STM32’s standard peripheral library, HAL, and LL software libraries all have many clever aspects worth learning from.
Today, I will talk about how the STM32Cube LL library cleverly uses “static inline” to make the code more efficient.
Some applications require the MCU to handle tasks efficiently, especially when running algorithms that demand high CPU execution efficiency.
Many articles online claim that STM32Cube HAL has low execution efficiency and large code size, which leads to various doubts among many beginners or those who have not yet started.
To be honest, HAL does have issues with low code efficiency and large code size compared to the standard peripheral library, while the corresponding STM32Cube LL library avoids these problems.
2Reasons for LL’s Efficiency
In summary: clever use of C language static and inline functions to directly operate registers.
Of course, this is one of the important reasons, and there are other reasons that will not be described here.
You will find many functions in the LL library .h files that directly read and write registers using static and inline functions.
For example, reading and writing IO ports:
__STATIC_INLINE uint32_t LL_GPIO_ReadOutputPort(GPIO_TypeDef *GPIOx){ return (uint32_t)(READ_REG(GPIOx->ODR));}
__STATIC_INLINE void LL_GPIO_SetOutputPin(GPIO_TypeDef *GPIOx, uint32_t PinMask){ WRITE_REG(GPIOx->BSRR, (PinMask >> GPIO_PIN_MASK_POS) & 0x0000FFFFU);}
Among them, __STATIC_INLINE refers to static and inline:
#define __STATIC_INLINE static __inline
And the definition for reading and writing bits:
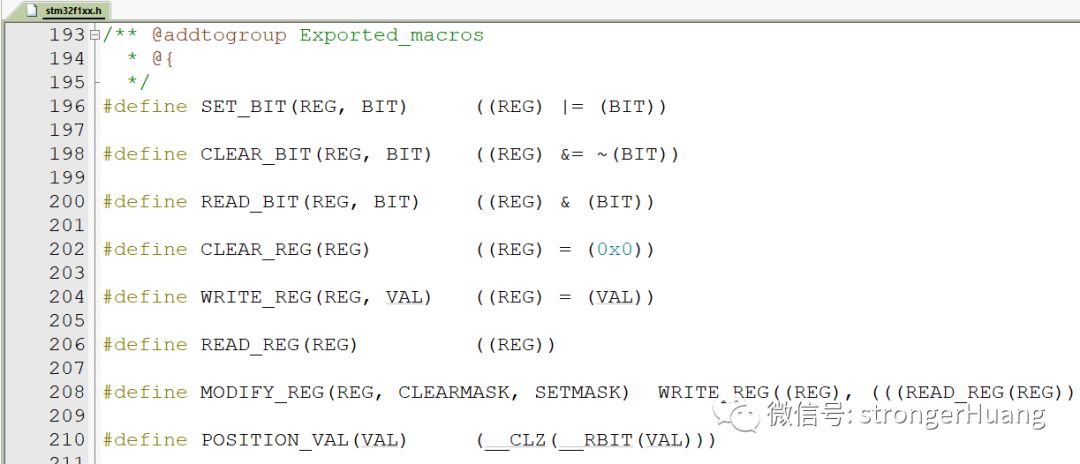
The macro definitions here are called in many peripheral .h files. For example, enabling USART:
LL library enables USART:
__STATIC_INLINE void LL_USART_Enable(USART_TypeDef *USARTx){ SET_BIT(USARTx->CR1, USART_CR1_UE);}
Standard peripheral library enabling USART:
void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState){ /* Check the parameters */ assert_param(IS_USART_ALL_PERIPH(USARTx)); assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE) { /* Enable the selected USART by setting the UE bit in the CR1 register */ USARTx->CR1 |= USART_CR1_UE; } else { /* Disable the selected USART by clearing the UE bit in the CR1 register */ USARTx->CR1 &= (uint16_t)~((uint16_t)USART_CR1_UE); }}
By comparing, you will clearly find that LL library has higher execution efficiency.
3What are Inline Functions?
At this point, some readers may ask: what are inline functions?
Inline functions are a programming language structure used to suggest the compiler to inline some special functions.
— From Baidu Encyclopedia
Typically, when a program executes, the processor reads code from memory. When a function is called in the program, the program jumps to the location in memory where the function is stored, starts reading the code, and returns after execution.
To improve speed, the C language defines inline functions, telling the compiler to directly copy the function code into the program during compilation, so there is no need to read the function code separately during execution.
Note:When inline functions are large, it can have the opposite effect, so generally only smaller functions use inline functions.
4Software Framework Thinking
LL is efficient because it cleverly uses some C language knowledge without much encapsulation, directly or indirectly operating on registers.
And this is made possible by the ST development team designing such an intermediate layer software framework.
For those with experience in large project development, the framework of a project has a significant impact on the entire project.
It’s like building a building; if the frame of the floors is not constructed properly, do you think the quality of the building will be good?
Therefore, it is mentioned that when programming, especially for larger projects, one needs to consider the software framework; a good framework can greatly enhance the efficiency of your project.
Copyright belongs to the original author. If there is any infringement, please contact for deletion.
Why does the CPU with 8 cores struggle with the first core?
God’s explanation: The four major communication interfaces: UART, I2C, SPI, 1-wire
Why are Chinese numbers four digits per increment, while Western numbers are three digits per increment?
→ Follow to avoid getting lost ←