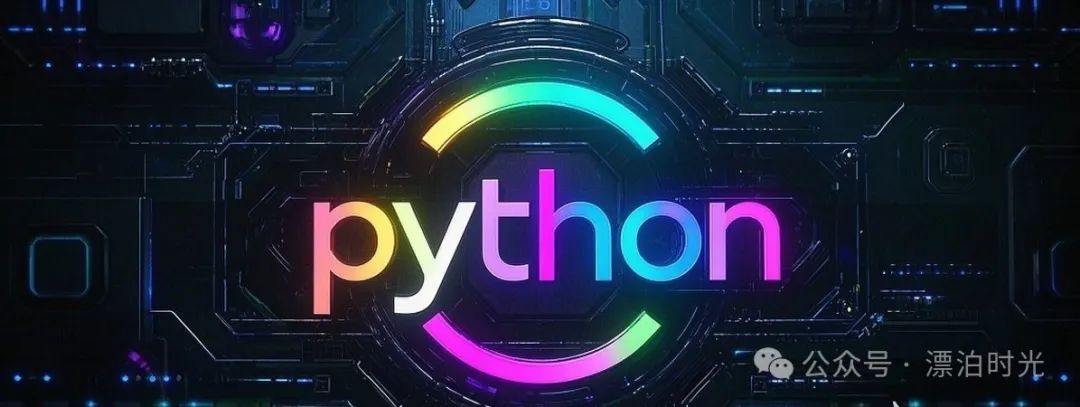
Pyfirmata – Making Arduino Programming Incredibly Simple!
Hello everyone! Today, I want to introduce you to a super useful Python library – Pyfirmata! 😎
I still remember the first time I played with Arduino, I was overwhelmed by the C++ code. Why did I have to write so much complex code just to make an LED blink?
Then I discovered this treasure library called Pyfirmata, and it was love at first sight! Using Python to control Arduino is as simple as chatting with hardware~
1
Preparation

First, you need to prepare the following items:
-
An Arduino development board (Uno/Mega/Leonardo is fine) -
USB data cable -
Python environment (3.6+) -
Arduino IDE
Install Pyfirmata:
pip install pyfirmata
In the Arduino IDE:
-
Open File > Examples > Firmata > StandardFirmata -
Upload to the board
That’s it! The environment is ready! 🚀
2
Light Up Your First LED

Let’s take a look at the most basic LED control code:
from pyfirmata import Arduino
import time
# Connect to Arduino, modify according to the actual port
board = Arduino('COM3') # Use COMx for Windows, /dev/ttyUSBx for Mac/Linux
# Set pin 13 (onboard LED) to output mode
led = board.get_pin('d:13:o')
# Blink the LED 5 times
for i in range(5):
led.write(1) # Turn on LED
time.sleep(1) # Wait 1 second
led.write(0) # Turn off LED
time.sleep(1)
board.exit()
Isn’t it super simple? Much cleaner than native Arduino code! 🎉
3
Mastering Analog Input and Output

Reading analog sensors is also very easy:
from pyfirmata import Arduino, util
import time
board = Arduino('COM3')
# Start iterator thread to read analog values
it = util.Iterator(board)
it.start()
# Set A0 to input mode
sensor = board.get_pin('a:0:i')
# Set D11 to PWM output
led = board.get_pin('d:11:p')
while True:
# Read sensor value (between 0-1)
sensor_value = sensor.read()
if sensor_value is not None: # Ensure a valid value is read
# Control LED brightness directly with sensor value
led.write(sensor_value)
time.sleep(0.1)
This allows you to control LED brightness with a sensor, super cool, right? ✨
4
Useful Tips

-
Batch setting multiple pins:
# Control multiple LEDs at once
pins = [board.get_pin(f'd:{i}:o') for i in range(8,13)]
-
Use Layout functionality to simplify code:
from pyfirmata import Arduino, Layout
board = Arduino('COM3')
layout = Layout(board)
# Access directly using pin numbers
layout.digital[13].write(1)
-
Error handling is important:
try:
board = Arduino('COM3')
except:
print('Please check the Arduino connection!')
exit()
5
Usage Suggestions

-
Remember to call <span>board.exit()</span>
to close the connection in time -
Use iterators for analog readings -
PWM output value range is 0-1 -
Add appropriate delays in the code to avoid excessive communication frequency
6
Interactive Session

Try these exercises:
-
Create a breathing light effect -
Control LED with a button -
Implement a running light with multiple LEDs
Pyfirmata really makes Arduino programming super easy, I hope this article helps you!
Come and give it a try, I believe you will also fall in love with this simple and elegant way of hardware programming! 😊
Previous Issues Review
01
02
03