This article will introduce how to create a wake-on-LAN device using ESP32 hardware and implement the WOL (Wake-on-LAN) function through .Net nanoFramework, sending WOL packets to wake up remote computers.
1. Background
In previous articles, we introduced how to use libraries related to .Net nanoFramework to achieve various functions, such as reading sensor data, controlling LED lights, and displaying on OLEDs. We also discussed how to implement WOL (Wake-on-LAN) functionality using .NET and deploy it on NAS or through ASP.NET. Some of you may not have a NAS or server, but if you have ESP32 hardware, we can create a wake-on-LAN device using ESP32 hardware to send WOL packets to wake up remote computers. In this article, we will explain how to implement WOL (Wake-on-LAN) functionality through .Net nanoFramework by sending WOL packets to wake up remote computers.
Related articles:
•Implementing WOL to Wake Remote PCs with .NET•Using ASP.NET Version WOL Service•Deploying .NET Version WOL Remote Wake Service on NAS•Getting Started with .NET nanoFramework Development on ESP32-Pico•Solving the Inverted Color Issue of WS2812B LED on ESP32 and Application of Status Indicator
The above articles cover basic implementations and introductions related to WOL and foundational development knowledge of .Net nanoFramework. If you are not familiar with .Net nanoFramework, it is recommended to read these articles first, which can help you better understand the content of this article.
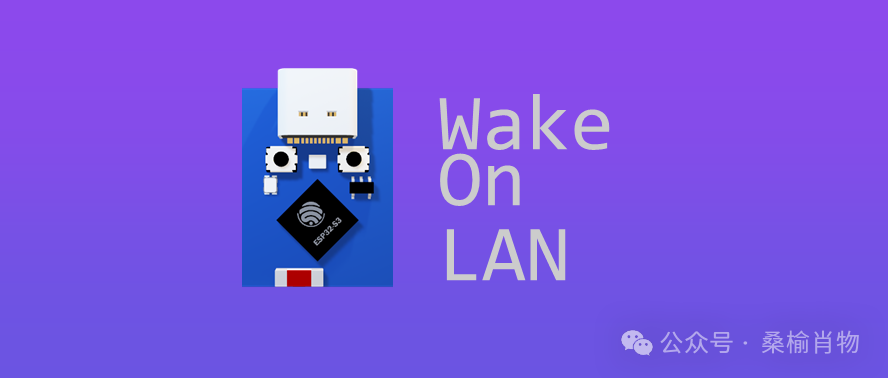
2. Implementation Method
In the article about the application of the status indicator, we introduced a sample project called <span>ProjectImprovWifi</span>
, which includes basic control of the status indicator. We can quickly implement WOL functionality based on this project framework.
The principle of WOL implementation is to send a specific data packet (Magic Packet) over the network to the target computer’s MAC address, which will wake up the target computer. In .Net nanoFramework, we can use the <span>nanoFramework.System.Net.Sockets.UdpClient</span>
library to send UDP packets.
The following code example demonstrates how to implement WOL functionality using .Net nanoFramework. It is important to note that due to environmental limitations, the use of <span>UdpClient</span>
and the absence of the <span>Replace</span>
method require us to modify the previous .NET code:
In this example, we define a <span>WakeOnLan</span>
class that contains the <span>Send</span>
method for sending WOL packets. In the <span>Send</span>
method, we first call the <span>CreateMagicPacket</span>
method to create a Magic Packet, and then call the <span>SendMagicPacket</span>
method to send the packet.
3. Page Design
After completing the core wake-up code, we need to allow users to input the target computer’s MAC address and click a button to send the WOL packet. We can use an OLED display to show prompt messages and the MAC address entered by the user. Here, we can create a web server using ESP32, allowing users to input the MAC address via a browser and send the WOL packet.
We will need the <span>nanoFramework.System.Net.Http</span>
library, which can be used to create a simple web server that receives user input and sends WOL packets.
The web content here is hardcoded in the code. We can create a simple web server using the <span>HttpListener</span>
class, which listens to user input. The user’s MAC address will be stored in local storage and displayed at the bottom of the page. Users can click the MAC addresses in the list to send WOL packets.
After starting the service, we use a simple infinite loop to listen for user requests, checking if the URL contains <span>/wol?mac=</span>
to send the WOL packet and then return a JSON formatted response.
4. Usage
After completing the code, we can deploy it to the ESP32. The first use requires completing the Improv Bluetooth configuration, which can be done using the “Improv Bluetooth Configuration” WeChat mini-program or through the Improv official website[1]. It is recommended to use the mini-program, as it can directly display the ESP32’s IP address after successful configuration.
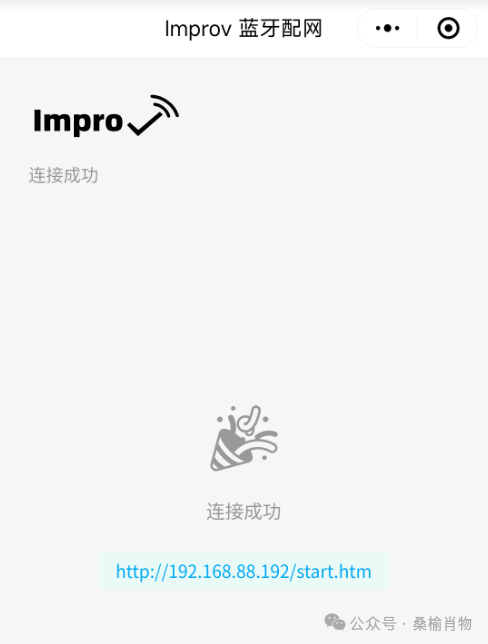
Then, by accessing the ESP32’s IP address via a browser, you can see the hardware’s web service, where you can input the MAC address and click the button to send the WOL packet.
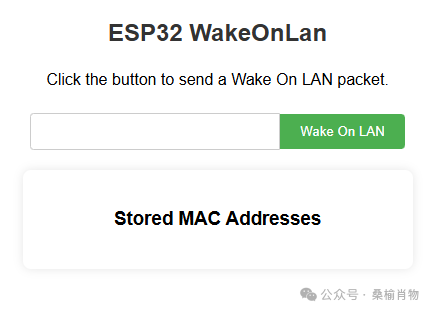
5. Summary
In this article, we introduced how to implement WOL functionality using .Net nanoFramework by sending WOL packets to wake up remote computers. We first implemented the core WOL code and then used a web server to receive user input, finally sending the WOL packets. This allows us to conveniently send WOL packets through a browser, achieving remote boot functionality.
The related code introduced in the article has been open-sourced on GitHub, and you are welcome to view and bookmark it. We hope this article is helpful to you, and if you have any questions or suggestions, please feel free to leave a comment.
If you are interested in the ESP32 version of WOL, you can follow “Sang Yu Xiao Wu” and reply “Network Wake” to get the complete source code.
GitHub homepage: https://github.com/sangyuxiaowu?WT.mc_id=DT-MVP-5005195
References
<span>[1]</span>
Improv official website: https://improv-wifi.com/