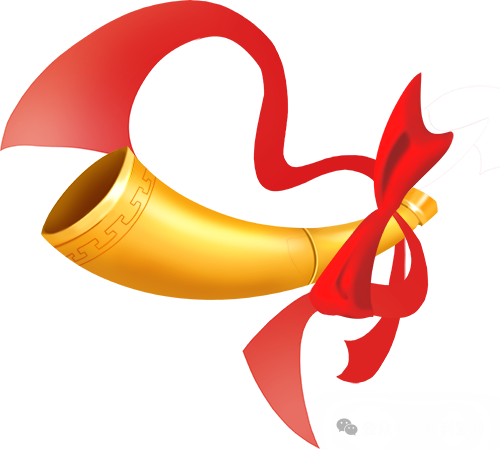
Core Technologies for IoT Device Development
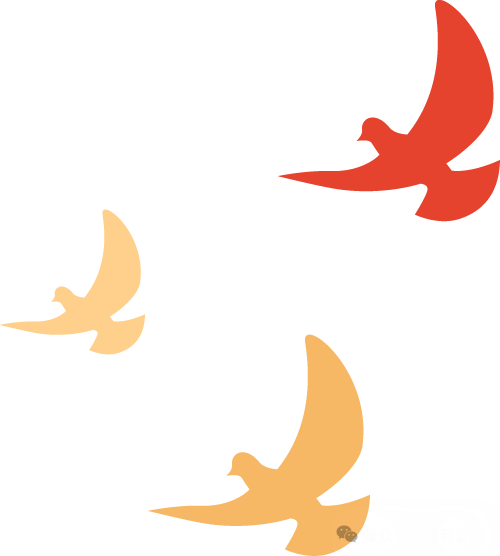
Hello, dear C++ friends, it’s great to meet you again! Today, we will explore the application of C++ in embedded systems, especially how to use C++ to develop IoT devices. Are you ready? Let’s embark on this exciting learning journey!
1. Introduction to C++ and Embedded Systems
In the world of IoT, embedded systems serve as the bridge between the physical and digital worlds. C++, with its high performance and flexibility, has become the programming language of choice for many embedded systems and IoT devices. C++ not only provides close-to-hardware control but also ensures the portability and maintainability of the code.
2. Application of Smart Pointers in Embedded Systems
In embedded systems, resource management is crucial, and smart pointers can help us manage memory better and avoid memory leaks. Let’s take a closer look at std::unique_ptr
, std::shared_ptr
, and std::weak_ptr
.
std::unique_ptr
<span>std::unique_ptr</span>
represents a pointer with unique ownership, ensuring that only one smart pointer can own an object at a time. This is very useful in embedded systems; for example, when handling sensor data, we can ensure that the data is not accessed by other threads during processing.
// Using std::unique_ptr to manage resources
#include <memory>
#include <iostream>
class SensorData {
public:
SensorData() { std::cout << "SensorData created" << std::endl; }
~SensorData() { std::cout << "SensorData destroyed" << std::endl; }
};
void processSensorData(std::unique_ptr<SensorData> data) {
// Process sensor data
}
int main() {
std::unique_ptr<SensorData> sensorData = std::make_unique<SensorData>();
processSensorData(std::move(sensorData)); // Transfer ownership to processSensorData
// sensorData is no longer valid here as ownership has been transferred
return 0;
}
Note: <span>std::unique_ptr</span>
manages resources through the RAII (Resource Acquisition Is Initialization) pattern, automatically releasing resources when the smart pointer goes out of scope.
std::shared_ptr
<span>std::shared_ptr</span>
represents a pointer with shared ownership, managing the object’s lifecycle through reference counting. In embedded systems, multiple components may need to share the same resource, and <span>std::shared_ptr</span>
ensures that the resource is released only after the last reference is destroyed.
// Using std::shared_ptr to share resources
#include <memory>
#include <iostream>
class Resource {
public:
Resource() { std::cout << "Resource created" << std::endl; }
~Resource() { std::cout << "Resource destroyed" << std::endl; }
};
int main() {
std::shared_ptr<Resource> res1 = std::make_shared<Resource>();
std::shared_ptr<Resource> res2 = res1; // Share the same resource
// Both smart pointers go out of scope, but the resource won't be destroyed until the last reference is destroyed
return 0;
}
Note: <span>std::shared_ptr</span>
ensures that resources are released only after all references are destroyed through reference counting.
std::weak_ptr
<span>std::weak_ptr</span>
is a smart pointer that does not control the object’s lifecycle, and it can be used to solve the circular reference problem of <span>std::shared_ptr</span>
. In embedded systems, <span>std::weak_ptr</span>
can be used to implement caching mechanisms to avoid memory leaks.
// Using std::weak_ptr to avoid circular references
#include <memory>
#include <iostream>
class Cache {
public:
std::weak_ptr<int> data;
void update(std::shared_ptr<int> newData) {
data = newData;
}
std::shared_ptr<int> getData() {
return data.lock(); // Try to get a shared_ptr
}
};
int main() {
std::shared_ptr<int> data = std::make_shared<int>(10);
Cache cache;
cache.update(data);
auto lockedData = cache.getData();
if (lockedData) {
std::cout << "Data: " << *lockedData << std::endl;
}
return 0;
}
Note: <span>std::weak_ptr</span>
does not increase the reference count, allowing it to observe resources managed by <span>std::shared_ptr</span>
without increasing their reference count.
3. Application Scenarios of C++ in Embedded Systems
C++ is widely used in embedded systems, from simple microcontrollers to complex IoT gateways, providing robust support. Here are some common application scenarios:
- Microcontroller Programming
: C++ can be used to write firmware for microcontrollers, implementing hardware control and data processing. - Real-Time Operating Systems
: C++ can be combined with real-time operating systems (RTOS) to implement real-time task scheduling and resource management. - Network Communication
: C++ can be used to implement network communication for IoT devices, including protocols like MQTT and CoAP.
4. Best Practices for C++ Embedded Programming
Best practices for C++ in embedded system development include:
- Resource Constraints
: Embedded devices often have limited resources, requiring optimization of memory and CPU usage. - Portability
: Code should be portable to adapt to different hardware platforms. - Security
: Security is crucial in embedded systems, ensuring the safety and stability of the code.
Conclusion
Alright, dear friends, that’s it for today’s learning about C++ in embedded systems! We explored the applications of C++, especially the use of smart pointers, as well as some common application scenarios and best practices. Remember to get hands-on with coding, and feel free to ask questions in the comments. Wishing you a happy learning experience and continuous improvement in C++!