#define KEY_MIN 0X01
#define KEY_PLUS 0X01
unsigned char KeyDat;
void ReadPort(void) {
if (P1 & KEY_PLUS == 0) {
KeyDat |= 0x01;
}
if (P2 & KEY_MIN == 0) {
KeyDat |= 0x02;
}
}
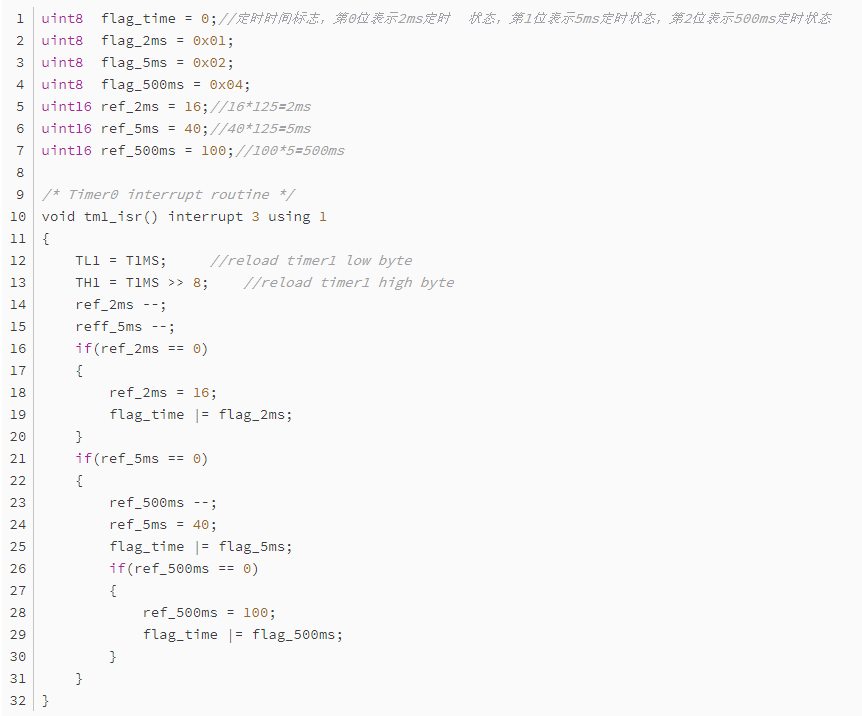
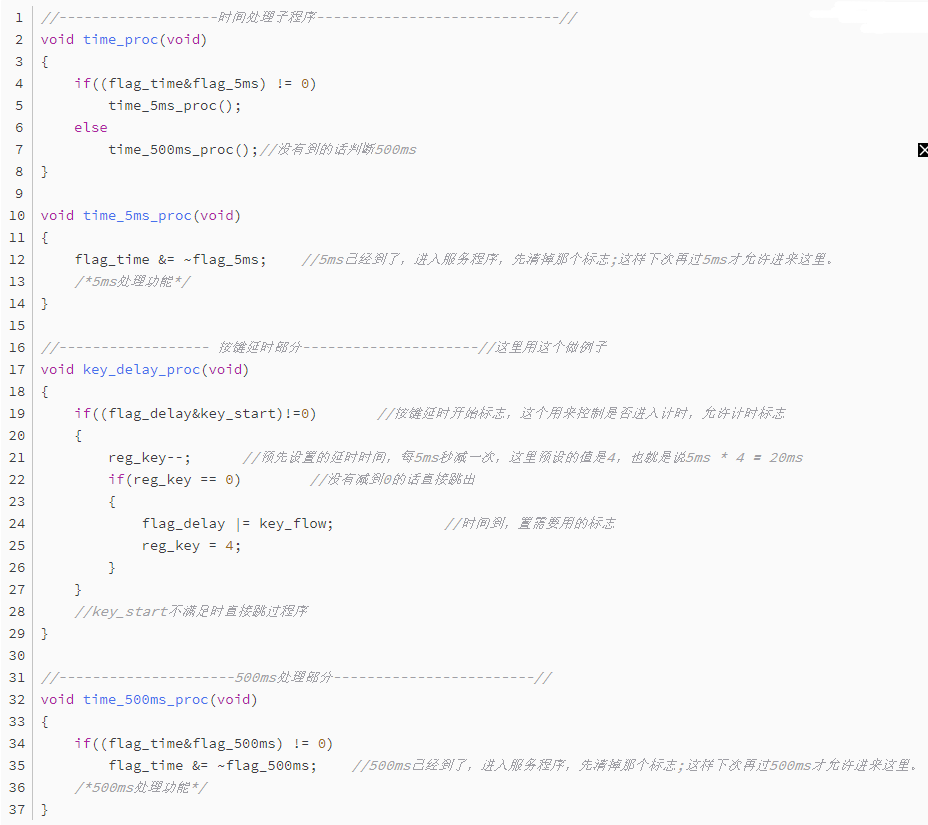
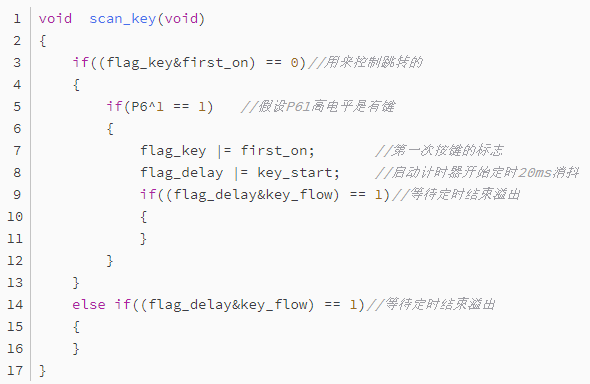
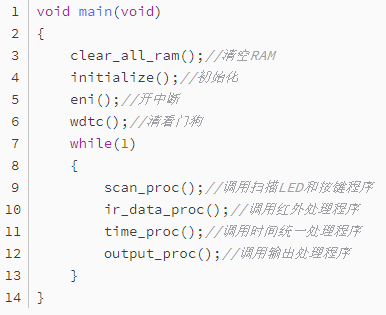
Disclaimer:This article is reprinted from the internet, and the copyright belongs to the original author. If there are any copyright issues, please contact us in a timely manner. Thank you!
Collection of Past Excellent Articles
Finally
Long press the QR code in the picture to follow