Python Video On-Demand System Development Tutorial
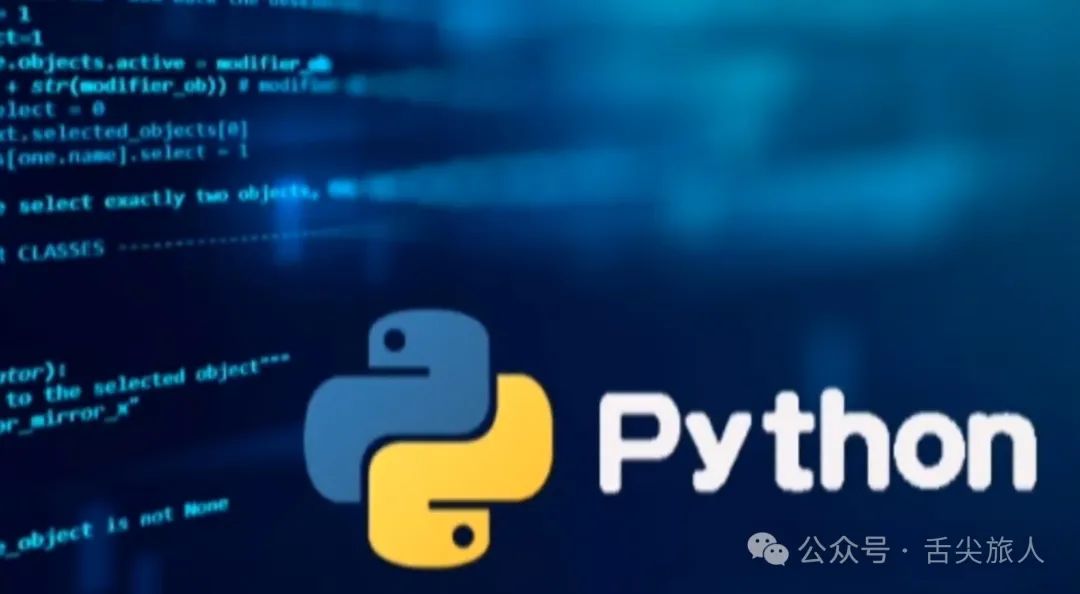
Hello everyone! Today I want to share with you how to develop a simple video on-demand system using Python. This system, although simple, includes many practical features: video file management, user login registration, playback history, etc. Through this project, you will not only learn important knowledge such as file operations, database usage, and object-oriented programming, but also understand how an actual system is organized and operated. Even if you are a beginner in Python, as long as you follow my steps, you can easily complete this interesting project.
1. Overview of System Design
Our video on-demand system mainly includes the following functional modules:
-
User Management: Registration, Login, Password Change -
Video Management: Upload, Delete, Query Videos -
Playback History: Record Viewing History, Save Playback Progress -
Video Playback: Basic Playback, Pause, Progress Adjustment Functions
First, let’s take a look at the basic architecture of the system:
# main.py
from database import Database
from user_manager import UserManager
from video_manager import VideoManager
class VideoSystem:
def __init__(self):
self.db = Database('video_system.db')
self.user_manager = UserManager(self.db)
self.video_manager = VideoManager(self.db)
def run(self):
print("Welcome to the Video On-Demand System")
while True:
self.show_menu()
choice = input("Please select a function:")
self.handle_choice(choice)
def show_menu(self):
print("\n1. User Login")
print("2. User Registration")
print("3. Video List")
print("4. Exit System")
2. Database Module
This module is responsible for all data storage and retrieval. We use SQLite database, which is lightweight and easy to use.
# database.py
import sqlite3
class Database:
def __init__(self, db_name):
self.conn = sqlite3.connect(db_name)
self.cursor = self.conn.cursor()
self.init_tables()
def init_tables(self):
# Create user table
self.cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
username TEXT UNIQUE,
password TEXT
)
''')
# Create video table
self.cursor.execute('''
CREATE TABLE IF NOT EXISTS videos (
id INTEGER PRIMARY KEY,
title TEXT,
path TEXT,
duration INTEGER
)
''')
self.conn.commit()
❝
Tip: Using a context manager (with statement) can ensure that the database connection is properly closed, avoiding resource leaks.
3. User Management Module
This module handles all user-related operations:
# user_manager.py
import hashlib
class UserManager:
def __init__(self, db):
self.db = db
def register(self, username, password):
# Encrypt and store password
hashed_password = hashlib.sha256(password.encode()).hexdigest()
try:
self.db.cursor.execute(
"INSERT INTO users (username, password) VALUES (?, ?)" ,
(username, hashed_password)
)
self.db.conn.commit()
return True
except sqlite3.IntegrityError:
return False
def login(self, username, password):
hashed_password = hashlib.sha256(password.encode()).hexdigest()
self.db.cursor.execute(
"SELECT * FROM users WHERE username=? AND password=?",
(username, hashed_password)
)
return self.db.cursor.fetchone() is not None
❝
Note: In actual projects, a more secure password encryption method should be used, such as bcrypt or Argon2. Here, sha256 is used only for demonstration.
4. Video Management Module
The video management module is responsible for handling the addition, deletion, modification, and querying of video files:
# video_manager.py
import os
class VideoManager:
def __init__(self, db):
self.db = db
def add_video(self, title, file_path):
# Check if the file exists
if not os.path.exists(file_path):
return False
try:
self.db.cursor.execute(
"INSERT INTO videos (title, path) VALUES (?, ?)" ,
(title, file_path)
)
self.db.conn.commit()
return True
except Exception as e:
print(f"Failed to add video: {e}")
return False
def get_video_list(self):
self.db.cursor.execute("SELECT id, title FROM videos")
return self.db.cursor.fetchall()
Practical Exercises
-
Try to add the following features to the system:
-
Video Classification Feature -
User Favorites Feature -
Playback History
Consider how to optimize the system:
-
How to handle large file uploads? -
How to implement online video streaming? -
How to improve system security?
Summary
Through this project, we learned:
-
Basic usage of SQLite database -
Practical application of object-oriented programming -
File operations and exception handling -
Basic system design concepts
Remember, the most important thing in programming is hands-on practice. I recommend that you first build this basic system, and then gradually add new features. If you encounter problems, you can check the official Python documentation or seek help in the development community.
Happy coding! Remember to practice more to improve your coding skills!