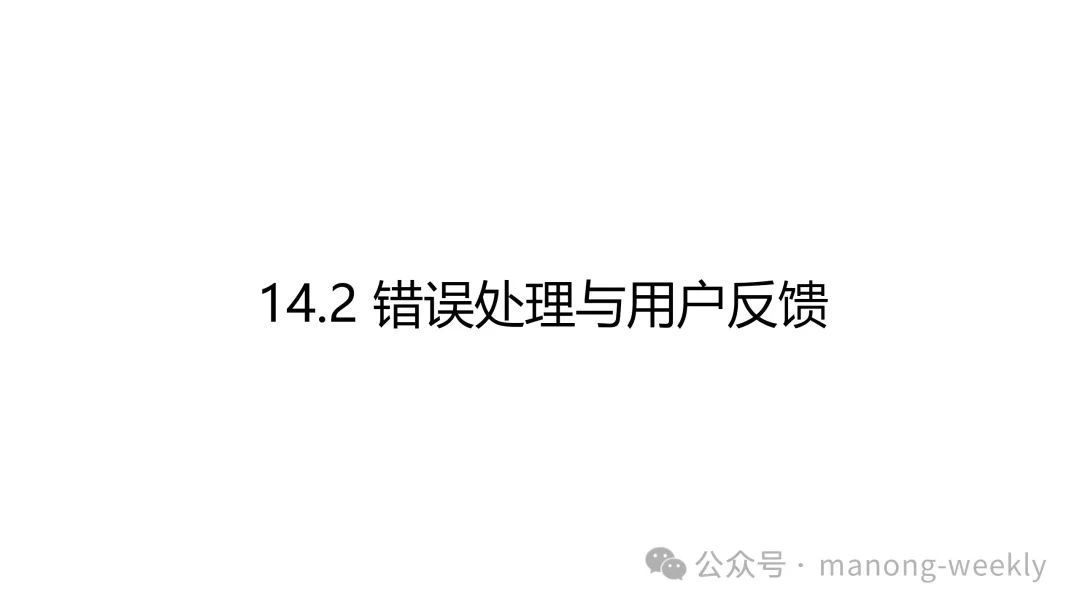
14.2 Error Handling and User Feedback
When building command-line tools, error handling and user feedback are crucial components. Proper error handling not only ensures the stability of the program but also provides users with clear and friendly feedback, helping them understand how to use the program and how to fix errors.
Rust itself provides a powerful error handling mechanism, allowing us to accurately capture and handle various types of errors in command-line tools, and to assist users with clear feedback. To achieve this, we typically use <span>Result</span>
and <span>Option</span>
types, combined with appropriate error messages and exit codes to handle errors.
14.2.1 Basic Concepts of Error Handling
Error handling in Rust is divided into two types:
-
Recoverable Errors: These errors can be handled through program logic, typically returning a
<span>Result<T, E></span>
type. The<span>Result</span>
enumeration has two variants:<span>Ok(T)</span>
and<span>Err(E)</span>
. -
Unrecoverable Errors: These errors are usually fatal errors of the program that cannot be recovered through program logic, and are typically thrown using the
<span>panic!</span>
macro.
Command-line tools typically need to handle recoverable errors and provide friendly error messages to users. Unrecoverable errors generally terminate the program and use appropriate exit codes to indicate the type of error.
14.2.2 Handling Errors with <span>Result</span>
In command-line tools, we can use <span>Result</span>
to handle errors that may occur during file operations, command-line argument parsing, etc. Below is an example of how to use the <span>Result</span>
type for error handling.
Assuming we have a command-line tool to read a file and output its content, if the file does not exist or cannot be read, it provides an error message to the user.
Step 1: Modify <span>Cargo.toml</span>
First, ensure that your <span>Cargo.toml</span>
includes the <span>clap</span>
dependency, as we will continue to use <span>clap</span>
to parse command-line arguments.
[dependencies]
clap = "4.0"
Step 2: Write Code
In <span>src/main.rs</span>
, use <span>Result</span>
to handle errors during file reading and provide feedback to the user:
use clap::{Arg, Command};
use std::fs;
use std::process;
fn main() {
let matches = Command::new("cli_file_reader")
.version("1.0")
.author("Rust Developer <[email protected]>")
.about("A simple CLI tool to read a file")
.arg(
Arg::new("file")
.short('f')
.long("file")
.takes_value(true)
.help("The file to read")
.required(true),
)
.get_matches();
// Get the file path parameter
let file_path = matches.value_of("file").unwrap();
// Read the file and handle errors
match read_file(file_path) {
Ok(content) => println!("{}", content),
Err(e) => {
eprintln!("Error: {}", e);
process::exit(1); // Non-zero exit code indicates error
}
}
}
// Function to read file content
fn read_file(path: &str) -> Result<string, string=""> {
fs::read_to_string(path).map_err(|e| format!("Failed to read file: {}", e))
}
</string,></[email protected]>
Step 3: Explain the Code
-
<span>Command</span>
and<span>Arg</span>
are used to parse command-line arguments, accepting a required file path parameter. -
<span>read_file</span>
function attempts to read the file at the specified path, returning<span>Ok(String)</span>
if successful, or<span>Err(String)</span>
if it fails, converting the error to a custom error message using<span>map_err</span>
. -
If the file is read successfully, the program outputs the file content; if an error occurs, the program outputs the error message and exits with <span>process::exit(1)</span>
, returning a non-zero exit code indicating an error occurred.
Step 4: Compile and Run
Compile and run the program, passing the correct file path:
cargo run -- --file test.txt
If the file is read successfully, the output will be:
Hello, this is a test file!
If the file does not exist or another error occurs, an error message similar to the following will be output:
Error: Failed to read file: No such file or directory (os error 2)
And the program will end with a non-zero exit code, indicating an error occurred.
14.2.3 Formatting and Feedback of Error Messages
To make error messages clearer, we can use formatted strings to add details, helping users understand why the error occurred. In the above example, <span>format!("Failed to read file: {}", e)</span>
is a typical way to format error messages, embedding the actual error information into a custom message using <span>e</span>
.
Additionally, error messages should be concise and clear, not only informing users of what the problem is but also providing suggestions on how to resolve the issue. For instance, if a file parameter is missing, we can provide help information to guide users in entering the parameter correctly.
14.2.4 Using <span>clap</span>
to Provide Help Information
<span>clap</span>
library has built-in functionality to automatically generate help information, assisting users in understanding how to properly use the command-line tool when errors occur.
If the user does not provide the required command-line arguments, <span>clap</span>
will automatically display an error message and help information:
cargo run -- --file
Output:
error: The following required arguments were not provided:
--file <file>
USAGE:
cli_file_reader --file <file>
</file></file>
14.2.5 Summary
Error handling and user feedback play an important role in command-line tools. By using Rust’s <span>Result</span>
type to handle recoverable errors, we can ensure that the program provides clear error messages to users when something goes wrong and guide them on how to fix the problem. Meanwhile, the <span>clap</span>
library allows us to easily generate help information, helping users understand how to properly use the program.
When developing command-line tools, reasonable error handling and friendly user feedback not only improve the usability and user experience of the program but also enhance the program’s stability and reliability.