Introduction
As a qualified programmer, it is impossible not to write bugs. How can we spend the least amount of time fixing bugs?
By using some static analysis tools during the coding phase, we can often achieve more with less effort and reduce the bugs in our code.
Static analysis tools can analyze source code without executing it, identifying bugs in the code. In C/C++ programs, static analysis tools can detect program errors such as null pointer dereferencing, memory leaks, division by zero, integer overflow, out-of-bounds access, and using variables before initialization.
Static Analysis in Compilers
The goal of a compiler is to generate an executable file, so they do not focus on static code analysis.
However, as compilers gradually improve, they are also getting better at static analysis.
For example, when we compile code, sometimes the compiler generates many annoying warnings. Most of the time, these warnings do not affect the program. Therefore, many people tend not to pay attention to these warnings.
However, we should fully trust the compiler. After all, no one understands this language better than the compiler.
Thus, we must take some time to carefully check the warnings generated by the compiler. This is much cheaper than spending several hours or even days trying to resolve a bug.
For example, look at the code below. Do you think it will print “ON” or “OFF”?
#include <stdio.h>
#define ON 0xFF
#define OFF 0x00
void print_message(char status)
{
if (status == ON)
printf("ON\n");
else
printf("OFF\n");
}
int main(int argc, const char *argv[])
{
print_message(ON);
return 0;
}
$ gcc main.c -o main
$ ./main
OFF!
The result is surprising! I also mistakenly thought this code would print “ON” the first time.
What about if we compile with Clang?
Clang main.c -o main
main.c:8:16: warning: comparison of constant 255 with expression of type 'char' is always
false [-Wtautological-constant-out-of-range-compare]
if (status == ON)
~~~~~~ ^ ~~~
1 warning generated.
Clang is an excellent static analyzer that can analyze potential issues in the code. For the above issue, GCC can also detect the bug by adding the -Wall and -Wpedantic compilation options.
$ gcc main.c -o main -Wall -Wpedantic
main.c: In function ‘main’:
main.c:3:13: warning: overflow in implicit constant conversion [-Woverflow]
#define ON 0xFF
^
main.c:16:19: note: in expansion of macro ‘ON’
print_message(ON);
^
However, the main task of Clang and GCC is to compile code, and static analysis is not always necessary during each compilation. Additionally, compilers require a lot of time to perform static analysis. This is why we need a dedicated static code analysis tool.
A list of static code analysis tools is provided on Wikipedia (https://en.wikipedia.org/wiki/List_of_tools_for_static_code_analysis). Cppcheck is one of the best open-source static analysis tools.
Introduction to Cppcheck
Cppcheck is a static analysis tool for C/C++ code focused on detecting undefined behaviors and dangerous coding practices. For example, null pointers, division by zero, integer overflow, invalid shift operations, invalid conversions, invalid uses of STL, memory management issues, null pointer dereferences, out-of-bounds checks, uninitialized variables, unused or duplicate code, etc.
Cppcheck is an open-source project hosted on Sourceforge and GitHub, supporting GNU/Linux, Windows, and Mac OS operating systems.
Installing Cppcheck
You can install Cppcheck online with the following command.
sudo apt install cppcheck
You can also download the source code and compile it manually:
wget https://github.com/danmar/cppcheck/archive/1.90.tar.gz
$ tar xfv 1.90.tar.gz
$ cd cppcheck-1.90/
$ make MATCHCOMPILER=yes FILESDIR=/usr/share/cppcheck HAVE_RULES=yes -j4
$ sudo make MATCHCOMPILER=yes FILESDIR=/usr/share/cppcheck HAVE_RULES=yes install
$ cppcheck --version
Cppcheck 1.90
Using Cppcheck to Analyze Code
Example 1
Below, we will introduce how to use Cppcheck through an example. Can you find the two bugs in the following code?
void calc(void)
{
int buf[10] = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
int result;
int i;
for (i = 0; i <= 10; i++) {
result += buf[i];
}
}
int main(void)
{
calc();
return 0;
}
Using GCC to compile the code does not generate any warnings or errors.
$ gcc -Wall -Wextra -Werror -Wpedantic main.c -o main
$ ls main
main
Clang detected one of the bugs.
$ clang -Wall -Wextra -Werror -Wpedantic -Weverything main.c -o main
main.c:8:9: error: variable 'result' is uninitialized when used here [-Werror,-Wuninitialized]
result += buf[i];
^~~~~~
main.c:4:15: note: initialize the variable 'result' to silence this warning
int result;
^
= 0
1 error generated.
Cppcheck found all the bugs.
$ cppcheck main.c
Checking main.c ...
main.c:8:22: error: Array 'buf[10]' accessed at index 10, which is out of bounds. [arrayIndexOutOfBounds]
result += buf[i];
^
main.c:8:9: error: Uninitialized variable: result [uninitvar]
result += buf[i];
^
Example 2
Let’s analyze Busybox with cppcheck to see what results we get.
$ wget https://busybox.net/downloads/busybox-1.31.1.tar.bz2
$ tar xfv busybox-1.31.1.tar.bz2
$ cd busybox-1.31.1/
$ cppcheck . 2>&1 | tee cppcheck.log
...
$ cat cppcheck.log | grep error | wc -l
146
The latest version of Busybox has over 140 potential bugs (as of when I wrote this article). Some errors may be false positives, but a few are worth analyzing.
$ cat cppcheck.log | grep "Uninitialized variable"
archival/libarchive/bz/blocksort.c:1034:20: error: Uninitialized variable: origPtr [uninitvar]
archival/libarchive/bz/compress.c:235:18: error: Uninitialized variable: ll_i [uninitvar]
archival/libarchive/bz/compress.c:679:20: error: Uninitialized variable: origPtr [uninitvar]
archival/libarchive/decompress_bunzip2.c:165:20: error: Uninitialized variable: runCnt [uninitvar]
console-tools/loadfont.c:146:6: error: Uninitialized variable: height [uninitvar]
...
$ cat cppcheck.log | grep "out of bounds"
util-linux/fdisk_sgi.c:138:10: error: Array 'freelist[17]' accessed at index 17, which is out of bounds. [arrayIndexOutOfBounds]
util-linux/fdisk_sgi.c:138:10: note: Array index out of bounds
util-linux/fdisk_sgi.c:139:10: error: Array 'freelist[17]' accessed at index 17, which is out of bounds. [arrayIndexOutOfBounds]
util-linux/volume_id/iso9660.c:114:15: error: Buffer is accessed out of bounds: hs->id [bufferAccessOutOfBounds]
...
$ cat cppcheck.log | grep "Resource leak"
scripts/kconfig/confdata.c:376:4: error: Resource leak: out [resourceLeak]
Cppcheck Extension Plugins
Cppcheck can also be extended by creating new check rules using regular expressions, or even by using modules written in Python.
Additionally, there are cppcheck plugins for several popular development tools such as Eclipse, Visual Studio, Code::Blocks, Sublime Text, and QtCreator.
Vim editor plugin link: https://github.com/vim-syntastic/syntastic
Conclusion
Static code analysis may sometimes produce false positives, but cppcheck strikes a good balance between real bugs and false positives.
Therefore, it is recommended that everyone integrate the cppcheck static analysis tool into their development tools. While it may not solve all your problems, it will certainly help improve the quality of your code and reduce the time you spend fixing bugs.
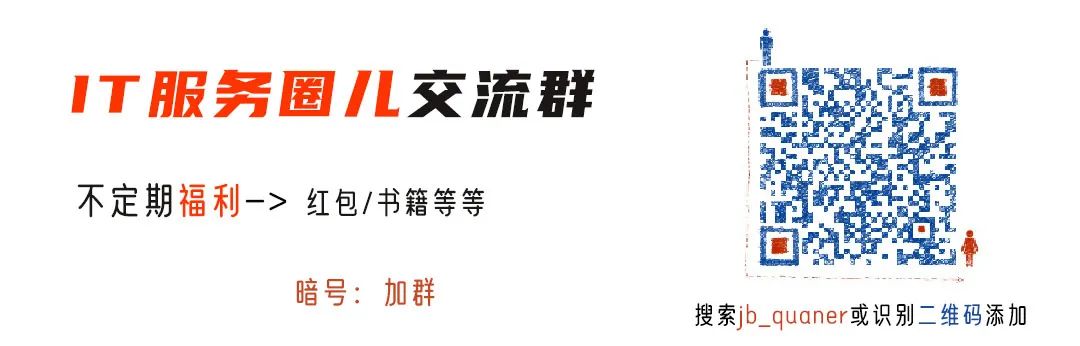
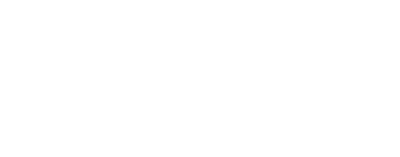