-
Layered Architecture
-
Multi-layer Architecture
-
Pipe/Filter Architecture
-
Client/Server Architecture
-
Model/View/Controller Architecture
-
Event-Driven Architecture
-
Microservices Architecture
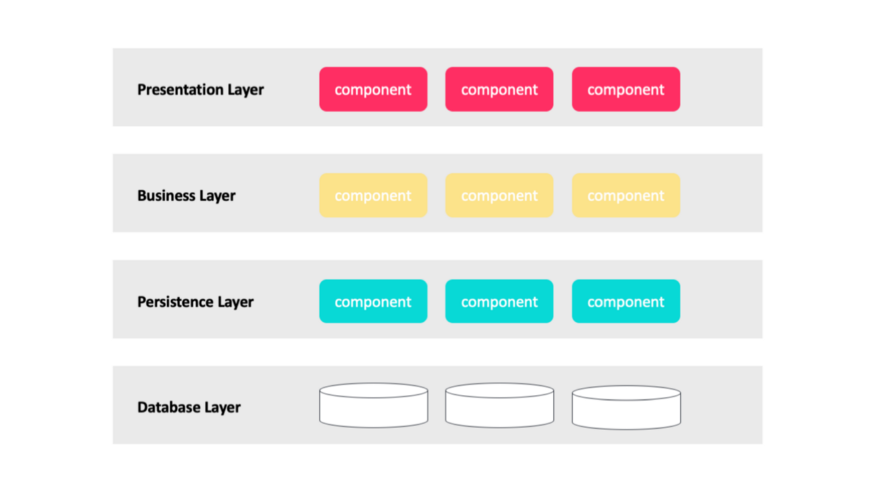
-
The first concept is that each layer has specific roles and responsibilities. For example, the presentation layer is responsible for handling all user interfaces. This separation of concerns in layered architecture makes it very simple to build efficient roles and responsibilities.
-
The second concept is that the layered architecture pattern is a technical partitioning architecture rather than a domain partitioning architecture. They are composed of components, not domains.
-
The last concept is that each layer in the layered architecture is marked as closed or open. A closed layer means that requests moving from one layer to another must go through the layer directly beneath it to reach the next layer. Requests cannot skip any layers.
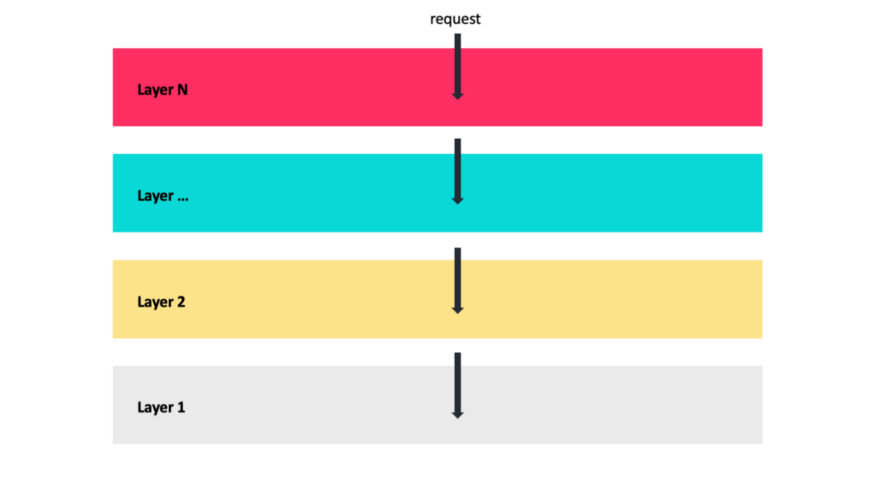
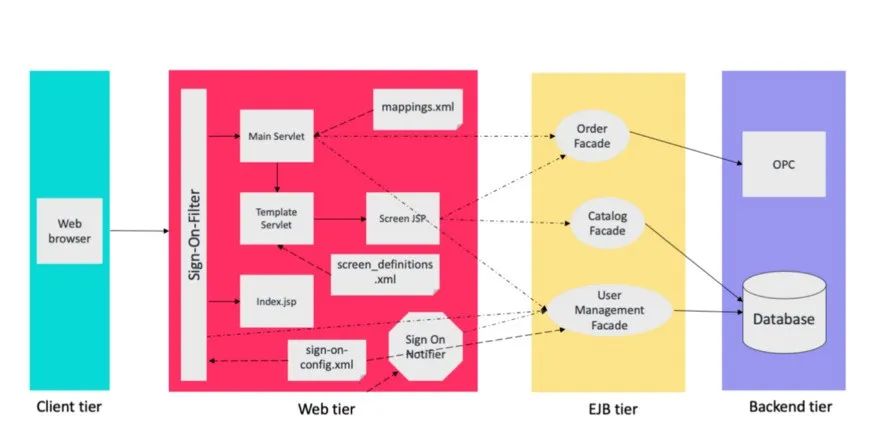
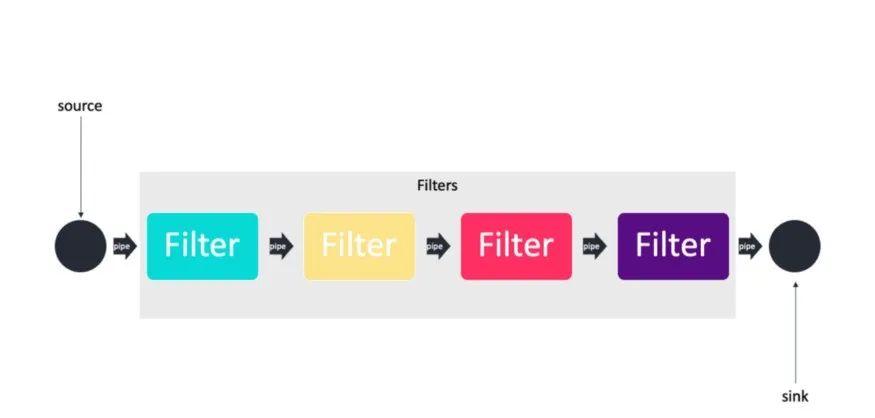
-
producer (source): the starting point of a process.
-
transformer (map): transforms some or all data.
-
tester (reduce): tests one or more conditions.
-
consumer (sink): the endpoint.
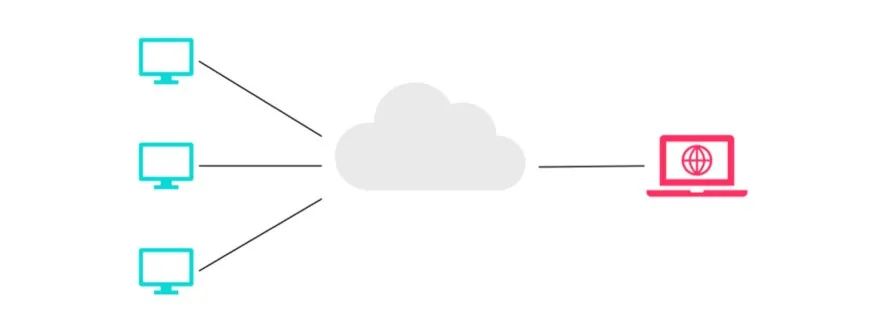
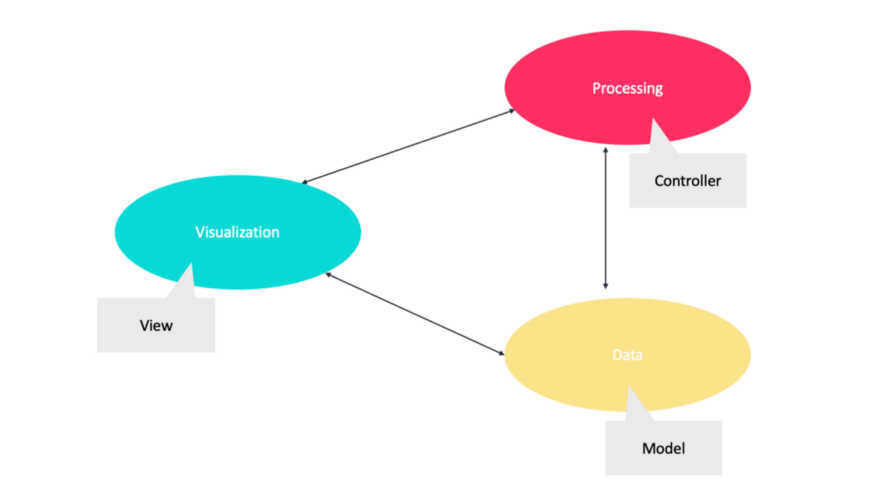
-
Model, which contains the application data.
-
View, which displays part of the underlying data and interacts with the user.
-
Controller, which mediates between the model and the view and manages notifications of state changes.
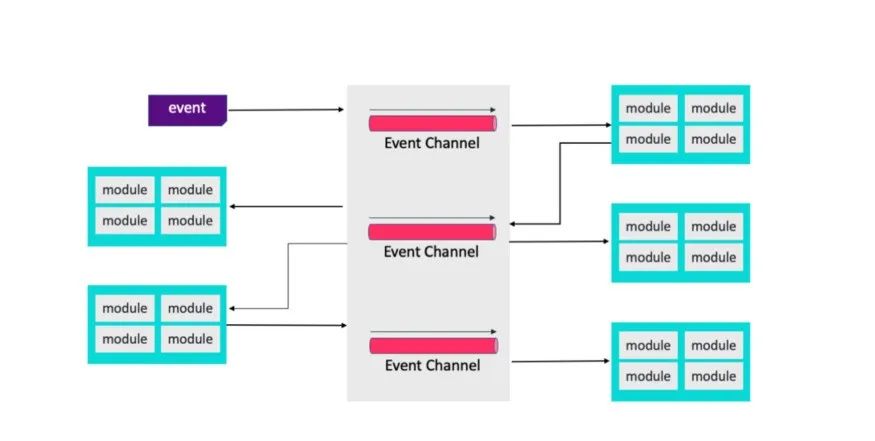
<span>OrderCreated</span>
event.-
Customer Service receives this event and attempts to charge credit for this Order. It then publishes a Credit Reserved event or
<span>CreditLimitExceeded</span>
(exceeded credit limit) event. -
Order Service receives the event sent by Customer Service and changes the order status to approved or canceled.
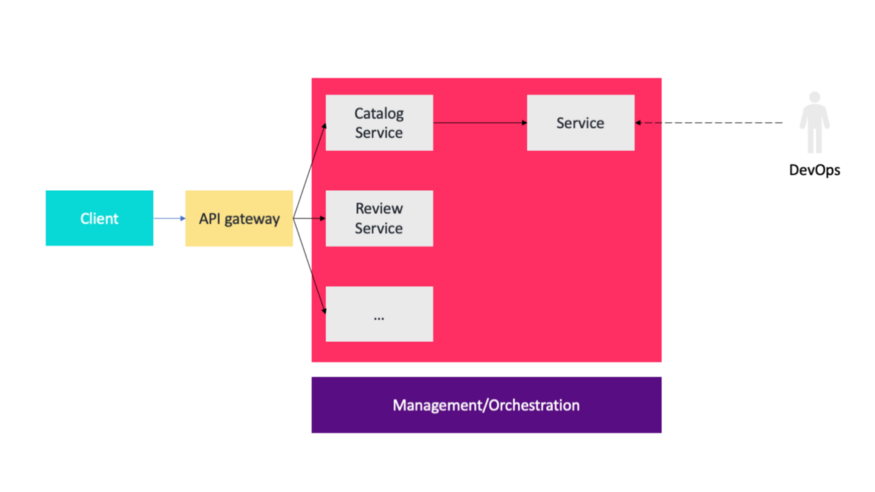