels to display seconds/any other value, or temperature.
No matter how you connect them, remember to always connect the first panel’sDOUT
to the second panel’sDIN
.
Step 3: Connect the Dashboard
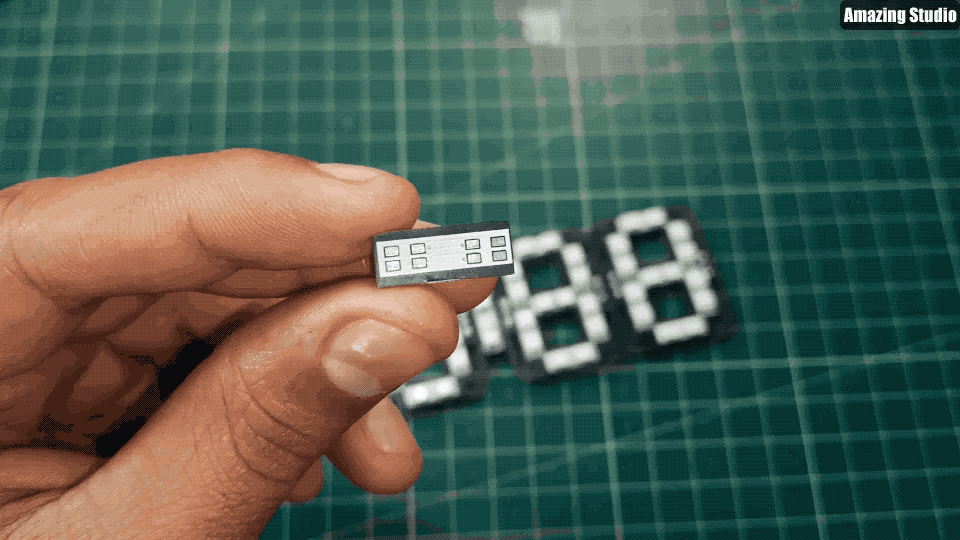
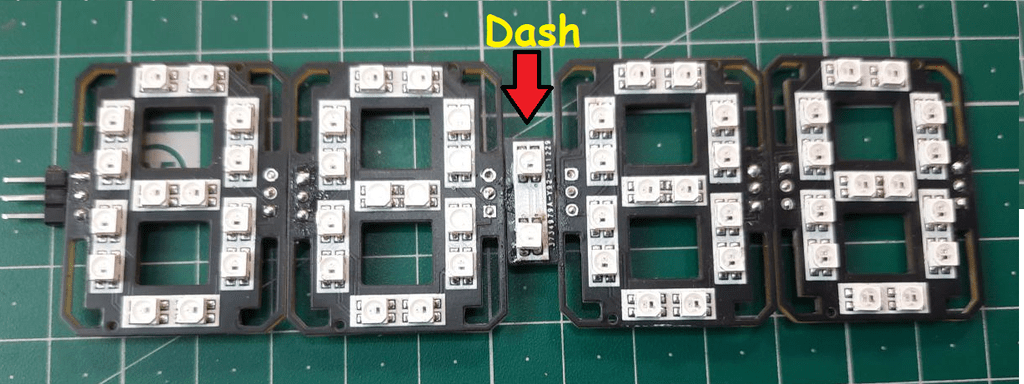
To connect the hour and minute panels, there is a small PCB board between the two panels calledDash
, which contains 2 LEDs as binary digits that will light up once every second.
Step 4: Introduction to NodeMCU/ESP8266
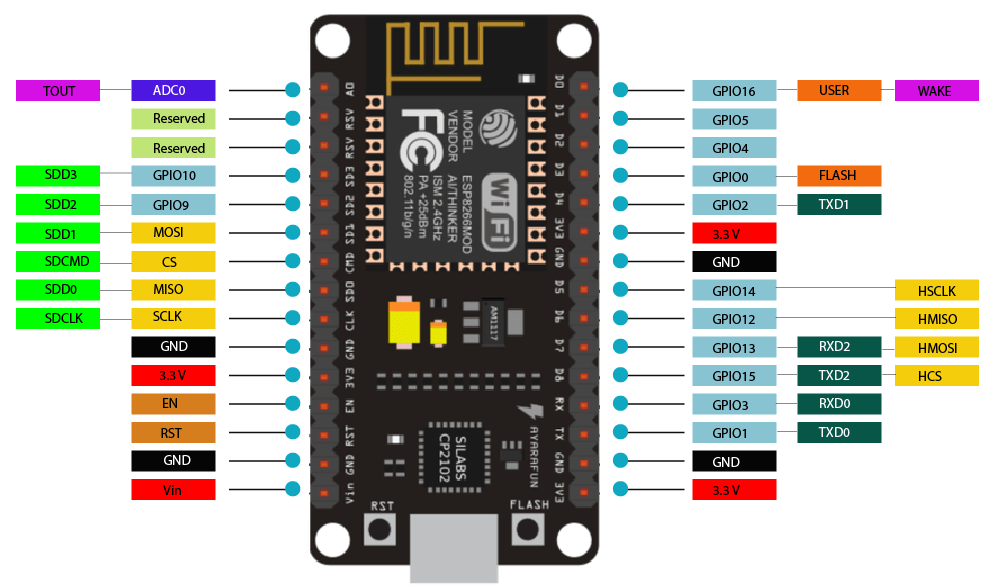
ESP8266 integrates a 32-bit Tensilica processor and standard digital peripheral interfaces.
Our ESP8266 has onboard Wi-Fi support, allowing us to connect to the internet to adjust the time without any RTC (real-time clock) modules.
This can reduce connections, making the whole project simpler.
Step 5: Features Supported in the Code
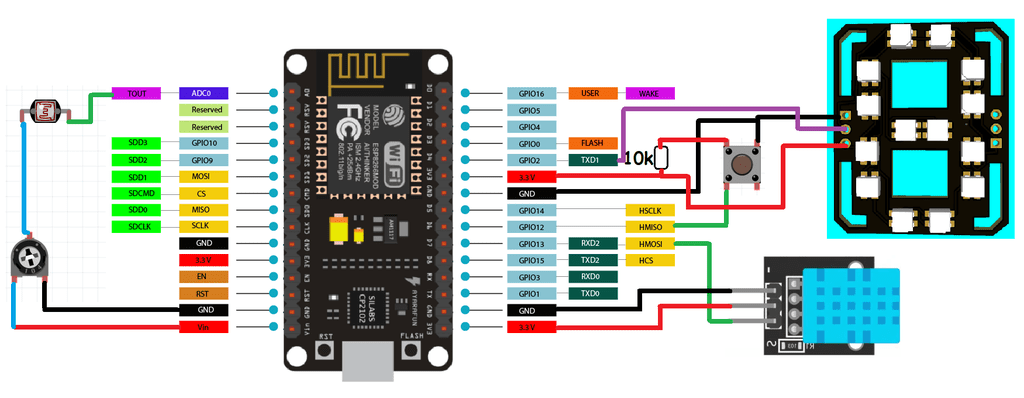
If you use the code provided in this article, we can add 2 additional features to this 7-segment clock:
-
Temperature and Humidity Display Using a Tactile Switch
Add a DHT11 sensor to pin 13, and a tactile button to pin 12, allowing you to obtain temperature readings in Celsius or Fahrenheit on the screen.
Connect one end of a 10k resistor from pin 12 of the button to 5V, and the other end to GND. This means when the button pin is pulled to GND, the display will show the temperature reading. If you do not have this temperature sensor, the code will still work, so if you want to simplify the project, you can skip these connections.
-
Brightness Control Using LDR Sensor on Pin A0
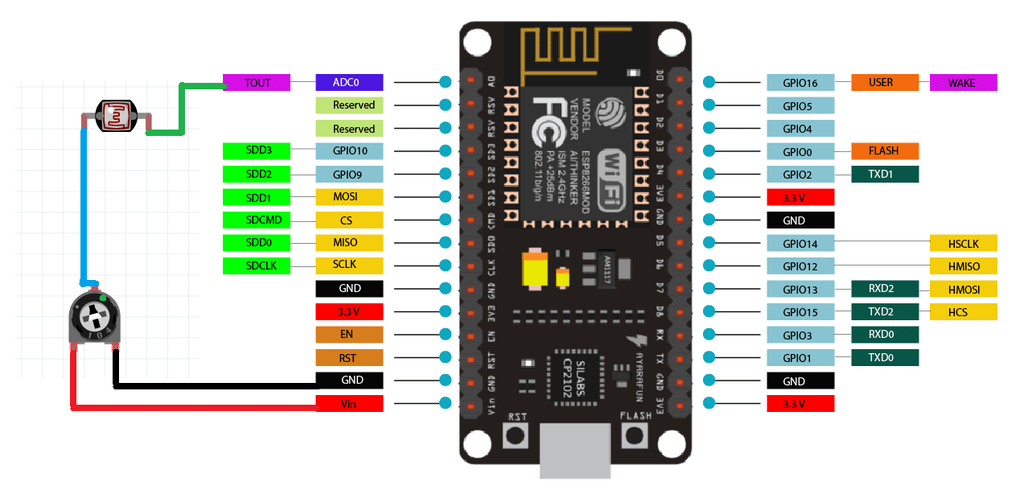
By creating a resistor divider network on pin A0, adding an LDR sensor with a 10k resistor can adjust the brightness accordingly.
High brightness during the day and low brightness at night. If you do not want adjustable brightness, this part of the code can also work without these sensors, locking at the default setting.
Step 6: Video Demonstration
Step 7: 7-Segment Clock
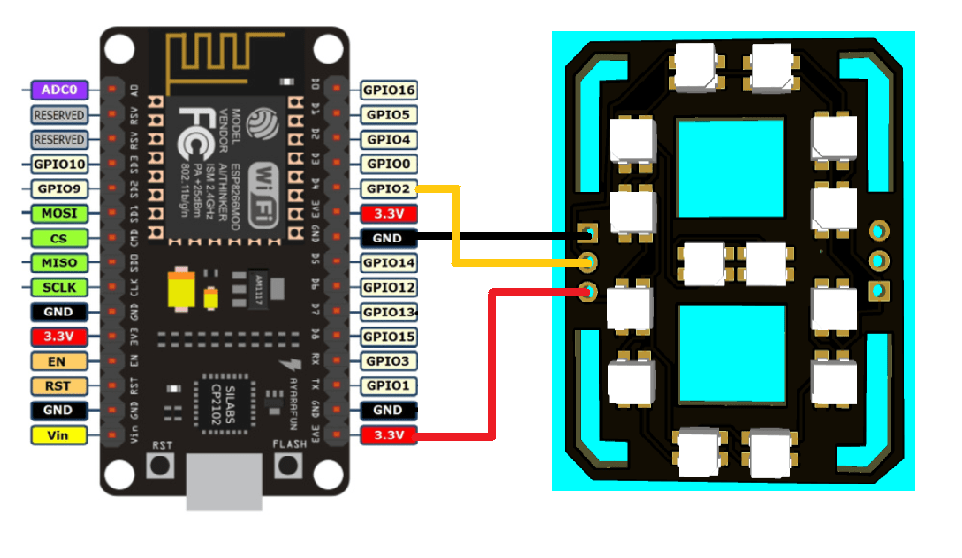
Now, we have 4 panels and a Dash (dashboard).
Connect the panels and dashboard according to the above GIF; connect 2 panels in series.
Then connect the NodeMCU using the schematic provided above.
Step 8: Code
-
First, use libraries to initialize the code:
#include <ESP8266WiFi.h>
#include <Adafruit_NeoPixel.h>
#include <WiFiUdp.h>
#include <NTPClient.h>
#include <TimeLib.h>
#include <DHT.h>
#include <Adafruit_Sensor.h>
-
Define all pixels, I/O pins, and sensor pins:
#define PIXEL_PER_SEGMENT 2 // Number of LEDs in each Segment
#define PIXEL_DIGITS 4 // Number of connected Digits
#define PIXEL_PIN 2 // GPIO Pin
#define PIXEL_DASH 1 // Binary segment
#define LDR_PIN A0 // LDR pin
#define DHT_PIN 13 // DHT Sensor pin
#define BUTTON_PIN 12 // Button pin
3. For time format, connect the ESP8266 to the internet via Wi-Fi:
WiFi.begin(ssid, password);
Serial.print("Connecting.");
while ( WiFi.status() != WL_CONNECTED )
-
Time setting:
void disp_Time() {
clearDisplay();
writeDigit(0, Hour / 10);
writeDigit(1, Hour % 10);
writeDigit(2, Minute / 10);
writeDigit(3, Minute % 10);
writeDigit(4, Second / 10);
writeDigit(5, Second % 10);
disp_Dash();
5. Color settings on the panel:
if (index == 0 || index == 1 ) color = strip.Color(0, Brightness, 0);
if (index == 2 || index == 3 ) color = strip.Color(0, Brightness, 0);
if (index == 4 || index == 5 ) color = strip.Color(Brightness, 0, 0);
This is just a simple introduction, and the code also includes temperature and automatic time options.
The temperature mode can be selected using the switch on digital pin 12.
Step 9: Complete Code
#include <ESP8266WiFi.h>
#include <Adafruit_NeoPixel.h>
#include <WiFiUdp.h>
#include <NTPClient.h>
#include <TimeLib.h>
#include <DHT.h>
#include <Adafruit_Sensor.h>
#define PIXEL_PER_SEGMENT 2 // Number of LEDs in each Segment
#define PIXEL_DIGITS 4 // Number of connected Digits
#define PIXEL_PIN 2 // GPIO Pin
#define PIXEL_DASH 1 // Binary segment
#define LDR_PIN A0 // LDR pin
#define DHT_PIN 13 // DHT Sensor pin
#define BUTTON_PIN 12 // Button pin
// Uncomment the type of sensor in use
#define DHT_TYPE DHT11 // DHT 11
//#define DHT_TYPE DHT22 // DHT 22 (AM2302)
//#define DHT_TYPE DHT21 // DHT 21 (AM2301)
#define TIME_FORMAT 12 // 12 = 12 hours format || 24 = 24 hours format
Adafruit_NeoPixel strip = Adafruit_NeoPixel((PIXEL_PER_SEGMENT * 7 * PIXEL_DIGITS) + (PIXEL_DASH * 2), PIXEL_PIN, NEO_GRB + NEO_KHZ800);
DHT dht(DHT_PIN, DHT_TYPE);
// set Wi-Fi SSID and password
const char *ssid = "Hackster";
const char *password = "Sainisagar7294";
WiFiUDP ntpUDP;
// 'time.nist.gov' is used (default server) with +1 hour offset (3600 seconds) 60 seconds (60000 milliseconds) update interval
NTPClient timeClient(ntpUDP, "time.nist.gov", 19800, 60000); //GMT+5:30 : 5*3600+30*60=19800
int period = 2000; //Update frequency
unsigned long time_now = 0;
int Second, Minute, Hour;
// set default brightness
int Brightness = 40;
// current temperature, updated in loop()
int Temperature;
bool Show_Temp = false;
//Digits array
byte digits[12] = {
//abcdefg
0b1111110, // 0
0b0110000, // 1
0b1101101, // 2
0b1111001, // 3
0b0110011, // 4
0b1011011, // 5
0b1011111, // 6
0b1110000, // 7
0b1111111, // 8
0b1110011, // 9
0b1001110, // C
0b1000111, // F
};
//Clear all the Pixels
void clearDisplay() {
for (int i = 0; i < strip.numPixels(); i++) {
strip.setPixelColor(i, strip.Color(0, 0, 0));
}
strip.show();
}
void setup() {
Serial.begin(115200);
strip.begin();
strip.show();
dht.begin();
pinMode(BUTTON_PIN, INPUT);
WiFi.begin(ssid, password);
Serial.print("Connecting.");
while ( WiFi.status() != WL_CONNECTED ) {
delay(500);
Serial.print(".");
}
Serial.println("connected");
timeClient.begin();
delay(10);
}
void loop() {
if (WiFi.status() == WL_CONNECTED) { // check WiFi connection status
int sensor_val = analogRead(LDR_PIN);
Brightness =40;
timeClient.update();
int Hours;
unsigned long unix_epoch = timeClient.getEpochTime(); // get UNIX Epoch time
Second = second(unix_epoch); // get seconds
Minute = minute(unix_epoch); // get minutes
Hours = hour(unix_epoch); // get hours
if (TIME_FORMAT == 12) {
if (Hours > 12) {
Hour = Hours - 12;
}
else
Hour = Hours;
}
else
Hour = Hours;
}
if (digitalRead(BUTTON_PIN) == LOW) {
Show_Temp = true;
}
else
Show_Temp = false;
if (Show_Temp) {
Temperature = dht.readTemperature();
Serial.println(Temperature);
clearDisplay();
writeDigit(0, Temperature / 10);
writeDigit(1, Temperature % 10);
writeDigit(2, 10);
strip.setPixelColor(28, strip.Color(Brightness, Brightness, Brightness));
strip.show();
delay(3000);
clearDisplay();
Show_Temp = false;
}
while (millis() > time_now + period) {
time_now = millis();
disp_Time(); // Show Time
}
}
void disp_Time() {
clearDisplay();
writeDigit(0, Hour / 10);
writeDigit(1, Hour % 10);
writeDigit(2, Minute / 10);
writeDigit(3, Minute % 10);
writeDigit(4, Second / 10);
writeDigit(5, Second % 10);
disp_Dash();
strip.show();
}
void disp_Dash() {
int dot, dash;
for (int i = 0; i < 2; i++) {
dot = 2 * (PIXEL_PER_SEGMENT * 7) + i;
for (int j = 0; j < PIXEL_DASH; j++) {
dash = dot + j * (2 * (PIXEL_PER_SEGMENT * 7) + 2);
Second % 2 == 0 ? strip.setPixelColor(dash, strip.Color(0,Brightness ,0)) : strip.setPixelColor(dash, strip.Color(0, Brightness,0));
}
}
}
void writeDigit(int index, int val) {
byte digit = digits[val];
int margin;
if (index == 0 || index == 1 ) margin = 0;
if (index == 2 || index == 3 ) margin = 1;
if (index == 4 || index == 5 ) margin = 2;
for (int i = 6; i >= 0; i--) {
int offset = index * (PIXEL_PER_SEGMENT * 7) + i * PIXEL_PER_SEGMENT + margin * 2;
uint32_t color;
if (digit & 0x01 != 0) {
if (index == 0 || index == 1 ) color = strip.Color(Brightness, 0, Brightness);
if (index == 2 || index == 3 ) color = strip.Color(Brightness, 0,Brightness);
if (index == 4 || index == 5 ) color = strip.Color(Brightness, 0, 0);
}
else
color = strip.Color(0, 0, 0);
for (int j = offset; j < offset + PIXEL_PER_SEGMENT; j++) {
strip.setPixelColor(j, color);
}
digit = digit >>> 1;
}
}
Step 10: Complete Circuit Diagram (HD version available for download at the end)
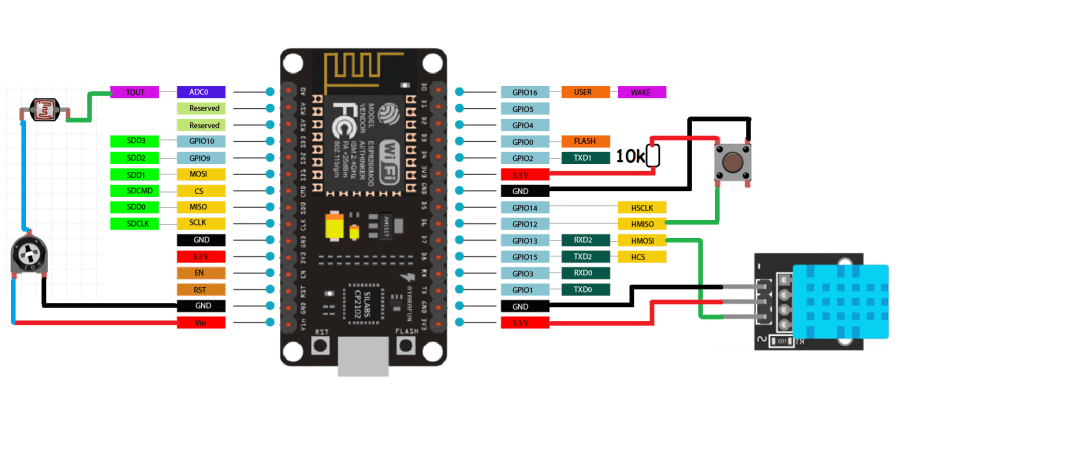
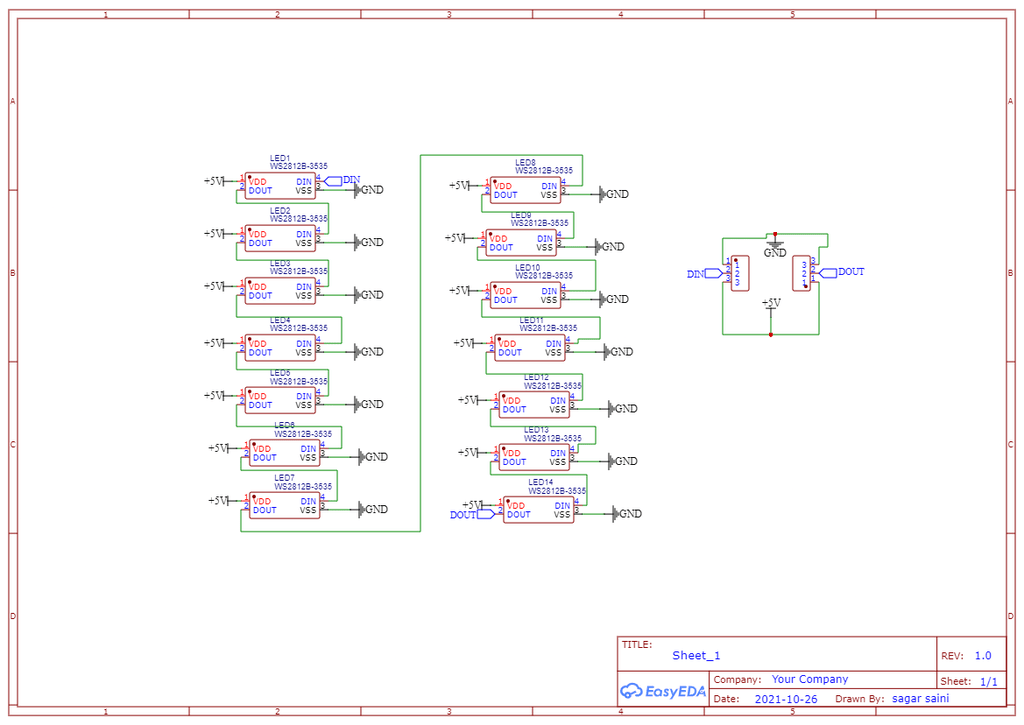
Step 11: PCB Design (Panel Part)
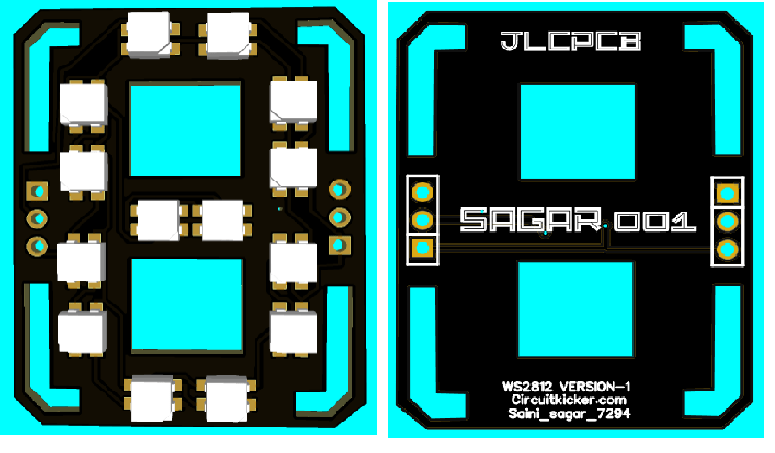
Main PCB design for displaying numbers and letters.
ClickRead the original text to download the source files.
Step 12: PCB Design (Dash Part)
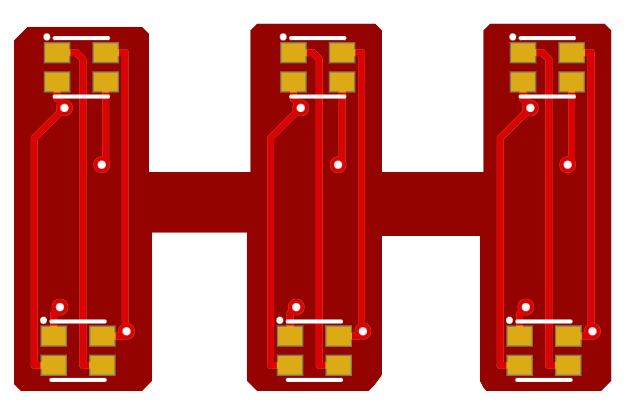
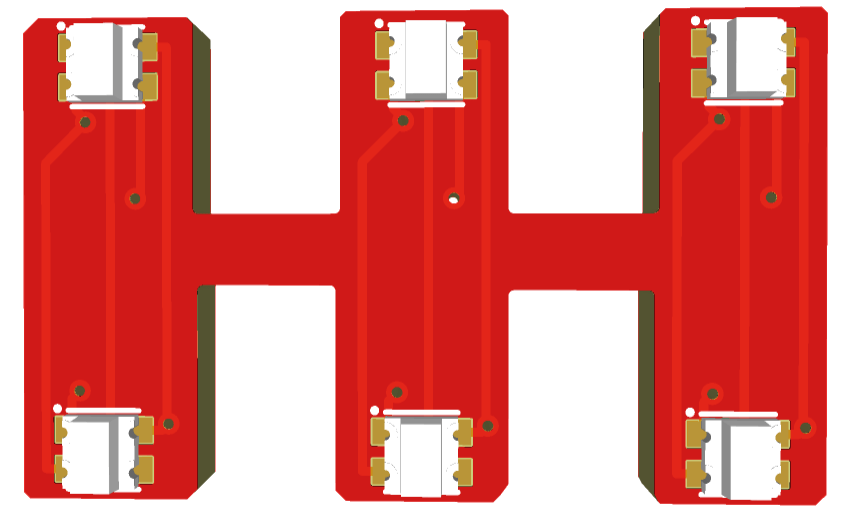
Step 13: Troubleshooting
-
DIN
should always be connected in series withDOUT
; if reversed or disconnected from anywhere, the entire device will stop working; -
Connect the Dash according to the above image;
-
Ensure all connections are well soldered; dry soldering can cause data values and colors to change;
-
When soldering, do not heat the PCB too much, keeping the temperature around 300 degrees.
Step 14: Complete Display
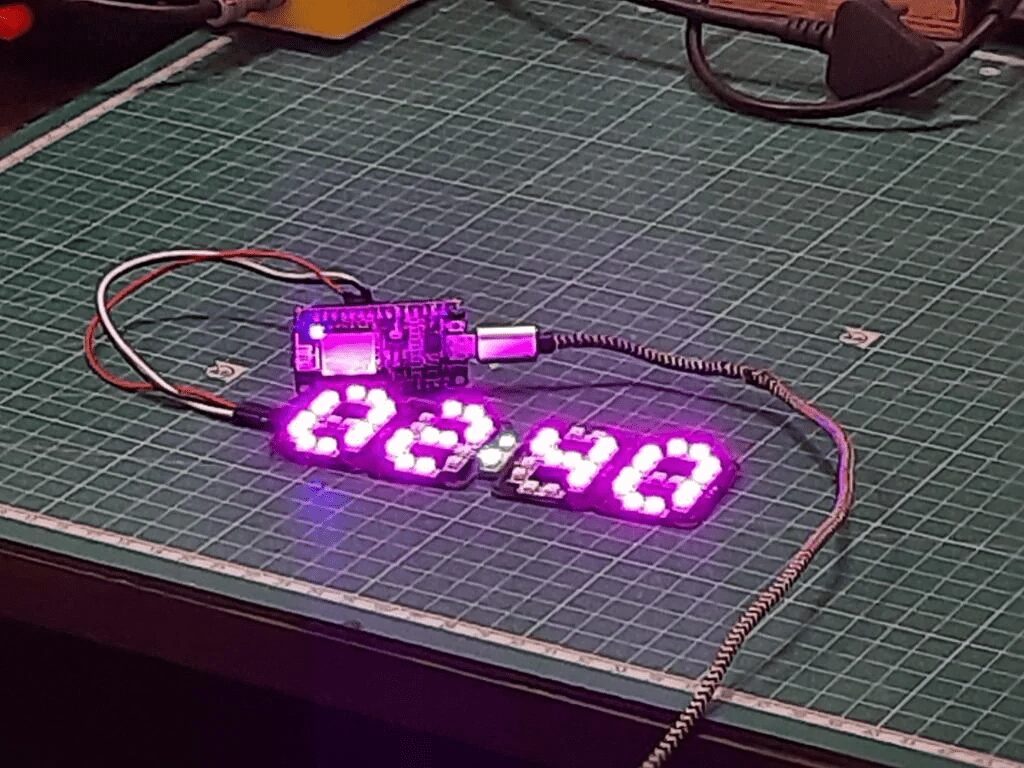
Hope everyone enjoys this project!
-END-
Previous recommendations: Click the image to jump to read
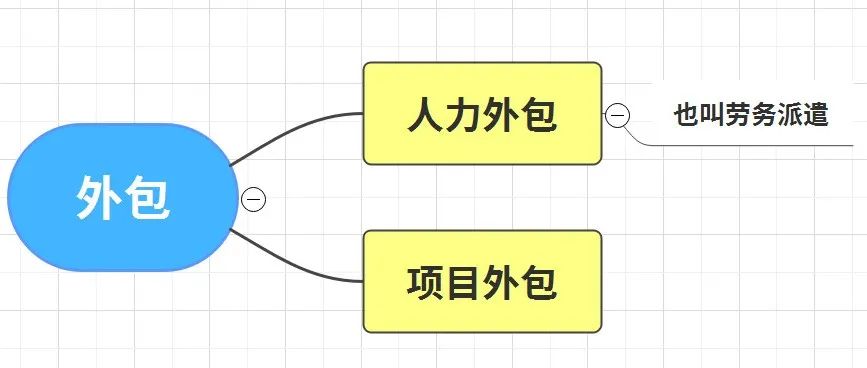
What is an outsourcing company? Should I go to an outsourcing company?
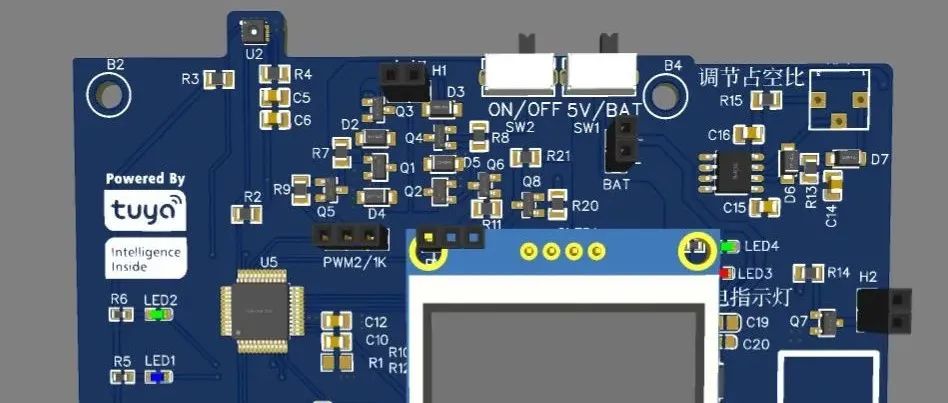
Comparison of STM32 and Arduino, who is more powerful?
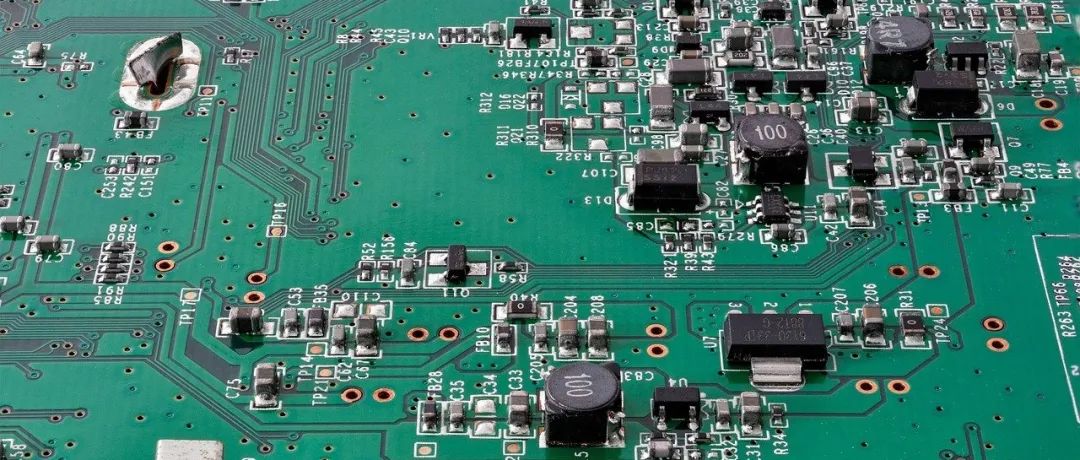
Summary | From Huawei’s regularization to resignation
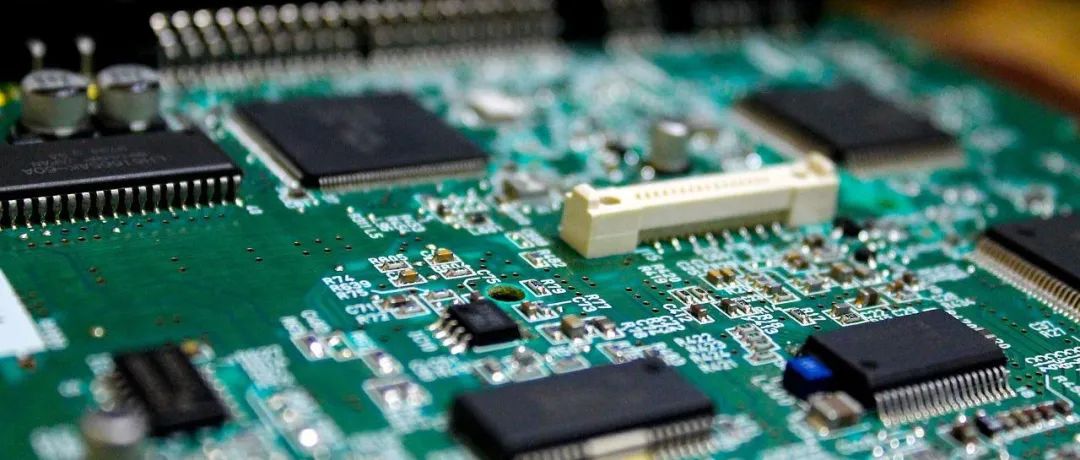
What I saw and gained in five months at Huawei!