Last time we had a brief introduction to ESP32 and the official examples. For more in-depth understanding, you can visit the official website of Anxin Ke.
Of course, it has a clock speed of up to 240MHz, external FLASH of 4M, which is sufficient for use, and it also comes with WIFI and Bluetooth, along with about 40 IO ports.
The most important thing is that the ESP32S model development board is only 27 yuan, and it includes free shipping.
After buying it, it looks like this
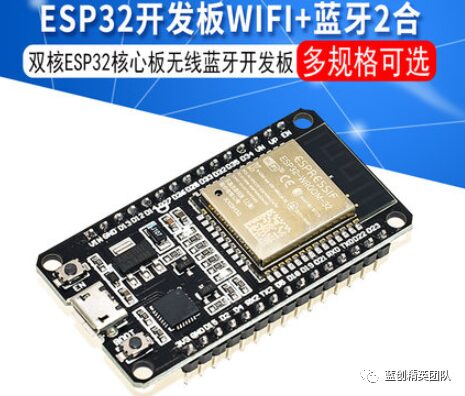
Introduction to NanoFramework
When it comes to C# embedded microcontroller development, we cannot ignore the early .Net Micro Framework, abbreviated as .Net MF. In 2018, I worked with Ye Fan and Liu Hongfeng in Beijing, and we were working on the .Net MF framework. Liu successfully developed a series of product systems based on .Net MF.
Of course, the .NET MF product was abandoned by Microsoft itself and is no longer maintained or developed.
However, some overseas experts did not want such projects to be abandoned, especially since I have always been optimistic about such projects, so they began open-source level maintenance.
This project is called NanoFramework, which can be said to be an upgraded version of .Net MF, mainly because it is more fun.
Moreover, this project NanoFramework is supported by the .Net Foundation. (It feels like back to Microsoft’s own home.)
Official Description of NanoFramework
.NET nanoFramework is a free open-source platform that allows writing managed code applications for resource-constrained embedded devices. It is suitable for various types of projects, including IoT sensors, wearable devices, academic proofs of concept, robotics, maker/manufacturer creations, and even complex industrial devices. It enables embedded developers to access modern technologies and tools used by desktop application developers, making development on such platforms easier, faster, and more cost-effective.
Writing Embedded Microcontroller Logic in C#
The newly purchased ESP32 requires firmware flashing (equivalent to flashing, or changing from XP to Windows system).
First, the board needs to be connected to the computer via USB, and a serial port device should appear as shown below
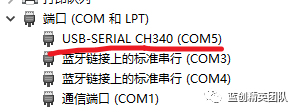
At this point, it proves that the board has been connected to the computer via the serial port.
Flashing (Writing Firmware, Bin File Flashing)
Since we are using VS2019 to develop NanoFramework, we need to install the Nanoff tool to flash (firmware, Bin file)
dotnet tool install -g nanoff
After installation
Execute the Flashing Command
My board is the ESP32S model, so I need to flash this command (COM5 should be replaced with your own COM port name)
nanoff --serialport COM5 --target ESP32_PSRAM_REV0 --update
If it is another one, you can refer to the Nanoff help command cmd and then enter nanoff to see its command help manual.
You might see the following situation:
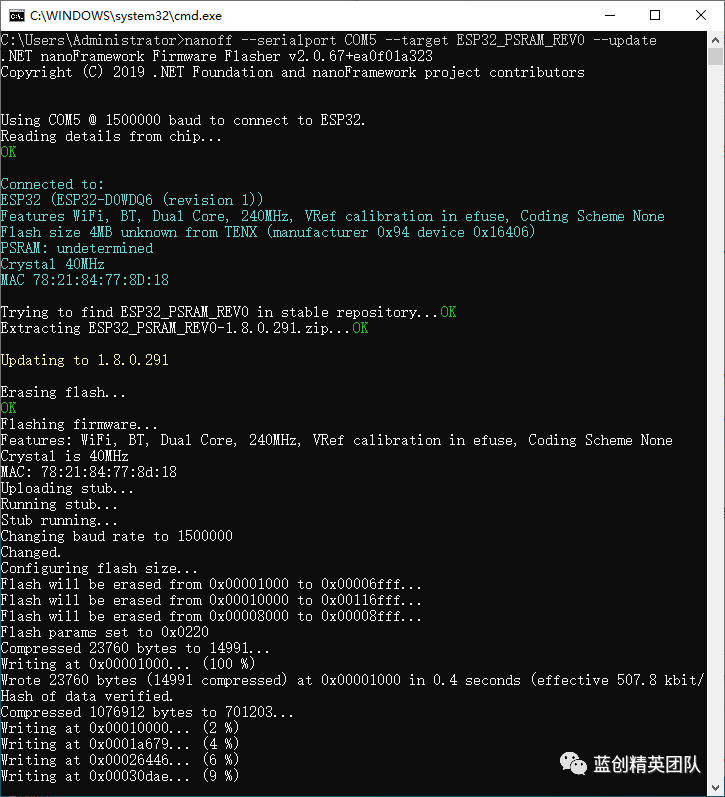
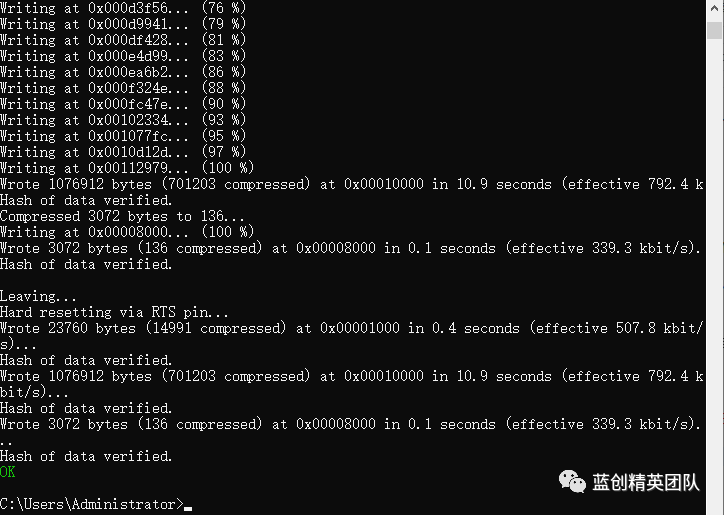
If it shows OK, then it’s done. There are no issues.
Of course, you can also enter the following command to see what all the current serial ports are
nanoff --listports
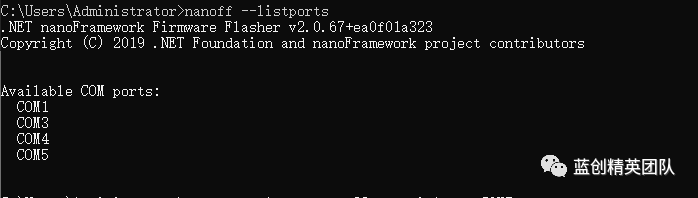
Starting a New Project in VS2022
Of course, I am using 2022, you can use other versions, the same principle.
NanoFramework VS Extension Installation
Open VS, Extensions, Manage Extensions
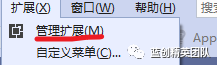
Search for the keyword NanoFramework, then click install (it might be a bit slow on my side, about 10 minutes?)
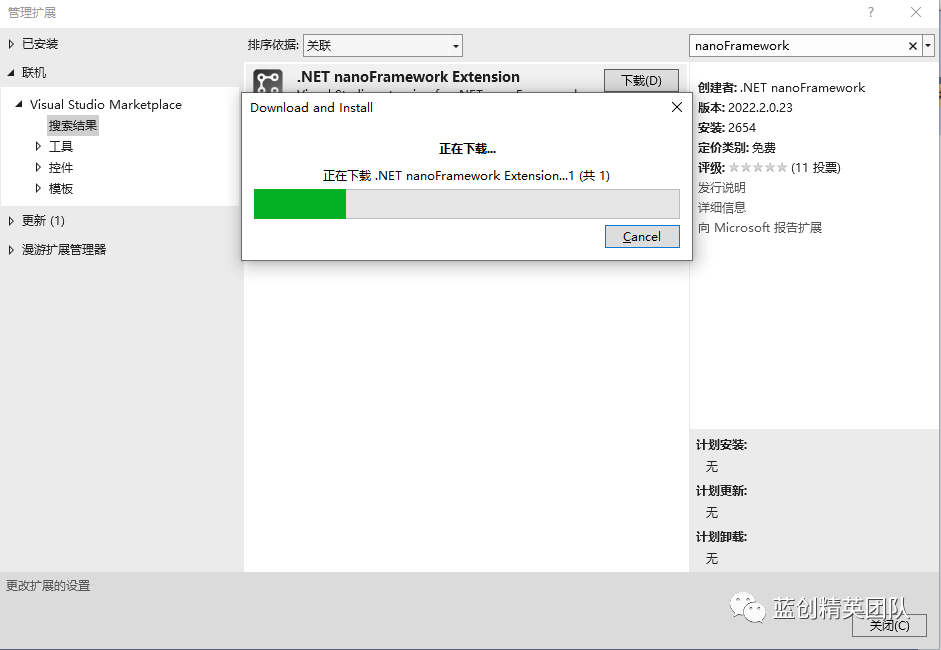
Then, close VS, and the installation will pop up automatically, just click next and it’s OK.
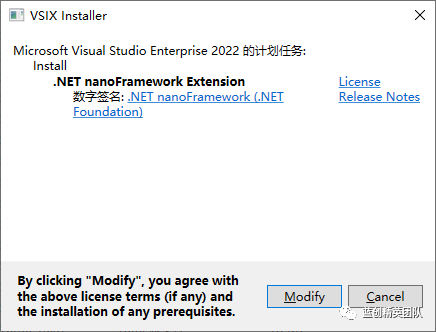
Continue
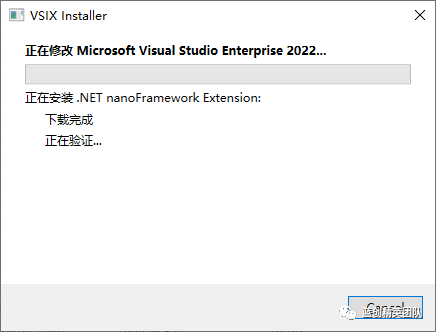
Creating a NanoFramework Project
Reopen VS.
Select Blank Application, and click Create
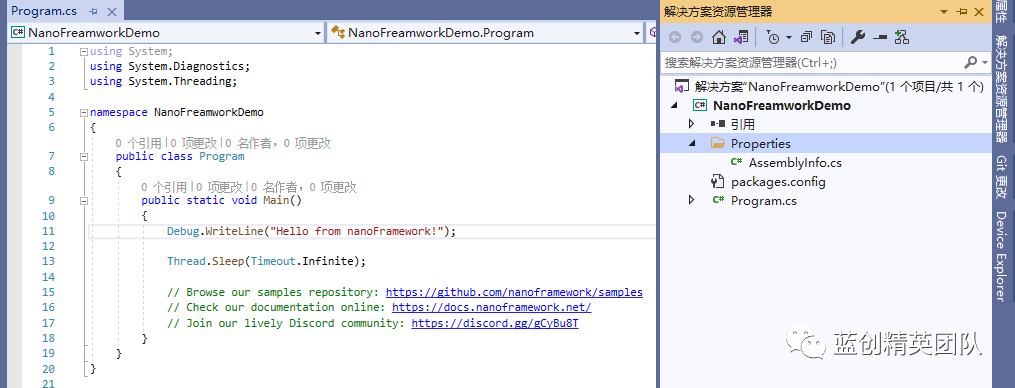
After creation, it looks like this.
A very simple example.
Of course, our example needs to show some features and highlight its advantages.
Change it to look like this
public class Program
{
public static void Main()
{
Thread thread = new(new ThreadStart(Testc));
thread.Start();
while (true)
{
Debug.WriteLine($"fine , think you !");
Thread.Sleep(1000);
}
}
private static void Testc()
{
while (true)
{
Debug.WriteLine($"how are you ? \r\n");
Thread.Sleep(1000);
}
}
}
Right-click the project, build the code, no errors, then start flashing to the device
Device Explorer
You can open the device explorer through the following operation.
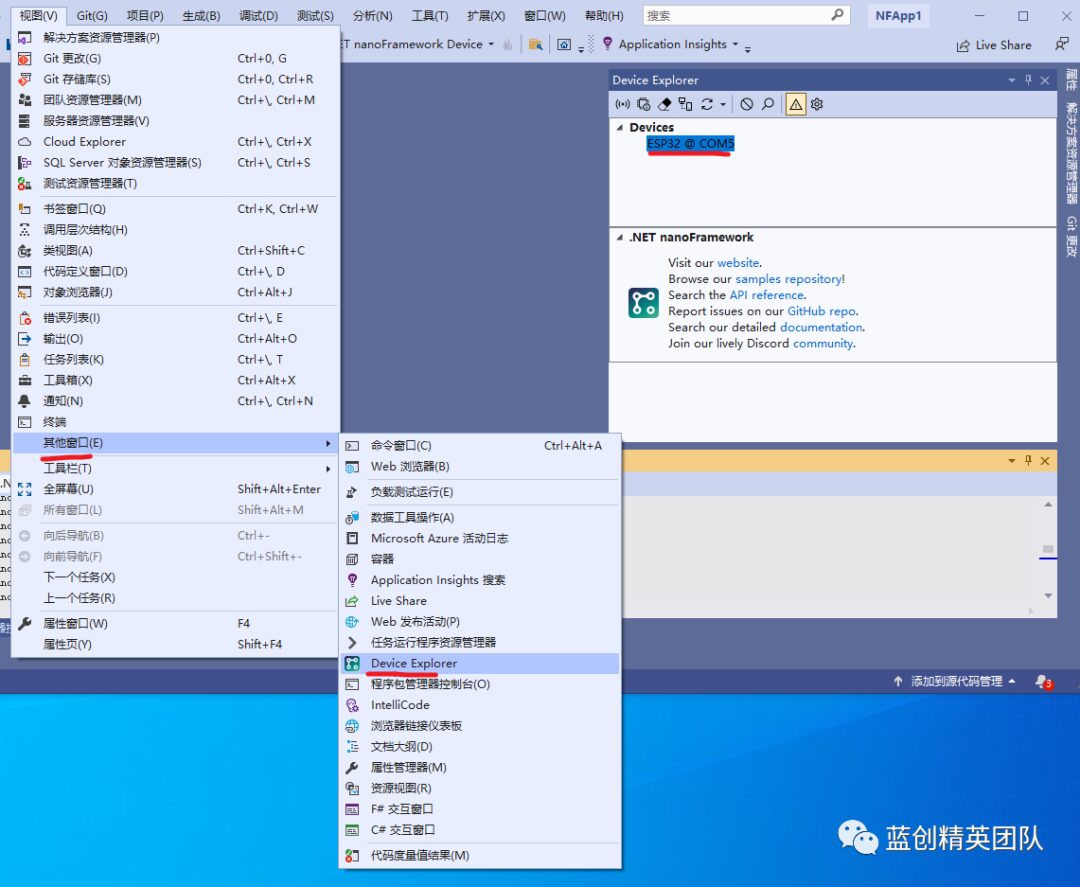
Device Explorer Explanation
If everything is normal, the following ESP32 @ COM5 information will appear
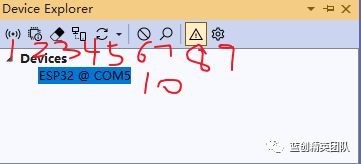
(1). Ping the device, after clicking, the VS output will show the following information
ESP32 @ COM5 is active running nanoCLR.
(2). Collect device information
After clicking, it will output all the information of the device
System Information
HAL build info: nanoCLR running @ ESP32 built with ESP-IDF v4.4.1
Target: ESP32
Platform: ESP32
Firmware build Info:
Date: Jun 27 2022
Type: MinSizeRel build, chip rev. >= 0, support for PSRAM
CLR Version: 1.8.0.291
Compiler: GNU ARM GCC v8.4.0
OEM Product codes (vendor, model, SKU): 0, 0, 0
Serial Numbers (module, system):
00000000000000000000000000000000
0000000000000000
Target capabilities:
Has nanoBooter: NO
IFU capable: NO
Has proprietary bootloader: YES
AppDomains:
Assemblies:
Native Assemblies:
mscorlib v100.5.0.17, checksum 0x004CF1CE
nanoFramework.Runtime.Native v100.0.9.0, checksum 0x109F6F22
nanoFramework.Hardware.Esp32 v100.0.7.3, checksum 0xBE7FF253
nanoFramework.Hardware.Esp32.Rmt v100.0.3.0, checksum 0x0A915860
nanoFramework.Device.OneWire v100.0.4.0, checksum 0xB95C43B4
nanoFramework.Networking.Sntp v100.0.4.4, checksum 0xE2D9BDED
nanoFramework.ResourceManager v100.0.0.1, checksum 0xDCD7DF4D
nanoFramework.System.Collections v100.0.1.0, checksum 0x2DC2B090
nanoFramework.System.Text v100.0.0.1, checksum 0x8E6EB73D
nanoFramework.Runtime.Events v100.0.8.0, checksum 0x0EAB00C9
EventSink v1.0.0.0, checksum 0xF32F4C3E
System.IO.FileSystem v1.0.0.0, checksum 0x3AB74021
System.Math v100.0.5.4, checksum 0x46092CB1
System.Net v100.1.5.0, checksum 0x5BAB8CB3
System.Device.Adc v100.0.0.0, checksum 0xE5B80F0B
System.Device.Dac v100.0.0.6, checksum 0x02B3E860
System.Device.Gpio v100.1.0.4, checksum 0xB6D0ACC1
System.Device.I2c v100.0.0.1, checksum 0xFA806D33
System.Device.Pwm v100.1.0.4, checksum 0xABF532C3
System.IO.Ports v100.1.6.0, checksum 0xB798CE30
System.Device.Spi v100.1.2.0, checksum 0xB6C4B3BD
System.Device.Wifi v100.0.6.4, checksum 0x1C1D3214
Windows.Storage v100.0.2.0, checksum 0x954A4192
++++++++++++++++++++++++++++++++
++ Memory Map ++
++++++++++++++++++++++++++++++++
Type Start Size
++++++++++++++++++++++++++++++++
RAM 0x3ffe4bbc 0x0001b000
FLASH 0x00000000 0x00400000
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
++ Flash Sector Map ++
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Region Start Blocks Bytes/Block Usage
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
0 0x00010000 1 0x1A0000 nanoCLR
1 0x001B0000 1 0x1F0000 Deployment
2 0x003C0000 1 0x040000 Configuration
+++++++++++++++++++++++++++++++++++++++++++++++++++
++ Storage Usage Map ++
+++++++++++++++++++++++++++++++++++++++++++++++++++
Start Size (kB) Usage
+++++++++++++++++++++++++++++++++++++++++++++++++++
0x003C0000 0x040000 (256kB) Configuration
0x00010000 0x1A0000 (1664kB) nanoCLR
0x001B0000 0x1F0000 (1984kB) Deployment
Deployment Map
Empty
(3). Erase the application deployment area (remove project application)
22:58:09.928 [Connected to CLR. Pausing execution...]
22:58:10.454 [Erasing sector @ 0x001B0000...]
22:58:10.721 [Rebooting (ClrOnly)]
22:58:10.736 [Reboot command executed successfully]
22:58:10.736 [Waiting 5000ms for reboot...]
22:58:10.786 [!! Device reboot confirmed !!]
ESP32 @ COM5 deployment area erased.
(4). Edit and set network information
It’s similar to the system’s built-in, doing WIFI might require this thing.
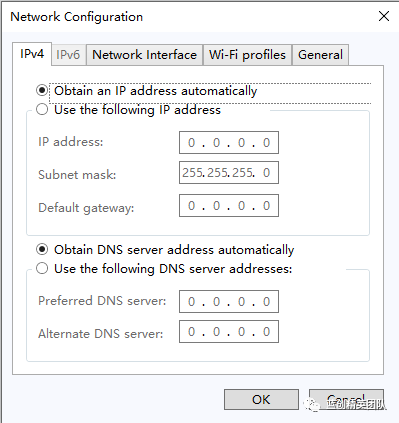
(5). Restart the device
(6). Start or stop device listening (which is to monitor whether the device exists)
(7). Re-search device information
(8). Output internal errors (see in VS output)
(9). Set information
(10). Specific device information
Troubleshooting Connection Issues
If it is not normal, then the flashing (firmware) has issues, please re-flash, then restart the microcontroller, and try again, it should be fine.
You can see the corresponding output in the output directory of VS.
Something like this
22:50:28.056 [NanoDevices: Serial device enumeration completed. Found 1 devices]
is normal
Deploy the project, just press F5, or click Run.
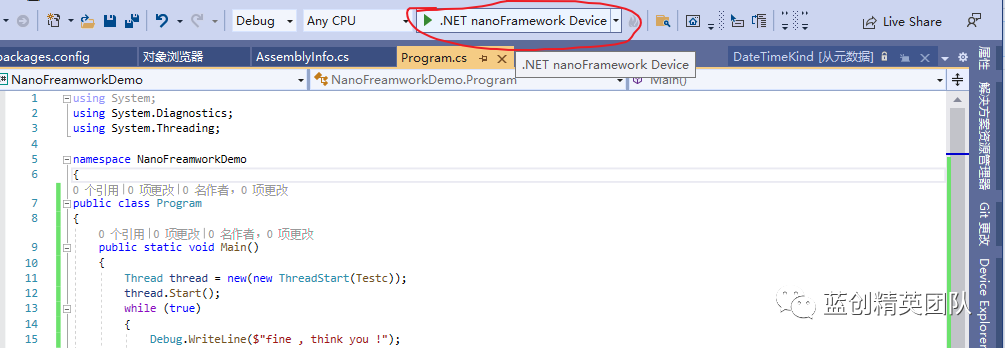
The effect is as shown:
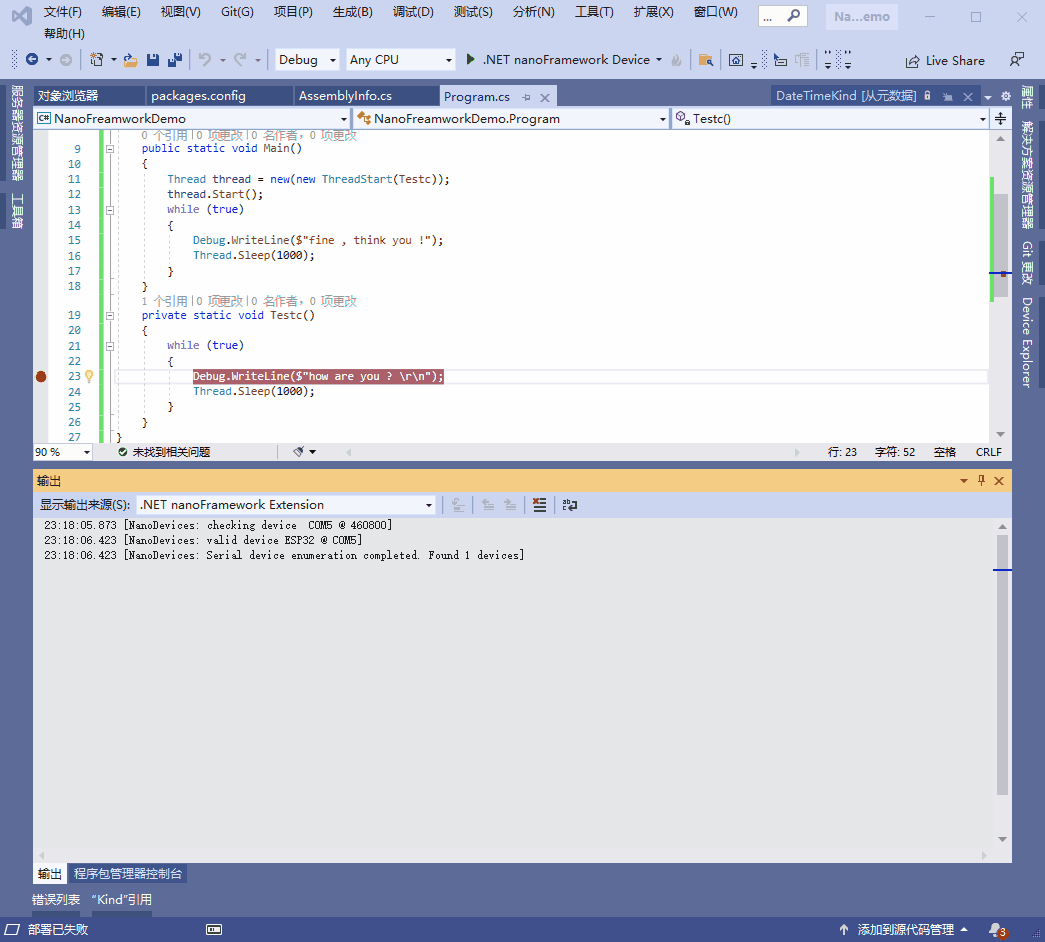
Achieving multithreading is simple and convenient, and it also supports VS debugging, which is even more enjoyable.
Conclusion
NanoFramework technology is very good, it can easily achieve the development of embedded devices like ESP32. Of course, some issues are normal; who doesn’t face problems in embedded development?
However, the board information and hardware information are all clear. From the application perspective, C# is still convenient, I feel it saves learning another language.
References
https://docs.nanoframework.net/content/getting-started-guides/getting-started-managed.html
End
One-click three links! Thank you for your support, your support is my motivation!