

Imagine after a busy workday, wanting to slack off to play games or watch movies, but afraid of being caught by the boss. Is there a way to successfully “slack off”? Or if someone is doing something on the computer that they don’t want others to find out about, is there a quick way to switch screens? Don’t worry, today I will teach you how to create a physical external device using Arduino to simulate a computer keyboard for automatic screen switching based on human detection.
1. Overall Design Concept
Previously, I did an experiment simulating a keyboard using ESP32, which of course has much better performance than ordinary Arduino boards like Arduino UNO, as it has built-in USB communication capabilities. Common Arduino development boards, such as Arduino UNO and Arduino MEGA, can connect to computers via USB and communicate with them through serial communication. This is because there is a chip on the development board that converts the MCU’s serial signals into USB signals, thus enabling communication with the PC.
On some special Arduino controllers, such as Arduino Leonardo, Esplora, Zero, Due, MKR, their MCUs have built-in USB communication capabilities (hereafter referred to as native USB). They communicate with computers without the need for external chips to convert signals, but instead simulate a serial communication interface on the computer through their own USB functionality, achieving a similar effect to using USB-to-serial chips for communication with the computer. These Arduino controllers with native USB can communicate with computers through native USB and can also simulate USB peripheral devices, such as USB keyboards and mice.
You can use the Keyboard and Mouse libraries provided by Arduino to simulate USB keyboards and mice.
In this case, we will use Arduino Leonardo to simulate keyboard operations, while using Arduino Nano as the transmitter. The two communicate via infrared, with the PIR human infrared sensor detecting human presence to trigger the screen switching operation. Here, taking a Windows computer as an example, pressing the “win” key and the “d” key can quickly show the desktop, and it can also simulate directly popping up the task manager, which is the combination key “ctrl+alt+delete”.
2. Circuit Connection
Introduction to Human Infrared Sensor:
There are many types of sensors that detect humans. The model I am using here is HC-SR505. This type of small human sensing module is an automatic control product based on infrared technology, with high sensitivity, strong reliability, ultra-small size, and ultra-low voltage operating mode. It has the following features:
1. Fully Automatic Sensing:When a person enters its sensing range, it outputs a high level; when the person leaves the sensing range, it automatically delays and turns off the high level, outputting a low level.
2. Repeatable Trigger Mode: That is, after the sensing output is high, if there is human activity in its sensing range during the delay time, the output will remain high until the person leaves, after which it will delay turning the high level into a low level (the sensing module automatically extends a delay time period after detecting each human activity, and the time of the next activity is the starting point of the delay time).
Transmitter:
Receiver:
3. Code Section
1. The receiver first obtains an infrared signal code by pointing any key of the remote control at the receiver to emit an infrared signal. The following code can display a segment of infrared signal code in the serial monitor, remember to prefix it with the hexadecimal symbol 0x. Note that the remote control uses the NEC encoding protocol, which is common in ordinary small remote controls.
#include <IRremote.h>//Import infrared libraryint RECV_PIN = 11;//Infrared signal receiving pinIRrecv irrecv(RECV_PIN);//Create infrared objectdecode_results results;void setup(){ Serial.begin(9600);//Open serial monitor // In case the interrupt driver crashes on setup, give a clue // to the user what's going on. Serial.println("Enabling IRin"); irrecv.enableIRIn(); // Start infrared reception Serial.println("Enabled IRin");//Print success}void loop() { if (irrecv.decode(&results)) { Serial.println(results.value, HEX);//Output infrared signal code in hexadecimal irrecv.resume(); // Prepare to receive the next infrared signal } delay(100);}
After successful upload, open the serial monitor, press a button on the remote control facing the receiver, and obtain the infrared coding information for one button. The infrared coding information I obtained for one button is: 0xFFA25D.
2. Upload the transmitter program to Arduino Nano
#include <IRremote.h> // Include IRRemote infrared library // The header file has defined PIN 3 as the signal output // So it can only connect to PIN 3, if changed please modify in the header fileconst int pir=2; //Define human infrared sensor signal pinconst int led=4;//LED signal pin to indicate whether a person is detected IRsend irsend; // Define IRsend object to emit infrared signals void setup(){ pinMode(pir,INPUT); pinMode(led,OUTPUT);} void loop(){ byte value=digitalRead(pir); if(value==1){ digitalWrite(led,HIGH); //Light up LED when a person is detected irsend.sendNEC(0xFFA25D, 32); //The FFA25D code here is one of the infrared codes obtained from the remote control, delay(2000); while(value!=0){ //While loop locks to avoid multiple high levels causing screen jump phenomenon if(digitalRead(pir)==0){ break; } } } else{ digitalWrite(led,LOW);//LED turns off when no person is detected }}
3. Upload the receiver program to Arduino Leonardo
#include <IRremote.h>int RECV_PIN = 11;//Infrared receiving tube signal pinIRrecv irrecv(RECV_PIN);decode_results results;#define OSX 0#define WINDOWS 1#define UBUNTU 2 //Macro definitions for computer operating systems#include "Keyboard.h" //Import the simulated keyboard libraryint platform = WINDOWS;// Select the operating system to matchvoid setup(){ Serial.begin(9600); Serial.println("Enabling IRin"); irrecv.enableIRIn(); // Start the receiver Serial.println("Enabled IRin"); Keyboard.begin();}void loop() { if (irrecv.decode(&results)) { Serial.println(results.value, HEX);//Print infrared coding information in hexadecimal in the serial monitor irrecv.resume(); // Receive the next infrared signal if(results.value==0xFFA25D){// // CTRL-ALT-DEL:// Keyboard.press(KEY_LEFT_CTRL);// Keyboard.press(KEY_LEFT_ALT);// Keyboard.press(KEY_DELETE); //If you want to return to the task manager, uncomment this part Keyboard.press(KEY_LEFT_GUI);//win+d combination key returns to desktop Keyboard.press('d'); delay(100); Keyboard.releaseAll();//Release keys delay(3000); } } delay(100);}
Swipe right to view the complete code and comments
4. Summary
After getting the code and hardware ready, you can try to pack the transmitter into a box. For those who can, you can 3D print a shell, and then place the entire transmitter device in a suitable location. Now, let me talk about some shortcomings I felt during the process. The prerequisite for using infrared for signal transmission is that the transmitter and receiver should face each other as much as possible; otherwise, some higher power wireless signals such as 2.4g, 433, etc., can be used in the local area.
This case is for entertainment purposes only. While “slacking off” is good, don’t overindulge! Work seriously and diligently!

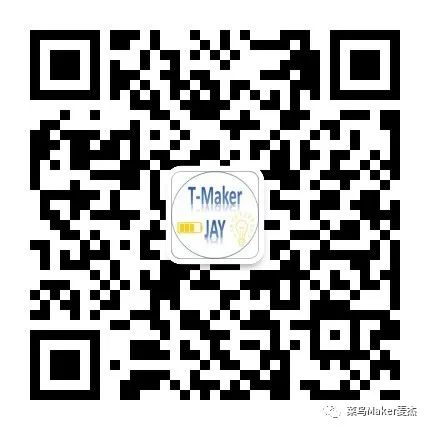

