Original link: https://zhuanlan.zhihu.com/p/394967586
Kubectl Common Command Guide
Kubectl commands are the most direct way to operate a Kubernetes cluster, especially for operations personnel, who need to have a detailed grasp of these commands.
Kubectl Autocomplete
# setup autocomplete in bash, bash-completion package should be installed first.
$ source <(kubectl completion bash) # setup autocomplete in zsh
$ source <(kubectl completion zsh)
Kubectl Context and Configuration
# Display merged kubeconfig configuration
$ kubectl config view
# Use multiple kubeconfig files and view the merged configuration
$ KUBECONFIG=~/.kube/config:~/.kube/kubconfig2 kubectl config view
# Get password for e2e user
$ kubectl config view -o jsonpath='{.users[?(@.name == "e2e")].user.password}'
# Display current context
$ kubectl config current-context
# Set default context to my-cluster-name
$ kubectl config use-context my-cluster-name
# Add a new cluster with basic authentication support to kubeconf
$ kubectl config set-credentials kubeuser/foo.kubernetes.com --username=kubeuser --password=kubepassword
# Set context with specified username and namespace
$ kubectl config set-context gce --user=cluster-admin --namespace=foo \
&& kubectl config use-context gce
Creating Objects
<span>.yaml</span>
, <span>.yml</span>
, or <span>.json</span>
.# Create resources
$ kubectl create -f ./my-manifest.yaml
# Create resources using multiple files
$ kubectl create -f ./my1.yaml -f ./my2.yaml
# Create resources using all manifest files in a directory
$ kubectl create -f ./dir
# Create resources using url
$ kubectl create -f https://git.io/vPieo
# Start an nginx instance
$ kubectl run nginx --image=nginx
# Get documentation for pod and svc
$ kubectl explain pods,svc
# Create multiple YAML objects from stdin
$ cat <<eof "1000"="" "1000000"="" "jane"="" "s33msi4"="" #="" $="" $(echo="" -="" ---="" -f="" <<eof="" a="" apiversion:="" args:="" base64)="" busybox="" busybox-sleep="" busybox-sleep-less="" cat="" code="" containers:="" create="" data:="" eof="" eof<="" image:="" keys="" kind:="" kubectl="" metadata:="" mysecret="" name:="" opaque="" password:="" pod="" secret="" several="" sleep="" spec:="" type:="" username:="" v1="" with="" |=""></eof>
Displaying and Finding Resources
# Get commands with basic output
# List all services in all namespaces
$ kubectl get services
# List all pods in all namespaces
$ kubectl get pods --all-namespaces
# List all pods and show detailed information
$ kubectl get pods -o wide
# List specified deployment
$ kubectl get deployment my-dep
# List all pods in the namespace including uninitialized ones
$ kubectl get pods --include-uninitialized
# Describe command with detailed output
$ kubectl describe nodes my-node
$ kubectl describe pods my-pod
# List Services Sorted by Name
$ kubectl get services --sort-by=.metadata.name
# List pods sorted by restart count
$ kubectl get pods --sort-by='.status.containerStatuses[0].restartCount'
# Get version label of all pods with app=cassandra
$ kubectl get pods --selector=app=cassandra rc -o \
jsonpath='{.items[*].metadata.labels.version}'
# Get ExternalIP of all nodes
$ kubectl get nodes -o jsonpath='{.items[*].status.addresses[?(@.type=="ExternalIP")].address}'
# List names of Pods belonging to a specific RC
# The "jq" command is used to convert complex jsonpath, refer to https://stedolan.github.io/jq/
$ sel=${$(kubectl get rc my-rc --output=json | jq -j '.spec.selector | to_entries | .[] | "
(.key)=
(.value),"')%?}$ echo $(kubectl get pods --selector=$sel --output=jsonpath={.items..metadata.name})
# Check which nodes are ready
$ JSONPATH='{range .items[*]}{@.metadata.name}:{range @.status.conditions[*]}{@.type}={@.status};{end}{end}' \
&& kubectl get nodes -o jsonpath="$JSONPATH" | grep "Ready=True"
# List Secrets used in current Pod
$ kubectl get pods -o json | jq '.items[].spec.containers[].env[]?.valueFrom.secretKeyRef.name' | grep -v null | sort | uniq
Updating Resources
$ kubectl rolling-update frontend-v1 -f frontend-v2.json # Rolling update pod frontend-v1
$ kubectl rolling-update frontend-v1 frontend-v2 --image=image:v2 # Update resource name and image
$ kubectl rolling-update frontend --image=image:v2 # Update image in frontend pod
$ kubectl rolling-update frontend-v1 frontend-v2 --rollback # Rollback existing ongoing rolling update
$ cat pod.json | kubectl replace -f - # Replace pod based on stdin input JSON
# Force replace, delete and recreate resource. It will cause service interruption.
$ kubectl replace --force -f ./pod.json
# Create service for nginx RC, enabling local port 80 to connect to port 8000 on container
$ kubectl expose rc nginx --port=80 --target-port=8000
# Update the image version (tag) of single-container pod to v4
$ kubectl get pod mypod -o yaml | sed 's/(image: myimage):.*$/\1:v4/' | kubectl replace -f -
# Add labels
$ kubectl label pods my-pod new-label=awesome # Add annotations
$ kubectl annotate pods my-pod icon-url=http://goo.gl/XXBTWq # Autoscale deployment "foo"
$ kubectl autoscale deployment foo --min=2 --max=10
Patching Resources
Use strategic merge patch and patch resources.
# Partially update node
$ kubectl patch node k8s-node-1 -p '{"spec":{"unschedulable":true}}'
# Update container image; spec.containers[*].name is required as it is the merge key
$ kubectl patch pod valid-pod -p '{"spec":{"containers":[{"name":"kubernetes-serve-hostname","image":"new image"}]}}'
# Use json patch with positional array to update container image
$ kubectl patch pod valid-pod --type='json' -p='[{"op": "replace", "path": "/spec/containers/0/image", "value":"new image"}]'
# Use json patch with positional array to disable livenessProbe of deployment
$ kubectl patch deployment valid-deployment --type json -p='[{"op": "remove", "path": "/spec/template/spec/containers/0/livenessProbe"}]'
Editing Resources
Edit any API resource in an editor.
# Edit service named docker-registry
$ kubectl edit svc/docker-registry # Use other editor
$ KUBE_EDITOR="nano" kubectl edit svc/docker-registry
Scale Resources
# Scale a replicaset named 'foo' to 3
$ kubectl scale --replicas=3 rs/foo # Scale a resource specified in "foo.yaml" to 3
$ kubectl scale --replicas=3 -f foo.yaml # If the deployment named mysql's current size is 2, scale mysql to 3
$ kubectl scale --current-replicas=2 --replicas=3 deployment/mysql # Scale multiple replication controllers
$ kubectl scale --replicas=5 rc/foo rc/bar rc/baz
Deleting Resources
# Delete pod defined by type and name in pod.json file
$ kubectl delete -f ./pod.json # Delete pod named "baz" and service named "foo"
$ kubectl delete pod,service baz foo # Delete pods and services with name=myLabel label
$ kubectl delete pods,services -l name=myLabel # Delete pods and services with name=myLabel label, including uninitialized ones
$ kubectl delete pods,services -l name=myLabel --include-uninitialized # Delete all pods and services in my-ns namespace
$ kubectl -n my-ns delete po,svc --all
Interacting with Running Pods
# Dump output pod logs (stdout)
$ kubectl logs my-pod # Dump output pod container logs (stdout, use when pod has multiple containers)
$ kubectl logs my-pod -c my-container # Stream output pod logs (stdout)
$ kubectl logs -f my-pod # Stream output pod container logs (stdout, use when pod has multiple containers)
$ kubectl logs -f my-pod -c my-container
# Run pod interactively shell
$ kubectl run -i --tty busybox --image=busybox -- sh # Connect to running container
$ kubectl attach my-pod -i # Forward port 6000 in pod to local port 5000
$ kubectl port-forward my-pod 5000:6000 # Execute command in existing container (when there is only one container)
$ kubectl exec my-pod -- ls / # Execute command in existing container (when pod has multiple containers)
$ kubectl exec my-pod -c my-container -- ls / # Show metrics for specified pod and container
$ kubectl top pod POD_NAME --containers
Interacting with Nodes and Clusters
# Mark my-node as unschedulable
$ kubectl cordon my-node # Drain my-node for maintenance
$ kubectl drain my-node # Mark my-node as schedulable
$ kubectl uncordon my-node # Show metrics for my-node
$ kubectl top node my-node
$ kubectl cluster-info # Output current cluster state to stdout
$ kubectl cluster-info dump # Output current cluster state to /path/to/cluster-state
$ kubectl cluster-info dump --output-directory=/path/to/cluster-state # If the key and affected taint exists, replace with specified value
$ kubectl taint nodes foo dedicated=special-user:NoSchedule
Set Command
<span>kubectl set --help</span>
to see its subcommands, <span>env</span>
, <span>image</span>
, <span>resources</span>
, <span>selector</span>
, <span>serviceaccount</span>
, <span>subject</span>
.Kubectl Set Resources Command
Available resource objects include (case insensitive): replicationcontroller, deployment, daemonset, job, replicaset.
For example:
# Limit cpu of nginx container in deployment to "200m", set memory to "512Mi"
$ kubectl set resources deployment nginx -c=nginx --limits=cpu=200m,memory=512Mi
# Set Requests and Limits for all nginx containers
$ kubectl set resources deployment nginx --limits=cpu=200m,memory=512Mi --requests=cpu=100m,memory=256Mi
# Remove compute resource values from nginx containers
$ kubectl set resources deployment nginx --limits=cpu=0,memory=0 --requests=cpu=0,memory=0
Kubectl Set Selector Command
<span>selector</span>
(selector). If a selector already exists before calling the “set selector” command, the newly created selector will override the original selector.<span>selector</span>
must start with a letter or number, contain a maximum of 63 characters, and can use: letters, numbers, hyphen ” – “, dot “.” and underscore ” _ “. If a resource-version is specified, the update will use this resource version; otherwise, the existing resource version will be used.Syntax: selector (-f FILENAME | TYPE NAME) EXPRESSIONS [—resource-version=version]
Kubectl Set Image Command
Used to update the container image of existing resources.
Available resource objects include:<span>pod (po)</span>
, <span>replicationcontroller (rc)</span>
, <span>deployment (deploy)</span>
, <span>daemonset (ds)</span>
, <span>job</span>
, <span>replicaset (rs)</span>
.
Syntax: image (-f FILENAME | TYPE NAME) CONTAINER_NAME_1=CONTAINER_IMAGE_1 … CONTAINER_NAME_N=CONTAINER_IMAGE_N
# Set nginx container image in deployment to "nginx:1.9.1"
$ kubectl set image deployment/nginx busybox=busybox nginx=nginx:1.9.1
# Update nginx container image for all deployments and rc to "nginx:1.9.1"
$ kubectl set image deployments,rc nginx=nginx:1.9.1 --all
# Update all container images in daemonset abc to "nginx:1.9.1"
$ kubectl set image daemonset abc *=nginx:1.9.1
# Update nginx container image from local file
$ kubectl set image -f path/to/file.yaml nginx=nginx:1.9.1 --local -o yaml
Resource Types
Formatted Output
<span>-o</span>
or <span>--output</span>
flag to the <span>kubectl</span>
command.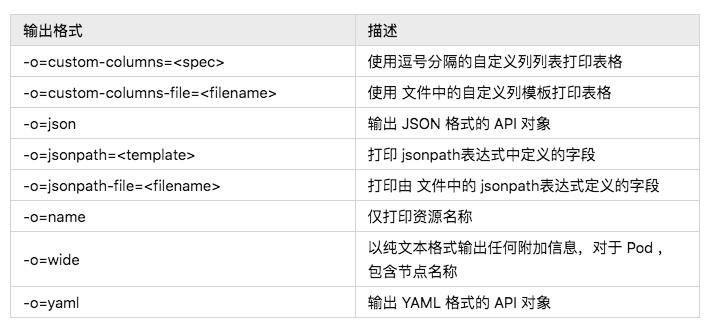
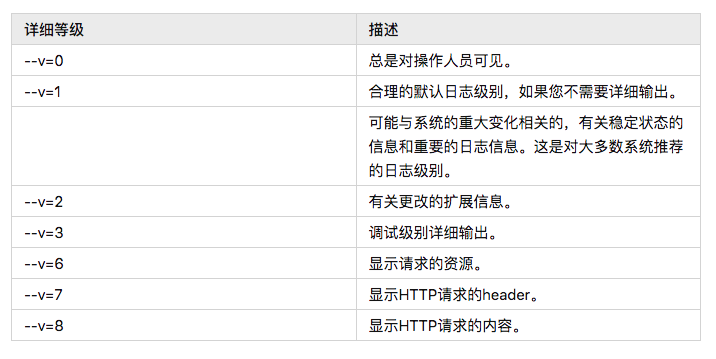
<span>-v</span>
or <span>--v</span>
flag followed by an integer to specify the log level.– END –
Recommended Reading
31 Days to Obtain the Most Valuable CKA+CKS Certificate!
Super Vim Editor Usage Tips
16 Diagrams to Hardcore Explain Kubernetes Networking
The Most Comprehensive Jenkins Pipeline Explanation
Share Several Kubernetes Logic and Architecture Diagrams
9 Practical Shell Scripts, Recommended to Collect!
Good Habits Summary for Linux Operations
Several Essential Linux Operation Scripts!
40 Frequently Asked Interview Questions on Nginx
This Article Will Help You Fully Master Nginx!
Kubernetes Network Troubleshooting Hardcore Chinese Guide
Dockerfile Customizing Exclusive Images, Super Detailed!
Mainstream Monitoring System Prometheus Learning Guide
Master Ansible Automation Operations in One Article
100 Classic Linux Shell Script Cases (with PDF)
Build a Complete Enterprise-level K8s Cluster (Binary Method)
Light Up, Server Runs Without Downtime for Three Years