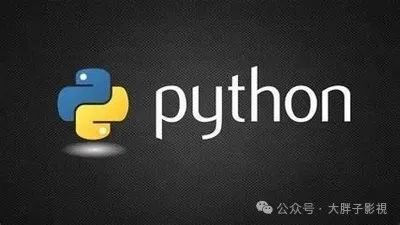
Hello everyone, today I want to share a super useful debugging tool – the Python extension for GDB! As a Python developer, debugging code is an essential skill. By combining GDB with Python scripts, we can make debugging smarter and more efficient. Let’s explore this powerful debugging tool together!
What is GDB-Python?
GDB-Python is a powerful extension of the GDB debugger that allows us to write debugging scripts in Python, enabling automated and customized debugging functions. In simple terms, it’s like giving GDB an “intelligent brain” that makes debugging more flexible and smart.
Setting Up the Basic Environment
We need to ensure that a GDB version supporting Python is installed on the system. You can check with the following commands:
gdb --version
python -v
If the GDB version supports Python, we can use Python in GDB!
Your First GDB-Python Script
Let’s look at a simple example where we create a custom command to display variable information:
import gdb
class PrintVarCommand(gdb.Command):
def __init__(self):
super(PrintVarCommand, self).__init__("pv", gdb.COMMAND_USER)
def invoke(self, arg, from_tty):
# Get variable value
try:
val = gdb.parse_and_eval(arg)
print(f"Variable {arg} has value: {val}")
except gdb.error:
print("Variable not found")
PrintVarCommand()
Save the above code as<span>debug_helper.py</span>
, then use it in GDB like this:
(gdb) source debug_helper.py
(gdb) pv some_variable
Tip: Remember to load the Python script using the<span>source</span>
command before using the custom command!
Automating Breakpoint Handling
Let’s see how to use Python to intelligently manage breakpoints:
import gdb
def break_on_function(func_name):
# Set breakpoint
bp = gdb.Breakpoint(func_name)
def stop_handler(event):
# Get function arguments
frame = gdb.selected_frame()
args = []
block = frame.block()
for symbol in block:
if symbol.is_argument:
args.append(f"{symbol.name} = {symbol.value(frame)}")
print(f"Function {func_name} was called")
print("Arguments:", ", ".join(args))
return False # Continue execution
bp.stop = stop_handler
Notes:
-
If the breakpoint handler function returns True, it will pause program execution -
Returning False will continue execution -
You can access any information provided by GDB in the handler function
Advanced Debugging Techniques
1. Memory Checker
class MemoryWatcher(gdb.Command):
def __init__(self):
super(MemoryWatcher, self).__init__("memwatch", gdb.COMMAND_USER)
def invoke(self, arg, from_tty):
addr = gdb.parse_and_eval(arg)
size = 16 # Display 16 bytes
inferior = gdb.selected_inferior()
mem = inferior.read_memory(addr, size)
print(f"Contents at memory address {addr}:")
print(' '.join(f"{b:02x}" for b in mem))
MemoryWatcher()
2. Variable Tracker
def track_variable(var_name):
def value_changed(event):
if hasattr(event, 'old_value') and hasattr(event, 'new_value'):
print(f"{var_name} changed from {event.old_value} to {event.new_value}")
watch = gdb.watch(var_name)
watch.callback = value_changed
Tip: Variable trackers are especially useful for debugging situations where values change unexpectedly!
Useful Tips
- Automatically Save Debug Logs
: Automatically save debugging information to a file - Conditional Breakpoints
: Set smarter breakpoint conditions - Data Visualization
: Use Python’s chart libraries to visualize data structures
Exercises:
-
Try writing a command to display all local variables -
Implement a function call counter -
Create a memory leak detector
Friends, that’s it for today’s Python learning journey! Remember to code actively, and feel free to ask me any questions in the comments. With GDB-Python, debugging is no longer a tedious task, but an exciting exploration filled with magic! I wish everyone happy learning and continuous improvement in Python!
Like and Share

LetMoneyandLoveFlow to You