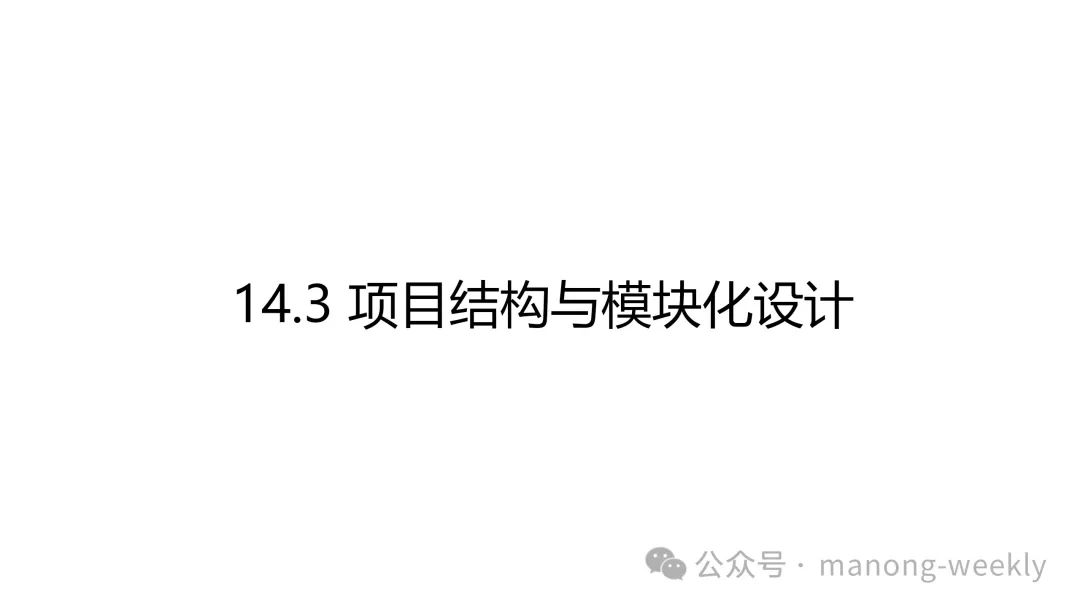
14.3 Project Structure and Modular Design
When building command-line tools, a reasonable project structure and modular design are key to ensuring the project’s scalability, maintainability, and readability. Rust’s module system is very powerful, allowing you to organize your code into multiple files and modules for better management of complex projects. In this section, we will discuss how to build a command-line tool with a good project structure and use modular design to organize the code.
14.3.1 Project Structure
The basic structure of a Rust project consists of a <span>Cargo.toml</span>
file and a <span>src</span>
folder.<span>Cargo.toml</span>
is used to configure the project’s metadata, dependencies, etc., while the <span>src</span>
folder contains the source code files.
For a command-line tool project, the recommended file structure is as follows:
cli_tool_project/
├── Cargo.toml // Project configuration file
└── src/
├── main.rs // Main entry file
├── args.rs // Module for handling command line arguments
├── file_reader.rs // Module for handling file reading
└── error.rs // Error handling module
-
<span>Cargo.toml</span>
: Configures project dependencies, versions, etc. -
<span>main.rs</span>
: The entry file of the program. It initializes the application and calls other modules to perform actual functions. -
<span>args.rs</span>
: Module for handling command line arguments, parsing command line input, and passing results to other modules. -
<span>file_reader.rs</span>
: Module for handling file reading, containing the logic for reading files. -
<span>error.rs</span>
: Unified error handling module, managing error types and messages in the project.
This structure helps us separate different functionalities into their respective modules, thus improving the maintainability and readability of the code.
14.3.2 Organizing Modules
Rust uses modules to organize code. Each module is a file or a folder (containing <span>mod.rs</span>
). By using the <span>mod</span>
keyword, we can break down the code into multiple functional units and share data and functions between different modules.
In Rust, we can organize modules into files as follows:
Step 1: Create the <span>args.rs</span>
Module
This module is responsible for parsing command line arguments. We can use <span>clap</span>
for argument parsing and pass the parsed results to <span>main.rs</span>
. Below is an example code for <span>args.rs</span>
:
// src/args.rs
use clap::{Arg, Command};
pub fn parse_args() -> Command {
Command::new("cli_tool")
.version("1.0")
.author("Rust Developer <[email protected]>")
.about("A command line tool that reads a file")
.arg(
Arg::new("file")
.short('f')
.long("file")
.takes_value(true)
.help("The file to read")
.required(true),
)
}
</[email protected]>
This module contains a <span>parse_args</span>
function that creates and returns a <span>Command</span>
instance for parsing command line arguments.
Step 2: Create the <span>file_reader.rs</span>
Module
This module handles file reading. It receives a file path and returns the content of the file, or an error if reading fails. Below is an example code for <span>file_reader.rs</span>
:
// src/file_reader.rs
use std::fs;
use std::io;
pub fn read_file(path: &str) -> Result<string, io::error=""> {
fs::read_to_string(path)
}
</string,>
This module defines a <span>read_file</span>
function that accepts a file path as a parameter, attempts to read the file, and returns its content. If it fails, it returns an <span>io::Error</span>
.
Step 3: Create the <span>error.rs</span>
Module
The error handling module is used to define error types and error messages in the project. We can define a unified error handling method in this module, such as returning a <span>Result</span>
type with error messages.
// src/error.rs
use std::fmt;
#[derive(Debug)]
pub enum CliError {
FileNotFound,
InvalidArgument,
}
impl fmt::Display for CliError {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
match *self {
CliError::FileNotFound => write!(f, "File not found!"),
CliError::InvalidArgument => write!(f, "Invalid argument!"),
}
}
}
This module defines a <span>CliError</span>
enum representing two types of errors: <span>FileNotFound</span>
and <span>InvalidArgument</span>
. We also implement the <span>fmt::Display</span>
trait for <span>CliError</span>
to facilitate converting errors into human-readable strings.
Step 4: Use These Modules in <span>main.rs</span>
Now that we have defined each module, we will organize the code in <span>main.rs</span>
and call these modules. <span>main.rs</span>
is responsible for starting the entire program, calling the argument parsing, file reading, and error handling modules.
// src/main.rs
mod args;
mod file_reader;
mod error;
use args::parse_args;
use file_reader::read_file;
use error::CliError;
use std::process;
fn main() {
// Parse command line arguments
let matches = parse_args().get_matches();
let file_path = matches.value_of("file").unwrap();
// Read file and handle errors
match read_file(file_path) {
Ok(content) => println!("{}", content),
Err(_) => {
eprintln!("{}", CliError::FileNotFound);
process::exit(1); // Error exit
}
}
}
In <span>main.rs</span>
, we introduce all other modules using the <span>mod</span>
keyword. Then, we call <span>args::parse_args</span>
to parse command line arguments and use <span>file_reader::read_file</span>
to read the file. If reading fails, we print the error message using <span>CliError::FileNotFound</span>
.
14.3.3 Build and Run with <span>Cargo</span>
Once you have completed the above steps and organized the file structure, use <span>cargo build</span>
and <span>cargo run</span>
to build and run the project.
cargo build // Build the project
cargo run -- --file example.txt // Run the project and pass parameters
14.3.4 Summary
By breaking down functionalities into multiple modules, we can improve the readability and maintainability of the code. Rust provides a powerful module system that helps us easily organize and reuse code. With a reasonable project structure, we can make the code of command-line tools clearer and easier to understand, facilitating future expansion and maintenance.
When building complex command-line tools, modular design is very important as it helps you separate different functionalities into independent files and modules, making the project more structured and clear.