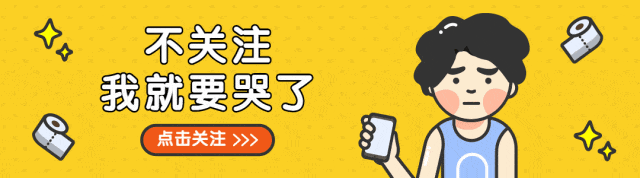
This article is based on a thorough review of authoritative literature and materials, forming professional and reliable content. All data in the full text can be referenced and traced back. Special note: Data and materials have been authorized. This article does not involve any biased views and describes the facts objectively with a neutral attitude.
At the end of the article, there are the latest trending articles; if interested, you can take a look.
The article is a bit long (3084 words, reading time: 8 minutes), and I hope you can persevere to the end and gain something.
Background
This document provides a detailed introduction to a minimal real-time operating system (RTOS) kernel implemented in C, aiming to illustrate basic concepts such as task management, scheduling, and context switching in a real-time environment. This example can serve as a teaching tool for understanding how an RTOS kernel operates and can be extended for more complex application scenarios.
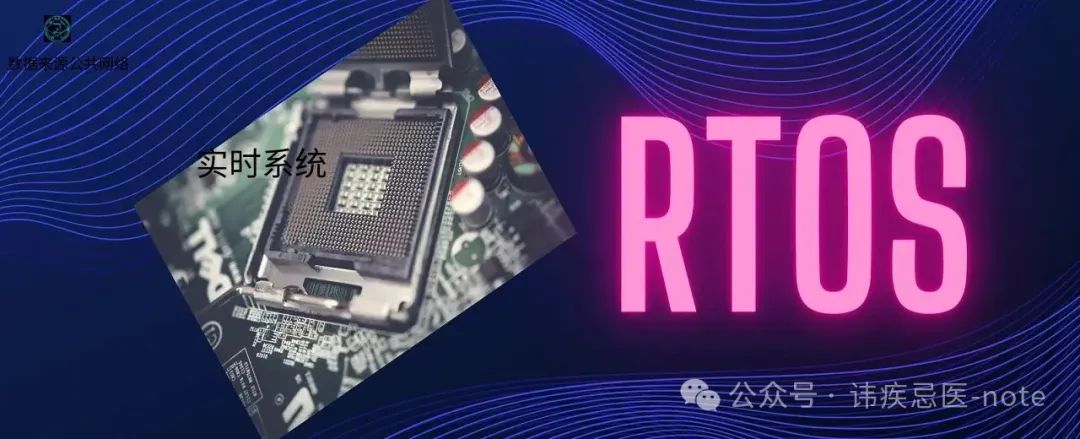
What is a Real-Time Operating System (RTOS)?
A real-time operating system (RTOS) is designed to manage time-sensitive tasks that must strictly adhere to deadlines for execution. Unlike general-purpose operating systems that focus on flexibility and multitasking, RTOS ensures timely execution of critical operations, making it very suitable for applications in embedded systems, robotics, and industrial automation.
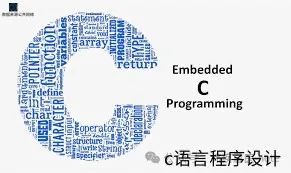
The provided code implements a basic RTOS with the following key components:
#include <stdint.h>
#include <stdbool.h>
#include <stdlib.h>
#define MAX_TASKS 5 // Maximum number of tasks
#define STACK_SIZE 256 // Size of each task stack
// Define task structure
typedef struct {
uint32_t* stackPointer; // Pointer to the task stack
uint32_t* stackBase; // Base address of the task stack
} Task;
static Task tasks[MAX_TASKS]; // Array of tasks
static uint8_t currentTask = 0; // Index of the current task
static uint8_t taskCount = 0; // Number of current tasks
// Function prototypes
void scheduler(void);
void switch_context(void);
// Initialize a task
bool create_task(void (*task_func)(void)) {
if (taskCount >= MAX_TASKS) return false; // Check if maximum task limit exceeded
// Allocate stack space for the new task
tasks[taskCount].stackBase = (uint32_t*)malloc(STACK_SIZE * sizeof(uint32_t));
tasks[taskCount].stackPointer = tasks[taskCount].stackBase + STACK_SIZE / sizeof(uint32_t) - 1;
// Set initial stack values for the new task
*(tasks[taskCount].stackPointer) = (uint32_t)(task_func); // Program Counter (PC)
*(--tasks[taskCount].stackPointer) = 0xFFFFFFF0; // xPSR (default value)
taskCount++; // Increment current task count
return true;
}
// Simple round-robin scheduler
void scheduler(void) {
currentTask++; // Switch to the next task
if (currentTask >= taskCount) {
currentTask = 0; // Reset to 0 if exceeding maximum count
}
}
// Context switching function
void switch_context(void) {
__asm volatile(
"PUSH {R0-R3, R12} \n" // Save registers
"LDR R0, =tasks \n" // Load address of task array
"LDR R1, =currentTask \n" // Load current task index
"LDR R2, [R1] \n" // Load current task index value
"LDR R3, [R0, R2, LSL #3] \n" // Load current task structure
"STR SP, [R3] \n" // Save current stack pointer
"BL scheduler \n" // Call scheduler
"LDR R2, [R1] \n" // Load new current task index value
"LDR R3, [R0, R2, LSL #3] \n" // Load new task structure
"LDR SP, [R3] \n" // Restore new stack pointer
"POP {R0-R3, R12} \n" // Restore registers
"BX LR \n" // Return from interrupt/exception
);
}
// Example task 1
void task1(void) {
while (1) {
// Code for task 1...
switch_context(); // Switch context to the next task
}
}
// Example task 2
void task2(void) {
while (1) {
// Code for task 2...
switch_context(); // Switch context to the next task
}
}
// Main function to start RTOS
int main(void) {
create_task(task1); // Create the first task
create_task(task2); // Create the second task
while (1) {
switch_context(); // Start scheduling process, switch context
}
return 0;
}
Key functions implemented:
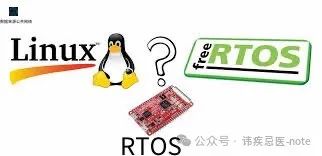
– The Task structure defines the representation of each task in the system, containing pointers to the stack being used by the task.
– The create task function allocates memory for the new task’s stack and initializes it using the starting address of the task function. This ensures that the number of tasks created does not exceed MAX_TASKS.
– The scheduler function implements a simple round-robin scheduling algorithm that cycles through all available tasks, incrementing the currentTask index and resetting to 0 when the maximum task count is reached.
– The switch context function uses inline assembly to save the state of the currently running task and restore the state of the next task, involving saving registers and manipulating the stack pointer.
– Example tasks: Two example tasks (task1 and task2) run in an infinite loop, yielding control back to the scheduler by calling the switch_context function.
What can be achieved with this implementation?
This minimal RTOS implementation allows you to:
1. Run multiple tasks: Concurrently execute multiple tasks in a round-robin manner.
2. Manage tasks flexibly, efficiently handling a maximum of five tasks.
3. Context switching: Gain insight into how context switching works at a low level through assembly language.
4. Serve as a foundation for further development: Build more complex real-time applications.
Real-world application cases
In practical projects, RTOS is widely used in embedded systems. For example, in medical devices, RTOS ensures that critical monitoring functions are completed within strict time constraints, thereby enhancing patient safety. In autonomous vehicles, RTOS is used to process sensor data and control algorithms to ensure that vehicles can react quickly at high speeds. This reliability and real-time performance are crucial for accident prevention.
According to a study by Beningo, a typical RTOS can maintain CPU load below 4% with less than 16KB of required flash memory and less than 4KB of RAM. This indicates that modern RTOS is highly efficient in terms of performance and resource usage. Furthermore, the study emphasizes that developers’ misunderstanding of RTOS configuration and usage often leads to performance issues, rather than issues with the RTOS itself.
Expansion opportunities:
This minimal real-time operating system can be expanded through the following ways:
New functional modules can be added to further enhance system performance; existing algorithms can be optimized to significantly improve operational efficiency; task scheduling mechanisms can be improved to make the system more responsive and stable. Additionally, introducing advanced debugging tools can help developers quickly identify and address potential issues; strengthening security measures can effectively prevent unauthorized access and malicious attacks. In summary, by implementing improvements in these areas, this real-time operating system will possess greater practicality and adaptability.
1. Inter-task communication: Implement mechanisms such as message queues or semaphores to allow different threads to communicate and synchronize with each other. This can be achieved by: Message queues: Allow one or more producer threads to send messages to the queue; meanwhile, consumer threads receive messages from the queue. Semaphores control the usage of shared resources, ensuring that only one thread can access a specific resource at any time.
2. Priority scheduling: Strengthen the scheduler to support scheduling at different priority levels. This can be achieved by: Assigning priorities to each created thread; modifying the scheduling algorithm to allow high-priority threads to preempt low-priority threads, ensuring timely execution of critical operations.
3. Timer management: Add timer functionality so that certain specific operations can be executed after a predetermined time. This can be achieved by setting timer callback functions, such as using hardware timers to generate interrupts and calling the corresponding callback function in the interrupt service routine.
4. Error handling mechanisms: Add handling mechanisms for error situations such as memory allocation failures or invalid operations to improve system stability. For example, checking the return value after each memory allocation, and taking appropriate actions if the return is NULL. Performance testing and data support: According to recent studies, modern RTOS typically has low context switch latency and scheduling latency. For example, some RTOS report context switch latencies below 10 microseconds, and scheduling latencies as low as 5 microseconds. These performance metrics are crucial for real-time applications that require high reliability.
Conclusion
This minimal RTOS kernel is an excellent starting point for understanding real-time operating systems and their core functionalities. By studying this code, readers can gain practical insights into task management and scheduling while identifying areas for expansion and optimization. As you continue to develop your own real-time operating system, consider adding additional functionalities to meet specific application needs or enhance performance. Through continuous optimization and expansion, you can provide stronger support for more complex applications and achieve higher efficiency.
References
-
FreeRTOS Documentation: FreeRTOS is an open-source real-time operating system kernel for embedded devices that provides multitasking and inter-task communication mechanisms. Real-Time Operating System Best Practices Guide: This document summarizes recommended practices for improving RTOS application performance and optimizing memory usage. -
Comprehensive Guide to RTOS and Related Concepts: This guide provides insights into RTOS architecture, performance optimization techniques, and kernel functions. -
What Is a Real-Time Operating System: Ultimate Guides overview of RTOS characteristics and architectural models. -
Optimization Techniques for Embedded Systems: Discusses advanced compiler optimizations and effective cache handling to enhance performance in embedded systems. Invite domain experts or experienced developers to review the content to ensure the accuracy and professionalism of the information. Meanwhile, citing authoritative literature can enhance the credibility of the content. Additionally, using illustrations, code comments, or additional explanations can improve accessibility for better understanding of complex concepts and technical details. Furthermore, timely updates to the content are necessary to keep the information consistent with the latest technological trends and standards.
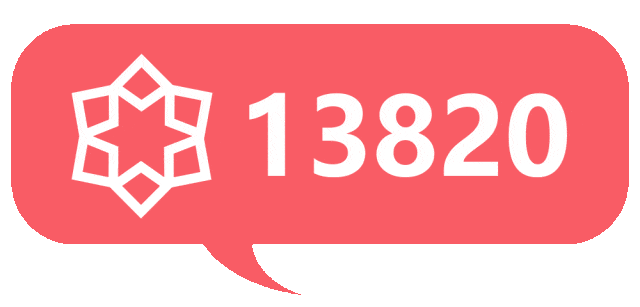
Latest trending articles recommended:
Pooling Layers in Deep Learning: Is Reducing Computation at the Cost of Classification Accuracy?
Can the Team of He Kaiming Challenge the Dominance of CNN? Will ViT Disrupt Traditional Object Detection?
Implementing Neural Networks in Pure C++: Training a Handwritten Digit Classifier with 99% Accuracy in 260 Lines of Code, Can You Believe It?
Reasons I Transitioned from VSCode to Cursor
Monitor Your Linux System with Just One Script!
Clean and Simple Implementation of Go Project Structure | GitHub 4.8k Stars
Why Do Developers Hate PHP? (The Best Language in the World)
Yoshua Bengio in the Eyes of Chinese People: Bringing AI Safety Concepts into Real Applications and Influencing Global Policy Making?
Why Are Developers Abandoning PostgreSQL, MySQL, and MongoDB?
Elon Musk and Other Big Names Question: Is the Path of AI Development Led by OpenAI Progress or Prelude to Disaster?
Foreign Programmers Share: C++ Crushes Rust in Low-Level Performance and Real-Time Thread Handling
Shocking! The Top Ten Legendary Figures in the AI Field in 2024, Leading Global Technological Transformation
Eight Rust Performance Tips Learned from Years of Rust Programming
Foreign Big Tech Programmers Share: Lessons Learned from Over 30 Failed Interviews
Don’t Blindly Solve Problems (LeetCode): Learn These 15 Patterns to Make Problem Solving Easy
Still Struggling with Code Deployment? Master These 25 Docker Commands to Make Everything Simple and Efficient!
Andrew Ng’s Path of Innovation: Each Breakthrough from Academia to Industry Has Completely Changed the Development Trajectory of an Industry
One Billion Lines of Data Challenge: CUDA Application Goes from 17 Minutes to 17 Seconds
One Billion Lines of Data Challenge: JAVA Application Goes from 71 Seconds to 1.7 Seconds through Gradual Optimization
One Billion Lines of Data Challenge: Rust Request Goes from 5 Minutes to 9 Seconds
One Billion Lines of Data Challenge: Python Application
One Billion Lines of Data Challenge: Go Application Goes from 15 Minutes to 5 Seconds
One Billion Lines of Data Challenge: How Does C++ Efficiently Process Massive Data?