1. The pipeline can only achieve maximum efficiency when filled with instructions, that is, completing the execution of one instruction per clock cycle (only referring to single-cycle instructions).If a jump occurs in the program, the pipeline will be cleared, which will take several clocks to refill. Therefore, minimizing the use of jump instructions can improve program execution efficiency; the solution is to use the “conditional execution” feature of instructions as much as possible.
2. In the LPC2200 series:
You can delay 10 milliseconds using the following program:
for(i=0;i<200;i++){for(j=0;j<200;j++);}
3. Place a 16-bit variable into two 8-bit variables using the following statements.
// IP datagram total length high byte
IpHeadUint8[10]=(IpHead.e_ip.Crc&0xff00)>>8;
// IP datagram total length low byte
IpHeadUint8[11]=IpHead.e_ip.Crc&0x00ff;
4. When initializing all array elements, the array length does not need to be specified.
eg;inta={1,2,3,4,5};
However, when accessing elements beyond a[5], the system will output random values, so this method should not be used to access elements beyond the initialized values.
5. Due to the inherent lack of support for printf in ADS, which is inconvenient for debugging, you can use serial output to replace printf for debugging.
6. Using OR operation can set a specific bit to 1 while keeping other bits unchanged.
eg: PINSEL0 |= 0x00000005; // Set serial port pins
This sets the 0th and 2nd bits to 1, leaving other bits unchanged.
7. Function Pointers
1> In C, the function name directly corresponds to the address of the instruction code generated by the function in memory, so the function name can be directly assigned to a pointer to the function.
2> Calling a function is essentially equivalent to “calling instruction + parameter passing + return address stack”, where the core operation is assigning the address of the target code generated by the function to the CPU’s PC register.
3> Since the essence of function calls is to jump to a specific address to execute code, it is possible to “call a function that does not exist in the function entity at all.”
4> int (*p); defines p as a pointer variable pointing to a function that returns an integer value. The parentheses around *P cannot be omitted, indicating that p is combined with * first, referring to a pointer variable, and then combined with what follows, indicating that this pointer points to a function.
Difference: int *p indicates that the return value of this function is a pointer to an integer variable.
Explanation:
(1) The general definition form of a pointer variable pointing to a function is:
DataType (*PointerVariableName);
1> Here, “DataType” refers to the type of the function’s return value.
(2) A function that returns a pointer:
TypeName *FunctionName(ParameterList)
eg: int * func(int x,int y)
func is the function name, and calling it will return a pointer to integer data. x,y are the parameters of func.
Distinguishing Method:
a. Find the first parenthesis from right to left; what is inside the parenthesis is the function’s parameters.
b. The first identifier outside the parenthesis is the function’s name, and what precedes the function indicates the return value of the function.
8. Array Pointers
1> int (*p)[4]
Indicates that *p has 4 elements, each of which is an integer. This means that p points to an object that has an array of 4 integer elements, i.e., P is a row pointer.
2> Pointer Arrays
Ø An array where each element is of pointer type is called a pointer array; each element in the pointer array is equivalent to a pointer variable.
Ø The definition form of a one-dimensional pointer array is:
TypeName *ArrayName[ArrayLength]
eg: int *p[4]:
Function: It is used to point to several strings, making string processing more convenient and flexible. Suitable for a two-dimensional string array where each row has a different length of character array.
eg: char * name={“Follow me”,”BASIC”,”GreatWall”};
9. Structures
1> Structure variables can be used as actual parameters. However, when using structure variables as actual parameters, the “value-passing” method is adopted, where the contents of the memory units occupied by the structure variable are passed in order to the formal parameters. The formal parameters must also be structure variables of the same type.
eg: pint(su);// note that su is a structure here
Note: This passing method has considerable overhead in space and time, especially when the structure is large.
2> Use a pointer to a structure variable (or array) as an actual parameter, passing the address of the structure variable (or array) to the formal parameter.
eg: print(&su);// note that su is a structure here
10. Unions
1> A union stores different data types in the same memory location. The variables in a union share the same memory.
2> The general form of defining a union type variable is:
union UnionName
{
MemberList;
}VariableList;
3> In a union, the same memory can be used to store different types of data, but at any given time, only one member variable can be stored. The member variable that takes effect in the union variable is the last one stored.
eg: union data{int i;char c;double d;};union data a;
The members i, c, and d of the union variable a are stored in memory starting from the same address. For example, if the following assignments are made:
a.i = 100;
a.c = ‘A’;
Then at this time, the member i of the union variable a no longer has a value because the memory that stored that value has now been used to store the value of member c.
3> The length of a union variable depends on the maximum length of its members:
Explanation:
The length of memory occupied by a structure variable is the sum of the lengths of its members, with each member occupying its own storage space. The length of memory occupied by a union variable is the length of its longest member. Of course, the compiler often performs alignment operations when allocating storage space to improve access efficiency.
Alignment operations are based on the largest basic type. That is, the largest basic type is used as the basic unit. If the actual calculated length is not a multiple of the basic unit, then its actual length should be a multiple of the basic unit.
(In TurboC, no alignment is performed; in Linux, alignment is performed)
11. Handling Inconsistency Between CPU Word Length and Memory Width
For example: using a union to resolve this conflict:
union send_temp{
uint16 words;
uint8 bytes[2];
}send_buff;
eg: send_buff.bytes[0]=a;// here a is 8 bits
send_buff.bytes[1]=b;// here b is 8 bits;
This combines the 8-bit words into a 16-bit word for storage.
When sending, send(send_buff.words) can send a 16-bit data each time.
12. C Language Operator Precedence:
1> Compound assignment operators:
a+=3*5;
is equivalent to a=a+(3*5);
13. A common debugging strategy is to scatter some printf function calls throughout the program to determine the exact location of errors.However, the output of these function calls is written to a buffer and does not immediately display on the screen. In fact, if the program fails, the buffered output may not be written, resulting in an incorrect error location. The solution is to call the fflush function immediately after each printf function used for debugging.
printf("something or other");fflush(stdout);
14. Usage of the keyword volatile
Volatile variables may be used in the following situations:
1> Hardware registers of devices (e.g.: status registers)
2> Global variables that may be accessed in an interrupt service routine
3> Variables shared by several tasks in multithreaded applications.
15. Usage of the keyword register:
When a variable is frequently read and written, it requires repeated access to memory, which consumes a lot of access time. Therefore, C provides a type of variable called a register variable. This variable is stored in the CPU’s register, so when used, it does not need to access memory but directly reads and writes from the register, thus improving efficiency. Register variables are indicated by the keyword register. Loop control variables and variables used repeatedly within the loop are good candidates for defining as register variables.
(1) Only local automatic variables and parameters can be defined as register variables. This is because register variables belong to dynamic storage, and any variable that requires static storage cannot be defined as a register variable, including: global variables between modules, global variables within modules, and local static variables;
(2) Register is a “suggestive” keyword, meaning the program suggests that the variable be stored in the register, but ultimately this variable may not become a register variable due to unsatisfied conditions and may be placed in memory instead, without causing an error in the compiler (in C++, there is another suggestive keyword: inline).
16. For program code that has been burned into FLASH or ROM, we can let the CPU read the code directly from there, but this is usually not a good idea. It is better to copy the target code from FLASH or ROM into RAM after the system starts for improved instruction fetching speed.
The CPU’s access speeds to various memory types are generally:
CPU internal RAM > external synchronous RAM > external asynchronous RAM > FLASH/ROM
17. Macro Definitions
In C, macros are the only way to generate inline code. For embedded systems, macros are a great substitute for functions to achieve performance requirements.
1> Macro definitions “look like” functions;
2> Macro definitions are not functions, so all “parameters” must be enclosed in parentheses;
3> Macro definitions may have side effects. Therefore, do not pass parameters with side effects to macro definitions.
To help more people who want to transition into the internet industry understand the technical development prospects, employment directions, and salary levels in the IT industry,Datane has opened 27 popular internet technology courses for free! Master the latest trends!5 days free listening, You can listen first and then decide!
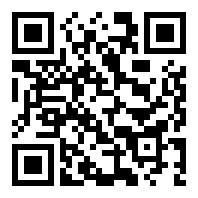
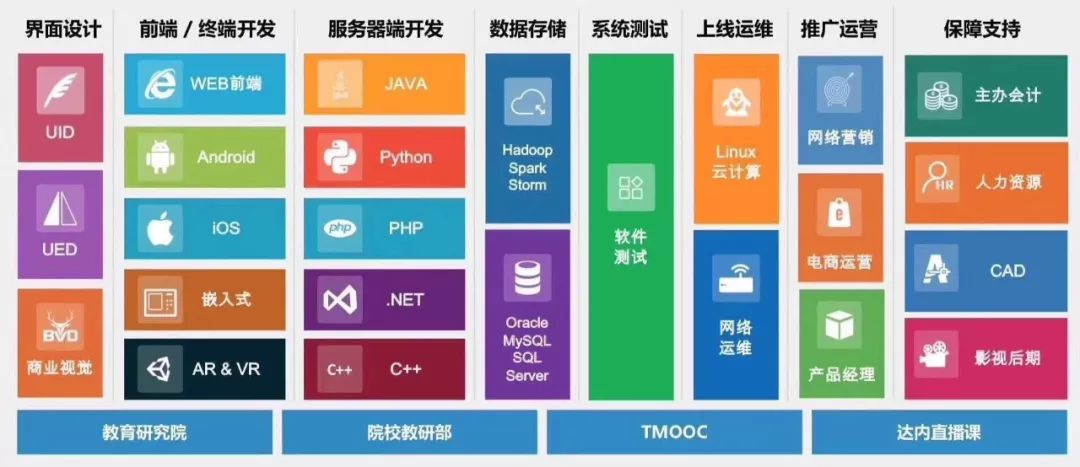
Recommended Content:
What IT technologies have good salaries but no educational requirements?
Essential skills for various positions in the IT industry, a must-read for entering the field!
Review! The top 10 IT skills in the 2019 recruitment ranking!
Datane Education provides free courses nationwide! (Apply in the text)
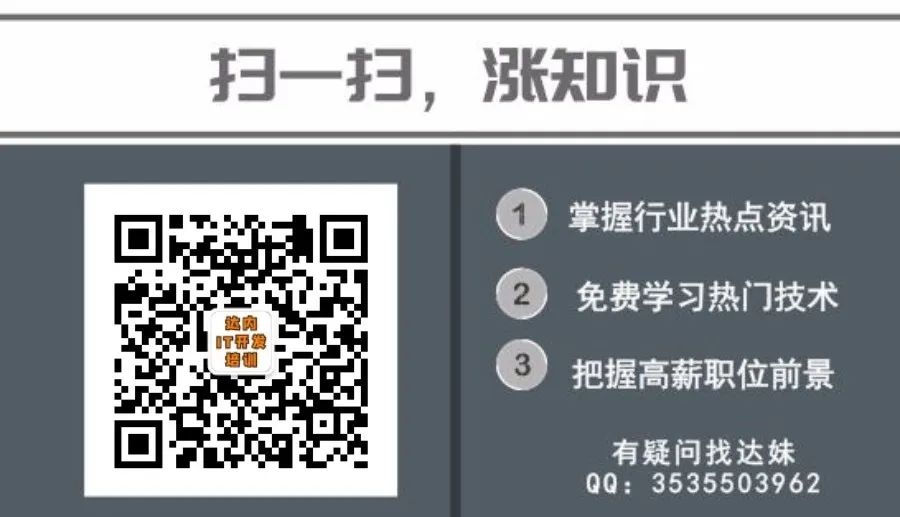