1、GPIO Structure and Registers
GPIO includes multiple 16-bit I/O ports, each of which can independently set 3 types of input modes and 4 types of output modes, and can be independently set or reset.
GPIO consists of registers, input drivers, output drivers, and other components, as shown in the figure below.
GPIO operates through 7 32-bit registers:
The 4 configuration bits for each port are CNF[1:0] and MODE[1:0]:
The input driver includes pull-up/pull-down resistors and a Schmitt trigger, implementing 3 types of input configurations:
In floating input, the pull-up/pull-down resistors are disconnected; in pull-up/pull-down input, the pull-up/pull-down resistors are connected according to the data from ODR, and the Schmitt trigger is enabled in these two input configurations, with input data being input to the input data register or on-chip device (multiplexed input); in analog input, the pull-up/pull-down resistors are disconnected, the Schmitt trigger is disabled, and the analog input is sent to the on-chip device (such as ADC, etc.).
The output driver includes output control and output MOS transistors, implementing 4 types of output configurations:
General output data comes from the output data register, multiplexed output data comes from the on-chip device; when push-pull output is 0, the N-MOS transistor is turned on, and when output is 1, the P-MOS transistor is turned on; in open-drain output, the P-MOS transistor is off, when output is 0, the N-MOS transistor is turned on, when output is 1, the N-MOS transistor is also off, and the port is in a high-impedance state. The output driver is disabled during input configuration, and the pull-up/pull-down resistors of the input driver are disconnected during output configuration, allowing the output data to be input to the input data register via the Schmitt trigger.
Input data is realized through IDR. Output data can be realized through ODR, or bit operations can be performed through BSRR and BRR, that is, only the bits corresponding to 1 can be set or cleared without affecting the bits corresponding to 0, equivalent to performing bitwise “or” (set) and bitwise “and” (clear) operations on ODR.2、 GPIO Configuration
Input function configuration:
GPIO Pull-up/Pull-down: No pull-up and no pull-down – floating input; Pull-up – pull-up input; Pull-down – pull-down input:
Output function configuration: GPIO output level: Low – low level or High – high level; GPIO mode: Output Push Pull – push-pull output or; Output Open Drain – open-drain output. Maximum output speed: Low – low speed (2MHz) Medium – medium speed (10MHz) High – high speed (50MHz).After GPIO configuration is completed, the corresponding HAL and LL initialization programs are generated in HAL\Core\Src\gpio.c and LL\Core\Src\gpio.c respectively.
/* HAL project */__HAL_RCC_GPIOA_CLK_ENABLE();GPIO_InitStruct.Pin = GPIO_PIN_2;GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;GPIO_InitStruct.Pull = GPIO_NOPULL;GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;HAL_GPIO_Init(GPIOD, &GPIO_InitStruct);/* LL project */LL_APB2_GRP1_EnableClock(LL_APB2_GRP1_PERIPH_GPIOA);GPIO_InitStruct.Pin = LL_GPIO_PIN_2;GPIO_InitStruct.Mode = LL_GPIO_MODE_OUTPUT;GPIO_InitStruct.Speed = LL_GPIO_SPEED_FREQ_LOW;GPIO_InitStruct.OutputType = LL_GPIO_OUTPUT_PUSHPULL;LL_GPIO_Init(GPIOD, &GPIO_InitStruct);
3、GPIO Library Functions(1)GPIO HAL Library Functions
The basic GPIO HAL library functions are declared in stm32f1xx_hal_gpio.h as follows:
void HAL_GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_Init);GPIO_PinState HAL_GPIO_ReadPin(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);void HAL_GPIO_WritePin(GPIO_TypeDef* GPIOx, uint_16 GPIO_Pin, GPIO_PinState PinState);
Note: HAL does not have functions for reading and writing IDR and ODR, only direct register reads and writes.
1)Initialize GPIO
void HAL_GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_Init);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ GPIO_Init: Pointer to GPIO initialization parameter structure, O initialization parameter structure is defined as follows:
typedef struct { uint32_t Pin; /* Pin */ uint32_t Mode; /* Mode */ uint32_t Pull; /* Pull-up/Pull-down */ uint32_t Speed; /* Speed */ } GPIO_InitTypeDef;void HAL_GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_Init);
Where Pin and Mode are defined in stm32f1xx_hal_gpio.h as follows: #define GPIO_PIN_0 ((uint16_t)0x0001) /* Pin 0 */ …………………………………………………………………………………………………… #define GPIO_PIN_15 ((uint16_t)0x8000) /* Pin 15 */ #define GPIO_PIN_ALL ((uint16_t)0xFFFF) /* All pins */void HAL_GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_Init);Where Pin and Mode are defined in stm32f1xx_hal_gpio.h as follows: #define GPIO_MODE_INPUT 0x00000000u /* Floating input */ #define GPIO_MODE_OUTPUT_PP 0x00000001u /* General push-pull output */ #define GPIO_MODE_OUTPUT_OD 0x00000011u /* General open-drain output */ #define GPIO_MODE_AF_PP 0x00000002u /* Multiplexed push-pull output */ #define GPIO_MODE_AF_OD 0x00000012u /* Multiplexed open-drain output */ #define GPIO_MODE_ANALOG 0x00000003u /* Analog input */
2)Read GPIO Pin
GPIO_PinState HAL_GPIO_ReadPin(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ GPIO_Pin: GPIO pin, values are GPIO_PIN_0 ~ GPIO_PIN_15 or GPIO_PIN_ALL Return value: GPIO pin status, GPIO pin status is defined in stm32f1xx_hal_gpio.h as follows:
typedef enum { GPIO_PIN_RESET = 0U, GPIO_PIN_SET } GPIO_PinState;
Note: For multiple pins, return GPIO_PIN_RESET (0) when all pins are low.
3)Write GPIO Pin
void HAL_GPIO_WritePin(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, GPIO_PinState PinState);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ GPIO_Pin: GPIO pin, values are GPIO_PIN_0 ~ GPIO_PIN_15 or GPIO_PIN_ALL ★ PinState: GPIO pin status, values are GPIO_PIN_RESET or GPIO_PIN_SET Note: For multiple pins, all pins must have the same state.
(2)GPIO LL Library Functions
The basic GPIO LL library functions are declared in stm32f1xx_ll_gpio.h as follows:
ErrorStatus LL_GPIO_Init(GPIO_TypeDef* GPIOx, LL_GPIO_InitTypeDef* GPIO_InitStruct);uint32_t LL_GPIO_ReadInputPort(GPIO_TypeDef* GPIOx);uint32_t LL_GPIO_IsInputPinSet(GPIO_TypeDef* GPIOx, uint32_t PinMask);void LL_GPIO_WriteOutputPort(GPIO_TypeDef* GPIOx, uint32_t PortValue);void LL_GPIO_SetOutputPin(GPIO_TypeDef* GPIOx, uint32_t PinMask);void LL_GPIO_ResetOutputPin(GPIO_TypeDef* GPIOx, uint32_t PinMask);
1)Initialize GPIO
ErrorStatus LL_GPIO_Init(GPIO_TypeDef* GPIOx, LL_GPIO_InitTypeDef* GPIO_InitStruct);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ GPIO_InitStruct: Pointer to GPIO initialization parameter structure, initialization parameter structure is defined as follows:
typedef struct { uint32_t Pin; /* Pin */ uint32_t Mode; /* Mode */ uint32_t Speed; /* Speed */ uint32_t OutputType; /* Output type */ uint32_t Pull; /* Pull-up/Pull-down */ } LL_GPIO_InitTypeDef;ErrorStatus LL_GPIO_Init(GPIO_TypeDef* GPIOx, LL_GPIO_InitTypeDef* GPIO_InitStruct);
Where Pin, Mode, and OutputType are defined in stm32f1xx_ll_gpio.h as follows:
#define LL_GPIO_PIN_0 /* Pin 0 */ …………………………………………………………………………………………………… #define LL_GPIO_PIN_15 /* Pin 15 */ #define LL_GPIO_OUTPUT_PUSHPULL 0x00000000U /* Push-pull output */ #define LL_GPIO_OUTPUT_OPENDRAIN GPIO_CRL_CNF0_0 /* Open-drain output */ErrorStatus LL_GPIO_Init(GPIO_TypeDef* GPIOx, LL_GPIO_InitTypeDef* GPIO_InitStruct);
Where Pin, Mode, and OutputType are defined in stm32f1xx_ll_gpio.h as follows:
#define LL_GPIO_MODE_ANALOG 0x00000000U /* Analog mode */ #define LL_GPIO_MODE_FLOATING GPIO_CRL_CNF0_0 /* Floating mode */ #define LL_GPIO_MODE_INPUT GPIO_CRL_CNF0_1 /* Input mode */ #define LL_GPIO_MODE_OUTPUT GPIO_CRL_MODE0_0 /* General output mode */ #define LL_GPIO_MODE_ALTERNATE (GPIO_CRL_CNF0_1 | GPIO_CRL_MODE0_0) /* Multiplexed mode */ErrorStatus LL_GPIO_Init(GPIO_TypeDef* GPIOx, LL_GPIO_InitTypeDef* GPIO_InitStruct);
Return value: Error status, the error status is defined in stm32f1xx.h as follows:
typedef enum { SUCCESS = 0U, ERROR = !SUCCESS } ErrorStatus;
2)Read Input Port
uint32_t LL_GPIO_ReadInputPort(GPIO_TypeDef* GPIOx);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD Return value: Port value
3)Input Pin Status
uint32_t LL_GPIO_IsInputPinSet(GPIO_TypeDef* GPIOx, uint32_t PinMask);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ PinMask: Pin mask, values are LL_GPIO_PIN_0~15 or LL_GPIO_PIN_ALL Return value: Pin status (0 or 1) Note: For multiple pins, return 1 when all pins are high.
4)Write Output Port
void LL_GPIO_WriteOutputPort(GPIO_TypeDef* GPIOx, uint32_t PortValue);
Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ PortValue: Port value
5)Set Output Pin void LL_GPIO_SetOutputPin(GPIO_TypeDef* GPIOx, uint32_t PinMask); Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ PinMask: Pin mask, values are LL_GPIO_PIN_0~15 or LL_GPIO_PIN_ALL 6)Reset Output Pin void LL_GPIO_ResetOutputPin(GPIO_TypeDef* GPIOx, uint32_t PinMask); Parameter description: ★ GPIOx: GPIO name, values are GPIOA~GPIOD ★ PinMask: Pin mask, values are LL_GPIO_PIN_0~15 or LL_GPIO_PIN_ALL4、Using LCD
LCD is a low-power display device that can be controlled via a parallel interface or a serial interface.LCD is connected to STM32 through a parallel interface:
Note: Since LED and LCD share PC8~PC15, the control signals for LED and LCD cannot be effective simultaneously, that is, the LED’s LE and the LCD’s CS# or WR# cannot be effective at the same time. LCD function introduction:
The software design of the LCD is modified based on the LCD driver program in the competition resource package. The LCD library functions are divided into three categories: low-level library functions (hardware interface functions), middle-level library functions, and high-level library functions (software interface functions).
1)Low-Level Library Functions
The low-level library functions implement the low-level write operations of the LCD, the specific implementation steps are: (1) Add the following function declarations in gpio.h:
void LCD_Write(uint8_t RS, uint16_t Value); /* LCD Write */
(2) Add the following code after LED_Disp() in gpio.c:
/* HAL project code */ void LCD_Write(uint8_t RS, uint16_t Value) /* LCD Write */{ HAL_GPIO_WritePin(GPIOB, GPIO_PIN_10, GPIO_PIN_SET); /* RD#=1 */ HAL_GPIO_WritePin(GPIOB, GPIO_PIN_9, GPIO_PIN_RESET); /* CS#=0 */ GPIOC->ODR = Value; /* Output data */ if (RS == 0) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_8, GPIO_PIN_RESET);/* RS=0 */ } else { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_8, GPIO_PIN_SET); /* RS=1 */ }
2)Middle-Level Library Functions
The middle-level library functions implement the specific operations of the LCD controller, the program code is in lcd.c, the main content is as follows:
/* Write Register */void LCD_WriteReg(uint8_t LCD_Reg, uint16_t LCD_RegValue) /* Prepare to write RAM */void LCD_WriteRAM_Prepare(void) /* Write RAM */void LCD_WriteRAM(uint16_t RGB_Code)
3)High-Level Library Functions
The high-level library functions are the library functions called by the application program, declared in lcd.h as follows:
void LCD_Init(void);void LCD_Clear(uint16_t Color);void LCD_SetTextColor(uint16_t Color);void LCD_SetBackColor(uint16_t Color);void LCD_DisplayChar(uint8_t Line, uint16_t Column, uint8_t Ascii);void LCD_DisplayStringLine(uint8_t Line, uint8_t* ptr);
4)LCD Design Implementation
LCD_Init(); /* LCD Initialization */ LCD_Clear(Black); /* LCD Clear */ LCD_SetTextColor(White); /* Set Character Color */ LCD_SetBackColor(Black); /* Set Background Color */
When debugging with the simulator, use a logic analyzer to observe the waveforms of “PORTC” (State, hexadecimal display), “PORTB.8” (Bit), and “PORTB.5” (Bit) as shown in the figure.
END
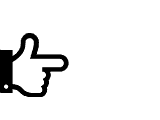