Introduction
When I first started working with STM32, I used Keil as my IDE. Having previously used VS and Eclipse, I found the basic features of the Keil editor to be extremely poor, making the coding experience very frustrating.
I then tried various IDEs, using Eclipse with Keil, but found Eclipse’s support for C language to be lacking. Using emBits with GCC was cumbersome if I needed to collaborate with others. Eventually, I discovered PlatformIO, which also uses GCC as the compiler but only supports HAL libraries. An important reason for not using GCC was that all my colleagues were using Keil, so I couldn’t collaborate effectively if I switched to GCC.
Finally, by using VS Code with Keil, I resolved the issues of coding experience and project collaboration. There are many tutorials online for using VS Code as an editor and Keil as a compiler, but they typically require editing in VS Code and then compiling, downloading, and debugging in Keil. This article aims to achieve editing, compiling, downloading, and debugging all within VS Code.
Part 1Environment
(1) VS Code; (2) Keil; Python; (3) GNU Arm Embedded Toolchain (ARM GCC toolchain); (4) C/C++ (VS Code plugin); (5) Cortex-Debug (VS Code plugin); (6) Other VS Code plugins (to enhance experience).
Part 2Prerequisites
Before writing code, you first need to set up a project, which needs to be done in Keil, including project configuration, file addition…
Part 3Editing
After installing the VS Code plugins, the experience of writing C code in VS Code is already quite good.
However, since we are using the Keil environment, we need to configure header file includes, macro definitions, etc. Open the c_cpp_properties.json file in the .vscode folder under the project path, or create one if it doesn’t exist. The content configuration is as follows:
{
"configurations": [
{
"name": "STM32",
"includePath": [
"D:/Program Files/MDK5/ARM/ARMCC/**",
"${workspaceFolder}/**",
""
],
"browse": {
"limitSymbolsToIncludedHeaders": true,
"databaseFilename": "${workspaceRoot}/.vscode/.browse.c_cpp.db",
"path": [
"D:/Program Files/MDK5/ARM/ARMCC/**",
"${workspaceFolder}/**",
""
]
},
"defines": [
"_DEBUG",
"UNICODE",
"_UNICODE",
"__CC_ARM",
"USE_STDPERIPH_DRIVER",
"STM32F10X_MD"
],
"intelliSenseMode": "msvc-x64"
}
],
"version": 4
}
In this, you need to add header file paths in includePath
and path
. ${workspaceFolder}/**
is the project path and should not be modified. You also need to add the Keil header file paths.
Then add macros in defines
, which are the macros configured in the C++ tab of Keil’s Options for Target. This allows you to experience the powerful code suggestions and function jump features of VS Code (leaving Keil’s editor in the dust).
Part 4Compiling and Flashing
Compiling and flashing are achieved through the Task feature of VS Code, using the command line to call Keil for compiling and flashing.
Keil itself supports command line calls, which can be referenced in the Keil manual, so I won’t elaborate here. However, the problem is that when calling Keil from the command line, regardless of the operation, its output will not show up in the console!!! (What’s the use of having command line support?)
Fortunately, Keil supports output to a file, so we can utilize this to perform some tricks. By executing commands and simultaneously reading the file content and printing it to the console, we can achieve console output, allowing us to see the compilation process directly in VS Code.
For this, I wrote a Python script to implement the command line call to Keil and simultaneously read the file output to the console.
#!/usr/bin/python
# -*- coding:UTF-8 -*-
import os
import threading
import sys
runing = True
def readfile(logfile):
with open(logfile, 'w') as f:
pass
with open(logfile, 'r') as f:
while runing:
line = f.readline(1000)
if line != '':
line = line.replace('\', '/')
print(line, end = '')
if __name__ == '__main__':
modulePath = os.path.abspath(os.curdir)
logfile = modulePath + '/build.log'
cmd = '"D:/Program Files/MDK5/UV4/UV4.exe" '
for i in range(1, len(sys.argv)):
cmd += sys.argv[i] + ' '
cmd += '-j0 -o ' + logfile
thread = threading.Thread(target=readfile, args=(logfile,))
thread.start()
code = os.system(cmd)
runing = False
thread.join()
sys.exit(code)
This script needs to be run in conjunction with VS Code’s Task. By configuring the Task, we also need to match the error messages in the output (compilation errors) so that clicking on errors in Keil will directly jump to the corresponding line of code. For specific configuration, please refer to the VS Code documentation; here is my Task configuration.
{
// See https://go.microsoft.com/fwlink/?LinkId=733558
// for the documentation about the tasks.json format
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "py",
"args": [
"-3",
"${workspaceFolder}/scripts/build.py",
"-b",
"${config:uvprojxPath}"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": [
{
"owner": "c",
"fileLocation": [
"relative",
"${workspaceFolder}/Project"
],
"pattern": {
"regexp": "^(.*)\((\d+)\):\s+(warning|error):\s+(.*):\s+(.*)$",
"file": 1,
"line": 2,
"severity": 3,
"code": 4,
"message": 5
}
}
]
},
{
"label": "rebuild",
"type": "shell",
"command": "py",
"args": [
"-3",
"${workspaceFolder}/scripts/build.py",
"-r",
"${config:uvprojxPath}"
],
"group": "build",
"problemMatcher": [
{
"owner": "c",
"fileLocation": [
"relative",
"${workspaceFolder}/Project"
],
"pattern": {
"regexp": "^(.*)\((\d+)\):\s+(warning|error):\s+(.*):\s+(.*)$",
"file": 1,
"line": 2,
"severity": 3,
"message": 4,
}
}
]
},
{
"label": "download",
"type": "shell",
"command": "py",
"args": [
"-3",
"E:\Work\Store\MyWork\STM32F1\FreeModbus_M3\scripts\build.py",
"-f",
"${config:uvprojxPath}"
],
"group": "test"
},
{
"label": "open in keil",
"type": "process",
"command": "${config:uvPath}",
"args": [
"${config:uvprojxPath}"
],
"group": "test"
}
]
}
For projects compiled with ARM Compiler 6, the problemMatcher in build and rebuild should be configured as follows:
"problemMatcher": [
{
"owner": "c",
"fileLocation": ["relative", "${workspaceFolder}/MDK-ARM"],
"pattern": {
"regexp": "^(.*)\((\d+)\):\s+(warning|error):\s+(.*)$",
"file": 1,
"line": 2,
"severity": 3,
"message": 4,
}
}
]
The config:uvPath
and config:uvprojxPath
in the file correspond to the path of Keil’s UV4.exe file and the project path (.uvprojx), which can be directly modified to specific paths or added in VS Code’s setting.json
file. Thus, we have perfectly achieved editing, compiling, and downloading in VS Code.
Compilation output:

Output when there are errors:
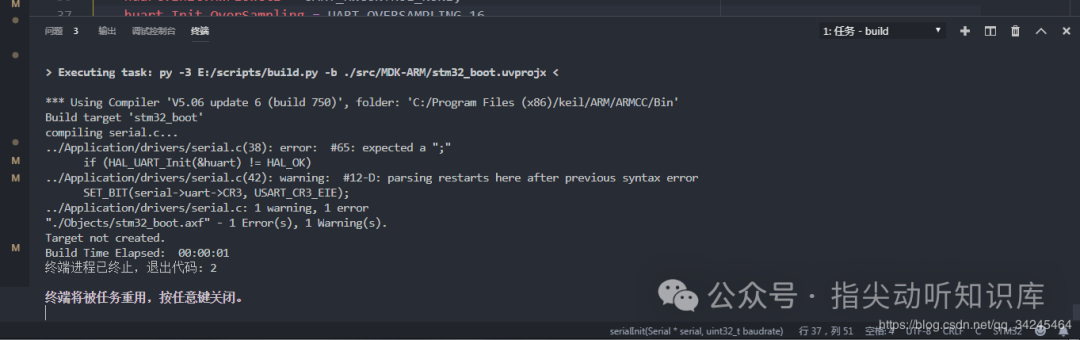
Error matching:
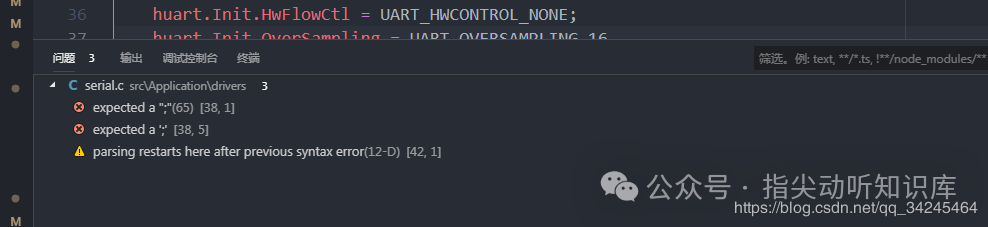
Part 5Debugging
Debugging requires the use of the Cortex-Debug plugin and the ARM GCC toolchain. This part can refer to the documentation for Cortex-Debug, which is quite detailed.
First, install the Cortex-Debug plugin and the ARM GCC toolchain, then configure the environment path. If using Jlink for debugging, download the Jlink suite, install it, and find the JLinkGDBServerCL.exe program. In VS Code settings, add “cortex-debug.JLinkGDBServerPath
“: “C:/Program Files (x86)/SEGGER/JLink/JLinkGDBServerCL.exe
“; the path after is your own path.
Additionally, for configuring the ARM GCC toolchain: “cortex-debug.armToolchainPath
“: “D:\Program Files (x86)\GNU Arm Embedded Toolchain\9 2020-q2-update\bin”; the path after is your own path. If using STLink for debugging, download the stutil tool, which can be found on GitHub, and configure the path accordingly.
After completing the above steps, you can directly click the debug button in VS Code, which will create a launch.json file, the debug configuration file for VS Code. You can refer to my file for configuration.
{
// Use IntelliSense to learn about related properties.
// Hover to view descriptions of existing properties.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"name": "Cortex Debug(JLINK)",
"cwd": "${workspaceRoot}",
"executable": "${workspaceRoot}/Project/Objects/Demo.axf",
"request": "attach",
"type": "cortex-debug",
"servertype": "jlink",
"device": "STM32F103C8",
"svdFile": "D:\Program Files\ARM\Packs\Keil\STM32F1xx_DFP\2.3.0\SVD\STM32F103xx.svd",
"interface": "swd",
"ipAddress": null,
"serialNumber": null
},
{
"name": "Cortex Debug(ST-LINK)",
"cwd": "${workspaceRoot}",
"executable": "${workspaceRoot}/Project/Objects/Demo.axf",
"request": "attach",
"type": "cortex-debug",
"servertype": "stutil",
"svdFile": "D:\Program Files\ARM\Packs\Keil\STM32F1xx_DFP\2.3.0\SVD\STM32F103xx.svd",
"device": "STM32F103C8",
"v1": false
}
]
}
Note the places that need to be modified: executable
should be changed to your project’s generated target file, which is the project’s .axf
file. svdFile
is for monitoring the MCU peripherals, and this file can be found in the Keil installation path. You can refer to my path to locate it. After configuration, click the debug button again to start debugging.
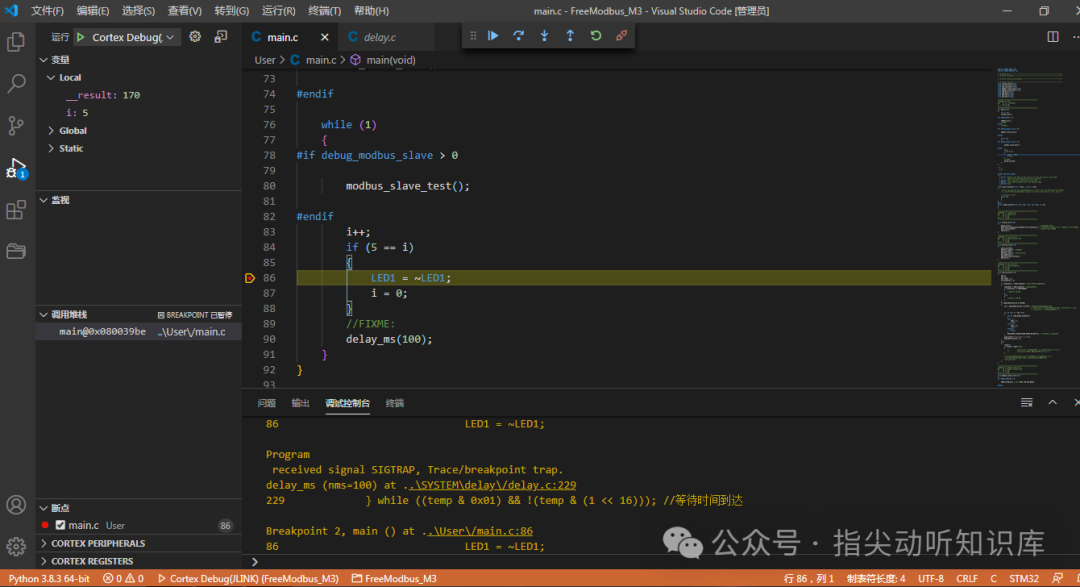
Compared to Keil’s own debugging features, VS Code also supports conditional breakpoints, allowing you to set hit conditions and counts, which greatly facilitates debugging.
Conclusion
Through the above configuration, we basically do not need to open Keil except for creating projects and adding files to projects. So it can be said, goodbye, dumb Keil!
Source:http://b.mtw.so/5W5Xlt
From zsky We Learn Embedded together WeChat Official Account