What is a framework?
A program framework is similar to a file outline or template. Writing a program is like writing an article; without an outline or template, it can be quite cumbersome.
Why Have a Framework?
To save time and reduce errors. For a specific type of program, the logical structure of the code is often the same, with many similarities or commonalities. By extracting these common elements into a fixed program framework, we can reuse this framework when developing new programs of the same type.
This greatly improves our development efficiency, and since the framework is public and maintained by many users, it makes the code logic less prone to errors.
Components of Embedded Systems
Embedded control systems are generally composed of “ordinary tasks” and “interrupt tasks”.
-
• Ordinary tasks: Tasks that do not have high time response requirements or are executed periodically;
-
• Interrupt tasks: Tasks that require immediate processing due to high time response requirements;
Common Frameworks
1. Polling without Interrupts
Description: All tasks are executed in order. To reduce the overall system response time, there are two methods:
-
1. Do not use waiting delay functions in tasks;
-
2. If a task cannot be completed in one go, it should be broken down into several smaller tasks, executing one small task in a loop until the task is complete; both methods will consume memory, and what could be handled with local variables now can only be managed with static or global variables.
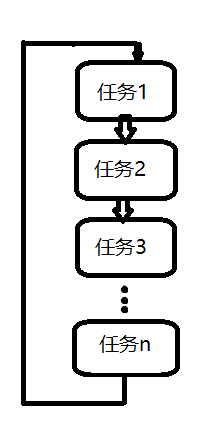
Advantages: The program execution flow is simple and clear;
Disadvantages: It is very inconvenient to modify the system’s functionality, and if the number of tasks increases, it will affect the overall system’s response time, making it feel sluggish;
Pseudocode Implementation:
int main(void)
{
while(1)
{
doSomething_1(); // Task 1
doSomething_2(); // Task 2
doSomething_3(); // Task 3
/* Other tasks */
}
return 0;
}
2. Only Interrupts
Description: In a system with “only interrupts”, the main function’s loop does nothing.
Advantages: Can respond in real-time to exceptional tasks (events).
Disadvantages: Interrupt resources are limited, and when there are too many tasks, responses may be delayed.
Pseudocode Implementation:
int main(void)
{
while(1)
{
;
}
}
/* Interrupt Service Function 1 */
void ISR1_IRQHandler(void)
{
doSomething_1();
}
/* Interrupt Service Function 2 */
void ISR2_IRQHandler(void)
{
doSomething_2();
}
3. Variants of the Only Interrupt Framework
Description: Uses a state machine mechanism to execute tasks. The interrupt function sets the state of the state machine, while the main function’s loop executes different tasks based on different state values. This does not truly belong to the “only interrupts” form.
int main(void)
{
while(1)
{
if(flag_1)
{
doSomething_1();
}
if(flag_2)
{
doSomething_2();
}
if(flag_3)
{
doSomething_3();
}
/* Other tasks */
}
return 0;
}
/********* Interrupt Service Function 1 ************/
void ISR1_IRQHandler(void)
{
flag_1 = ~flag_1;
}
/********* Interrupt Service Function 2 ************/
void ISR2_IRQHandler(void)
{
flag_2 = ~flag_2;
}
/********* Interrupt Service Function 3 ************/
void ISR3_IRQHandler(void)
{
flag_3 = ~flag_3;
}
4. Polling with Interrupts
Description: Places some periodic tasks in the main function’s loop.
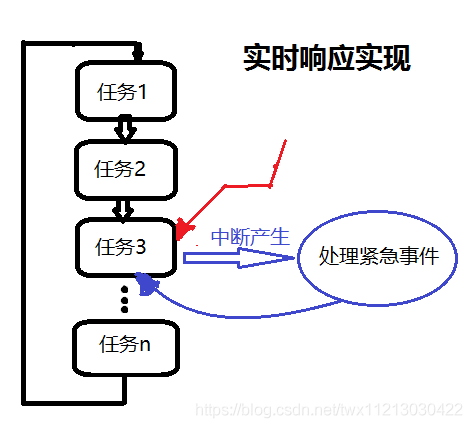
Advantages: Reasonably utilizes resources by separating regular tasks from urgent tasks.
Disadvantages: The program structure and logic are relatively complex, requiring significant effort in task allocation and collaboration.
Pseudocode Implementation:
int main(void)
{
while(1)
{
if(flag_1)
{
doSomething_1();
}
if(flag_2)
{
doSomething_2();
}
if(flag_3)
{
doSomething_3();
}
/* Other tasks */
}
return 0;
}
/********* Timer Interrupt Service Function ************/
void ISR1_IRQHandler(void)
{
}
5. Polling with Interrupts – Virtual Timer
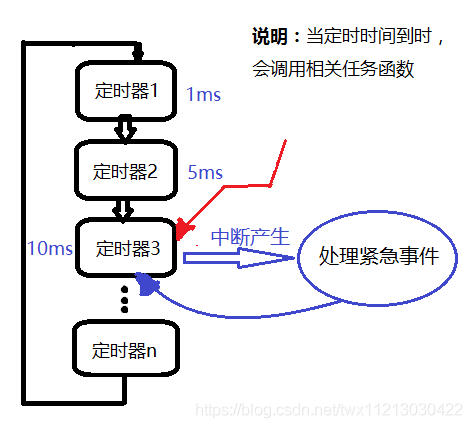
Description: Uses different “virtual timer” timing to invoke different tasks. When the timer’s timing expires, it executes a callback function or calls the task function.
This is very suitable for periodic tasks, while interrupts can handle external sudden events. This ensures real-time performance, but care must be taken to avoid using waiting delays. Generally, the time base for virtual timers is 1ms.
Advantages: The time interval for tasks can be controlled relatively accurately, and the overall system’s real-time performance is good due to the use of interrupts.
Disadvantages: The execution time of tasks cannot be controlled; if the execution time of a timed task is too long, it will affect the timing accuracy of the virtual timer.
Virtual timer implementation code:
https://codeload.github.com/0x1abin/MultiTimer/zip/master
6. Non-Preemptive Real-Time Operating System
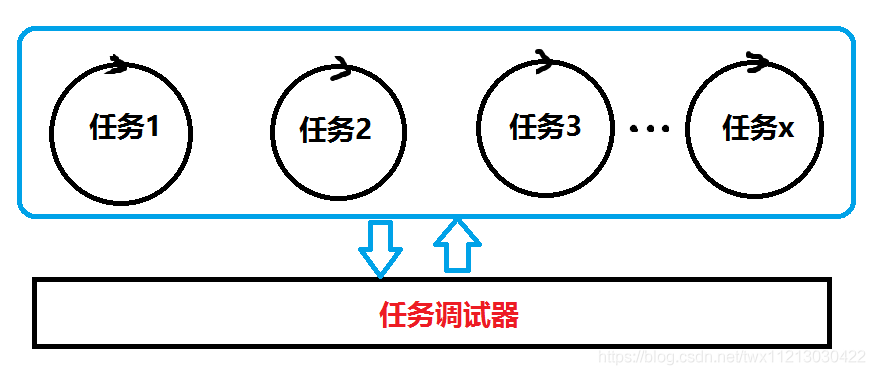
Description: There are no priority distinctions between tasks; each task is executed sequentially. However, the execution time of tasks is strictly controlled by the operating system. Even if a task is not completed, it will be suspended when the time slice expires.
Advantages: There is no need to carefully reduce delays in tasks; we can focus our energy on business logic.
Disadvantages: Tasks are on the same level, which can lead to some tasks not being processed urgently.
Pseudocode implementation:
https://blog.csdn.net/twx11213030422/article/details/104637273
7. Preemptive Real-Time Operating System
Description: Each task is in a “dead loop” while having a priority. High-priority tasks can interrupt low-priority tasks, similar to interrupts. Thus, the overall system’s real-time performance is excellent, and each task is also controlled by time slices, meaning their execution times can be predicted. It also supports interrupts to respond to urgent events.
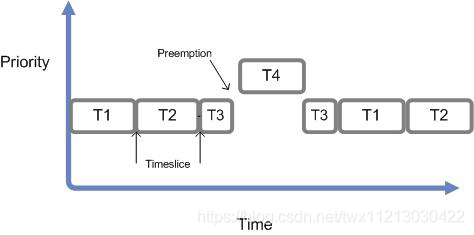
Advantages: Real-time response, allowing engineers to focus on implementing business logic.
Disadvantages: Requires porting and has certain hardware resource requirements for microcontrollers.
Common Preemptive Real-Time Operating Systems: Keil RTX, FreeRTOS, uCosII/III, etc.
Source:https://blog.csdn.net/twx11213030422
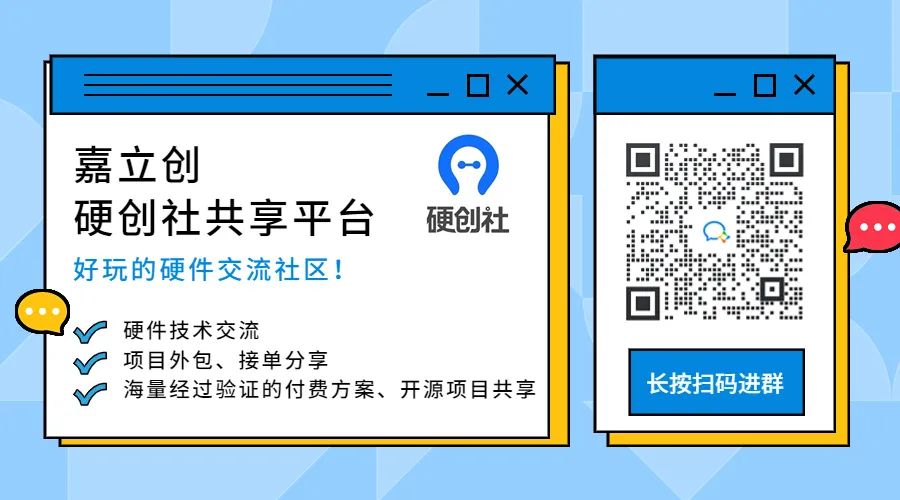