

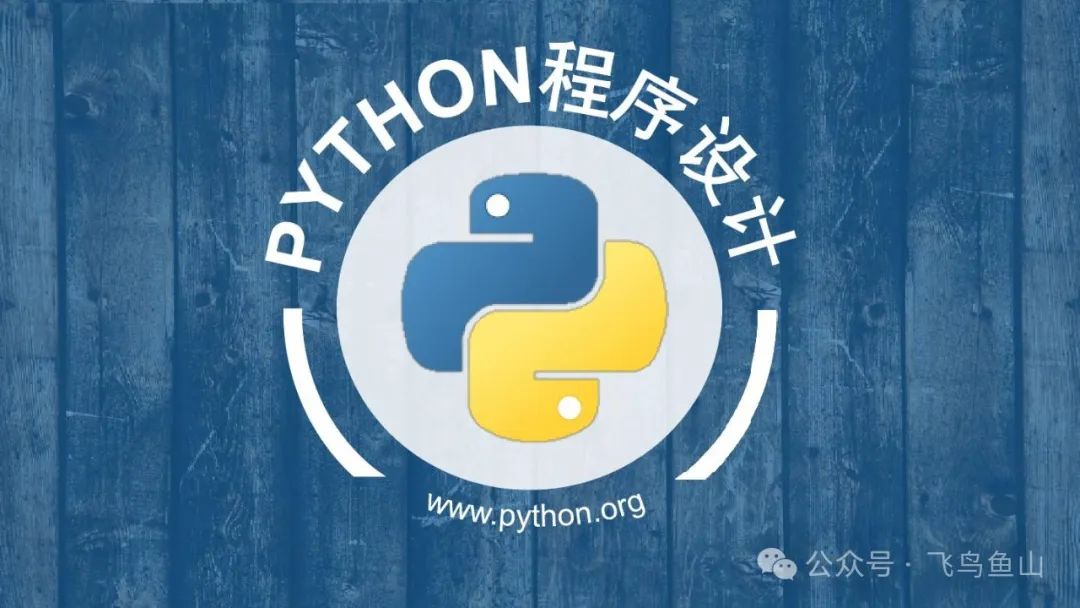
Do you have an Arduino board sitting idle at home? Do you want to control Arduino with Python to make it move, light up, or make sounds? Don’t hesitate, pyfirmata is your best choice! With it, you can easily control Arduino with Python and let your creativity fly!
Pyfirmata is a Python library that allows you to control Arduino using Python. It communicates with Arduino through the standard Firmata protocol, so you need to upload a Firmata firmware to Arduino first. But don’t worry, it’s simple; the Arduino IDE has a ready-made Firmata example.
The brilliance of pyfirmata lies in:
1. Easy Installation. Just one command `pip install pyfirmata` installs pyfirmata and its dependencies. No extra drivers or software are needed.
2. User-Friendly. Pyfirmata provides an intuitive API to easily control Arduino’s digital pins, analog pins, PWM, Servo, etc. You don’t need to understand the details of the Firmata protocol, just call the API.
3. Fast Response. Thanks to the efficient Firmata protocol, pyfirmata can quickly read and write the pin states of Arduino. Typical control commands can be completed in a few milliseconds.
4. Powerful Features. Pyfirmata can not only control Arduino but also read data from it. You can use Arduino to collect data from various sensors and then analyze and process it with Python.
Are you eager to try it out? Hold on, let’s start with a small example to warm up.
In this example, we will use pyfirmata to control a digital pin on Arduino to make an LED connected to that pin blink.
First, connect the Arduino to your computer using a USB cable. Then open `File -> Examples -> Firmata -> StandardFirmata` in the Arduino IDE and upload it to the Arduino board.
Next, write a control script in Python called `blink.py`:
“`python
from pyfirmata import Arduino, util
import time
board = Arduino(‘COM3’) # Change to your Arduino’s serial port
led_pin = board.get_pin(‘d:13:o’) # Pin 13, output mode
while True:
led_pin.write(1) # Turn on LED
time.sleep(1)
led_pin.write(0) # Turn off LED
time.sleep(1)
“`
This code first imports the `Arduino` and `util` classes from pyfirmata. Then it creates an `Arduino` object representing our Arduino board. Note that `’COM3’` should be changed to your Arduino’s actual serial port, which you can check in the Arduino IDE under `Tools -> Port`.
Next, we use the `get_pin()` method to gain control of digital pin 13 and set it to output mode. This pin is connected to an onboard LED on the Arduino.
Finally, there’s an infinite loop that alternately writes 1 and 0 to pin 13, turning the LED on and off. A `time.sleep(1)` is added to control the blinking frequency.
Run this script, and you will see the LED on the Arduino blinking. Isn’t it satisfying?
**Tip**: If your LED doesn’t light up, check if the Arduino’s serial port is correctly selected and if the Firmata firmware has been successfully uploaded. Also, don’t forget to `import time`, or it will throw an error.
Controlling an LED is just an appetizer. Pyfirmata can do much more. For example:
1. Read Analog Values. Arduino’s analog pins can read voltage values from 0-5V, corresponding to digital values from 0-1023. You can connect a potentiometer, photoresistor, or other sensors to read their values using pyfirmata.
2. Control PWM. Arduino’s PWM pins can output adjustable voltages from 0-5V. With pyfirmata, you can easily set the duty cycle of PWM to control the brightness of LEDs, the speed of motors, etc.
3. Drive Servo Motors. Many servo motors can be directly connected to Arduino’s digital pins. You can use pyfirmata to precisely control the angle of the servo, enabling various mechanical movements.
4. Serial Communication. Pyfirmata essentially communicates with Arduino via serial. You can also write custom Firmata commands on Arduino and use pyfirmata to send and receive these commands for more flexible control.
You may have noticed that pyfirmata is just a bridge, mapping Arduino’s functionality into the world of Python. With this bridge, you can leverage Python’s powerful data processing, GUI, and network communication capabilities combined with Arduino’s hardware control abilities, creating infinite possibilities!
Of course, pyfirmata is not omnipotent. It can only control the pins on Arduino and cannot directly control I2C, SPI, or other bus devices. However, these devices can generally be controlled through Arduino’s pins. Moreover, due to bandwidth limitations of serial communication, pyfirmata’s real-time performance is not sufficient for scenarios with high timing requirements.
That said, there are also pitfalls with pyfirmata. For example, communication between Python and Arduino may occasionally freeze, requiring you to unplug and replug the USB cable. Additionally, the documentation for pyfirmata is not detailed enough, and many advanced usages require exploration. But these are not major issues; as long as you’re willing to tinker, you can always find solutions.
Alright, that’s it for the introduction to pyfirmata. Are you already bursting with ideas and eager to try? Quickly find an Arduino board to test it out! Remember to upload the Firmata firmware first. Also, if you want to connect more complex hardware, besides pyfirmata, you may need to learn Arduino programming and basic circuit design knowledge. Don’t be afraid; as long as you put in the effort, these are not issues!
By the way, pyfirmata is open-source on GitHub. If you encounter any issues or have suggestions, feel free to raise issues or contribute code. Let’s work together to improve this small but beautiful library! Also, if you create interesting projects with pyfirmata, be sure to share them with everyone! Who knows, you might get famous, haha!
That’s about it; I should roll up my sleeves and start tinkering with some amazing projects using pyfirmata. What? You ask what projects? Well, you’ll have to use your imagination for that. I can only say that with Arduino and Python, you can create many wonderful things. Enough talk, let’s get started!
