Click Blue Text To FollowServo and Motion Control
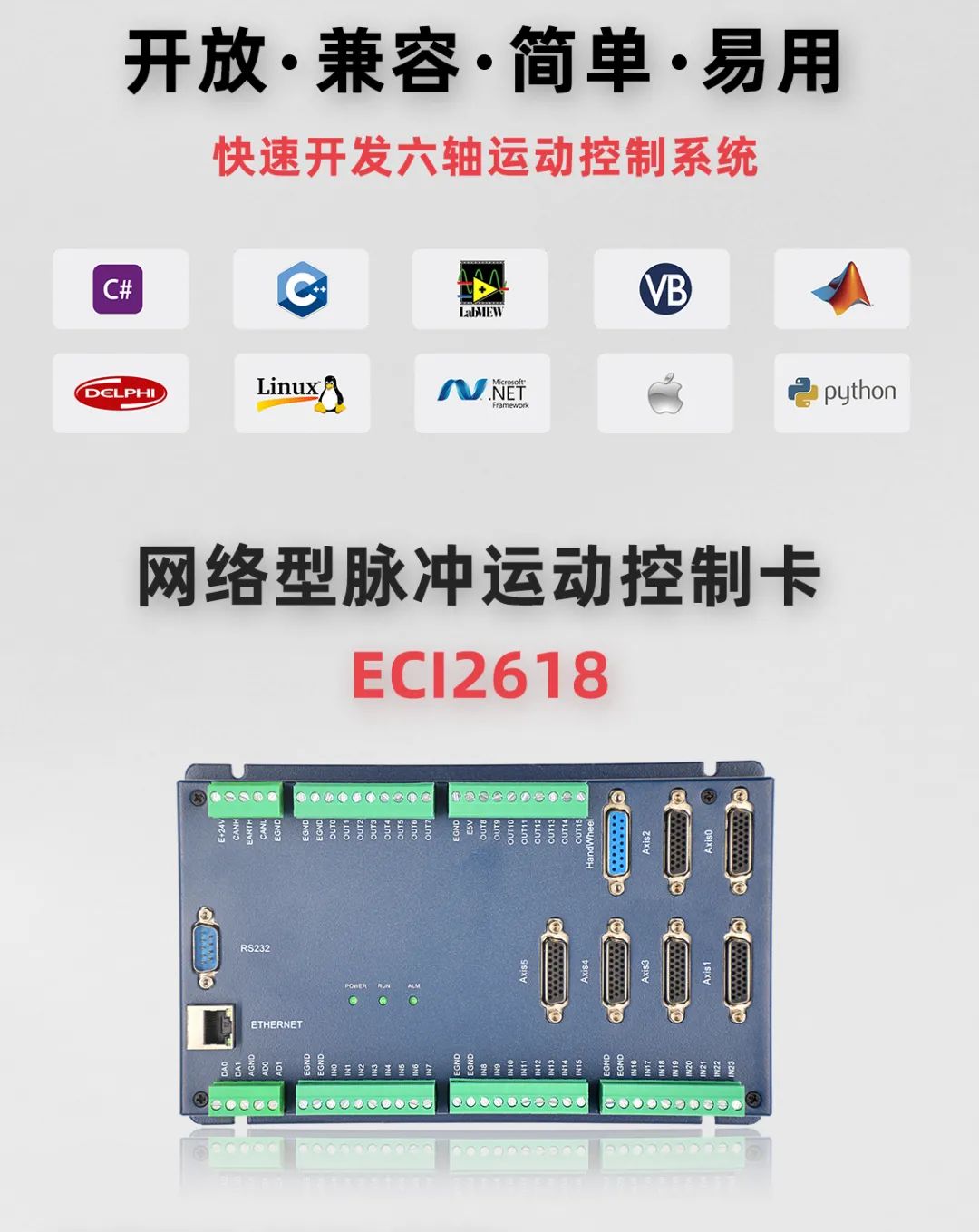
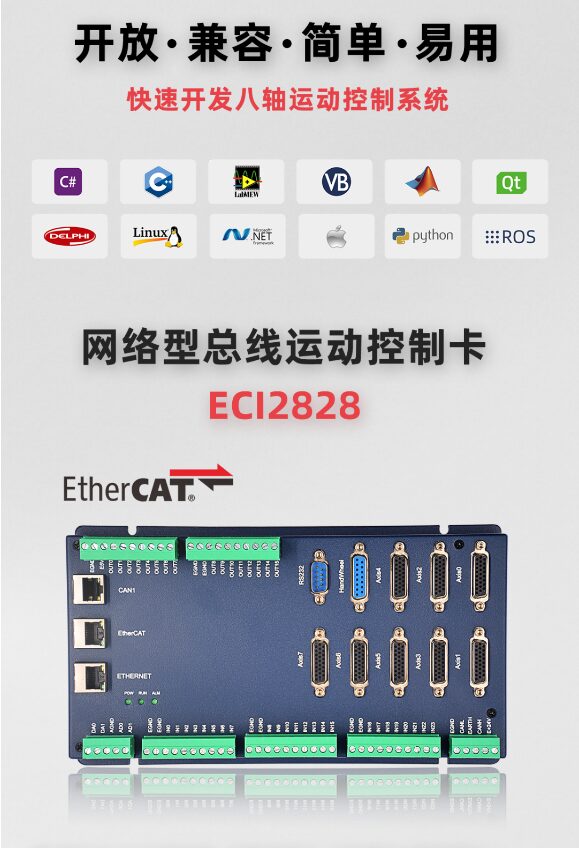
ECI2828 supports 8-axis bus-type input and encoder feedback, onboard 24-point input, 16-point output, 2-way AD, 2-way DA, and supports handwheel interface, with specific output ports supporting high-speed PWM control.
Both ECI2618 and ECI2828 use the same set of API functions and support development languages such as C, C++, C#, LabVIEW, Python, Delphi, etc., supporting platforms like VC6.0, VB6.0, Qt, .Net, and operating systems like Windows, Linux, WinCE, iMac.
Next, let’s get to the point. In this section, we will mainly discuss how to use C# for homing motion with the EtherCAT motion control card ECI2820 and the reading and writing function of the controller’s peripheral information.
The ECI2828 series control card supports up to 16-axis linear interpolation, arbitrary arc interpolation, spatial arcs, helical interpolation, electronic cam, electronic gear, synchronous follow, virtual axes, robotic arm commands, etc.; it uses an optimized network communication protocol to achieve real-time motion control.
The ECI2828 series motion control card supports Ethernet and 232 communication interfaces to connect with computers, receiving commands from the computer to operate. It can connect various expansion modules through EtherCAT and CAN buses, thus expanding the number of input/output points or motion axes.
The application program of the ECI2828 series motion control card can be developed using software such as VC, VB, VS, C++, C#, etc. The program requires the dynamic library zmotion.dll during operation. During debugging, the ZDevelop software can be connected to the controller simultaneously for convenient debugging and observation.
The ECI2828 series motion control card can be paired with ZCAN expansion modules using the CAN bus protocol for remote expansion. When resources such as IO, AIO, and axes are insufficient, ZCAN expansion modules can be added to increase the number of IO ports, AIO ports, and axis interfaces. Below are several interface diagrams of expansion boards.
ZIO1632MT
EIO24088
1. Create a New MFC Project and Add Function Library
(1) In the VS2015 menu, click “File” → “New” → “Project” to start the project creation wizard.
(2) Choose the development language as “Visual C#” and .NET Framework 4, and select Windows Forms Application.
(3) Find the C# function library provided by the manufacturer in the CD materials, with the following path (64-bit library as an example):
A. Enter the “8.PC Functions” folder in the materials provided by the manufacturer.
B. Select the “Function Library 2.1” folder.
C. Select the “Windows Platform” folder.
D. Choose the corresponding function library as needed; here we choose the 64-bit library.
E. Unzip the C# compressed package, which contains the corresponding function library for C#.
F. The specific path of the function library is as follows.
(4) Copy the C# library files and related files provided by the manufacturer into the newly created project.
A. Copy the zmcaux.cs file into the new project.
B. Place the zaux.dll and zmotion.dll files into the bin\debug folder.
(5) Open the newly created project file in VS, right-click on the zmcaux.cs file in the Solution Explorer, and click “Include in Project”.
(6) Double-click Form1.cs inside Form1 to open the code editing interface, write “using cszmcaux” at the beginning of the file, and declare the controller handle g_handle.
At this point, the project has been created and is ready for C# project development.
2. View the PC Function Manual
A. The PC function manual is also in the CD materials, with the specific path as follows: “CD Materials\8.PC Functions\Function Library 2.1\ZMotion Function Library Programming Manual V2.1.pdf”.
B. For PC programming, generally, if the network port links the controller and the industrial computer, the network port link function interface is ZAux_OpenEth(); if the link is successful, this interface will return a link handle. By operating this link handle, control of the controller can be achieved.
ZAux_OpenEth() Interface Description:
Project Application Screenshot:
C. Use homing motion-related commands to operate the link handle “g_handle” for homing motion control of the controller and reading various peripheral interfaces. The homing motion-related commands are as follows.
Homing Motion Commands:
Analog Input AD Read Command:
Analog Output DA Read Command:
Set the homing signal point, positive limit signal point, and negative limit signal point:
3. Development of Homing Motion in C#
(1) The human-computer interaction interface for homing motion control is as follows.
(2) A simple flowchart of the example.
(3) In the constructor of Form1, call the interface ZAux_OpenEth() to automatically link the controller during system initialization.
public Form1(){ InitializeComponent(); // Link controller zmcaux.ZAux_OpenEth("192.168.0.11", out g_handle); if (g_handle != (IntPtr)0) { MessageBox.Show("Controller linked successfully!", "Tip"); timer1.Enabled = true; } else { MessageBox.Show("Controller link failed, please check the IP address!", "Warning"); }}
(4) Update the controller axis status: current coordinates, peripheral information, etc., using a timer.
// Timerprivate void timer1_Tick(object sender, EventArgs e){ int[] runstate = new int[4]; float[] curpos = new float[4]; float adValue = 0; float daValue = 0; int axisstate = 0; int canstatus = 0; for (int i = 0; i < 4; i++) { // Get axis motion status and current position zmcaux.ZAux_Direct_GetIfIdle(g_handle, i, ref runstate[i]); zmcaux.ZAux_Direct_GetDpos(g_handle, i, ref curpos[i]); } canstatus = CanioStatus(); if (canstatus == 0) label_CanStatus.Text = "CAN expansion board status: Disconnected "; else label_CanStatus.Text = "CAN expansion board status: Normal "; label_X.Text = "X " + Convert.ToString(runstate[0] == 0 ? " Running " : " Stopped ") + curpos[0]; label_Y.Text = "Y " + Convert.ToString(runstate[1] == 0 ? " Running " : " Stopped ") + curpos[1]; label_Z.Text = "Z " + Convert.ToString(runstate[2] == 0 ? " Running " : " Stopped ") + curpos[2]; label_R.Text = "R " + Convert.ToString(runstate[3] == 0 ? " Running " : " Stopped ") + curpos[3]; // Scan analog value zmcaux.ZAux_Direct_GetAD(g_handle, Convert.ToInt32(textBox_AD.Text), ref adValue); zmcaux.ZAux_Direct_GetDA(g_handle, Convert.ToInt32(textBox_DA.Text), ref daValue); label_AD.Text = "AD size: " + Convert.ToString(adValue); label_DA.Text = "DA size: " + Convert.ToString(daValue); // Scan axis status zmcaux.ZAux_Direct_GetAxisStatus(g_handle, nAxis, ref axisstate); if (axisstate == 0) { label_AxisStatus.Text = "Axis status: Normal"; } else if (((axisstate >> 4) & 1) == 1) { label_AxisStatus.Text = "Axis status: Positive hard limit alarm!"; } else if (((axisstate >> 5) & 1) == 1) { label_AxisStatus.Text = "Axis status: Negative hard limit alarm!"; } else if (((axisstate >> 22) & 1) == 1) { label_AxisStatus.Text = "Axis status: Servo alarm!"; }}
(5) Use the event handler for the homing button to set the axis parameters and start the motion.
// Homing motionprivate void button_home_Click(object sender, EventArgs e) { if (g_handle == (IntPtr)0) { MessageBox.Show("Not linked to the controller!", "Tip"); } else { // Set axis parameters zmcaux.ZAux_Direct_SetAtype(g_handle, nAxis, 7); // Can be used for Z-phase homing zmcaux.ZAux_Direct_SetUnits(g_handle, nAxis, Convert.ToSingle(TextBox_units.Text)); zmcaux.ZAux_Direct_SetLspeed(g_handle, nAxis, Convert.ToSingle(TextBox_lspeed.Text)); zmcaux.ZAux_Direct_SetSpeed(g_handle, nAxis, Convert.ToSingle(TextBox_speed.Text)); zmcaux.ZAux_Direct_SetAccel(g_handle, nAxis, Convert.ToSingle(TextBox_accel.Text)); zmcaux.ZAux_Direct_SetDecel(g_handle, nAxis, Convert.ToSingle(TextBox_decel.Text)); zmcaux.ZAux_Direct_SetCreep(g_handle, nAxis, Convert.ToSingle(TextBox_creep.Text)); zmcaux.ZAux_Direct_SetDatumIn(g_handle, nAxis, Convert.ToInt32(TextBox_homeio.Text)); // Configure the origin signal. ECI series defaults ON when the signal is valid, normally open sensors need to reverse the input port to OFF zmcaux.ZAux_Direct_Single_Datum(g_handle, nAxis, home_mode); }}
(6) Use the event handler for the stop button to stop the interpolation motion.
// Stop motionprivate void Stop_Click(object sender, EventArgs e){ if (g_handle == (IntPtr)0) { MessageBox.Show("Not linked to the controller!", "Tip"); } else { zmcaux.ZAux_Direct_Single_Cancel(g_handle, nAxis, 2); }}
(7) Use the event handler for the parameter setting button to set the peripheral parameters and axis parameters for motion.
// Parameter settingsprivate void button_Para_Click(object sender, EventArgs e){ // Set parameters zmcaux.ZAux_Direct_SetDA(g_handle,Convert.ToInt32(textBox_DA.Text),(float)Convert.ToDouble(textBox_DaValue.Text)); zmcaux.ZAux_Direct_SetFwdIn(g_handle,nAxis,Convert.ToInt32(textBox_Fwd.Text)); zmcaux.ZAux_Direct_SetRevIn(g_handle,nAxis,Convert.ToInt32(textBox_Rev.Text)); // Set axis parameters zmcaux.ZAux_Direct_SetAtype(g_handle, nAxis, 7); // Can be used for Z-phase homing zmcaux.ZAux_Direct_SetUnits(g_handle,nAxis,Convert.ToSingle(TextBox_units.Text)); zmcaux.ZAux_Direct_SetLspeed(g_handle,nAxis,Convert.ToSingle(TextBox_lspeed.Text)); zmcaux.ZAux_Direct_SetSpeed(g_handle,nAxis,Convert.ToSingle(TextBox_speed.Text)); zmcaux.ZAux_Direct_SetAccel(g_handle,nAxis,Convert.ToSingle(TextBox_accel.Text)); zmcaux.ZAux_Direct_SetDecel(g_handle,nAxis,Convert.ToSingle(TextBox_decel.Text)); zmcaux.ZAux_Direct_SetCreep(g_handle,nAxis,Convert.ToSingle(TextBox_creep.Text)); zmcaux.ZAux_Direct_SetDatumIn(g_handle,nAxis,Convert.ToInt32(TextBox_homeio.Text)); // Configure the origin signal. ECI series defaults ON when the signal is valid, normally open sensors need to reverse the input port to OFF}
4. Debugging and Monitoring
Compile and run the example while connecting to the ZDevelop software for debugging to monitor the axis parameters and motion status of the motion control.
(1) Connect to the ZDevelop software and click “View” → “Oscilloscope” to open the oscilloscope to monitor the axis motion status.
(2) Run the upper computer software for debugging and monitoring.
(3) Zdevelop connection expansion board status display.
This time, Zheng Motion Technology shared the hardware wiring of the EtherCAT motion control card and C# hardware peripheral read/write and homing motion. More exciting content, please follow the “Zheng Motion Assistant” public account. For related development environments and example codes, please consult Zheng Motion Technology sales engineers: 400-089-8936.
This article is original to Zheng Motion Technology, and everyone is welcome to reprint it for mutual learning and improvement of China’s intelligent manufacturing level. The copyright of the article belongs to Zheng Motion Technology. If reprinted, please indicate the source.
Review Previous Content
VPLC Series Machine Vision Motion Control Integrated Machine Quick Start (Five) Size Measurement
VPLC Series Machine Vision Motion Control Integrated Machine Quick Start (Four) BLOB Presence Detection
VPLC Series Machine Vision Motion Control Integrated Machine Quick Start (Three) Vision Positioning Based on Shape Matching
VPLC Series Machine Vision Motion Control Integrated Machine Quick Start (Two) Basic Use of Camera
VPLC Series Machine Vision Motion Control Integrated Machine Quick Start (One) Introduction to Software and Hardware and Counting Example
Zheng Motion Technology XPLC516E Open Linux Platform Usage (Upper)
Zheng Motion Technology XPLC516E Open Linux Platform Usage (Lower)
Motion Control Card Application Development Tutorial for ROS (Lower)
Motion Control Card Application Development Tutorial for ROS (Upper)
Motion Control Card Application Development Tutorial for C++
Motion Control Card Application Development Tutorial for C#
Motion Control Card Application Development Tutorial for Python
Motion Control Card Application Development Tutorial for Linux
Motion Control Card Application Development Tutorial for VB.NET
Motion Control Card Application Development Tutorial for VB6.0
Motion Control Card Application Development Tutorial for VC6.0
Motion Control Card Application Development Tutorial for Using Qt
Motion Control Card Application Development Tutorial for LabVIEW
Motion Control Card Application Development Tutorial for Laser Galvo Control
Zheng Motion Technology | High-Precision Hardware Comparison Output Application in Visual Flying Photography
Zheng Motion Technology | UVW Positioning Platform Control Algorithm Application in Visual Guidance
About Zheng Motion Technology
Zheng Motion Technology focuses on research in motion control technology and the development of general motion control software and hardware products, is a national high-tech enterprise, and its main products include motion controllers, motion control cards, vision motion control integrated machines, human-machine interfaces, and expansion modules.
Zheng Motion Technology brings together outstanding talents from companies such as Huawei and ZTE, actively collaborating with major universities on research in fundamental motion control technology while adhering to independent innovation, making it one of the fastest-growing companies in the domestic industrial control field, and one of the few companies in China that fully master core motion control technologies and real-time industrial control software platform technologies.
Popular Articles
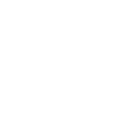
Industrial Fulian Revenue Exceeds 430 Billion
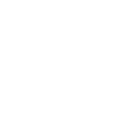
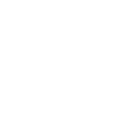
How Huawei Mate 40 is Made?
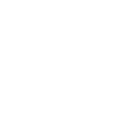
Introduction and Differences of Japan’s Tamagawa
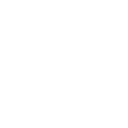