
module check(rst_i,clk_i,data_i,data_o); input rst_i,clk_i; input data_i; output data_o; reg[3:0] current_state,next_state; parameter[3:0] idle="0000", state1="0001", state2="0010", state3="0100", state4="1000"; always@(posedge clk_i or negedge rst_i)if (!rst_i) current_state <= idle; else current_state <= next_state;always@(current_state,data_i)case(current_state)idle : if (data_i==1)next_state=state1;else next_state=idle;state1: if (data_i==0)next_state=state2;else next_state=idle;state2: if (data_i==0)next_state=state3;else next_state=idle;state3: if (data_i==1)next_state=state4;else next_state=idle;state4: if (data_i==0)next_state=idle;else next_state=idle;endcaseassign data_o= (current_state==state4);endmodule
library ieee;use ieee.std_logic_1164.all;use ieee.std_logic_arith.all;use ieee.std_logic_unsigned.all;entity check is port( rst_i :in std_logic; clk_i :in std_logic;data_i:in std_logic;data_o:out std_logic);end entity;architecture behave of check is type state is (state_a,state_b,state_c,state_d,state_e); signal current_state:state; signal next_state:state;begin process(rst_i,clk_i)beginif rst_i='1' then data_o <='0'; current_state <= state_a;elsif rising_edge(clk_i) then current_state <= next_state;end if;end process; process(current_state,data_i)begincase current_state is when state_a => if data_i='1' then next_state <= state_b;else next_state <= state_a;end if; when state_b => if data_i='0' then next_state <= state_c;else next_state <= state_a;end if; when state_c => if data_i='0' then next_state <= state_d;else next_state <= state_a;end if; when state_d => if data_i='1' then next_state <= state_e;else next_state <= state_a;end if; when state_e => if data_i='0' then next_state <= state_a; else next_state <= state_a;end if; when others => null;end case; end;data_o <='1' when current_state = state_e else '0'; end;

module divide2( clk , clk_o, reset); input clk , reset; output clk_o; wire in; reg out ; always @ ( posedge clk or posedge reset)if ( reset)out <= 0;elseout <= in;assign in = ~out;assign clk_o = out; endmodule
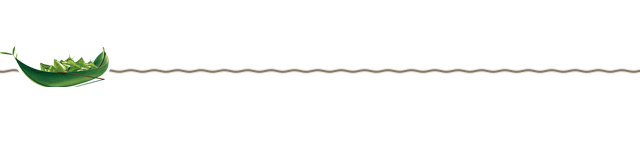
module dff8(clk , reset, d, q);input clk;input reset;input [7:0] d;output [7:0] q;reg [7:0] q;always @ (posedge clk or posedge reset)if(reset)q <= 0;elseq <= d;endmodule

module dff8(clk , reset, d, q);input clk;input reset;input d;output q;reg q;always @ (posedge clk or posedge reset)if(reset)q <= 0;elseq <= d;endmodule


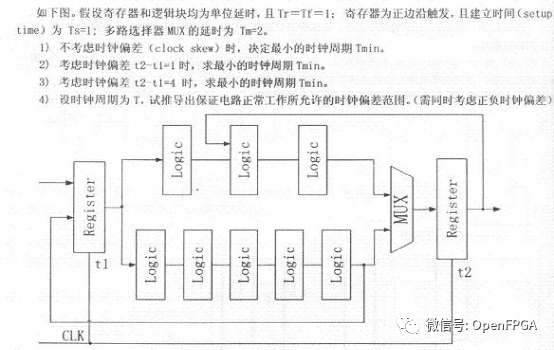

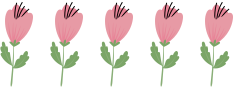
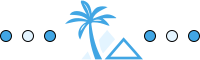
input a,b;output c;assign c=a?(~b):(b);



module dff8(clk , reset, d, q);input clk;input reset;input [7:0] d;output [7:0] q;reg [7:0] q;always @ (posedge clk or posedge reset)if(reset)q <= 0;elseq <= d;endmodule

module divide2( clk , clk_o, reset); input clk , reset; output clk_o; wire in;reg out ; always @ ( posedge clk or posedge reset)if ( reset)out <= 0;elseout <= in;assign in = ~out;assign clk_o = out; endmodule

State transition diagram:
Code design:

FIFO stands for First In First Out memory, and FIFO controllers are widely used in digital systems as data buffers.
The clock-synchronized FIFO controller interface is shown below, with the main interface signal definitions as follows:
RST_N: Asynchronous reset signal. When RST_N is low, FULL outputs ‘0’, EMPTY outputs ‘1’, and the FIFO pointer points to 0, indicating that the FIFO is cleared;
CLK: Clock signal, output signals are synchronized with CLK;
DATAIN: Data input signal, 8-bit bus;
RD: Read valid signal, high level valid. When RD is high, at the rising edge of the clock signal CLK, DATAOUT outputs a valid 8-bit data;
WR: Write valid signal. When WR is high, at the rising edge of CLK, a valid 8-bit data is written from DATAIN to memory;
DATAOUT: Data output signal, 8-bit bus. At the rising edge of CLK, when RD is high, outputs an 8-bit data from FIFO;
FULL: Memory full flag signal. High level indicates that the data in memory has been fully written;
EMPTY: Memory empty flag signal. High level indicates that the data in memory has been read empty.
Requirement: Write an 8×16 FIFO in Verilog to complete the FIFO function, and output EMPTY valid signal when FIFO is empty. The read pointer RP should not move anymore; when FIFO is full, output FULL valid signal, and even if WR is valid, it should not write data into the memory unit (the write pointer WP should not move).
The storage unit is modeled using a two-dimensional array. Note that the address of the storage unit should be able to return to the lowest value when written to or read from the highest address.
module fifo_mem(data,clk,rstN,wrN,rdN,empty,full);inout [7:0] data;input clk,rstN,wrN,rdN;output empty,full;reg [4:0] _cntr,rd_cntr;wire [3:0] add;ram16X8 ram(.data(data),.addr(addr),.wrN(wrN),.oe(wrN));always @(posedge clk or negedge rstN)if(!rstN) wr_cntr<=0;else if (!wrN) wr_cntr<=wr_cntr+1;always @ (posedge clk or negedge rstN) if(!rstN) rd_cntr<=0; else if(!rdN) rd_cntr<=rd_cntr+1;assign addr=wrN?rd_cntr [3:0]: wr_cntr [3:0];assign empty=(wr_cntr [3:0] == rd_cntr [3:0])&&!(wr_cntr[4]^rd_cntr[4]);assign full=(wr_cntr [3:0] ==rd_cntr [3:0])&&(wr_cntr[4]^rd_cntr[4]);endmodule
18 Design a presettable initial value 7-segment cyclic counter and a 15-segment one using your familiar design method.
module counter7(clk,rst,load,data,cout);input clk,rst,load;input [2:0] data;output reg [2:0] cout;always@(posedge clk)beginif(!rst)cout<=3’d0;else if(load)cout<=data;else if(cout>=3’d6) cout<=3’d0;else cout<=cout+3’d1;endendmodule
– END –
NOW take action!